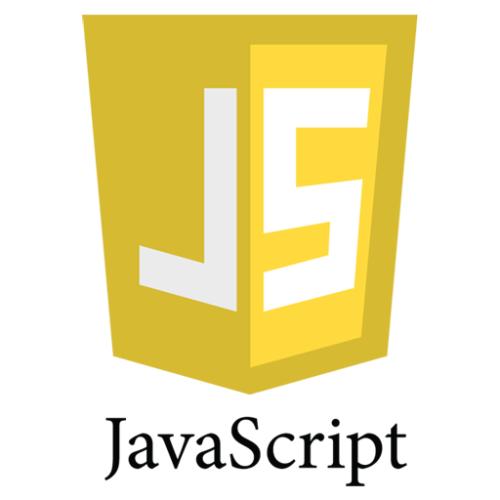
Using JavaScript technology to process statistics in browsers has the best dissemination effect
A class is a collection of common attributes and methods.JS is an object-oriented language whose objects as objects are simulated and encapsulated using the prototype property.
The class of JS is actually a function function, which is also called pseudo class because it is not a typical object-oriented class.Understanding the classes of JS requires a better understanding of the functions in JS.First, function itself is an object that can be used as either a parameter or a return value of a function, just like a normal object.
1. Constructor Method
[Back]console.clear(); //Constructor to get a oString class var oString = function(str){ this.content = str; }; var name = new oString("Lei"); //Create an object instance var addr = new oString("Lijun"); console.log(name.content + " live in " + addr.content); name.age = oString.prototype; //name object adds age attribute name.age = 18; console.log(name); //Add a gender property for oString oString.prototype.gender = "F"; //Add a toString method for oString oString.prototype.toString = function(){ return this.content; }; console.log(name.toString()); //Lei, the object instance inherits the toString method console.log(name.gender); //Lei, the object instance inherits the gender property console.log(addr.toString()); //Lijun console.log(addr.gender); //F
2.object.create() method
[Back]console.clear(); var Person = { name: 'pawn', sayHello: function() { console.log(this.name); } } Person.age = 28; //Prototype adds properties, note that prototype is not used Person.gender = "F"; Person.toString = function(str){ //Prototype Adding Method return this.name + " " +str +"."; }; var p = Object.create(Person); //Create Object Instance console.log(p.age); //28 p.sayHello(); //pawn console.log(p.toString("hello")); //pawn hello. console.log(p); console.log(Person);
3. ES6 Class Method
[Back]console.clear(); //Define a class (parent class) with the class keyword first class Point{ constructor(x, y){ //Attribute Construction this.x = x; this.y = y; } toString(){ //Creation Method return 'Point coordinates: ' + this.x + ',' + this.y; } } let fp = new Point(12, 34); console.log(fp.x); //x-coordinate: 12 console.log(fp.toString()); //Point coordinates: 12,34 //Declarations of subclasses class ColoredPoint extends Point{ constructor(x, y, color){ ////Attribute construction super(x, y); //x,y inherits parent class this.color = color; //Define color attributes for subclasses } toString(){ //Override method (super denotes parent class) return this.color + super.toString(); } } let sp = new ColoredPoint(56, 78, 'gules'); console.log(sp.x); //56 console.log(sp.color); //gules console.log(sp.toString()); //"Red point coordinates: 56,78"
Inheritance between classes can be achieved through the extends keyword, and a new keyword super appears in the constructor of the subclass, without which instance properties of the parent class cannot be inherited (constructor in the subclass, super inside).Super refers to an instance of the parent class (this object of the parent class), where super(x,y) is the constructor that calls the parent class.super.toString() is the call to the parent toString() method.
This paper introduces several common basic usages of inheritance and encapsulation of JS classes, and recommends using the newer ES6 Class method.Inheritance and encapsulation of JS classes can greatly simplify the statistical analysis work code, provide efficient rules for code reuse and flexible expansion, and are important tools for developing large statistical analysis projects.
Tip: The JS script code on this page can be copied and pasted to JS Code Run Window Debugging experience; Text editing shortcuts: Ctrl+A-Select All; Ctrl+C-Copy; Ctrl+X-Cut; Ctrl+V-Paste
Above: JavaScript Statistics Processing (4) - Conditions and Loops
Below:JavaScript Statistics Processing (6) - New Method for ES6 Array