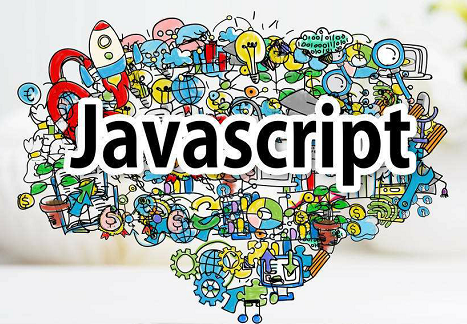
JavaScript
0x01 content introduction
Many friends will encounter various time related problems in the process of development, such as obtaining a specific time stamp, formatting the time object, etc. This article will help you to summarize and solve some common time problems. You can regard it as a dictionary article for future reference and use
0x02 time stamp
const date = new Date(); //Get time object date.getTime(); //Get the current timestamp, the number of milliseconds from zero on January 1, 1970 to now Math.round(date.getTime()/1000); //Gets the current timestamp, rounded to the nearest millisecond Math.floor(date.getTime()/1000); //Get the current time stamp, and round it according to the number of milliseconds Date.parse(date); // Get current timestamp, MS is 0
0x03 get date and time
let date = new Date(); let year = date.getFullYear();//Get the complete year (4 digits, 1970 -????) let month = date.getMonth() + 1;//Get the current month (0-11,0 for January) let day = date.getDate();//Get current day (1-31) let weekDay = date.getDay();//Get current week X(0-6,0 for Sunday) let hour = date.getHours();//Get current hours (0-23) let minute = date.getMinutes();//Get current minutes (0-59) let second = date.getSeconds();//Get current seconds (0-59) let milliseconds = date.getMilliseconds();//Get the current number of milliseconds (0-999) let setWeekDay = ''; switch (weekDay) { case 0: setWeekDay = 'Sunday'; break; case 1: setWeekDay = 'Monday'; break; case 2: setWeekDay = 'Tuesday'; break; case 3: setWeekDay = 'Wednesday'; break; case 4: setWeekDay = 'Thursday'; break; case 5: setWeekDay = 'Friday'; break; case 6: setWeekDay = 'Saturday'; break; } console.log(`Today is ${year}year ${month}month ${day}day ${setWeekDay} ${hour}Time ${minute}branch ${second}second ${milliseconds}Millisecond`); // The following three statements are the results of running in the Node environment. The results of running in the browser are different from this date.toLocaleDateString(); //Acquisition date, YYYY-MM-DD date.toLocaleTimeString(); //Get time, HH:mm:ss date.toLocaleString(); //Get date and time, YYYY-MM-DD HH:mm:ss
0x04 actual combat
Requirement: write a general function or class to obtain the timestamp and time format of the current time or user-defined time, or realize the mutual conversion between the two
class Datetime { constructor(date) { if(date) { if (date instanceof Date) { this.date = date; } else { throw new Error('Constructor parameter error, expected Date()object') } } else { this.date = new Date(); } } // Convert to timestamp transTimeStamp() { return this.date.getTime(); } // Convert to time format YYYY-MM-DD HH:mm:ss transDateTime() { const {date, dateTimeFormat} = this; let year = date.getFullYear();//Get the complete year (4 digits, 1970 -????) let month = dateTimeFormat(date.getMonth() + 1);//Get the current month (0-11,0 for January) let day = dateTimeFormat(date.getDate());//Get current day (1-31) let hour = dateTimeFormat(date.getHours());//Get current hours (0-23) let minute = dateTimeFormat(date.getMinutes());//Get current minutes (0-59) let second = dateTimeFormat(date.getSeconds());//Get current seconds (0-59) return `${year}-${month}-${day} ${hour}:${minute}:${second}` } // Format dateTimeFormat(v) { return v < 10 ? `0${v}` : `${v}` } setDateTime(date) { if(date) { if (date instanceof Date) { this.date = date; } else { throw new Error('setDateTime Function parameter error, expected Date()object') } } else { this.date = new Date(); } } } // Realization const date = new Datetime(); console.log(date.transDateTime()); console.log(date.transTimeStamp()); date.setDateTime(new Date('2017-06-05 12:22:23')); console.log(date.transDateTime()); console.log(date.transTimeStamp());
Please contact the author for authorization