A simple Echo example of WebSocket: The sample code comes from: http://www.websocket.org/echo.html
Using a text editor, copy and save the following code in a websocket.html file, and as soon as you open it in the browser, the page will automatically connect using websocket, send a message, display the received server response, and then close the connection.
<!DOCTYPE html> <meta charset="utf-8" /> <title>WebSocket Test</title> <script language="javascript"type="text/javascript"> var wsUri ="ws://echo.websocket.org/"; var output; function init() { output = document.getElementById("output"); testWebSocket(); } function testWebSocket() { websocket = new WebSocket(wsUri); websocket.onopen = function(evt) { onOpen(evt) }; websocket.onclose = function(evt) { onClose(evt) }; websocket.onmessage = function(evt) { onMessage(evt) }; websocket.onerror = function(evt) { onError(evt) }; } function onOpen(evt) { writeToScreen("CONNECTED"); doSend("WebSocket rocks"); } function onClose(evt) { writeToScreen("DISCONNECTED"); } function onMessage(evt) { writeToScreen('<span style="color: blue;">RESPONSE: '+ evt.data+'</span>'); websocket.close(); } function onError(evt) { writeToScreen('<span style="color: red;">ERROR:</span> '+ evt.data); } function doSend(message) { writeToScreen("SENT: " + message); websocket.send(message); } function writeToScreen(message) { var pre = document.createElement("p"); pre.style.wordWrap = "break-word"; pre.innerHTML = message; output.appendChild(pre); } window.addEventListener("load", init, false); </script> <h2>WebSocket Test</h2> <div id="output"></div> </html>
Main Code Interpretation:
To apply for a WebSocket object, the parameter is the address of the server side that needs to be connected. Like HTTP protocol using http://start, the URL of WebSocket protocol uses ws://start, and the secure WebSocket protocol uses wss://start.
var wsUri ="ws://echo.websocket.org/"; websocket = new WebSocket(wsUri);
WebSocket objects support four messages, onopen, onmessage, onclose and onerror.
We can see that all operations are triggered by messages, so that the UI will not be blocked, making the UI have faster response time and better user experience.
When the connection between Browser and WebSocketServer is successful, the onopen message will be triggered.
websocket.onopen = function(evt) { };
If the connection fails, sending or receiving data fails or processing data errors occur, browser triggers onerror messages.
websocket.onerror = function(evt) { };
When Browser receives the data sent by WebSocket Server, it triggers the onmessage message message. The parameter evt contains the data transmitted by server.
websocket.onmessage = function(evt) { };
When Browser receives a close connection request sent by WebSocket Server, the onclose message is triggered.
websocket.onclose = function(evt) { };
The relationship between WebSocket and TCP, HTTP, WebSocket and HTTP are all based on TCP, so they are reliable protocols. The send function of WebSocket invoked by Web developers in browser implementation is ultimately transmitted through TCP system interface.
WebSocket, like Http, belongs to the application layer protocol, so what is the relationship between them? The answer is yes, when WebSocket establishes handshake connection, data is transmitted through HTTP protocol, but after establishing connection, the real data transmission stage does not need HTTP protocol to participate.
Detailed Interpretation of WebSocket Communication:
As can be seen from the figure below, there are three stages:
- Open the handshake.
- Data transfer
- Close handshake
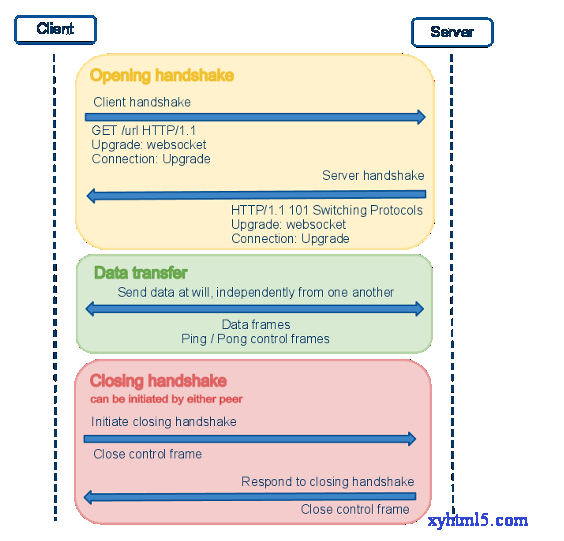
The following figure shows what WebSocket's main three-step browsers and servers do, respectively.
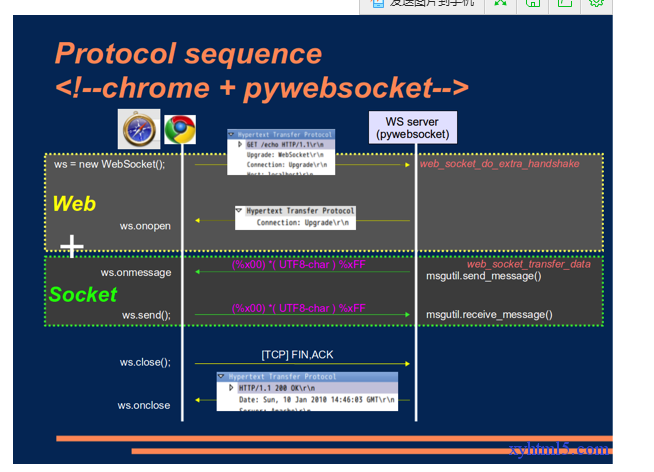
Advantages of WebSocket
The header information exchanged between the server and the client is very small, only about 2 bytes.
b) Both client and server can actively transmit data to each other.
c) Creating TCP requests and destroying requests without frequency can reduce the occupation of network bandwidth resources and save server resources.
A Connected Handshake
When a Web application calls a new WebSocket(url) interface, Browser begins the process of establishing a handshake connection with a Web Server whose address is url.
1. Browser establishes a connection with WebSocket server through three TCP handshakes. If this connection fails, the subsequent process will not be executed and the Web application will receive an error message notification.
2. After TCP is successfully established, Browser/UA transmits the version number supported by WebSocket, the version number of the protocol, the original address, the host address and other column fields to the server through http protocol.
3. After receiving the handshake request from Browser/UA, the WebSocket server accepts the handshake connection and gives the corresponding data reply if the data package and format are correct, the protocol version number of client and server are matched, and so on. Similarly, the reply packet is transmitted by http protocol.
4. When Browser receives the data packet that the server replies to, if the content and format of the data packet are all right, it means that the connection is successful and the onopen message is triggered. At this time, the Web developer can send data to the server through the send interface. Otherwise, the handshake connection fails, and the Web application receives onerror messages and knows why the connection fails.
This handshake looks like HTTP, but it's not. It allows the server to interpret some handshake requests in HTTP and switch to websocket.
data transmission
In WebScoket protocol, data is transmitted in the form of frame sequence.
Considering data security, data frames transmitted from client to server must be masked. If the server receives data frames that have not been masked, it must actively close the connection.
The data frames transmitted from the server to the client must not be masked. If a client receives a masked data frame, it must actively close the connection.
In view of the above situation, the wrong party can send close frame to the other party (the status code is 1002, indicating protocol error) to close the connection.
Close WebSocket (shake hands)
Use the data from the above WebSocket example monitored by Wireshark.
GET / HTTP/1.1 Upgrade: websocket Connection: Upgrade Host: echo.websocket.org Origin: null Pragma: no-cache Cache-Control: no-cache Sec-WebSocket-Key: Qcgtb1RJ6HceeTRLPFux/A== Sec-WebSocket-Version: 13 Sec-WebSocket-Extensions: x-webkit-deflate-frame Cookie: __utma=9925811.951031439.1365242028.1365980711.1366068689.5; __utmc=9925811; __utmz=9925811.1365242028.1.1.utmcsr=websocket.org|utmccn=(referral)|utmcmd=referral|utmcct=/ HTTP/1.1 101 Web Socket Protocol Handshake Upgrade: WebSocket Connection: Upgrade Sec-WebSocket-Accept: 84Qpane33QhxOmcz8bGkFdE1AHk= Server: Kaazing Gateway Date: Tue, 16 Apr 2013 09:51:25 GMT Access-Control-Allow-Origin: null Access-Control-Allow-Credentials: true Access-Control-Allow-Headers: content-type Access-Control-Allow-Headers: authorization Access-Control-Allow-Headers: x-websocket-extensions Access-Control-Allow-Headers: x-websocket-version Access-Control-Allow-Headers: x-websocket-protocol ..a[ J6>h..8a/.{x%.0y..WebSocket rocks..i.....