JavaScript Chapter for Front-end Development
Keywords:
Javascript
Attribute
Python
ECMAScript
Introduction to JavaScript
The front-end three swordsmen's JavaScript, referred to as js, may be the most difficult of the three. Long ago, there were three versions of JS in circulation on the market. In order to unify, ECMA (European Computer Manufacturing Association) defined the standard version, namely EcmaScript.
In fact, ECMAScript is only a part of JavaScript. The complete JavaScript consists of three parts:
Core (ECMAScript)
Document object model
Browser Object Model
Compared with html and css, JS is a mature programming language, and is the only programming language in the front end. Many of the code structures of JS are similar to Java, but there is no very mandatory requirement in the grammar. In use, you will gradually find that this thing is very difficult to report errors. Since it is a programming language, we need to learn from variables, data types, operators, process control statements, functions (not all languages have the concept of functions), classes and objects (js does not have "classes").
ECMAScript (Core)
First, js annotations are the same as Java annotations
// One-line comment
/* Multi-line annotations*/
js code segments are separated by braces, statements are separated by semicolons, and line breaks are defaulted if there is no semicolon.
1. variable
(1) When declaring variables, there is var keyword. If the VaR keyword is not used, it is a global variable. Unless necessary, it is not recommended to use global variables, which will slow down the efficiency.
(2) Variables should be declared before they are defined.
var i;
i=10;
Or it can be abbreviated as var i=10; (This is usually done)
(3) js also supports declaring multiple variables on a single line, and can be of different types
var i=10,j="zhang";
(4) If a variable x is declared without assigning a value to it, it will print undefined instead of reporting an error.
(5) The nomenclature of a variable is not specific to a language. As long as it is a variable, it will involve this problem. A Hungarian type marker is recommended: a lowercase letter is added before the variable name to indicate the type of the variable, and a hump nomenclature is used after the name.
(6) Variable naming: The initials must be one of the letters, underscores or $3, and the rest can be underscores, dollar symbols or any letters, numbers. Avoid switch keywords:
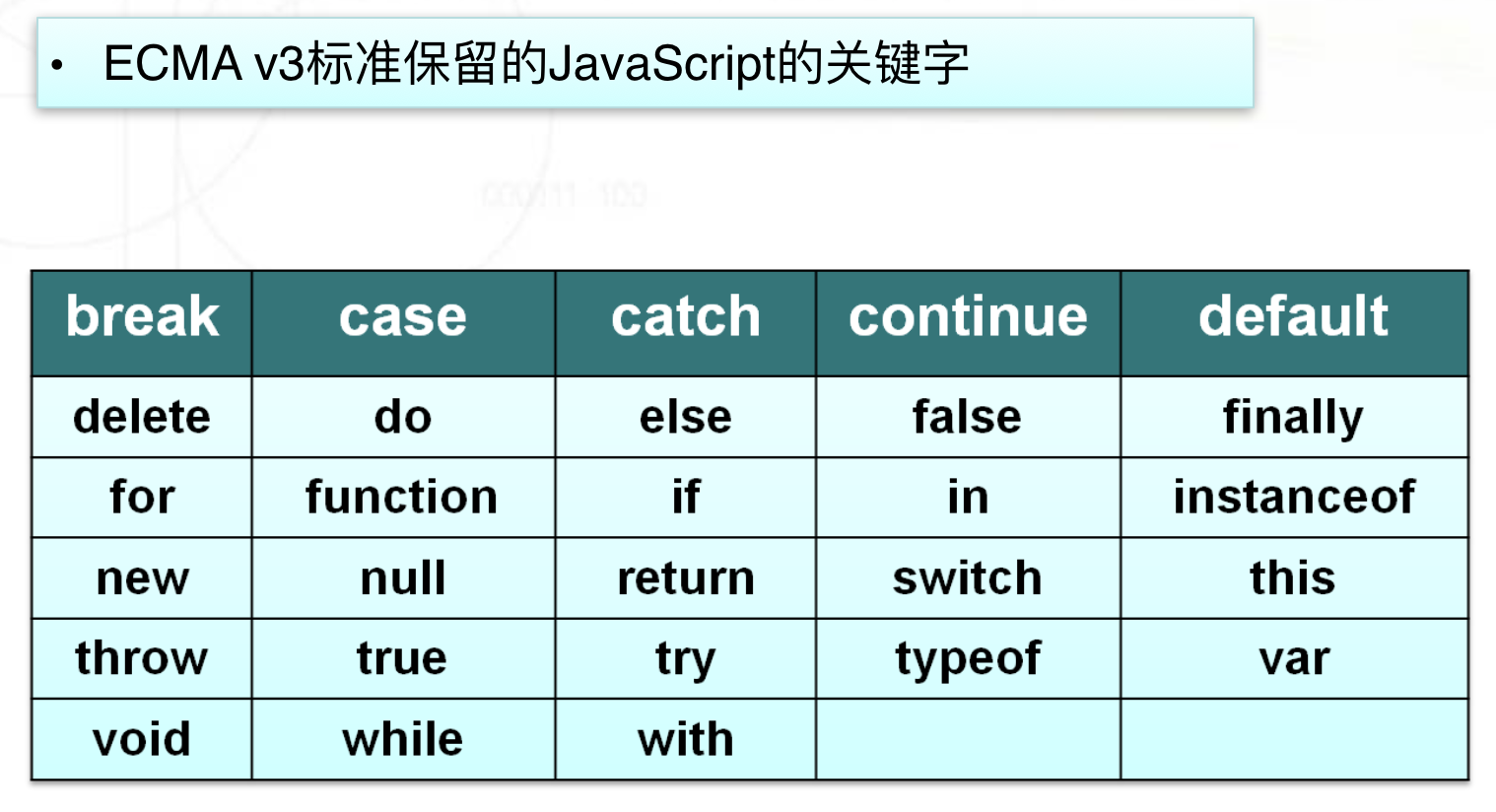
2. Data type
There is no print in js. We can print our desired results with alert or console. log (printed on the browser's console).
Use the typeof command to view data types
var x=10;
console.log(typeof(x));
(1) The data types in js are:
Basic data types: Number, String, Boolean, Null, Undefined
Reference data type:object
There is no list, tuple, dictionary data type in js. List and tuple are implemented by array objects
(2) Number (Number type)
There is no distinction between integer type and floating-point type.
(3) String (string type)
A sequence of unicode characters, numbers, and punctuation symbols, with special characters escaped with backslashes.
(4) Boolean (Boolean type)
Boolean type has only two values, true and false. In practice, true=1, false=0
This is used after if judgement statements. In fact, if statements can be followed by any data type, as long as there is a Boolean value, which is the same as python, but in other languages, if must be followed by an expression.
(5) null and undefined types
The undefined type has only one value, undefined, which is generated when the function does not explicitly return a value, or when the declared variable is not initially recognized.
null often occurs when objects cannot be found.
3. operator
The operators in js are basically the same as those in python.
(1) i++ and ++ I
This one who has learnt c++ must know that there is nothing to say. It should be something like this that comes out for exams.
i++ is assignment before calculation
++ i is first calculated and then assigned
(2)"==="
First, in python, if such a statement
print(2=="2")
The result of printing must be false, because Python is a strongly typed language and does not automatically convert the types of variables, but JS is a weakly typed language. Printing this statement in JS will result in true, because JS automatically converts 2 of numerical type into 2 of string type. Then the problem arises. If in js, it is to judge whether the types are the same, don't. The operator "===" is used for automatic conversion.
In the number type of js, another data type is NaN type, which is the result of invalid string conversion. For example:
var s="hello";
var ret2=+s;
console.log(ret2)
So you get a NaN.
4. Process control
(1) if conditional statement
Unlike Python, there is no elif in js, and multiple branches can only use else if
if (expression){
Execution statement
}
Else if (expression){
Execution statement
}
else{
Execution statement
}
(2) switch-case statement
switch (expression){
case value 1: statement 1;break;
case value 2: statement 2;break;
case value 3: statement 3;break;
default: Statement 4;
}
Note: Value 1, value 2... These are deterministic values, default is the statement executed when none of the above values are values of the expression.
This break is necessary, and without it, these statements will be executed.
(3) while and for loops
Like Python, while loops have three elements: initial variables, judgement conditions, and variable self-addition. Let's say nothing more.
Talk about the for loop
for loop mode 1: conditional loop (most commonly used)
for(var i=0;i<10;i++){
console.log("i")
}
for loop mode 2: traversal loop
var arr=["a","b","c"];
for (var i in arr){
console.log(i)
}
This result prints the index values 0, 1, 2.
If you want to get the value in an array, you can use arr[i]. As for the array object, I'll talk about it later.
This is an array. There is no dictionary in js, but there is a similar way of writing and using it.
var info={"a":123,"b":234,"c":345};
for (var i in info){
console.log(i)
}
In this case, I is the key in the dictionary: a,b,c, and the same value is info[i]
5. objects
After we have learned object-oriented, we should have this idea. When we see the word "object", we immediately think that objects have attributes and methods.
(1) String objects
There are two ways to create it: \\\\\\
Variable = string; var STR1 = Hello World
String object name = new String (string) var str1=new String("hello world")
String objects have one attribute, length attribute
Method: s is a string object
s.toLowerCase() - - Convert to lowercase
s.toUpperCase() - - Convert to uppercase
s.trim() - - Remove the spaces on both sides of the string
s.charAt() - - Gets the character at the specified position, with the character index in parentheses to be retrieved
s.indexOf() - - - - - Query the index position of the specified character, in parentheses for the character to be found
s.lastIndexOf() ----------- The same function as the one above, but the opposite is true.
s.match() - - - - - Returns an array of matched strings, not null
s.seach() ------------ Returns the location index of the first character of the matched string, which does not match to return - 1.
s.substr(start,length) -- Intercepts a specified length string. Start is the index value of the starting position, and length is the length.
s.substring(start,end) intercepts strings. Start is the starting position index, end is the ending position index, and the strings between them
s.slice(start,end) slice string
s.replace(oldstr,newstr) Replace strings, (old, new)
s.split() Split the string and put the result in an array
s.concat(addstr) -- String stitching, parentheses for the string to be stitched
Demonstration:
s="hello";
console.log(s.toLowerCase()); //hello
console.log(s.toUpperCase()); //HELLO
console.log(s.charAt(2)); //l
console.log(s.indexOf("o")); //4
console.log(s.lastIndexOf("o")); //4
console.log(s.match("lo")); //[object Array]["lo"]
console.log(s.match("lol")); //null
console.log(s.search("lo")); //3
console.log(s.search("lol")); //-1
console.log(s.substr(1,3)); //ell
console.log(s.substring(1,3)); //el
console.log(s.slice(1,3)) ; //el
console.log(s.replace("ell","o")) ; //hoo
console.log(s.split("e")) ; //[object Array]["h", "llo"]
console.log(s.concat("world")) ; //helloworld
(2) array objects
Three ways to create arrays:
- var arr=[1,2,3];
- var arr=new Array[1,2,3];
- var arr=new Array(3);
arr[0]=1;
arr[1]=2;
arr[2]=3;
You can also create a two-dimensional array, similar to a list, arr [] [], with the first index as a row and the second index as a column.
join method:
The use of the join method in Python is as follows:
s1="hello"
s2="world"
s3="-".join([s1,s2])
print(s3) #hello-world
In the js array, the reverse is true.
console.log(["hello","world"].join("-")) //hello-world
reverse method and sort method:
Arrays are sorted, reverses are reversed, and sorts are sorted by the ascii code table position of the initials
slice method:
Slice arrays with index values for start and end positions
splice method:
Delete subarrays with several parameters
arr.splice(start,deleteCount,value)
Start stands for the start position (delete after the start position), deleteCount for the number of deleted array elements, and value for the array elements inserted at the delete position (omitable)
push and pop methods:
Adding and deleting elements at the end of an array
unshift and shift methods:
Adding and deleting elements at the beginning of an array
(3) Time object
Create a time object: var timer = new Date()
Get the date and time
getDate() Acquisition date
getDay () Get week
getMonth () Get month (0-11)
getFullYear () Acquire the full year
getYear () Year of acquisition
getHours () Acquisition hours
getMinutes () Get minutes
getSeconds () Get seconds
getMilliseconds () millisecond
getTime () Returns the cumulative milliseconds(From 1970/1/1 Mid-night)
//Setting Date and Time
//setDate(day_of_month) Set date
//setMonth (month) Set month
//setFullYear (year) Set year
//setHours (hour) Set hours
//setMinutes (minute) Set minute
//setSeconds (second) Set seconds
//setMillliseconds (ms) Set milliseconds(0-999)
//setTime (allms) Setting cumulative milliseconds(From 1970/1/1 Mid-night)
var x=new Date();
x.setFullYear (1997); //Setup year 1997
x.setMonth(7); //Set month 7
x.setDate(1); //Set day 1
x.setHours(5); //Set Hour 5
x.setMinutes(12); //Set Minutes 12
x.setSeconds(54); //Set seconds 54
x.setMilliseconds(230); //Set the millisecond 230
document.write(x.toLocaleString( )+"<br>");
//Back to 5:12:54 on August 1, 1997
x.setTime(870409430000); //Set cumulative milliseconds
document.write(x.toLocaleString( )+"<br>");
//Return to 12:23:50 seconds on August 1, 1997
Time object
(4) Math object is to encapsulate built-in functions into math object, which needs to use Math to call methods.
Absolute value of abs(x) return number.
exp(x) returns the exponent of e.
floor(x) logarithms are rounded down.
log(x) returns the natural logarithm of the number (bottom e).
max(x,y) returns the highest values in X and y.
min(x,y) returns the lowest values in X and y.
pow(x,y) returns the Y power of X.
random() returns a random number between 0 and 1.
round(x) rounds the number to the nearest integer.
sin(x) returns the sine of the number.
sqrt(x) returns the square root of the number.
tan(x) returns the tangent of the angle.
(5) Function objects (emphasis)
// Define a function
Function function name (parameter){
Function body
}
// Call function
Function name ()
Note: Function calls in js can be made before they are defined, unlike Python, which is a line-by-line execution, while js compiles them first and then executes them.
On the parameters of a function:
There is an arguments object inside the function, which contains the parameters passed when the function is called.
function add() {
console.log(arguments);
var sum=0;
for (var i=0;i<arguments.length;i++){
sum+=arguments[i] ;
}
console.log(sum);
}
add(1,2,3,5,7);
//[object Arguments]{0: 1, 1: 2, 2: 3, 3: 5, 4: 7, length: 5}
//18
There are also anonymous functions in js, which are often used in later event binding
3. BOM Objects (Browser Objects)
1.windows Objects
In browsers, each open web page is a window, and there are three ways to implement pop-up boxes:
(1) alert(123) pops up "123" in the window and clicks to make sure it disappears
(2)var ret=confirm('zhang');
console.log(ret); "zhang" pops up in the window with two buttons to confirm and cancel, click OK, return a true in the background, click Cancel, and return a false.
(3) var ret=prompt("input a number") pops up an input box in the window, which can also receive user input through a variable.
2.setInterval() and clearInterval()
setInterval() is a continuous call to a function or expression in milliseconds. The first parameter is a function and the second parameter is a time interval.
clearInterval() is to cancel the operation set by setInterval(), the parameter of which is the return value of setInterval().
Example: There are two buttons on the right side of an input box. Click on the start to print the current time in the box every 1 second. Click on stop to stop refreshing.

<body>
<input type="text">
<button class="start">start</button>
<button class="end">Stop it</button>
<script>
ele_start=document.getElementsByClassName("start")[0];//Start button
ele_end=document.getElementsByClassName("end")[0]; //Stop button
function foo() {
var timer=new Date().toString();
var ele=document.getElementsByTagName("input")[0];
ele.value=timer.slice(4,25);//It's the part in the box that shows the best way to see the effect of the code.
}
var ID;//Define a global variable to receive the return value
ele_start.onclick=function () {
if (ID==undefined){ //To solve the problem that the code does not execute at the beginning of the second click bug
foo(); //In order to start with a single hit, the effect of time will appear immediately.
ID=setInterval(foo,1000);
}
}
ele_end.onclick = function () {
clearInterval(ID);
ID=undefined; //Clear the timer and give it back id Reassign
}
</script>
</body>
3.setTimeout() and clearTimeout()
This is to call a function or evaluate an expression after a specified time, in the same way as the two above. The difference is that this will only be executed once.
DOM Objects
In DOM, all objects are node objects, where
The document object is: the entire html document
Element object means that any tag in html is an element object
Two steps are required to perform operations on labels: finding labels and operating labels
1. Find labels
(1) Direct search
document.getElementsByClassName("classname");//according to class Lookup tag
document.getElementsByTagName("elementname");//Search for labels based on label names
document.getElementById("idname"); //according to ID Name Search Label
document.getElementsByName("name"); //according to name Property lookup label
Of the four methods, only document.getElementById("idname") is to find a label, and the other three are to find more than one label. Put the results into an array, which can be retrieved individually by index.
(2) Finding by node attributes
var ele=document.getElementsByClassName("start")[0];
ele.parentElement;// Find the parent node
ele.children; // Find all subtags
ele.firstElementChild; //Find the first sublabel element
ele.lastElementChild; //Find the last sublabel element
ele.nextElementSibling; //Find the next brothers label
ele.previousElementSibling;//Find a Brother Label
It should be noted that there is no direct attribute to find all sibling tags. If you want to find all child tags in DOM, you can traverse all child tags of the parent tag of the operation tag, and then use if condition to judge the method of excluding the current operation tag.
2. Event Binding
Method 1:
<body>
<div>
<button onclick="foo()">click</button>
</div>
<script>
function foo() {
alert(123)
}
</script>
</body>
In this way, js code and html code are mixed together, and the coupling is too strong.
Mode two:
<body>
<div>
<button>click</button>
</div>
<script>
ele_button=document.getElementsByTagName("button")[0];
ele_button.onclick=function () {
alert(123)
}
</script>
</body>
But this method has a problem: js code will be loaded when the page appears, if there are new tags added later, it can not bind this event, because js code has been loaded once, several tags of binding events have been determined, this problem can be easily solved in jQuery, in DOM objects, there are two solutions available. Reference resources:
1. Write label-bound events into functions, the first way to bind events
2. In the tagged code, write the event binding function again
If you don't understand the above, maybe you don't quite understand that the tag you find is an array. Write another example:
<body>
<div>
<button>Add to</button>
<button>delete</button>
<button>edit</button>
</div>
<script>
eles_button=document.getElementsByTagName("button");
//here eles_button It's not a label, it's a collection of three labels.
//Each tag binding event is iterated through the array to get each tag.
for(var i=0;i<eles_button.length;i++){
eles_button[i].onclick=function () {
alert(123)
}
}
</script>
</body>
This example illustrates the above problem again. When binding events, the process of finding tags has been completed, only three tags have been found, but after we add a new button, the code will not refresh again, so the newly added button does not bind events.
3. Tag operation
(1) Operating on class.
ele.classList.add("hide") //Add Tags class
ele.classList.remove("hide") //Remove Tags class
Exercise: Left menu
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
<style>
.left{
width: 20%;
height: 800px;
background: grey;
float: left;
}
.right{
width:80%;
height:800px;
background: navajowhite;
float: left;
}
.title{
background: purple;
margin-top: 10px;
}
ul{
background: green;
}
.hide{
display: none;
}
</style>
</head>
<body>
<div class="left">
<div class="menu">
<div class="title">Menu 1</div>
<ul>
<li>111</li>
<li>111</li>
<li>111</li>
</ul>
</div>
<div class="menu">
<div class="title">Menu two</div>
<ul class="hide">
<li>222</li>
<li>222</li>
<li>222</li>
</ul>
</div>
<div class="menu ">
<div class="title">Menu three</div>
<ul class="hide">
<li>333</li>
<li>333</li>
<li>333</li>
</ul>
</div>
</div>
<div class="right"></div>
<script>
// Lookup tag
var eles_title=document.getElementsByClassName("title");
for (var i=0;i<eles_title.length;i++){
eles_title[i].onclick=function () {
this.nextElementSibling.classList.remove("hide"); //this It's the tag that triggers the event.
var eles_child=this.parentElement.parentElement.children;
for (var j=0;j<eles_child.length;j++){
if(eles_child[j].firstElementChild!=this) {
eles_child[j].lastElementChild.classList.add("hide")//Add to hide Attribute, hide the label
}
}
}
}
</script>
</body>
</html>
4. Node operation
ele.createElement() //Create a node,In parentheses is the label name.
ele.removeChild() //The parent tag deletes a child node with the tag object in parentheses
ele.replaceChild() //Replace the label. ele It's the parent tag, with the old tag object in parentheses and the new tag object in parentheses.
ele.appendChild() //Parent Label Adds Child Label
Here's a separate copy label: cloneNode
<script>
Var ele_copy = document. getElements ByTagName ("div") [0]. cloneNode (); // This is just the outermost node to copy.
Var ele_copy = document. getElements ByTagName ("div") [0]. cloneNode (true); //This is the complete copy.
Here's a bug. If the object being copied has an id attribute, it can still be copied, and the object being copied also has this id.
Another problem is that the binding event of the source tag will not be copied
</script>
5. Get the text in the label
ele.innerText and ele.innerHTML
This is the value, the assignment is
ele.innerText="<a href='#'>click</a>"
ele.innerHTML="<a href='#'>click</a>"
innerText is plain text, but innerHTML will take the element as a label (if the element is a label).
6. Attribute value operation attribute
We usually use labels directly when we take attribute values.
Attribute assignment is a direct label. Attribute=""
But in fact, there is an attribute method.
Attribute value: ele. getAttribute (attribute name)
Attribute assignment: ele. setAttribute (attribute name)
Delete attributes: ele. removeAttribute (attribute name)
7.DOM incident
onclick //Click tab
ondbclick //Double click label
onfocus //Elements get focus
onblur //Elements lose focus
onchange //Domain content changes, often used in form elements
onkeydown //Keyboard keys pressed
onkeypress //A keyboard key is pressed and released.
onkeyup //A keyboard key is released.
onload //A page or an image is loaded.
onmousedown //The mouse button is pressed.
onmousemove //The mouse is moved.
onmouseout //Mouse moves away from an element.
onmouseover //Mouse over an element.
onmouseleave //Mouse leaves from element
onselect //Text is selected.
onsubmit //The confirmation button is clicked.
(1) onchange event
Usually used for form labels, triggering events when the contents of a table are changed (when we select an option)
Exercise: Provincial and Municipal Second-tier Action
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
</head>
<!--Requirements: Two forms, provinces on the left, cities on the right, provinces on the left and cities on the right.-->
<body>
<select name="" id="province">
<option value="">Please choose the province</option>
<option value="">Hebei</option>
<option value="">Shandong</option>
</select>
<select name="" id="city">
<option value="" id="select_city">Please choose the city</option>
</select>
</body>
<script>
var info={
"Hebei":["Beijing","Hengshui"],
"Shandong":["Qingdao","Weifang"]
};
// js Although there is no dictionary in Chinese, it has similar usage.
var ele_pvn=document.getElementById("province");
var ele_city=document.getElementById("city");
var ele_select; //Define a global variable
ele_pvn.onchange=function () {
ele_city.options.length=1; //Keep one item and clear all the rest.
for (var i in info){
if (i==(this.children[this.selectedIndex]).innerText){ //judge
// this.selectedIndex Is the index value of the selected content
for(var j in info[i]){
//The idea is to empty the contents of the cities behind each province, and then rebuild the label of the cities behind according to the selected provinces in the dictionary.
var city_option=document.createElement("option");
city_option.id="selected";
ele_select=document.getElementById("selected");
city_option.innerText=info[i][j];
ele_city.appendChild(city_option);
}
}
}
}
</script>
</html>
(2) onkeydown event for input input box
This event is triggered by any key on the keypad, which is of little significance. If you want to specify the key, what should you do?
An event object is used here. The event object is an object that holds the trigger state of the event and is sent by the operating system.
ele.onkeydown=function (e) {
e=e||window.event; //This code is designed to avoid incompatibility problems with some browsers
console.log(e.keyCode);//This will print the keys. ascii code
console.log(String.fromCharCode(e.keyCode))//This code can be used to ascii Code to character conversion
}
(3) onload event
When it comes to finding tags in script, js code must be written under html code, otherwise it will be wrong to find tags.
But if you want js code on it, you use onload events
Or an application scenario: Browser loading images is actually separate from loading other text labels, and it's a new thread. When there is no speed, there will be mistakes. This event can then be used to allow other tags to wait until the image is loaded.
The usage is as follows:
<script>
window.onload=function () {
var ele=document.getElementsByTagName("div")
ele.style.color="green";
}
</script>
(4) onsubmit event
This event is used for form forms and triggered when the form is submitted. The submit tag in the form tag refreshes the page after clicking and submits the data to the background server (if the action specifies the address), which is the default event of submit itself. The meaning of onsubmit event is to prevent the default event of submit. For example, when a user logs in and enters a username password, the front end can first determine whether the format is correct or not. If the format is incorrect, it is not necessary to send it to the back end.
The onsubmit event must have been triggered before the submit event, otherwise it would be meaningless.
There are two ways to prevent submit default events:
1.return false
2.e.preventDefault()
Exercise: User login validation
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
<style>
div{
margin-top: 30px;
}
</style>
</head>
<body>
<form action="" class="form">
<div id="abd" ><input type="text" placeholder="User name" class="text"></div>
<div><input type="password" placeholder="Password" ></div>
<div id="abc"></div>
<div><input type="submit" value="Submission" class="submit"></div>
</form>
</body>
<script>
var ele_text=document.getElementsByClassName("text")[0];
var ele_submit=document.getElementsByClassName("form")[0];
var ele_abd=document.getElementById("abd");
ele_submit.onsubmit=function () {
if (ele_text.value.length>5){
var ele_warm=document.createElement("h5");
ele_warm.innerText="User name exceeding specified length!";
ele_warm.style.color="red";
ele_warm.style.display="inline";
ele_abd.appendChild(ele_warm);
return false;//prevent submit Default event
}
}
</script>
</html>
(5) onmouseout and onmouseleave events
Both events are triggered when the mouse moves away. The difference is that:
- Whether the mouse pointer leaves the selected element or any child element, the onmouseout event is triggered
- onmouseleave events are triggered only when the mouse pointer leaves the alternate element
The difference between the two can be clarified by a case study of the left menu.
<style>
.container{
width: 300px;
}
.title{
background:green;
line-height: 44px;
text-align: center;
}
.list{
display: none;
}
.list div{
line-height: 40px;
}
.item1{
background: purple;
}
.item2{
background: orange;
}
.item3{
background: greenyellow;
}
</style>
</head>
<body>
<div class="container">
<div class="title">text</div>
<div class="list">
<div class="item1">111</div>
<div class="item2">222</div>
<div class="item3">333</div>
</div>
</div>
<script>
var ele=document.getElementsByClassName("title")[0];
var ele_list=document.getElementsByClassName("list")[0];
var ele_box=document.getElementsByClassName("container")[0];
ele.onmouseover =function () {
ele_list.style.display="block";
};
// ele.onmouseleave =function () {
// ele_list.style.display="none";
// }This is problematic. The mouse goes down and hides.,The box should be bound
ele_box.onmouseleave =function () {
ele_list.style.display="none";
};
</script>
8. Event Dissemination
Parent tag binding event a, child tag binding event b, then when triggering event b, affirmative event a will also trigger, which is called event binding.
What we need to know is how to unbind the event by adding E. stop Propagation to the subtag
<script>
ele_inner.onclick =function (e) {
alert(123);
e.stopPropagation()
}
</script>
Write an example of a horse-running lamp. It feels funny. It rains. I will stay when it rains.

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
</head>
<body>
<h4>Leave me on rainy days and leave me on rainy days</h4>
</body>
<script>
function foo() {
var ele=document.getElementsByTagName("h4")[0];
var first_str= ele.innerText.charAt(0);
var second_str=ele.innerText.slice(1,ele.innerText.length);
var new_str=second_str + first_str;
ele.innerText=new_str
}
setInterval(foo,500)
</script>
</html>
To stay is not to stay.
9. Binding between Labels and Labels
Consider a requirement: the following commodity details, comments and after-sales contents in Jingdong can be displayed by clicking on the buttons of "commodity details", "comments" and "after-sales". How can the corresponding relationship be realized? This applies to custom attributes.
As I said before, we usually use point attributes to get attribute values, but here we can only use getAttribute to get this custom attribute.