1. Built in objects
There are three kinds of objects in JavaScript: custom object, built-in object and browser object
The first two objects are the basic content of JS and belong to ECMAScript; The third browser object is unique to JS. JS API explains that built-in objects refer to some objects of JS language. These objects are used by developers and provide some common or most basic rather than necessary functions (properties and methods). The biggest advantage of built-in objects is to help us develop quickly.
2. Math object
"Math object" is not a constructor, so we don't need new to call it. Instead, use the properties and methods directly.
Property, method name | function |
---|---|
Math.PI | PI |
Math.floor() | Round down |
Math.ceil() | Round up |
Math.round() | Round to the nearest Note - 3.5 The result is - three |
Math.abs() | absolute value |
Math.max()/Math.min() | Find the maximum and minimum values |
Math.random() | Gets a random value in the range [0,1] |
-Gets a random integer in the specified range
function getRandom(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; }
Properties:
PI:
console.log(Math.PI); //3.141592653589793
method:
Min, Max:
//Maximum: //Format: Math.max(value1[,value2,...]) bracket parameters are optional console.log(Math.max(1, 99, 3)); //99 console.log(Math.max(-1, -10)); //-1 console.log(Math.max(1, 99, 'pink teacher')); //NaN (Not a Number) console.log(Math.max()); //-Infinity (negative infinity) //Minimum: //Format: Math.min([value1[,value2,...]]) console.log(Math.min(1, 2, 3)); //1 console.log(Math.min()); //Infinity
Case: encapsulating your own mathematical objects with objects There are maximum and minimum PI values
var myMath = { PI: 3.141592653, max: function() { //arguments does not need to write formal parameters var max = arguments[0]; for (var i = 1; i < arguments.length; i++) { if (arguments[i] > max) { max = arguments[i]; } } return max; }, min: function() { var min = arguments[0]; for (var i = 1; i < arguments.length; i++) { if (arguments[i] < min) { min = arguments[i]; } } return min; } } console.log(myMath.PI); console.log(myMath.max(1, 5, 9)); console.log(myMath.min(1, 5, 9));
Absolute value:
console.log(Math.abs(1)); // 1 console.log(Math.abs(-1)); // 1 console.log(Math.abs('-1')); // Implicit conversion converts string - 1 to number console.log(Math.abs('pink')); // NaN
Three rounding methods:
// (1) Math.floor() rounded the floor down to the minimum console.log(Math.floor(1.1)); // 1 console.log(Math.floor(1.9)); // 1 // (2) Math. Ceil() ceil ceiling rounded up to the maximum value console.log(Math.ceil(1.1)); // 2 console.log(Math.ceil(1.9)); // 2 // (3) Math.round() is rounded. All other numbers are rounded, but. 5 is special. It takes the larger one console.log(Math.round(1.1)); // 1 console.log(Math.round(1.5)); // 2 console.log(Math.round(1.9)); // 2 console.log(Math.round(-1.5)); // The result is - 1
Random number method random()
<script> // 1.Math object random number method random() returns a random floating point number 0 = < x < 1 // 2. There are no parameters in this method // 3. Code verification console.log(Math.random()); // 4. We want to get a random integer between two numbers and include these two integers // Math.floor(Math.random() * (max - min + 1)) + min; function getRandom(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } console.log(getRandom(1, 10)); // 5. Random roll call var arr = ['Zhang San', 'Zhang Sanfeng', 'Zhang San madman', 'Li Si', 'Li sisi', 'pink teacher']; // console.log(arr[0]); console.log(arr[getRandom(0, arr.length - 1)]); </script>
3. Date object
Date object is different from Math object. Date is a constructor, so you need to instantiate it before you can use its specific methods and properties. Date instances are used to process date and time
three point one Instantiate a Date object with Date
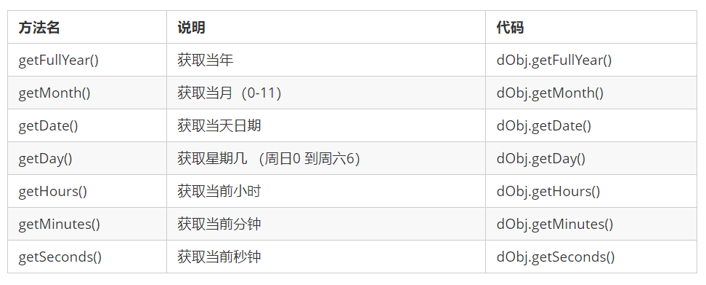
-
Get current time must be instantiated
-
Gets the date object for the specified time
// 1. Use Date. If there is no parameter, return the current time of the current system var date = new Date; console.log(date); //Sun Oct 17 2021 10:16:51 GMT+0800 (China standard time) // 2. The common writing method of parameters is numeric 2021, 10, 01 or string '2021-10-1 8:8:8' var date1 = new Date(2021, 10, 01); console.log(date1); //November is returned instead of October Mon Nov 01 2021 00:00:00 GMT+0800 (China standard time) var date2 = new Date('2021-10-1 8:8:8'); console.log(date2); //Fri Oct 01 2021 08:08:08 GMT+0800 (China standard time)
summary:
1. The date object is different from the Math object. It is a constructor, so we need to instantiate it (new) before using it
2. Date instance is used to process date and time
3. Use of date() method: if there is no parameter, the current time is obtained. If there are parameters, there are two forms: numeric type and string type (common)
3.2 format: mm / DD / yy
//The. getFullYear() method returns the year of the specified date based on the local time. var date = new Date(); console.log(date.getFullYear()); //2021 //The. getMonth() method returns the month of a specified date object according to the local time, which is a value based on 0 (0 represents the first month of the year). var date = new Date(); console.log(date.getMonth() + 1); //Month the returned month is 1 month smaller. Remember month + 1 //. getDate() returns the date of a specified month (from 1 to 31) according to the local time. var dates = new Date(); console.log(dates.getDate()); //17 //The getDay() method returns the day of the week in a specific date according to the local time. 0 represents Sunday. For the day of a month var date = new Date(); console.log(date.getDay()); //0
Case: let's write a Wednesday, May 1, 2019
var date = new Date(); var year = date.getFullYear(); var month = date.getMonth(); var dates = date.getDate(); var day = date.getDay(); var arr = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']; console.log(day); //0 console.log('Today is:' + year + 'year' + month + 'month' + dates + 'day' + ',' + arr[day]); //Today is Sunday, September 17, 2021 console.log('Today is:' + year + 'year' + month + 'month' + dates + 'day ' + arr[day]);
3.3 format date: hour, minute and second
var date = new Date(); console.log(date.getHours()); //Time console.log(date.getMinutes()); //branch console.log(date.getSeconds()); //second
Case: it is required to encapsulate a function to return the current time in the format of hour, minute and second 08:08:08
function getTimer() { var time = new Date(); var h = time.getHours(); h = h < 10 ? '0' + h : h; // The ternary operator is reassigned to the left var m = time.getMinutes(); m = m < 10 ? '0' + m : m; var s = time.getSeconds(); s = s < 10 ? '0' + s : s; return h + ':' + m + ':' + s; } console.log(getTimer());
3.4 get the total milliseconds of the date
Get the total number of milliseconds (timestamp) of Date, Not the number of milliseconds of the current time, but the number of milliseconds since January 1, 1970
// 1. Use valueof() gettime() var date = new Date(); console.log(date.valueOf()); // Is the total number of milliseconds from 1970.1.1 console.log(date.getTime()); // 2. Simple writing method (the most commonly used writing method) var date1 = +new Date(); // +new Date() returns the total number of milliseconds console.log(date1); // 3. The total number of milliseconds added in H5 console.log(Date.now());
Case: countdown effect
4. Array object
4.1 two ways to create arrays
1. Literal mode var arr = [1,"test",true];
var arr = [1, 2, 3]; console.log(arr[0]);
2. Instantiate array object new array() var arr = new Array();
var arr1 = new Array(); // An empty array was created // console.log(arr1); var arr2 = new Array(2); // This 2 indicates that the length of the array is 2 and there are 2 empty array elements in it console.log(arr2); var arr3 = new Array(2, 3); // Equivalent to [2,3], it means that there are two array elements, 2 and 3 console.log(arr3);
Note: in the above code, arr1 creates an empty Array. If you need to use the constructor Array to create a non empty Array, you can pass in parameters when creating the Array.
If only one parameter (number) is passed in, the parameter specifies the length of the array.
If more than one parameter is passed in, the parameter is called an element of the array.
4.2 check whether it is an array
4.2.1 instanceof operator
instanceof can determine whether an object is an instance of a constructor
(1) instanceof Operator, which can be used to detect whether it is an array var arr = []; var obj = {}; console.log(arr instanceof Array); //true console.log(obj instanceof Array); //false
4.2.2 Array.isArray()
Array.isArray() is used to determine whether an object is an Array.isArray() is a method provided in HTML5
(2) Array.isArray(parameter); H5 New method ie9 Supported by the above versions var arr = []; var obj = {}; console.log(Array.isArray(arr)); //true console.log(Array.isArray(obj)); //false
Case: flipping arrays
<script> // Flip array function reverse(arr) { // if (arr instanceof Array) { if (Array.isArray(arr)) { var newArr = []; for (var i = arr.length - 1; i >= 0; i--) { newArr[newArr.length] = arr[i]; } return newArr; } else { return 'error This parameter must be in array format [1,2,3]' } } console.log(reverse([1, 2, 3])); console.log(reverse(1, 2, 3)); //Cannot flip because it is not an array </script>
4.2.3 Pay attention to the usage of typeof
-
typeof is used to determine the type of variable
var arr = [1, 23]; console.log(typeof arr) // object The object arr is an instance of a constructor and is therefore an object data type
4.3 methods of adding and deleting array elements
-
There are methods to add and delete elements in the array. Some methods are shown in the table below 👇
4.3.1 add
1. push() adds one or more array elements to the end of our array push PUSH
var arr = [1, 2, 3]; // arr.push(4, 'pink'); console.log(arr.push(4, 'pink')); //5 console.log(arr); //[1, 2, 3, 4, "pink"] // (1) push is a new element that can be appended to the array // (2) Just write the array element directly with the push () parameter // (3) After the push is completed, the returned result is the length of the new array // (4) The original array will also change
2. unshift add one or more array elements at the beginning of our array
console.log(arr.unshift('red', 'purple')); //7 console.log(arr); // ["red", "purple", 1, 2, 3, 4, "pink"] // (1) unshift is a new element that can be appended to the front of the array // (2) The unshift () parameter can be written directly to the array element // (3) After unshift, the returned result is the length of the new array // (4) The original array will also change
4.3.2 deletion
1. pop() which can delete the last element of the array
console.log(arr.pop()); //pink console.log(arr); // ["red", "purple", 1, 2, 3, 4] // (1) pop is the last element of the array that can be deleted. Remember that only one element can be deleted at a time // (2) pop() has no parameters // (3) After pop, the returned result is the deleted element // (4) The original array will also change
two shift() which deletes the first element of the array
console.log(arr.shift()); //red console.log(arr); // ["purple", 1, 2, 3, 4] // (1) shift is the first element of the array that can be deleted. Remember that only one element can be deleted at a time // (2) shift() has no parameters // (3) After the shift is completed, the returned result is the deleted element // (4) The original array will also change
Cases: filtering arrays
4.4 array sorting
-
There are methods to sort the array itself in the array. Some methods are shown in the table below
Method name | explain | Modify original array |
---|---|---|
reverse() | Invert the order of elements in the array without parameters | This method will change the original array and return a new array |
sort() | Sort the elements of the array | This method will change the original array and return a new array |
-
Note: the sort method needs to pass in parameters (functions) to set ascending and descending sorting
-
If "function (a, b) {return A-B;}" is passed in, it is in ascending order
-
If "function (a, b) {return B-A;}" is passed in, it is in descending order
-
// Stepping pit Array sort (bubble sort) return a - b Ascending order // Fixed writing Reference is as follows var arr1 = [13,4,77,1,7]; arr1.sort(function(a,b){ return a-b; }); console.log(arr1);
4.4.1 flip array
var arr = ['pink', 'red', 'blue']; arr.reverse(); console.log(arr);
4.4.2 array sorting (bubble sorting)
var arr1 = [13, 4, 77, 1, 7]; arr1.sort(function(a, b) { // return a - b; Ascending order return b - a; // Descending order }); console.log(arr1);
4.5 array index method
There are methods to obtain the index value of the specified element of the array. Some methods are shown in the table below
<script> // The indexof (array element) method is used to return the index number of the array element and search from the front // It returns only the first index number that satisfies the condition // If it cannot find an element in the array, it returns - 1 // var arr = ['red', 'green', 'blue', 'pink', 'blue']; var arr = ['red', 'green', 'pink']; console.log(arr.indexOf('blue')); //-1 // The function of the method lastIndexOf (array element) to return the index number of the array element is to search from the back var arr = ['red', 'green', 'blue', 'pink', 'blue']; console.log(arr.lastIndexOf('blue')); // 4 </script>
Case: array de duplication (key case)
var arr = [1, 2, 3, 4, 5, 4, 1, 2]; // Find index of element 2 console.log(arr.indexOf(2)); // 1 // Find the last index of element 1 in the array console.log(arr.lastIndexOf(1)); // 6
"Convert array to string"
-
There are methods to convert an array into a string in the array. Some methods are shown in the table below
-
Note: if the join method does not pass in parameters, the elements will be spliced according to ","
var arr = [1, 2, 3, 4]; var arr2 = arr; var str = arr.toString(); // Convert array to string console.log(str); // 1,2,3,4 var str2 = arr2.join("|");//Converts an array to a string according to the characters you type console.log(str2);
"Other methods"
var arr = [1, 2, 3, 4]; var arr2 = [5, 6, 7, 8]; var arr3 = arr.concat(arr2); console.log(arr3); // [1,2,3,4,5,6,7,8] // slice(begin,end) It is a left closed right open interval [1,3) // Intercept from index 1 to index 3 var arr4 = arr.slice(1, 3); console.log(arr4); // [2,3] var arr5 = arr2.splice(0, 3); console.log(arr5); // [5,6,7] console.log(arr2); // [8] splice() affects the original array
String object
Basic wrapper type to facilitate the operation of basic data types, JavaScript also provides three special reference types: String, Number and Boolean.
The basic wrapper type is to wrap a simple data type into a complex data type, so that the basic data type has properties and methods.
// What's wrong with the following code? var str = 'andy'; console.log(str.length); // 4
In principle, basic data types have no attributes and methods, while objects have attributes and methods, but the above code can be executed because js will package basic data types as complex data types. The execution process is as follows:
// one Generate temporary variables and wrap simple types into complex data types var temp = new String('andy'); // two Assign to the character variable we declare str = temp; // three Destroy temporary variables temp = null;
"Immutability of string"
-
It means that the value inside is immutable. Although it seems that the content can be changed, in fact, the address has changed and a new memory space has been opened up in the memory.
-
When re assigning a value to a string variable, the previously saved string of the variable will not be modified. Re assigning a value to the string in memory will re open up space in memory. This feature is the immutability of the string.
-
Due to the immutability of strings, there will be efficiency problems when "splicing a large number of strings"
"Return position according to character"
-
String through the basic wrapper type, you can call some methods to operate the string. The following is the method to return the position of the specified character:
var str = "anndy"; console.log(str.indexOf("d")); // 3 //Specifies that the character "d" is found starting at an index number of 4 console.log(str.indexOf("d", 4)); // -1 console.log(str.lastIndexOf("n")); // 2
Case: find the location and number of occurrences of all o in the string "abcoefoxyozopp"
-
First find the location where the first o appears
-
Then, as long as the result returned by indexOf is not - 1, continue to look back
-
Because indexOf can only find the first one, the subsequent search uses the second parameter to add 1 to the current index to continue the search
var str = "oabcoefoxyozzopp"; var index = str.indexOf("o"); var num = 0; while (index !== -1) { console.log(index); num++; index = str.indexOf("o", index + 1); }
"Return character by position"
-
String through the basic wrapper type, you can call some methods to operate the string. The following is the character at the specified position returned according to the position:
// Returns characters based on position // one charAt(index) Returns characters based on position var str = 'andy'; console.log(str.charAt(3)); // y // Traverse all characters for (var i = 0; i < str.length; i++) { console.log(str.charAt(i)); } // a n d y // 2. charCodeAt(index) //Returns the ASCII character value of the corresponding index number Purpose: Determine which key the user pressed console.log(str.charCodeAt(0)); // 97 // three str[index] H5 New console.log(str[0]); // a
-
Case: judge the character that appears the most times in a string 'abcoefoxyozzopp', and count its times
-
Core algorithm: use charAt() to traverse this string
-
Store each character to the object. If the object does not have this attribute, it will be 1. If it exists, it will be + 1
-
Traverse the object to get the maximum value and the character. Note: in the process of traversal, each character in the string is stored in the object as the attribute of the object, and the corresponding attribute value is the number of occurrences of the character
-
var str = "abcoefoxyozzopp"; var o = {}; for (var i = 0; i < str.length; i++) { var chars = str.charAt(i); // chars yes Each character of the string if (o[chars]) { // o[chars] The result is the attribute value o[chars]++; } else { o[chars] = 1; } } console.log(o); // two Traversal object var max = 0; var ch = ""; for (var k in o) { // k Get yes Attribute name // o[k] The result is the attribute value if (o[k] > max) { max = o[k]; ch = k; } } console.log(max); console.log("The maximum number of characters is" + ch);
String operation method
-
String through the basic wrapper type, you can call some methods to operate the string. The following are some operation methods:
// String operation method // one concat('string 1 ',' string 2 '...) var str = 'andy'; console.log(str.concat('red')); // andyred // two substr('Start position of interception ', ' Intercept a few characters'); var str1 = 'The spring breeze of reform is blowing all over the ground'; // First 2 Is 2 of the index number From which Second 2 Is to take a few characters console.log(str1.substr(2, 2)); // spring breeze
-
replace() method
-
The replace() method is used to replace some characters with others in the string. Its format is as follows:
-
character string.replace(The replaced string, String to replace with);
-
split() method
-
The split() method is used to segment strings. It can segment strings into arrays. After segmentation, a new array is returned.
-
Its format is as follows:
-
character string.split("Split character")
// one Replace character replace('replaced character ', ' Replace with the character ') It only replaces the first character var str = "andyandy"; console.log(str.replace("a", "b")); // bndyandy // There is a string ' abcoefoxyozzopp' Ask for everything inside o Replace with * var str1 = "abcoefoxyozzopp"; while (str1.indexOf("o") !== -1) { str1 = str1.replace("o", "*"); } console.log(str1); // abc*ef*xy*zz*pp // two Convert character to array split('separator ') // We learned earlier join Convert array to string var str2 = "red, pink, blue"; console.log(str2.split(",")); //[red,pink,blue] var str3 = "red&pink&blue"; console.log(str3.split("&")); // [red,pink,blue]
Simple and complex data types
"Simple type (basic data type, value type)": during storage, the value itself is stored in the variable, including string, number, boolean, undefined and null
"Complex data type (reference type)": during storage, only the address (Reference) is stored in the variable. Objects (system objects, user-defined objects) created through the new keyword, such as Object, Array, Date, etc;
Stack