Essence of ES6 class
Before ES6 → realize object-oriented programming through constructor + prototype
- Constructor has prototype object prototype
- There is a constructor in the prototype that points to the constructor itself
- You can add methods through prototype objects
- Creating an instance object has__ proto__ The prototype points to the prototype object of the constructor
Nature of class
- class is essentially a function
- All methods of the class are defined on the prototype attribute of the class
- Class, which also contains__ proto __ prototype object pointing to class
- Class writing just makes the writing of object prototype clearer and more like object-oriented programming language, so the class of ES6 is actually syntax sugar.
Grammar sugar is a convenient writing method. It is simple to understand. There are two methods that can achieve the same function, but one writing method is clearer and more convenient, so this method is grammar sugar.
ES6 object oriented
1. Class creation
Create class
class name{ // class body } // To create an instance class, you must use new to instantiate the object var xx = new name();
2. constructor constructor
- Class constructor (default method), used to pass parameters and return instance objects
- This method is called automatically when an object instance is generated through new
- If no definition is displayed, it will be automatically created inside the class
class Person{ constructor(name,age){ this.name = name; this.age = age; } say(){ console.log(this.name + 'Hello!'); } } // Create instance var ldh = new Person('Lau Andy',18); console.log(ldh.name) // Lau Andy
- You cannot add commas between methods, and methods do not need the function keyword
3. Class inheritance
Subclasses can inherit some properties and methods of the parent class.
class Father{ // Parent class } class Son extends Father{ // The subclass inherits the parent class }
example:
class Father{ constructor(surname){ this.name = surname; } say(){ console.log('What's your last name' + this.name); } } class Son extends Father{ // The child class inherits the parent class } var ss = new Son('Liao') ss.say(); // Your last name is Liao
- Search principle for attributes or methods in inheritance: proximity principle
4. super keyword
Call the constructor of the parent class, or call the normal function of the parent class.
class Father{ constructor(surname){ this.surname = surname; } saySurname(){ console.log('My last name is' + this.surname); } } class Son extends Father{ constructor(surname,firstname){ // Descendant parent super(surname); // Call the parent class constructor(surname) this.firstname = firstname; // Define properties unique to subclasses } sayFirstname(){ console.log('My name is:' + this.firstname); } } var ss = new Son('Wu','Yanzu') ss.saySurname(); ss.sayFirstname();
Call the normal function of the parent class
class Father{ say(){ return 'I'm a parent -->' } } class Son extends Father{ say(){ // Super. Say() calls the superclass method return super.say() + 'Subclass of'; } } var dome = new Son(); console.log(dome.say()); // I'm a parent! Subclass of
super must be called before subclass this.
The subclass uses super in the constructor and must be placed before this (the constructor of the parent class must be called first, and the constructor of the subclass must be used)
Attention
- There is no variable promotion (variable declaration in advance) in ES6, so you must define a class before you can instantiate an object.
- The common properties and methods in the class must be used with this.
this in the class points to the problem:
- This in the constructor points to the instance object, and this in the method points to the caller of this method.
Some methods:
usage | explain |
---|---|
insertAdjacentHTML('appended position ',' appended character ') | The element created with a string is appended to the parent element |
beforeend | After inserting the last child node inside the element |
ondblclick | Double click the event |
input.select() | The content of the text box is selected |
Double click to suppress the selected text:
window.getSelection ? window.getSelection().removeAllRanges() : document.selection.empty();
insertAdjacentHTML(position,text);
// Create li element and section element var li = '<li class="liactive"><span>Test 1</span><span class="iconfont icon-guanbi"></span></li>'; // Append these two elements to the corresponding parent element before end (after the content of the parent element) that.ul.insertAdjacentHTML('beforeend', li)
ES6 new syntax
let declaration variable keyword
-
let declared variables are only valid at the block level
if (true) { let b = 20; console.log(b) if (true) { let c = 30; } console.log(c); // c is not defined } console.log(b) // b is not defined
-
There is no variable promotion
console.log(a); // a is not defined let a = 20;
-
There is a temporary dead band problem
var num = 10 if (true) { console.log(num); // Cannot access 'num' before initialization let num = 20; }
-
Prevent circular variables from becoming global variables
```js for (let i = 0; i < 5; i++) { console.log(i); } // Output 0 1 2 3 4 5 in sequence ```
const declaration constant
Function: declare a constant. A constant is an amount whose value (memory address) cannot be changed
-
Constants declared with the const keyword have block level scope
if (true) { const a = 10; if (true) { const a = 20; console.log(a); // 20 } console.log(a); // 10 } console.log(a); // a is not defined
-
A value must be assigned when declaring a constant
const PI; // Missing initializer in const declaration
- After constant assignment, the value cannot be modified, but the value in the array can be replaced.
const = PI; PI = 100; // assignment to constant variable. const ary = [100, 200]; ary[0] = 'a'; ary[1] = 'b'; console.log(ary); // ['a', 'b']; ary = ['a','b']; // assignment to constant variable.
Destructuring assignment
ES6 allows to extract values from numerical values and assign values to variables according to corresponding positions. Objects can also be deconstructed.
Numerical deconstruction
// Array deconstruction allows us to extract values from arrays according to one-to-one correspondence, and then assign values to variables let ary = [1,2,3]; let [a, b, c, d, e] = ary; console.log(a,b,c) // 1 2 3 console.log(e) // undefined // If the deconstruction is unsuccessful, the value of the variable is undefined let [foo] = []; let [bar, foo] = [1];
Object deconstruction
let person = { name: 'zhangsan', age: 20 }; // Deconstruct (match) the name and age attributes from the person object let { name, age } = person; console.log(name); // 'zhangsan' console.log(age); // 20 let {name: myName, age: myAge} = person; // myName myAge property alias console.log(myName); // 'zhangsan' console.log(myAge); // 20
Arrow function
Writing method:
() => {} const fn = () => {}
- There is only one sentence of code in the function body, and the execution result of the code is the return value. Curly braces can be omitted
function sum(num1, num2) { return num1 + num2; } const sum = (num1, num2) => num1 + num2;
- If there is only one formal parameter, you can omit the parentheses
function fn(v){ retuen v; } const fn = v => v;
- The arrow function does not bind this and does not have its own this keyword. In the arrow function, the this keyword will point to this in the definition position of the arrow function.
const obj = { name: 'Zhang San'} function fn () { console.log(this); // obj return () => { console.log(this) // obj } } const resFn = fn.call(obj); resFn();
Arrow function interview questions:
var age = 100; var obj = { age: 20, say: () => { alert(this.age) // 100 } } obj.say();
Remaining parameters
Add
- The residual parameter syntax allows us to represent (store) an indefinite number of parameters as an array
function sum (first, ...args) { console.log(first); // 10 console.log(args); // [20, 30] } sun(10, 20, 30);
- The remaining parameters are used in conjunction with deconstruction
let students = ['wangwu', 'zhangsan', 'lisi']; let [s1, ...s2] = students; console.log(s1); // wangwu console.log(s2); // ['zhangsan', 'lisi']
ES6 new method
Array method
Extension operator (expand syntax)
- Converts an array or object to a comma separated sequence of parameters
let aty = [1, 2, 3]; // ...ary // 1, 2, 3 console.log(...ary); // 1 2 3 console.log(1, 2, 3); // 1 2 3
- Use the extension operator to apply to merged arrays
//Method 1 let ary1 = [1, 2, 3]; let ary2 = [3, 4, 5]; let ary3 = [...ary1, ...ary2]; // Method 2 ary1.push(...ary2);
- Converts a class (pseudo) array or traversable object to a true array
let oDivs = document.getElemenetsByTagName('div'); // HTMLCollection(10) oDivs = [...oDivs]; // Array(7)
Constructor method
- Converts a class (pseudo) array or traversable object to a true array
let arrayLike = { '0': 'a', '1': 'b', '2': 'c', length: 3 }; let arr2 = Array.from(arrayLike); // ['a', 'b', 'c']
- You can pass in the second parameter, which is similar to the map method of array. It is used to process each element and put the processed value into the returned array.
let arrayLike = { "0": 1, "1": 2, "length": 2 } let newAry = Array.from(aryLike, item => item * 2)
Example method
find()
Returns the first qualified element. If it is not found, it returns undefined
let ary = [{ id: 1, name: 'Zhang San' },{ id: 2, name: 'Li Si' }]; let target = ary.find((item, index) => item.id == 2);
findIndex()
Returns the index position of the first qualified element. If it is not found, it returns undefined
let ary =[1, 5, 10, 15]; let index = ary.findIndex((value,index) => value > 9); console.log(index); // 2
includes()
Finds whether an array contains the given value and returns a Boolean value.
[1, 2, 3].includes(2); // true [1, 2, 3].includes(4); // false let ary = ["a", "b", "c"]; console.log(ary.includes('a'))
Template string
The new method of creating strings in ES6 is defined by backquotes.
1. Analytic variable
let name = 'Zhang San'; let sayHello = `hello,my name is ${name}`; // hello,my name is zhangsan
2. Template string wrap
let resilt = { name: 'zhangsan', age: 20, sex: 'male' } let html = ` <div> <span>${result.name}</span> <span>${result.name}</span> <span>${result.name}</span> </div> `;
3. Functions can be called in the template string
colst aryHello = function () { return 'Hello world!'; }; let greet = `${sayHello()} Ha ha ha ha`; console.log(greet); // Hello world!
String method
startsWith()
- Indicates whether the parameter string is at the head of the original string and returns a Boolean value
endsWith()
- Indicates whether the parameter string is at the tail of the original string, and returns a Boolean value
let str = 'Hello world!'; str.startsWith('Hello') // true str.endsWith('!') // true
repeat()
- Repeat the original string n times and return a new string.
'x'.repeat(3) // "xxx" 'hello'.repeat(2) // "hellohello"
Set data structure
- ES6 provides a new data structure Set, which is similar to array, but the values of members are unique without duplicate values.
- Set itself is a constructor used to generate a set data structure.
- The Set function can accept an array as a parameter for initialization.
// Create a set() data structure const s = new Set; const set = new Set([1, 2 ,3 ,4 , 5]);
set() instance method
method | explain |
---|---|
add(value) | Add a value and return the Set structure itself |
delete(value) | Indicates whether the deletion is successful, and returns a Boolean value |
has(value) | Indicates whether the value is a member of Set and returns a Boolean value |
clear() | Clear all members |
Example:
const s = new Set(); s.add(1).add(2).add(3); // Adds a value to the set structure s.delete(2) // Delete the 2 value in the set structure s.has(1) // Indicates whether there is 1 in the set structure. This value returns a Boolean value s.clear() // Clear all values in the set structure
set() traversal
- An instance of a Set structure, like an array, also has a forEach method.
// Traverse the set data structure to get values from it const s = new Set(['a', 'b', 'c']); s.forEach(value => { console.log(value) })
regular expression
Regular tables are often used to extract and replace text that conforms to a pattern (rule).
Columns such as validation forms, filtering sensitive words, and getting the specific parts we want from the string.
Create regular expressions
// 1. Use RegExp object to create regular expression var regexp = new RegExp(/123/); console.log(regexp); // 2. Create regular expressions with literals var rg = /123/;
test() detects regular expressions
The regular object method is used to detect whether the string conforms to the rule, and returns true or false
var rg = /123/; console.log(rg.test(123));
Boundary character
Boundary character | explain |
---|---|
^ | Represents the text that matches the beginning of the line (starting with who) |
$ | Represents the text that matches the end of the line (with whom) |
- ^When used with $, the representation must be an exact match
Character class
- Indicates that there are a series of characters to choose from, as long as you match one of them
- All selectable characters are enclosed in square brackets [].
var rg = /[abc]/; // It returns true as long as it contains a, b or c console.log(rg.test('andy')); // 2. The character combination matches 26 English letters and numbers 1-9 /^[a-z0-9]$/.test('a') // true // 3. [^] inverse character inside square brackets^ /^[^abc]$/.test('a') // false
Quantifier
Quantifier is used to set the number of times a pattern appears.
classifier | explain |
---|---|
* | Matches zero or more times |
+ | Repeat one or more times |
? | Repeat zero or once |
{n} | Repeat n times |
{n,} | Repeat n or more times |
{n,m} | Repeat n to m times |
Use of parentheses
brackets | explain |
---|---|
{} quantifier | The number of repetitions is indicated in parentheses |
[] character set | Matches any character in parentheses |
() grouping | Indicates priority |
Predefined Class
Predefined classes are shorthand for some common patterns
Predefined | explain | Equivalent to |
---|---|---|
\d | Match any number between 0 and 9 | [0-9] |
\D | Match all characters except 0-9 | [ ^0-9] |
\w | Match any letters, numbers, and underscores | [A-Za-z0-9_] |
\W | Characters other than all letters, numbers, and underscores | [ ^A-Za-z0-9_] |
\s | Match spaces (including line breaks, tabs, spaces, etc.) | [\t\r\n\v\f] |
\S | Matches characters that are not spaces | [ ^\t\r\n\v\f] |
Global match and ignore case
Matching pattern | explain |
---|---|
g | Global matching (only one is matched by default) |
i | ignore case |
gi | Global match + ignore case |
Substitution in regular expressions
Replace with the replace() method, and the return value is the new string after replacement.
stringObject.replace(regexp/substr,replacement)
- The first parameter: the replaced string or regular expression
- Second parameter: replaced string
<textarea name="" id="message"></textarea> <button>Submit</button> <div></div> // Replace replace var text = document.querySelector('textarea'); var btn = document.querySelector('button'); var div = document.querySelector('div'); btn.onclick = function() { div.innerHTML = text.value.replace(/passion|gay/g, '**'); }
Constructor and prototype of ES5
Before ES6, objects were not created based on classes, but special functions of building functions were used to define objects and their characteristics.
Constructor is a special function. It uses new to initialize the object, extracts some public properties and methods from the object, and then encapsulates them into this function.
The constructor has the problem of wasting memory
Solution: we can directly define those Invariant Methods on the prototype object, so that all object instances can share these methods.
Constructor prototype
The function allocated by the constructor through the prototype is shared by all objects.
- The role of prototypes is to share methods.
Object prototype__ proto __
Objects have a property__ proto __ The prototype object that points to the constructor. The reason why we can use the properties and methods of the constructor prototype object is that the object has__ proto __ The existence of prototypes.
__ proto__ The significance of object prototype is to provide a direction, or a route, for the object member search mechanism
example:
function Star(uname, age) { this.uname = uname; this.age = age; } // Our public properties are defined in the constructor, and the public methods are placed on the prototype object Star.prototype.sing = function() { console.log('I can sing'); } var ldh = new Star('Joker Xue', 18); var zxy = new Star('Spiritual realm', 19); console.log(ldh.sing === zxy.sing); // true ldh.sing(); zxy.sing(); // The system adds one to the object itself__ proto__ Point to the prototype object prototype of our constructor console.log(ldh); console.log(ldh.__proto__ === Star.prototype); // true
-
__ proto __ Object prototype and prototype object prototype are equivalent
-
Method lookup rules: first, check whether there is a sing method on the ldh object. If so, execute the sing method on the object
-
If there is no sing method, because there is__ proto__ For the existence of, go to the constructor prototype object prototype to find the method of sing.
Constructor constructor
Objects have a property__ proto __ The prototype object that points to the constructor. The reason why we can use the properties and methods of the constructor prototype object is that the object has__ proto __ The existence of prototypes.
Constructor is mainly used to record the constructor referenced by the object. It can make the prototype object point to the original constructor again.
Note: in many cases, we need to manually use the constructor attribute to refer back to the original constructor.
function Star(uname, age) { this.uname = uname; this.age = age; } // In many cases, we need to manually use the constructor attribute to refer back to the original constructor // Star.prototype.sing = function() { // console.log('I can sing '); // }; // Star.prototype.movie = function() { // console.log('I can play movies'); // } Star.prototype = { // If we modify the original prototype object and assign an object to the prototype object, we must manually use the constructor to refer back to the original constructor constructor: Star, sing: function() { console.log('I can sing'); }, movie: function() { console.log('I can play movies'); } } var ldh = new Star('Joker Xue', 28); var zxy = new Star('Spiritual realm', 26); console.log(Star.prototype); // Object console.log(ldh.__proto__); // Object console.log(Star.prototype.constructor); console.log(Star.prototype.constructor === ldh.__proto__.constructor); // true
Relationship between constructor, instance, prototype object
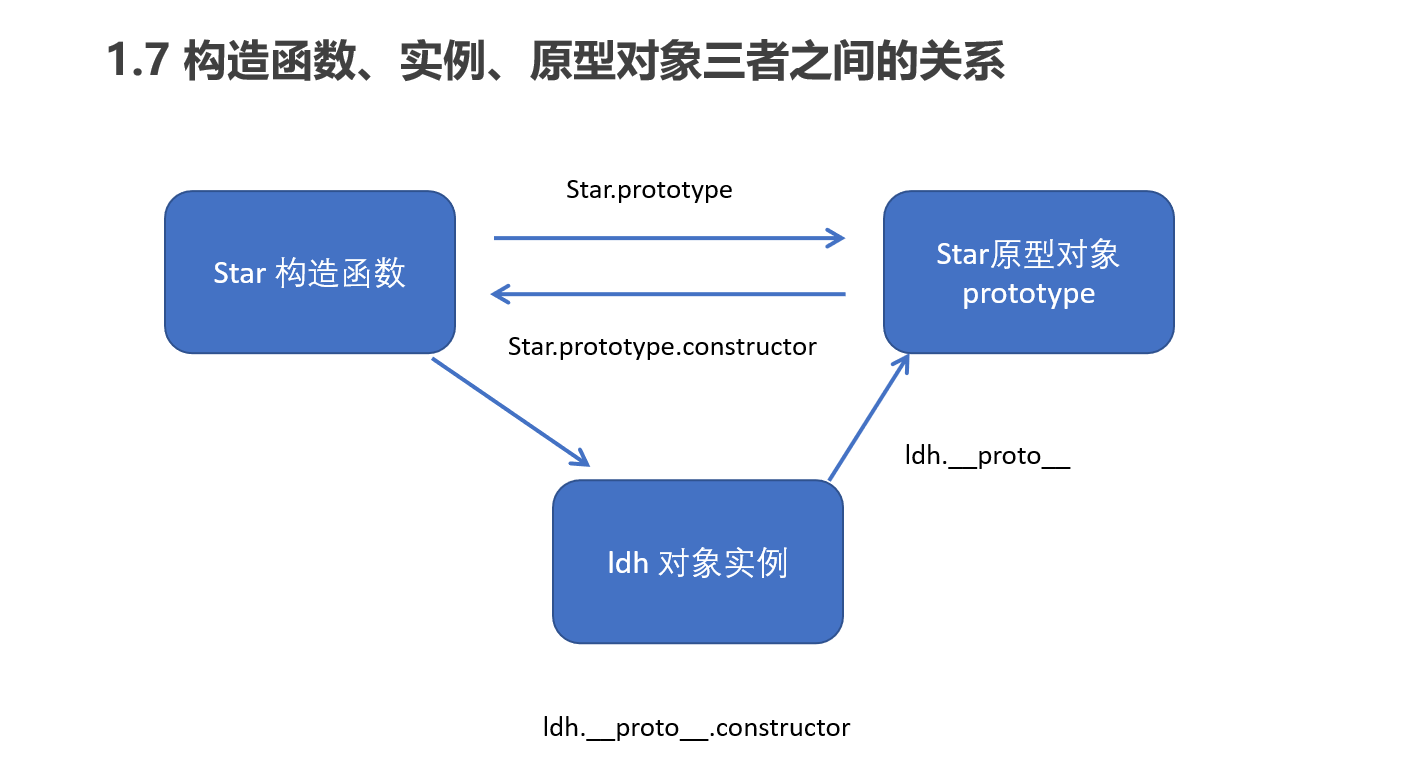
Prototype chain
Member lookup mechanism
-
When accessing an object's property, first find out whether the object itself has the property.
-
If not, find the prototype (_proto__ points to the prototype).
-
If not, find the prototype of the prototype Object (the prototype Object of the Object).
-
Until the Object is found (null).
this in the method in the prototype object refers to the caller of the method, that is, the instance object
Extend built-in object methods
Through the prototype object, the original built-in object can be extended and customized, such as adding the function of custom even sum to the object.
Array.prototype.sum = function(){ var sum = 0; for (var i = 0; i < this.length; i++) { sum += this[i]; } return sum; } var arr = [1, 2, 3]; console.log(arr.sum());
The method created in the form of object will be overwritten (e.g. Array.prototype = {}), so the built-in objects of array and string can only be Array.prototype.xxx = function() {}.
call() method
call (set the object pointed to when calling, pass other parameters...)
- Call the function and modify the point of this when the function runs.
function fn(x, y) { console.log('I'd like some hand ground coffee'); console.log(this); console.log(x + y); } var o = { name: 'andy' }; // fn.call(); 1. Use call() to call the function // 2. You can change this point of this function, and this point of this function points to o this object fn.call(o, 1, 2);
Inheritance in ES5
ES6 did not provide us with extensions inheritance before. We can implement inheritance through constructor + prototype object simulation, which is called composite inheritance.
Borrowing the parent constructor to inherit properties and methods
// 1. Parent constructor function Father(uname, age) { // this points to the object instance of the parent constructor this.uname = uname; this.age = age; } // Define sharing method Father.prototype.money = function() { console.log(100000); }; // 2. Sub constructor function Son(uname, age, score) { // Borrowing the inheritance property of the parent constructor, use call() to set this as the this of the child constructor when calling Father.call(this, uname, age); // this points to the object instance of the child constructor this.score = score; } // Son.prototype = Father.prototype; If the child prototype object is modified, the parent prototype object will change with it Son.prototype = new Father(); // If you modify the prototype object in the form of an object, don't forget to use the constructor to refer back to the original constructor Son.prototype.constructor = Son; // This is a special method of the sub constructor Son.prototype.exam = function() { console.log('The child wants an exam'); } var son = new Son('Zhang quandan', 28, 100); console.log(son); console.log(Father.prototype); console.log(Son.prototype.constructor);
Review of ES5 methods
Array method
- Iterative (traversal) methods: forEach(), map(), filter(), some(), every()
forEach() traverses the array
- forEach is suitable when you don't intend to change the data (print or store in the database)
array.forEach(function(currentValue, index, arr))
-
currentValue: each array element
-
Index: the index of the current item of the array
-
arr: array object itself
map() array traversal
- It applies when you want to change the data value. Faster and returns a new array.
- You can also use composition (map (), filter (), reduce ()) and other combinations.
let arr = [1, 2, 3, 4, 5]; // Use the map to multiply each element by itself, then filter out those elements greater than 10, and assign the final result to arr2. let arr2 = arr.map(value => value * value).filter(value => value > 10); // arr2 = [16, 25]
filter() filter array
A new array is returned directly when filtering.
array.filter(function(currentValue, index, arr))
example:
var arr = [12,66,88,99,56,4,3] var newarr = arr.filter(function(value,index){ // Filter values greater than 20 in the array return value > = 20; //Return value% 2 = = = 0; / / returns an even number }); console.log(newarr);
Does some() satisfy the condition
Returns a Boolean value. If the first element satisfying the condition is found, the loop is terminated and the search is no longer continued.
var arr1 = ['red', 'pink', 'blue']; var flag1 = arr1.some(function(value) { return value == 'pink'; }); console.log(flag1); // true
filter returns a new array containing multiple elements, and find returns the first qualified element.
The indexof() method returns the first occurrence of a specified string value in the string.
String method
trim() deletes white space characters at both ends
- Returns a new string without affecting the original string.
var str = ' an dy '; var str1 = str.trim();
Object method
Object.keys()
Get all the properties of the object itself
var obj = { id: 1, pname: 'millet', price: 1999, num: 2000 }; // Get all the properties of the object itself var arr = Object.keys(obj); // Use Object.keys() to get all the attributes and traverse the object arr.forEach(function(value) { console.log(value); })
Object.defineProperty()
Define new properties or modify existing properties
Object.defineproperty(obj, prop, descriptor)
- obj: required, target object
- prop: required, the name of the attribute to be defined or modified
- descriptor: required, the attribute owned by the target attribute
Description of the third parameter descriptor:
- Must be written in object form {}
- Value: set the attribute value to undefined by default
- writable: sets whether the property value can be modified. true | false is false by default and cannot be modified.
- enumerable: whether the target attribute can be traversed.
- configurable: whether the target attribute can be deleted or modified again. The default is false and cannot be deleted or modified.
var obj = { id: 1, pname: 'millet', price: 1999 }; Object.defineProperty(obj, 'address', { value: 'Xiaomi Technology Co., Ltd', // If the value is false, it is not allowed to modify this property value. The default value is also false writable: true, // enumerable if the value is false, traversal is not allowed, and the default value is false enumerable: true, // Configurableif false, this attribute cannot be deleted. The default value is false configurable: true }); console.log(obj.address);
Function advanced order
Definition method
Call mode
- Ordinary function
function fn() { console.log('Hello !'); } // fn(); fn.call()
- Object method
var o = { sayHi: function() { console.log('The peak of life'); } } o.sayHi();
- Constructor
function Star() { // do somesing... }; new Star();
- Binding event function
btn.onclick = function() {}; // This function can be called by clicking the button
- Timer Functions
setInterval(function() {}, 1000); // This function is called once a second automatically by the timer
- Execute function now
// The immediate execution function is self calling (function() { console.log('hello 2021'); })();
this points to the problem
The different calling methods determine the different points of this.
Call mode | this point |
---|---|
Ordinary function call | window |
constructor call | The method in the (created) instance object prototype object points to the instance object |
Object method call | Change the object to which the method belongs |
Event binding method | The object that binds the event |
Timer Functions | window |
Execute function now | window |
Detailed explanation:
<button>click</button> <script> // The different calling methods of the function determine the different points of this // 1. The normal function this points to window function fn() { console.log('Ordinary function this' + this); } window.fn(); // 2. Object method this refers to object o var o = { sayHi: function() { console.log('Object method this:' + this); } } o.sayHi(); // 3. The constructor this points to the instance object ldh. This in the prototype object also points to the instance object ldh function Star() {}; Star.prototype.sing = function() { } var ldh = new Star(); // 4. The binding event function this points to the function caller btn, the button object var btn = document.querySelector('button'); btn.onclick = function() { console.log('Binding time function this:' + this); }; // 5. The timer function this also points to window window.setTimeout(function() { console.log('Timer this:' + this); }, 1000); // 6. Execute the function this now or point to window (function() { console.log('Execute function immediately this' + this); })(); </script>
Change this point inside the function:
Using bind(), call(), and apply() can gracefully solve the problem of this pointing inside the function.
call() and apply() call functions to change this point at the same time
var o = { name: 'andy' } function fn(a, b) { console.log(this); console.log(a + b); }; // 1. call() calls the function and changes the direction of this at the same time fn.call(o, 1, 2); // apply() calls the function to change this point at the same time. The parameter must be an array (pseudo array) fn.apply(o, ['pink']);
bind() does not call the function to change this point
Example: we have a button. When we click it, we disable it and turn it on after 3 seconds:
<button>click</button> <button>click</button> <button>click</button> var btns = document.querySelectorAll('button'); for (var i = 0; i < btns.length; i++) { btns[i].onclick = function() { this.disabled = true; setTimeout(function() { this.disabled = false; }.bind(this), 2000); } }
Summary:
Similarities:
- Can change the internal this point of the function.
difference:
- Call and apply will call the function and change the internal this point of the function;
- bind will not call the function. You can change the internal this point of the function
Application scenario:
- call() often inherits;
- apply() is often related to arrays, such as finding max | min with the help of mathematical objects;
- bind() is used when you don't want to call the function and want to change the this point, such as changing the this point inside the timer.
Higher order function
Callback function
Higher order functions are functions that operate on other functions. They receive functions as parameters or output functions as return values.
function fn(a, b, callback) { console.log(a + b); callback && callback(); } fn(7, 10, function() { console.log('I was the last to call it.'); });
Function is also a data type. It can also be passed to another parameter as a parameter. The most typical is as a callback function.
Recursive function
If a function can call itself internally, the function is a recursive function
- Recursive functions have the same effect as loops
- Because recursion is prone to "stack overflow" errors, you must add an exit condition return
Example: using recursive function to find factorial of 1~n
// Factorization of 1~n by recursive function 1 * 2 * 3 * 4 *. N function fn(n) { if (n == 1) { return 1; } return n * fn(n - 1); } console.log(fn(3)); console.log(fn(4)); // Detailed idea if the user enters 3 //return 3 * fn(2) //return 3 * (2 * fn(1)) //return 3 * (2 * 1) //return 3 * (2) //return 6
Example 2: find Fibonacci sequence (rabbit sequence) using recursive function
// 1,1,2,3,5,8,13,21... // The user can input a number n to calculate the rabbit sequence value corresponding to this number // We only need to know the first two items (n-1, n-2) of N entered by the user to calculate the sequence value corresponding to n function fb(n) { if (n === 1 || n === 2) { return 1; } return fb(n - 1) + fb(n - 2); } console.log(fb(3)); console.log(fb(6));
closure
Variable scopes are divided into global variables and local variables
- Global variables can be used inside functions
- Local variables cannot be used outside a function
- When the function is executed, the local variables of this scope will be destroyed.
What is a closure?
A scope can access local variables inside another function.
Function of closure:
Extends the scope of the variable
The scope outside the function can access the local variables inside the function.
function fn1(){ // fn1 is the closure function var num = 10; function fn2(){ console.log(num); // 10 } fn2() }
Thinking question 1:
var name = "The Window"; var obj1 = { name: "My Object", getNameFunc: function () { return function () { return this.name; }; } }; console.log(obj1.getNameFunc()()) // The Window
Thinking question 2:
var name = "The Window"; var obj2 = { name: "My Object", getNameFunc: function () { var that = this; return function () { return that.name; }; } }; console.log(obj2.getNameFunc()()) // My Object
Light copy and deep copy
- Shallow copy just copies one level, and deeper object level values copy references
- Deep copy copies multiple layers, and data at each level is copied.
The newly added method in ES6 can be shallow copy
Object.assign(target, ...sources)
var obj = { id: 1, name: 'andy', msg: { age: 18 } }; var o = {}; Object.assign(o, obj);
Strict mode
- Turn on strict mode for the entire js script
<script> "use strict"; console.log("This is a strict model."); </script>
Some scripts are basically in strict mode and some scripts are in normal mode, which is not conducive to file merging. Therefore, the whole script file can be placed in an anonymous function to be executed immediately, so as to create a scope independently without affecting other script files.
(function(){ "use strict"; var num = 10; function fn(){} })();
Turn strict mode on for functions
// At this time, we just turn on strict mode for fn function function fn() { 'use strict'; // The following code executes in strict mode }
Strict mode change
Variable general:
- The variable name must be declared before use without variable declaration in advance;
- It is strictly prohibited to delete declared variables;
this pointing problem in strict mode:
- In strict mode, this in the function in the global scope is undefined, not Windows;
- If the constructor does not add a new call, this will report an error;
- The constructor instantiated by new points to the created object instance;
- The timer this still points to window;
- Event, object, or point to the caller;
- In strict mode, duplicate names are not allowed for parameters in functions.
{/ / fn1 is the closure function
var num = 10;
function fn2(){
console.log(num); // 10
}
fn2()
}
Thinking question 1: ```js var name = "The Window"; var obj1 = { name: "My Object", getNameFunc: function () { return function () { return this.name; }; } }; console.log(obj1.getNameFunc()()) // The Window
Thinking question 2:
var name = "The Window"; var obj2 = { name: "My Object", getNameFunc: function () { var that = this; return function () { return that.name; }; } }; console.log(obj2.getNameFunc()()) // My Object
Light copy and deep copy
- Shallow copy just copies one level, and deeper object level values copy references
- Deep copy copies multiple layers, and data at each level is copied.
The newly added method in ES6 can be shallow copy
Object.assign(target, ...sources)
var obj = { id: 1, name: 'andy', msg: { age: 18 } }; var o = {}; Object.assign(o, obj);
Strict mode
- Turn on strict mode for the entire js script
<script> "use strict"; console.log("This is a strict model."); </script>
Some scripts are basically in strict mode and some scripts are in normal mode, which is not conducive to file merging. Therefore, the whole script file can be placed in an anonymous function to be executed immediately, so as to create a scope independently without affecting other script files.
(function(){ "use strict"; var num = 10; function fn(){} })();
Turn strict mode on for functions
// At this time, we just turn on strict mode for fn function function fn() { 'use strict'; // The following code executes in strict mode }
Strict mode change
Variable general:
- The variable name must be declared before use without variable declaration in advance;
- It is strictly prohibited to delete declared variables;
this pointing problem in strict mode:
- In strict mode, this in the function in the global scope is undefined, not Windows;
- If the constructor does not add a new call, this will report an error;
- The constructor instantiated by new points to the created object instance;
- The timer this still points to window;
- Event, object, or point to the caller;
- In strict mode, duplicate names are not allowed for parameters in functions.