outline
Introduction to Spring mvc
2. Spring mvc code practice
3. Project Source Download
IV. Reference Articles
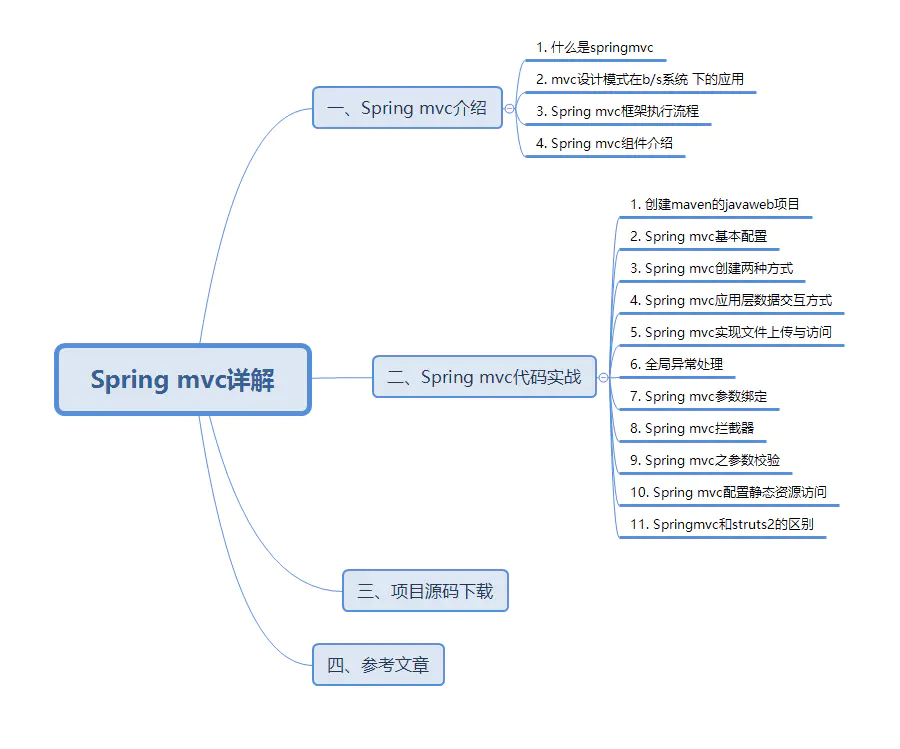
Introduction to Spring mvc
1. What is spring MVC
_Spring MVC is a module of spring framework. Spring MVC and spring need not be integrated through the middle integration layer. Spring MVC is a web framework based on mvc.
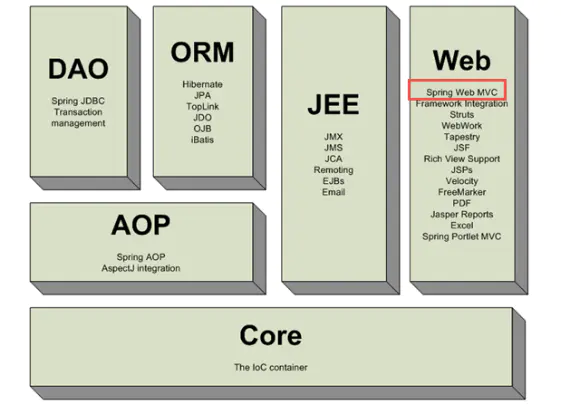
2. Application of MVC design pattern in b/s system
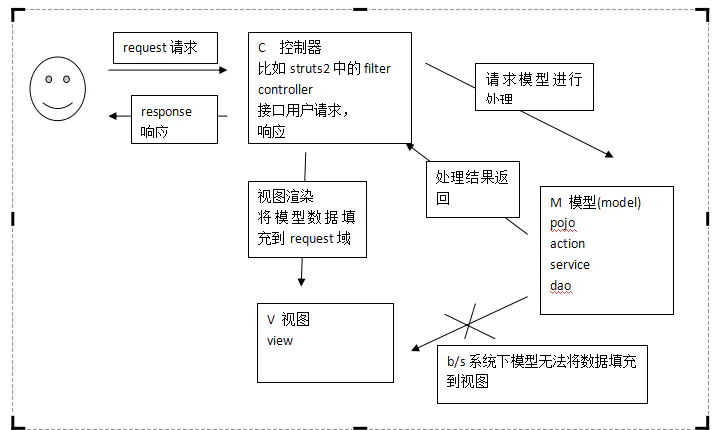
3. Spring mvc framework execution process
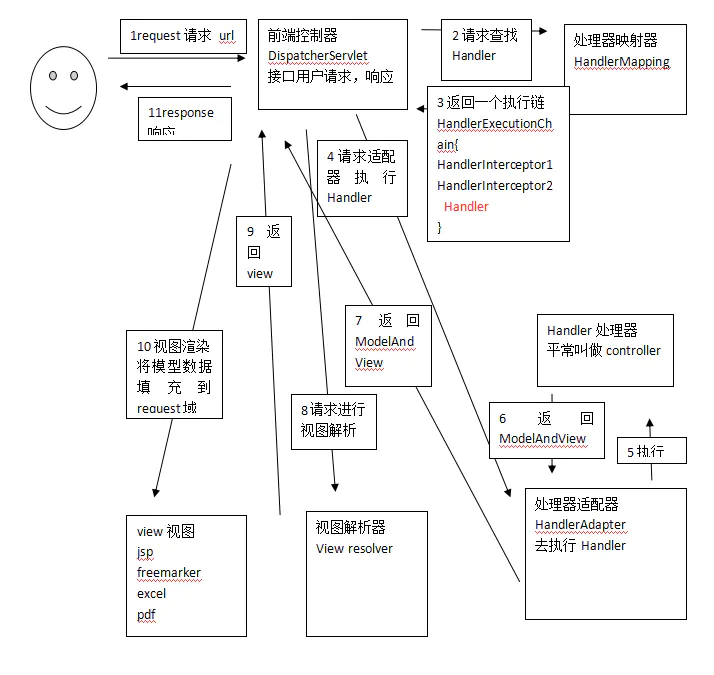
Step 1: Initiate a request to the front-end controller (Dispatcher Servlet)
Step 2: The front-end controller requests Handler Mapping to find Handler, which can be searched according to xml configuration and annotations, and through the test in @RequestMapping (value ="/test").
Step 3: Processor mapper Handler Mapping returns Handler to the front-end controller
Step 4: The front-end controller calls the processor adapter to execute the Handler
Step 5: Processor adapter to execute Handler
Step 6: Handler completes execution and returns ModelAndView to the adapter
Step 7: The processor adapter returns ModelAndView to the front-end controller, which is an underlying object of the spring MVC framework, including Model and view
Step 8: The front-end controller requests the view parser to parse the view, and parses it into a real view (jsp) according to the logical view name.
Step 9: View parser returns View to front-end controller
Step 10: The front-end controller renders the view, which fills the model data (in the ModelAndView object) into the request field.
Step 11: Front-end controller responds to user results
4. Introduction to Spring MVC components
(1) Front-end controller Dispatcher Servlet (without programmer development)
The function of receiving requests and responding to results is equivalent to a transponder and a central processing unit.
Dispatcher Servlet reduces the coupling between other components.
(2) Processor mapper Handler Mapping (without programmer development)
Role: Find Handler based on the requested url
(3) Processor adapter Handler Adapter
Function: Execute Handler according to specific rules (rules required by Handler Adapter)
(4) Processor Handler (need programmer development)
Note: Write Handler according to the requirements of Handler Adapter, so that the adapter can correctly execute Handler.
(5) View resolver (without programmer development)
Function: View parsing, parsing into a real view based on the logical view name
(6) View View (requiring programmers to develop jsps)
View is an interface that implements classes that support different View types (jsp, freemarker, pdf...)
2. Spring mvc code practice
_Spring mvc's common functions include data interaction mode (Model AndView and JSON), static resource parsing, parameter checking, global exception handling, interceptor, uploading pictures, etc.
1. Create maven's javaweb project
The article focuses on explaining Spring mvc function, so the way to create a project is not explained in depth. The catalogue of the created project is as follows:
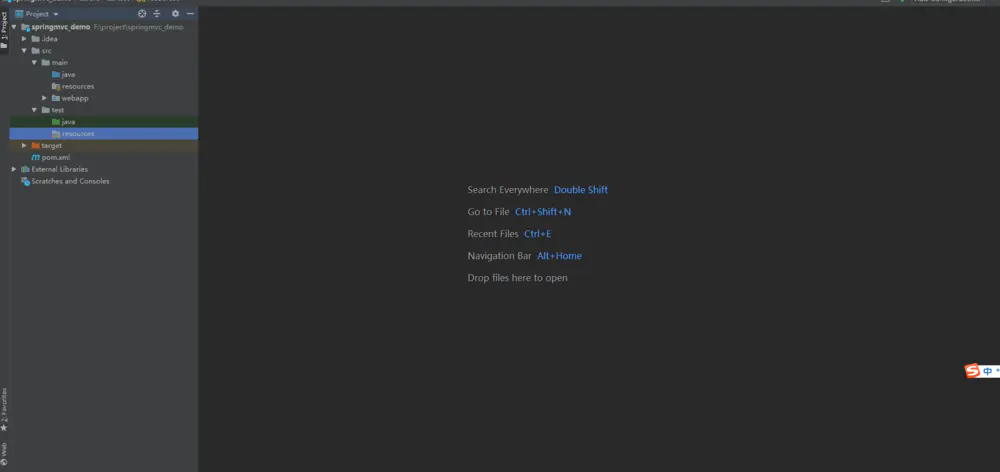
2. Basic configuration of Spring MVC
2.1 Adding maven dependencies to pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>springmvc_demo</groupId>
<artifactId>springmvc_demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name/>
<description/>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<!-- spring version number -->
<spring.version>4.2.5.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.2</version>
</dependency>
<dependency>
<groupId>org.apache.openejb</groupId>
<artifactId>javaee-api</artifactId>
<version>5.0-1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.1</version>
<scope>provided</scope>
</dependency>
<!-- paging -->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>4.1.4</version>
</dependency>
<!--Test package-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- c3p0 Database connection pool -->
<dependency>
<groupId>c3p0</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.1.2</version>
</dependency>
<!-- commons Tool kit -->
<!--Picture upload related-->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>commons-beanutils</groupId>
<artifactId>commons-beanutils</artifactId>
<version>1.7.0</version>
</dependency>
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.7</version>
</dependency>