Java originally appeared as a network programming language, which provides a high degree of support for the network, making the communication between client and server become a reality, and in network programming, Socket is the most used. Like QQ, MSN uses Socket technology.
Socket programming
I. Basic Knowledge of Network (Reference to Computer Network)
1. Communication between two computers requires three conditions:
IP address, protocol, port number
2. TCP/IP protocol:
At present, it is the most widely used protocol in the world. It is a collection of multiple protocols at different levels based on TCP and IP. It is also a family of TCP/IP protocols or a TCP/IP protocol stack.
TCP: Transmission Control Protocol
IP: Internet Protocol Internet Protocol
3. TCP/IP Five-Layer Model
Application Layer: HTTP, FTP, SMTP, Telnet, etc.
Transport Layer: TCP/IP
Network layer:
Data Link Layer:
Physical layer: wire, twisted pair, network card, etc.
4, IP address
In order to realize the communication between different computers in the network, each computer must have a unique ID - IP address.
32-bit binary
5, port
Distinguish different applications from one host. The port number ranges from 0 to 65535, of which 0 to 1023 bits are reserved for the system.
For example: HTTP: 80 FTP: 21 Telnet: 23
IP address + port number constitute the so-called Socket, which is the end point of two-way communication link between programs running on the network and the basis of TCP and UDP.
6. Socket socket:
Only when IP addresses and ports with unique identifiers are combined on the network can a unique identifier socket be formed.
Socket Principle and Mechanism:
Both ends of the communication have Socket s
Network communication is actually the communication between Socket s.
Data is transmitted between two Socket s via IO
7. Network Support in Java
For different levels of network communication, Java provides different API s, which provide four types of network functions:
InetAddress: Used to identify hardware resources on the network, mainly IP addresses
URL s: Unified Resource Locator, through which data on the network can be read or written directly
Sockets: Classes Related to Sockets in Network Communication Implemented by TCP Protocol
Datagram: Uses UDP protocol to store data in user datagrams and communicate through the network.
II. InetAddress
The InetAddress class is used to identify hardware resources on the network and Internet Protocol (IP) addresses.
There is no construction method for this class.
import java.net.InetAddress; import java.net.UnknownHostException; import java.util.Arrays; /* * InetAddress class */ public class Test01 { public static void main(String[] args) throws UnknownHostException { // Get an instance of InetAddress on this machine InetAddress address = InetAddress.getLocalHost(); System.out.println("Name of calculation:" + address.getHostName()); System.out.println("IP Address:" + address.getHostAddress()); byte[] bytes = address.getAddress();// Get the IP address in byte array form System.out.println("Byte array IP: " + Arrays.toString(bytes)); System.out.println(address);// Direct output of InetAddress object // Getting an InetAddress instance from the machine name // InetAddress address2=InetAddress.getByName("laurenyang"); InetAddress address2 = InetAddress.getByName("1.1.1.10"); System.out.println("Name of calculation:" + address2.getHostName()); System.out.println("IP Address:" + address2.getHostAddress()); } }
Console Printing:
Computational name: chun chunchunhudell**
IP address: 169.254.119. **
IP in byte array form: [-87, -2, 119, **]
chunchunhudell**/169.254.119.**
Computational name: 1.1.1. **
IP address: 1.1.1. **
Three, class URL
1. Uniform Resource Locator (URLs) Uniform Resource Locator, which represents the address of a resource on the Internet. Protocol Name: Resource Name
import java.net.MalformedURLException; import java.net.URL; /* * URL common method */ public class Test02 { public static void main(String[] args) { try { //Create a URL instance URL imooc=new URL("http://www.imooc.com"); //The latter represents the parameter, the latter represents the anchor point. URL url=new URL(imooc, "/index.html?username=tom#test"); System.out.println("Agreement:"+url.getProtocol()); System.out.println("Host:"+url.getHost()); //If no port number is specified, the default port number is used, and the getPort() method returns a value of -1. System.out.println("Port:"+url.getPort()); System.out.println("File path:"+url.getPath()); System.out.println("Filename:"+url.getFile()); System.out.println("Relative path:"+url.getRef()); System.out.println("Query string:"+url.getQuery()); } catch (MalformedURLException e) { e.printStackTrace(); } } }
Printer input:
Protocol: http
Host: www.imooc.com
Port: -1
File path: / index.html
File name: / index.html?username=tom
Relative path: test
Query string: username=tom
2. Use URL to read web pages
The input stream of the specified resource can be obtained by the openStream() method of the URL object, through which the resources on the web page can be read or accessed.
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.net.MalformedURLException; import java.net.URL; /* * Use URL to read web page content */ public class Test03 { public static void main(String[] args) { try { //Create a URL instance URL url = new URL("http://www.baidu.com"); //Obtain byte input stream of resource represented by URL object through open Stream method of URL InputStream is = url.openStream(); //Converting byte input stream to character input stream InputStreamReader isr = new InputStreamReader(is, "utf-8"); //Add buffers to character input streams BufferedReader br = new BufferedReader(isr); String data = br.readLine();//Read data while (data != null) {//Loop read data System.out.println(data);//output data data = br.readLine(); } br.close(); isr.close(); is.close(); } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Console Printing:
Http://s1.bdstatic.com/r/www/cache/bdorz/baidu.min.css> Baidu, you know
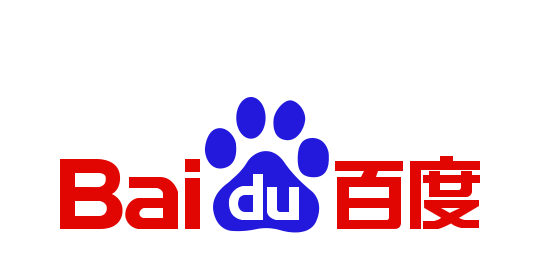
http://home.baidu.com > About Baidu http://ir.baidu.com>About Baidu
©2017 Baidu http://www.baidu.com/duty/ > Must read before using Baidu. http://jianyi.baidu.com/ Class = cp-feedback > Opinion Feedback Beijing ICP Certificate 030173
The above is the basic knowledge of Socket, to share with you!
This is my public name. Focusing on two-dimensional codes, there will be more blog posts, which can help you improve your thinking on the Internet. What are the problems? Let's talk together.