(1) . summary
1, Classification of loop statements
1. while loop
The while cycle first checks the cycle continuation condition. If the condition is true, the loop body is executed; if the condition is false, the loop ends
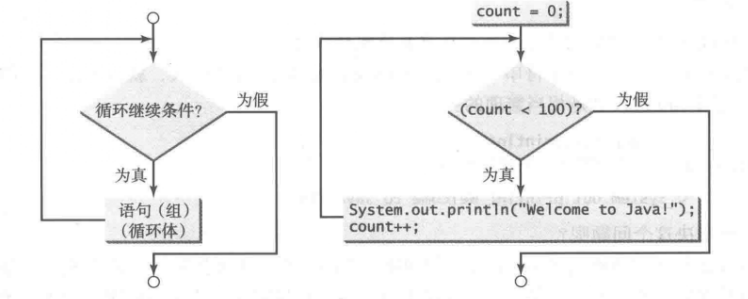
2. do - while loop
Similar to the while loop, only the do - while loop executes the loop body first, and then checks the loop continue condition to determine whether to continue or terminate
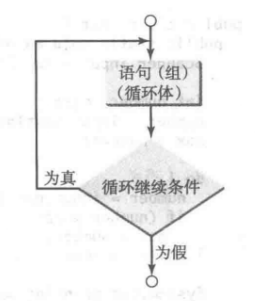
3. for loop
Before the for loop is executed, check the loop continue condition
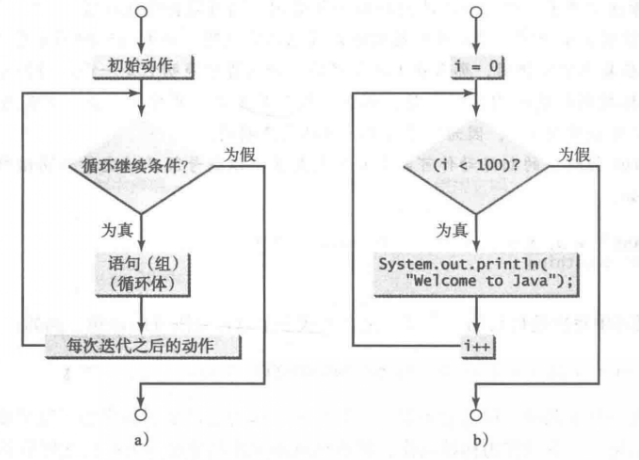
2, When and what cycle to use
1. Both the while loop and for loop are called pretest loop, because the continuation condition is executed in the loop body
Pre detected, do - while loops are called post test loops because the loop condition is after the loop body executes
Detected. Three kinds of loop statements, while, do - while and for, are equivalent in expression. That is, you can write a loop using one of these three forms.
2, difference
If you already know the number of repetitions in advance, use the for loop
If the number of repetitions cannot be determined, use a while loop
If you need to execute the body of the loop before verifying the continuation condition, replace the while loop with a do - while loop
3, Loop nesting
1, concept
A nested loop consists of an outer loop and one or more inner loops. Each time the outer loop is repeated, it will enter the inner loop again, and then start again.
2, example
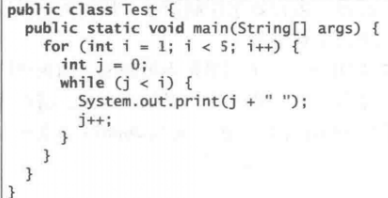
4, Keywords break and continue
1. Keyword break: immediately terminate the innermost loop containing break.
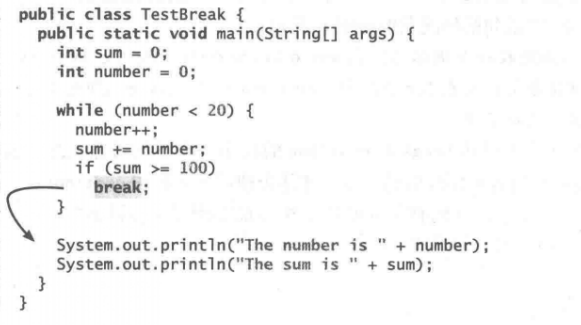
16. The keyword continue only terminates the current iteration.
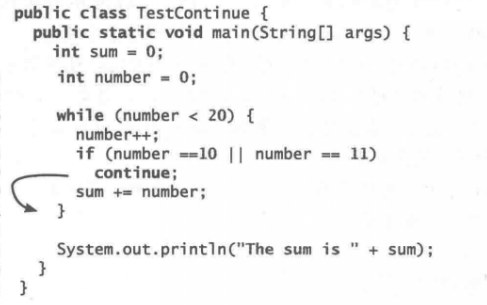
(2) Exercises
1,
import java.util.Scanner; public class Test12 { public static void main(String[] args) { System.out.println("Please enter a 1~15 Integer between:"); Scanner scanner = new Scanner(System.in); int n = scanner.nextInt(); int[][] array = new int[n][2*n+1]; for (int i = 0; i < n; i++) { for (int j = n-i; j <= n + i; j++){ array[i][j]=Math.abs(n-j)+1; } } for(int i=0;i<n;i++){ for (int j = 0; j < 2*n + 1; j++){ System.out.print(array[i][j]==0?" ":array[i][j]+" "); } System.out.println(); } } }
2,
Pattern1: public class Pattern1{ public static void main(String[] args) { for(int i = 1;i<=6;i++){ for(int j = 1;j<=i;j++) System.out.print(j); System.out.println(); } } } Pattern2: public class Pattern2{ public static void main(String[] args) { for(int i = 6;i>=1;i--){ for(int j = 1;j<=i;j++) System.out.print(j); System.out.println(); } } } Pattern3 : public class Pattern3{ public static void main(String[] args) { for(int i = 1;i<=6;i++){ for(int j = 1; j<=(6-i);j++) System.out.print(" "); for(int j = i;j>=1;j--) System.out.print(j); System.out.println(); } } } Pattern4: public class Pattern4{ public static void main(String[] args) { for(int i = 1;i<=6;i++){ for(int j = 1; j<=(i-1);j++) System.out.print(" "); for(int j = 1;j<=(7-i);j++) System.out.print(j); System.out.println(); } } }
3,
import java.util.*; public class Home3_15 { public static void main(String[] args) { Scanner sc=new Scanner(System.in); System.out.print("Enter the number of lines to print: "); int x=sc.nextInt(); for(int j=1;j<=x;j++) {//This for loop is used to control the number of rows for(int z=1;z<=x-j;z++) { System.out.print(" "); } /* * The above for loop is used to control the number of spaces in front of each line, so that it can be printed in the shape of a triangle * For example, the first line prints x-1 spaces, the second line prints x-2 spaces, and so on */ for(int i=1;i<=j+1;i++) { if(i<=j) { //It is mainly to understand the meaning of% 4d, that is, when the following numbers are printed, they must occupy four spaces. When the numbers are less than four spaces, they will be filled with spaces. Because no numbers occupy more than four spaces, they will still be separated by spaces. System.out.printf("%4d",(int)Math.pow(2, i-1)); } else { for(int y=j-2;y>=0;y--) { System.out.printf("%4d",(int)Math.pow(2,y)); } } } /* * The above nested loop is divided into two parts for printing. The statement in if is to print the first j numbers in sequence * When the outer loop i is equal to j+1, directly enter the for loop in else to print the next j-1 number */ System.out.println();//Control the line break after a line is typed } } }