I. Introduction
With the development of enterprise systems, applications are mostly distributed, which depends heavily on the stability of the network. However, due to the inherent instability of the network, it is necessary to consider how to ensure the robustness of the application when the network is unstable. Setting up network timeouts is one of the means to ensure application robustness. After setting the network timeout setting, the request will be forced to terminate if the setting time is not completed, which ensures that the program does not appear unlimited thread blocking, and effectively improves the usability of the application.
2. Comparing the situation of no timeout with that of setting timeout
1. Network Request Legend:
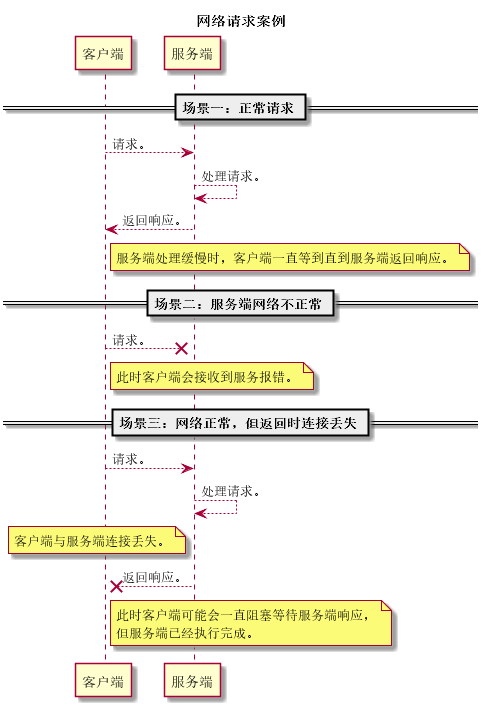
2. After setting the timeout time, request legend:
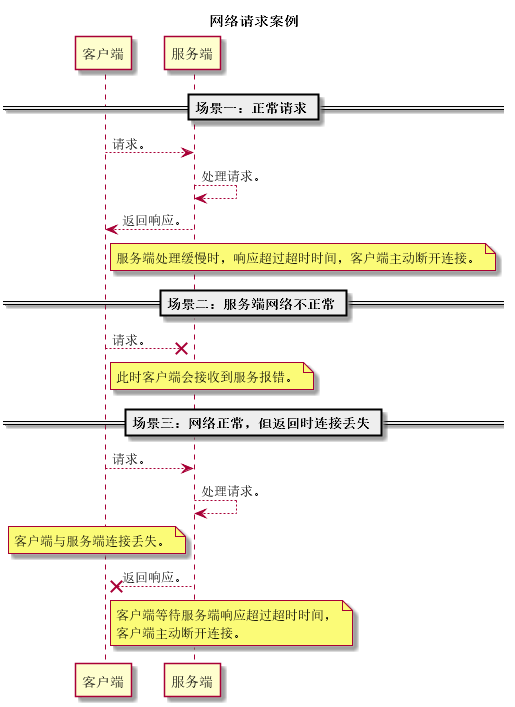
3. Common network timeout settings
1. httpclient timeout settings (Spring beans)
-
To configure
<bean id="multiThreadedHttpConnectionManager" class="org.apache.commons.httpclient.MultiThreadedHttpConnectionManager"> <property name="params"> <bean class="org.apache.commons.httpclient.params.HttpConnectionManagerParams"> <property name="maxTotalConnections" value="${maxTotalConnections:300}" /> <property name="defaultMaxConnectionsPerHost" value="${defaultMaxConnectionsPerHost:300}" /> <!-- Connection timeout, milliseconds. --> <property name="connectionTimeout" value="${connectTimeout:10000}" /> <!-- socket Timeout, milliseconds. --> <property name="soTimeout" value="${readTimeout:600000}" /> <property name="staleCheckingEnabled" value="${staleCheckingEnabled:true}" /> </bean> </property> </bean> <bean id="httpClient" class="org.apache.commons.httpclient.HttpClient"> <constructor-arg> <ref bean="multiThreadedHttpConnectionManager" /> </constructor-arg> </bean>
httpinvoker usage scenario
Configure HttpInvokerRequest Executor, override Simple HttpInvokerProxyFactoryBean, which is used by default in HttpInvokerRequest Executor, and configure network timeout. See Configuration.
<bean id="httpInvokerRequestExecutor" class="org.springframework.remoting.httpinvoker.CommonsHttpInvokerRequestExecutor"> <constructor-arg> <ref bean="httpClient" /> </constructor-arg> </bean> <bean id="xxxxService" class="org.springframework.remoting.httpinvoker.HttpInvokerProxyFactoryBean"> <property name="serviceUrl" value="${xxxxServiceUrl}" /> <property name="serviceInterface" value="com.xxxxService" /> <property name="httpInvokerRequestExecutor" ref="httpInvokerRequestExecutor" /> </bean>
2. HttpClient timeout settings (hard-coded)
-
Example
RequestConfig config = RequestConfig.custom() .setSocketTimeout(1*1000) // Socket socket timeout, milliseconds. .setConnectionRequestTimeout(1*1000) //When using connection pools to manage connections, the connection timeout time, in milliseconds, is obtained from the connection pool. .setConnectTimeout(5*1000) // Connection establishment timeout in milliseconds. .build(); CloseableHttpClient httpClient = HttpClients.custom() .setDefaultRequestConfig(config) // .build(); CloseableHttpResponse httpResponse = httpClient.execute(httpGet); // Execution request
3. Mail timeout settings
Projects based on Spring Framework can be easily used
Org. spring framework. mail. javamail. JavaMailSenderImpl implements mail reminders and other functions.
-
To configure
<bean id="mailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl" p:host="${mailSender.host}" p:username="${mailSender.username}" p:password="${mailSender.password}"> <property name="javaMailProperties"> <props> <prop key="mail.smtp.auth">${mailSender.smtp.auth:true} </prop> <prop key="mail.smtp.timeout">${mailSender.smtp.timeout:10000} </prop> <prop key="mail.smtp.connectiontimeout">${mailSender.smtp.connectiontimeout:10000} </prop> </props> </property> </bean>
-
Description of Java Mail Properties
- mail.smtp.timeout: Smtp mail server read timeout.
- Mail. smtp. connection timeout: SMTP mail server connection timeout.
- mail.smtp.auth: authenticate users.
Note: The list of property parameter names can be queried for JavaMail API documentation.
-
Reference resources