After working for many years, I found that there are many tool class libraries that can greatly simplify the amount of code and improve development efficiency, but junior developers don't know. These class libraries have long become the industry standard class libraries, and they are also used in large companies. If someone told me to use these tool class libraries when I first started working, how good it would be!
Take a look at the tool class libraries you have also used.
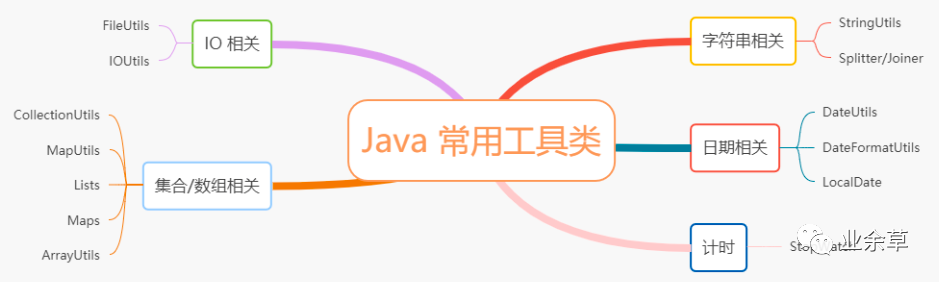
Java's own tools and methods
The List collection is spliced into comma separated strings
// How to splice a list set into comma separated strings a,b,c List<String> list = Arrays.asList("a", "b", "c"); // The first method can use stream String join = list.stream().collect(Collectors.joining(",")); System.out.println(join); // Output a,b,c // The second method, in fact, String also has a join method to realize this function String join = String.join(",", list); System.out.println(join); // Output a,b,c
Compare two strings for equality, ignoring case
if (strA.equalsIgnoreCase(strB)) { System.out.println("equal"); }
Compares whether two objects are equal
When we use equals to compare whether two Objects are equal, we also need to judge the null of the object on the left, otherwise a null pointer exception may be reported. We can use the method encapsulated by Objects in java.util package to compare whether they are equal.
Objects.equals(strA, strB);
The source code is like this
public static boolean equals(Object a, Object b) { return (a == b) || (a != null && a.equals(b)); }
Intersection of two List sets
List<String> list1 = new ArrayList<>(); list1.add("a"); list1.add("b"); list1.add("c"); List<String> list2 = new ArrayList<>(); list2.add("a"); list2.add("b"); list2.add("d"); list1.retainAll(list2); System.out.println(list1); // Output [a, b]
apache commons tool class library
apache commons is the most powerful and widely used tool class library. There are many sub libraries. Here are some of the most commonly used tools
Commons Lang, enhanced version of java.lang
It is recommended to use commons Lang 3. Some APIs have been optimized. The original commons Lang has stopped updating.
Maven's dependencies are:
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.12.0</version> </dependency>
Empty string
The passed parameter CharSequence type is the parent class of String, StringBuilder and StringBuffer, which can be directly null judged by the following methods. The following is the source code:
public static boolean isEmpty(final CharSequence cs) { return cs == null || cs.length() == 0; } public static boolean isNotEmpty(final CharSequence cs) { return !isEmpty(cs); } // Blank characters in the string, such as spaces, line breaks, and tabs, will be removed during blank judgment public static boolean isBlank(final CharSequence cs) { final int strLen = length(cs); if (strLen == 0) { return true; } for (int i = 0; i < strLen; i++) { if (!Character.isWhitespace(cs.charAt(i))) { return false; } } return true; } public static boolean isNotBlank(final CharSequence cs) { return !isBlank(cs); }
Capitalize initial
String str = "yideng"; String capitalize = StringUtils.capitalize(str); System.out.println(capitalize); // Output Yideng
Repeat splice string
String str = StringUtils.repeat("ab", 2); System.out.println(str); // Output abab
format date
No more handwritten SimpleDateFormat formatting.
// Date type to String type String date = DateFormatUtils.format(new Date(), "yyyy-MM-dd HH:mm:ss"); System.out.println(date); // Output 2021-05-01 01:01:01 // String type to Date type Date date = DateUtils.parseDate("2021-05-01 01:01:01", "yyyy-MM-dd HH:mm:ss"); // Calculate the date after one hour Date date = DateUtils.addHours(new Date(), 1);
Packaging temporary objects
When a method needs to return two or more fields, we usually encapsulate it as a temporary object. Now we don't need it with Pair and Triple.
// Return two fields ImmutablePair<Integer, String> pair = ImmutablePair.of(1, "yideng"); System.out.println(pair.getLeft() + "," + pair.getRight()); // Output 1,yideng // Return three fields ImmutableTriple<Integer, String, Date> triple = ImmutableTriple.of(1, "yideng", new Date()); System.out.println(triple.getLeft() + "," + triple.getMiddle() + "," + triple.getRight()); // Output 1,yideng,Wed Apr 07 23:30:00 CST 2021
Ommons collections collection tool class
Maven's dependencies are:
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-collections4</artifactId> <version>4.4</version> </dependency>
Set empty judgment
Encapsulates the method of set empty judgment. The following is the source code:
public static boolean isEmpty(final Collection<?> coll) { return coll == null || coll.isEmpty(); } public static boolean isNotEmpty(final Collection<?> coll) { return !isEmpty(coll); } // Intersection of two sets Collection<String> collection = CollectionUtils.retainAll(listA, listB); // Union of two sets Collection<String> collection = CollectionUtils.union(listA, listB); // Difference set of two sets Collection<String> collection = CollectionUtils.subtract(listA, listB);
Common bean utils operand
Maven dependency:
<dependency> <groupId>commons-beanutils</groupId> <artifactId>commons-beanutils</artifactId> <version>1.9.4</version> </dependency>
public class User { private Integer id; private String name; }
Set object properties
User user = new User(); BeanUtils.setProperty(user, "id", 1); BeanUtils.setProperty(user, "name", "yideng"); System.out.println(BeanUtils.getProperty(user, "name")); // Output yideng System.out.println(user); // Output {"id":1,"name":"yideng"}
Object and map rotate with each other
// Object to map Map<String, String> map = BeanUtils.describe(user); System.out.println(map); // Output {"id":"1","name":"yideng"} // map to object User newUser = new User(); BeanUtils.populate(newUser, map); System.out.println(newUser); // Output {"id":1,"name":"yideng"}
Commons IO file stream processing
Maven dependency:
<dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.8.0</version> </dependency>
File processing
File file = new File("demo1.txt"); // read file List<String> lines = FileUtils.readLines(file, Charset.defaultCharset()); // write file FileUtils.writeLines(new File("demo2.txt"), lines); // Copy file FileUtils.copyFile(srcFile, destFile);
Google Guava tool class library
Maven dependency:
<dependency> <groupId>com.google.guava</groupId> <artifactId>guava</artifactId> <version>30.1.1-jre</version> </dependency>
Create collection
List<String> list = Lists.newArrayList(); List<Integer> list = Lists.newArrayList(1, 2, 3); // Reverse list List<Integer> reverse = Lists.reverse(list); System.out.println(reverse); // Output [3, 2, 1] // There are too many elements in the list set, which can be divided into several sets, with 10 elements in each set List<List<Integer>> partition = Lists.partition(list, 10); Map<String, String> map = Maps.newHashMap(); Set<String> set = Sets.newHashSet();
Multimap a key can map a HashMap of multiple value s
Multimap<String, Integer> map = ArrayListMultimap.create(); map.put("key", 1); map.put("key", 2); Collection<Integer> values = map.get("key"); System.out.println(map); // Output {key":[1,2]} // It can also return the bloated Map you used before Map<String, Collection<Integer>> collectionMap = map.asMap();
It's easy and concise to save you from creating map < string, list >
BiMap is a HashMap that can't even duplicate value s
BiMap<String, String> biMap = HashBiMap.create(); // If the value is repeated, the put method throws an exception unless the forcePut method is used biMap.put("key","value"); System.out.println(biMap); // Output {"key":"value"} // Since value cannot be repeated, why not implement a method to flip key/value? It already exists BiMap<String, String> inverse = biMap.inverse(); System.out.println(inverse); // Output {"value":"key"}
This is actually a two-way mapping, which is very practical in some scenarios.
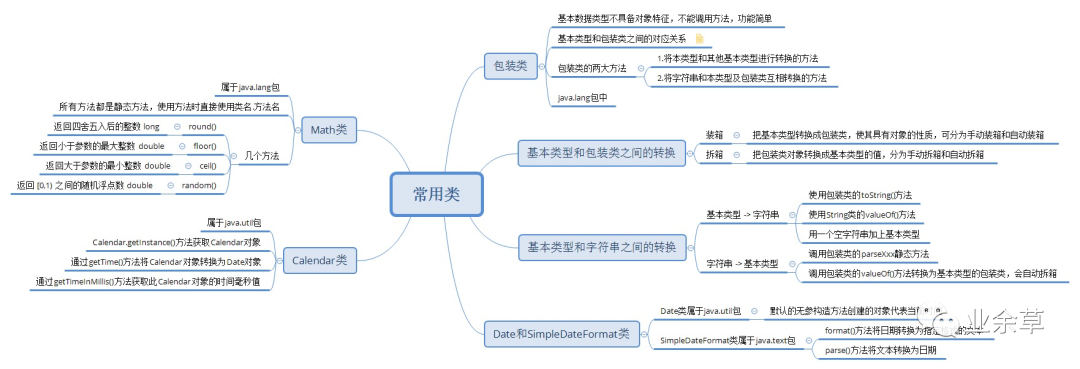
Table is a HashMap with two key s
// A group of users, grouped by age and gender Table<Integer, String, String> table = HashBasedTable.create(); table.put(18, "male", "yideng"); table.put(18, "female", "Lily"); System.out.println(table.get(18, "male")); // Output yideng // This is actually a two-dimensional Map, which can view row data Map<String, String> row = table.row(18); System.out.println(row); // Output {"male": "yideng", "female": "Lily"} // View column data Map<Integer, String> column = table.column("male"); System.out.println(column); // Output {18:"yideng"}
Multiset a Set used for counting
Multiset<String> multiset = HashMultiset.create(); multiset.add("apple"); multiset.add("apple"); multiset.add("orange"); System.out.println(multiset.count("apple")); // Output 2 // View de duplicated elements Set<String> set = multiset.elementSet(); System.out.println(set); // Output ["orange","apple"] // You can also view elements that have not been de duplicated Iterator<String> iterator = multiset.iterator(); while (iterator.hasNext()) { System.out.println(iterator.next()); } // You can also manually set the number of occurrences of an element multiset.setCount("apple", 5);
The above is personal experience. I hope I can give you a reference. If there are mistakes or not considered completely, spray gently.