Topic: a company organizes annual meeting. There are two entrances when the conference entrances. Every employee can get a double chromosphere lottery ticket when entering, assuming that the company has 100 employees, using multithreading to simulate the admission process of annual meeting.
The number of entries per entry and the number of lottery tickets received by each employee were counted separately. The print format after the thread runs is as follows:
Employees numbered: 2 enter from the back door! The number of the two-color ball lottery ticket they get is: [17, 24, 29, 30, 31, 32, 07]
Employees numbered 1 enter from the back door! The number of the two-color ball lottery ticket they get is: [06, 11, 14, 22, 29, 32, 15]
//.....
A total of 13 employees were admitted from the back door.
A total of 87 employees were admitted from the front door.
Analysis: two entries correspond to two threads. The method of obtaining the lottery ticket is overlaid with the run implementation of the Runnable interface. I call this class of Runnable interfaces the Paper class, which uses the id field to record employee numbers, and provides get and set methods. Third threads are set up to control the access of 100 employees in a random way, because it is the employees who enter the entrance and the entrance is the corresponding lottery method. So I used the wake-up mechanism. )
Code:
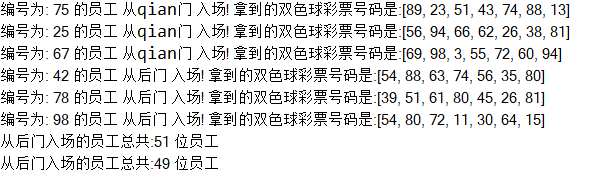
class Paper implements Runnable { boolean bFlag = false; private int id; public void setId(int id) { this.id = id; } public int getId() { return this.id; } public String position; private List<String> listPosCount = new ArrayList<String>(); private HashMap<Integer, int[]> dic = new HashMap<Integer, int[]>(); private List<Integer> myList = new ArrayList<Integer>(); private int[] getNumbers(int size) { int numbers[] = new int[size]; for (int i = 0; i < size;) { boolean flag = false; numbers[i] = (int) (Math.random() * 100); for (int j = 0; j < i; j++) { if (numbers[j] == numbers[i]) { flag = true; break; } } if (flag == true) { continue; } i++; } return numbers; } @Override public void run() { synchronized (this) { while (myList.size() < 100) { while (!bFlag) { try { this.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } this.bFlag = false; if (!myList.contains(id)) { myList.add(id); if (position == "qian door" || position == "back door") listPosCount.add(position); int nums[] = getNumbers(7); dic.put(id, nums); System.out .println("Number is: " + id + " Employees from" + position + " admission! The number of the two-colour ball lottery ticket you get is ___________:" + Arrays.toString(nums)); } else { } this.notifyAll(); } int a = 0, b = 0; for (String str : listPosCount) { if (str == "back door") a++; if (str == "qian door") b++; } System.out.println("The total number of employees admitted from the back door:" + a + " Position employee"); System.out.println("The total number of employees admitted from the back door:" + b + " Position employee"); } } }
//--------------------------------------------------------------------------------
// Test main function
public class ThreadLearn2 { public static void main(String[] args) { Paper p = new Paper(); Thread th1 = new Thread(p); Thread th2 = new Thread(p); Runnable run1 = new Runnable() { @Override public void run() { synchronized (p) { List<Integer> list = new ArrayList<Integer>(); while (list.size() < 100) { int i = (int) (Math.random() * 100); if (list.indexOf(i) == -1) { p.setId(i); list.add(i); p.bFlag = true; int num = (int) (Math.random() * 100) % 2; if (num == 1) { p.position = "back door"; } else { p.position = "qian door"; } p.notifyAll(); while (p.bFlag) { try { p.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } } } } } }; Thread th3 = new Thread(run1); th1.start(); th2.start(); th3.start(); } }
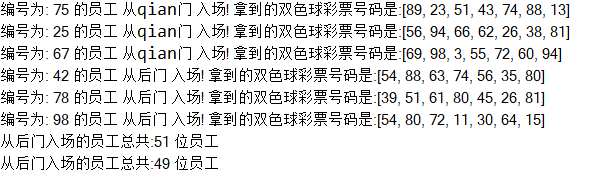