Java in those let you love the manual tool library, refining the amount of code
1, New features of JDK1.8 Stream
1. Common generation methods of Stream
① Collections in the Collection system can generate streams using the default method stream()
//list to stream List<String> list = new ArrayList<>(); Stream<String> listStream = list.stream(); //Set to stream Set<String> set = new HashSet<>(); Stream<String> setStream = set.stream(); //map to stream Map<String, Integer> map = new HashMap<>(); Stream<String> keyStream = map.keySet().stream(); Stream<Integer> valueStream = map.values().stream(); Stream<Map.Entry<String, Integer>> entryStream = map.entrySet().stream();
② Array to stream
//The first method: use the java.util.Arrays.stream(T[] array) method to create a stream with an array int[] array={1,3,5,6,8}; IntStream stream = Arrays.stream(array); //The second method: the Stream can be generated through the static method of(T... values) of the Stream interface String[] strArray = {"hello", "world", "java"}; Stream<String> strArrayStream = Stream.of(strArray); Stream<String> strArrayStream2 = Stream.of("hello", "world", "java"); Stream<Integer> intStream = Stream.of(10, 20, 30);
2,void forEach(Consumer<? super T> action)
Stream<String> stringStream = Stream.of("Sedum", "Xuejian", "Changqing", "Zixuan"); stringStream.forEach(System.out::println); //Print results: [Jingtian "," Xuejian "," Changqing "," Zixuan "]
3,Stream filter(Predicate predicate)
(Note: used to filter data in convection)
List<String> nameList = Arrays.asList("Sedum", "Xuejian", "Changqing", "Zixuan"); nameList.stream().filter(s -> s.startsWith("purple")).forEach(System.out::println); //Print result: Zixuan
4,Stream map(Function<? super T, ? extends R> mapper)
(Note: Elements in a stream can be mapped to another stream)
List<Integer> num = Arrays.asList(1, 2, 3, 4, 5); num.stream().map(n -> n * 2).forEach(System.out::println); //Print results: [2, 4, 6, 8, 10]
5,Stream flatMap(Function function)
(Note: flatmap is an intermediate operation of a stream. The stream is "flattened". It is the same as the stream map. It is a collection type of stream intermediate operation. However, unlike map, it can split (slice) a single element in the stream. On the other hand, using it means using a double for loop.)
// Map generates a 1:1 map, and each input element is transformed into another element according to the rules. There are also some scenarios that have a one to many mapping relationship. At this time, flatMap is required String[] strs = {"java8", "is", "easy", "to", "use"}; List<String[]> distinctStrs = Arrays.stream(strs) .map(str -> str.split("")) // Map to stream < string [] > .distinct().collect(Collectors.toList()); //distinct operation is to de duplicate the string array as a unit /* After executing the map operation, we get a stream containing multiple string arrays. The print results are as follows [j, a, v, a, 8] [i, s] [e, a, s, y] [t, o] [u, s, e] */ List<String> distinctStr = Arrays.stream(strs) .map(str -> str.split("")) // Map to stream < string [] > .flatMap(Arrays::stream) // Flatten to stream < string > .distinct().collect(Collectors.toList()); /* flatMap Convert the stream < string [] > mapped by the map into the stream < string > mapped by each string array, and then flatten these small streams into a large stream composed of all strings, So that we can achieve our goal. Print results: [j, a, v, 8, i, s, e, y, t, o, u] */
6,Stream limit(long maxSize)
(Description: return the stream composed of elements in this stream, and intercept the data of the specified number of parameters before)
List<String> limitList = Arrays.asList("Sedum", "Xuejian", "Changqing", "Zixuan"); //Take the first three data and output it on the console limitList.stream().limit(3).forEach(System.out::println); //Print results: [Jingtian "," Xuejian "," Changqing "]
7,Stream skip(long n)
(Description: skip the data of the specified number of parameters and return the stream composed of the remaining elements of the stream)
//Skip 3 elements and output the remaining elements on the console List<String> list = Arrays.asList("Sedum", "Xuejian", "Changqing", "Zixuan"); list.stream().skip(3).forEach(System.out::println); //Print result: [Zixuan]
8,static Stream concat(Stream a, Stream b)
(Note: merge two streams a and b into one stream)
List<String> concatList1 = Arrays.asList("Sedum", "Xuejian", "Changqing", "Zixuan"); List<String> concatList2 = Arrays.asList("Chonglou", "Maomao", "Bi Ping", "nightshade"); Stream<String> stream1 = concatList1.stream(); Stream<String> stream2 = concatList2.stream(); Stream.concat(stream1, stream2).forEach(System.out::println); // Print results: [Jingtian "," Xuejian "," Changqing "," Zixuan "," Chonglou "," Maomao "," Biping "," Solanum nigrum "]
9,Stream distinct()
(Note: the same elements in the convection are removed)
List<String> distinctList = Arrays.asList("Sedum", "Xuejian", "Changqing", "Zixuan", "Zixuan", "Xuejian"); distinctList.stream().distinct().forEach(System.out::println); // Print results: [Jingtian "," Xuejian "," Changqing "," Zixuan "]
10,Stream sorted()
(Description: returns the stream composed of the elements of this stream, which is sorted according to the natural order)
//Title: output data on the console in alphabetical order. sorted() is in positive order List<String> sortList = Arrays.asList("Lucy", "Jack", "Anny", "Vincent", "Charles","William"); sortList.stream().sorted().forEach(System.out::println); //Print result: [Anny,Charles,Jack,Lucy, Vincent, William] //Title: output the data on the console from short to long in the order of name length sortList.stream().sorted(Comparator.comparingInt(String::length)) .forEach(System.out::println); //Print result: [Lucy,Jack,Anny,Vincent,Charles,William]
11,Stream parallelStream()
(Note: parallel operation and multi-threaded execution are better than stream() in large amount of data. The underlying idea of parallelStream() is that ForkJoinPool is mainly used to solve problems using divide and conquer algorithm)
List<String> stringList = new ArrayList<>(); for (int i = 0; i < 10; i++) { stringList.add("The first" + i + "Data bar"); } long parallelStreamNowTime = System.currentTimeMillis(); stringList.parallelStream().forEach(s -> { try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } }); long parallelStreamTime = System.currentTimeMillis(); System.out.println("Stream It takes time" + (parallelStreamTime - parallelStreamNowTime)); //Print result: Stream takes 2027
PS: in addition to directly creating parallel streams, you can also convert sequential streams into parallel streams through parallel()
Optional<Integer> findFirst = list.stream().parallel().filter(x->x>6).findFirst();
12,count()
(Description: returns the number of elements in the collection stream)
List<String> countList = Arrays.asList("Lucy", "Jack", "Anny", "Vincent","Charles", "William","William"); long count1 = countList.stream().count(); System.out.println("The total number of elements is:"+count1); // Print result: the total number of elements is: 7 long count2 = countList.stream().distinct().count(); System.out.println("The number of elements after de duplication is:"+count2); // Print result: the number of elements after de duplication is 6
13,boolean allMatch(Predicate predicate)
(Note: allMatch means to judge the elements in the condition, and return true if all elements meet the condition)
List<Integer> integerList = Arrays.asList(1,2,3,4,5); if(integerList.stream().allMatch(i->i>3)){ System.out.println( "All values are greater than 3"); }
14,boolean anyMatch(Predicate predicate)
(Note: anyMatch means that in the judged conditions, if any element meets the conditions, it returns true)
List<Integer> integerList = Arrays.asList(1,2,3,4,5); if(integerList.stream().anyMatch(i->i>3)){ System.out.println( "There is a value greater than 3"); }
15,boolean noneMatch(Predicate predicate)
(Note: noneMatch is the opposite of allMatch. Judge the elements in the condition and return true only if all of them do not meet the condition)
List<Integer> integerList = Arrays.asList(1,2,3,4,5); if(integerList.stream().noneMatch(i -> i > 3)){ System.out.println("All values are less than 3"); }
14,A[] toArray(IntFunction<A[]> generator);
(Note: use the provided generator function to return an array containing the elements of this stream to allocate the returned array and any other arrays that may be required for partition execution or resizing.)
15,Stream concat(Stream a, b)
(Description: merge 2 stream s)
List<String> strings = Arrays.asList("abc", "def", "gkh", "abc"); //concat merge flow List<String> strings2 = Arrays.asList("xyz", "jqx"); List<String> concatList = Stream.concat(strings2.stream(),strings.stream()) .collect(Collectors.toList()); System.out.println(concatList); //Print result: [xyz, jqx, abc, def, gkh, abc]
16,IntSummaryStatistics summaryStatistics()
(Note: perform statistical analysis on the data in the stream)
//Statistics on arrays, such as using List<Integer> number = Arrays.asList(1, 2, 5, 4); IntSummaryStatistics statistics = number.stream().mapToInt((x) -> x).summaryStatistics(); System.out.println("Maximum number of in the list : "+statistics.getMax()); System.out.println("Minimum number in the list : "+statistics.getMin()); System.out.println("average : "+statistics.getAverage()); System.out.println("Sum of all numbers : "+statistics.getSum());
17,Optional findAny()
(Note: the elements returned by findAny() operation are uncertain. Calling findAny() multiple times for the same list may return different values. findAny() is used for more efficient performance.
① In the case of serial flow, the first result that meets the conditions will generally be returned;
List<String> list1 = Arrays.asList("A1", "B1", "C1", "A2", "B2", "C2", "A3", "B3","C3"); for(int i=0;i<10;i++) { Optional<String> c = list1.stream().filter(s -> s.contains("A")).findAny(); System.out.println("====Serial stream findAny()======" + c.get()); } //Print results: // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1 // ====Serial stream findany() = = = = A1
② In the case of parallel flow, if an element is randomly selected from a given element, it cannot be guaranteed to be the first.)
List<String> list1 = Arrays.asList("A1", "B1", "C1", "A2", "B2", "C2", "A3", "B3","C3"); Optional<String> a = list1.parallelStream().filter(s -> s.contains("A")).findAny(); System.out.println("====findAny()======" + a.get()); //Print result: any one of A1, A2 or A3 is printed each time // ====Parallel stream findany() = = = = = A3 // ====Parallel stream findany() = = = = = A3 // ====Parallel stream findany() = = = = A2 // ====Parallel stream findany() = = = = A1 // ====Parallel stream findany() = = = = A1 // ====Parallel stream findany() = = = = A2 // ====Parallel stream findany() = = = = = A3 // ====Parallel stream findany() = = = = = A3 // ====Parallel stream findany() = = = = = A3 // ====Parallel stream findany() = = = = A2
18,Optional findFirst()
(Description: returns the first element in the Stream)
List<String> list2 = Arrays.asList("A1", "B1", "C1", "A2", "B2", "C2", "A3", "B3","C3"); Optional<String> b = list2.parallelStream().filter(s -> s.contains("B")).findFirst(); System.out.println("====findFirst()====" + b.get()); // Print result: = = = findFirst()====B1
19,Optional max(Comparator comparator)
(Description: returns the element with the largest result after comparison by the comparator)
//Gets the longest element in the String collection. List<String> maxList = Arrays.asList("Lucy", "Jack", "Anny", "Vincent","Charles","William"); Optional<String> max = maxList.stream(). max(Comparator.comparing(String::length)); System.out.println("Longest string:" + max.get()); //Print result: longest string: Vincent
PS: the optional min (comparator <? Super T > comparator) is similar. I won't say more!
20,Optional reduce(BinaryOperator accumulator)
(Note: calculate the value in the Stream according to the specified calculation model to get a final result)
List<Integer> reduceList = Arrays.asList(1, 3, 2, 8, 11, 4); // Summation method 1 Optional<Integer> sum = reduceList.stream().reduce((x, y) -> x + y); // Summation method 2 Optional<Integer> sum2 = reduceList.stream().reduce(Integer::sum); // Summation method 3 Integer sum3 = reduceList.stream().reduce(0, Integer::sum); // Product Optional<Integer> product = reduceList.stream().reduce((x, y) -> x * y); // Maximum method 1 Optional<Integer> max2 = reduceList.stream().reduce((x, y) -> x > y ? x : y); // Find the maximum 2 Integer max3 = reduceList.stream().reduce(1, Integer::max); System.out.println("list Summation:" + sum.get() + "," + sum2.get() + "," + sum3); //Print result: list summation: 29,29,29 System.out.println("list Quadrature:" + product.get()); //Print result: list quadrature: 2112 System.out.println("list Maximum value:" + max2.get() + "," + max3); //Print result: list maximum: 11,11
2, List to String
// How to splice a list set into comma separated strings a,b,c List<String> list = Arrays.asList("a", "b", "c"); // The first method can use stream String join = list.stream().collect(Collectors.joining(",")); System.out.println(join); // Output a,b,c // The second method, in fact, String also has a join method to realize this function. The array is combined into a single String, and the separator can be passed String join1 = String.join(",", list); System.out.println(join1); // Output a,b,c
3, Play with StringUtils tool class
String str = "I love JavaAlliance forever"; // 1. Deletes the specified character in the string and returns a string String remove = StringUtils.remove(str, "forever"); // Remove forever from string System.out.println(remove); // The print result is I love JavaAlliance // 2. Invert the string and return a string String reverse = StringUtils.reverse(str); System.out.println(reverse); // The print result is reverof ecnaillAavaJ evol I // 3. Compare whether two strings are equal. If both are null, they are also considered equal StringUtils.equals("Java", "Java"); // The result is true StringUtils.equals("", ""); // The result is true StringUtils.equals(null, null); // The result is true StringUtils.equals(null, ""); // The result is false StringUtils.equals("", null); // The result is false StringUtils.equals(null, ""); // The result is false StringUtils.equalsIgnoreCase("java", "JAVA"); // Case insensitive -- the result is true // 4. Repeat splice string String str1 = StringUtils.repeat("ab", 2); System.out.println(str1); // Output abab // 5. Capitalize initial String str2 = "javaAlliance"; String capitalize = StringUtils.capitalize(str2); System.out.println(capitalize); // Output JavaAlliance // 6. String fixed length padding StringUtils.leftPad("test", 8, "0"); // The string has a fixed length of 8 bits. If it is insufficient, fill 0 to the left StringUtils.rightPad("test", 8, "0"); // The string has a fixed length of 8 bits. If it is insufficient, fill 0 to the right // 7. String keyword replacement StringUtils.replace("aba", "a", "z") = "zbz"; // Replace all keywords by default StringUtils.replaceOnce("aba", "a", "z") = "zba";// Replace keyword, only once StringUtils.replacePattern("ABCabc123", "[^A-Z0-9]+", "") = "ABC123"; // Replace with regular expressions // 8. Concatenate arrays into strings StringUtils.join(["a", "b", "c"], ",") ; //The splicing result is "a,b,c" // 9. String splitting StringUtils.split("a..b.c", '.') //The split result is ["a", "b", "c"] StringUtils.splitByWholeSeparatorPreserveAllTokens("a..b.c", ".") //The split result is ["a", "B", "C"]
4, Play with BeanUtils tool class
User user = new User(); user.setUserName("JavaAlliance").setEmail("196432@qq.com"); // Object to map Map<String, String> map = BeanUtils.describe(user); System.out.println(map); // output // map to object User newUser = new User(); BeanUtils.populate(newUser, map); System.out.println(newUser); // output
5, Play with DateUtils/DateFormatUtils tool class
// Date type to String type String date = DateFormatUtils.format(new Date(), "yyyy-MM-dd HH:mm:ss"); System.out.println(date); // Output 2021-05-01 01:01:01 // String type to Date type. This method will compare the Date strings according to the string array in the second parameter, and select the appropriate Pattern to parse. Date date1 = DateUtils.parseDate("2021-05-01 01:01:01", new String[]{"yyyy-MM-dd HH:mm:ss"}); Date now = new Date(); // Date plus 1 day Date addDays = DateUtils.addDays(now, 1); // Date plus 33 minutes Date addMinutes = DateUtils.addMinutes(now, 33); // Date minus 233 seconds Date addSeconds = DateUtils.addSeconds(now, -233); // Determine whether Wie is on the same day boolean sameDay = DateUtils.isSameDay(addDays, addMinutes); // The filtering time is minutes and seconds. If now is 2020-05-07 22:13:00, after calling the truncate method // The return time is 2020-05-07 00:00:00 Date truncate = DateUtils.truncate(now, Calendar.DATE);
6, Play with LocalDateTime tool class
Date now = new Date(); // Convert Date to LocalDateTime. Here you specify to use the default time zone of the current system LocalDateTime localDateTime = now.toInstant().atZone(ZoneId.systemDefault()) .toLocalDateTime(); // Convert LocalDateTime to Date. Here, the default time zone of the current system is specified Date date = Date.from(localDateTime.atZone(ZoneId.systemDefault()).toInstant()); // According to yyyy MM DD HH: mm: SS conversion time LocalDateTime dateTime = LocalDateTime.parse("2020-05-07 22:34:00", DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss")); // Format LocalDateTime as a string String format = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss").format(dateTime); //LocalDateTime gets the current time, year and month LocalDateTime now = LocalDateTime.now(); // year int year = now.getYear(); // month int month = now.getMonthValue(); // day int day = now.getDayOfMonth(); // LocalDateTime adds or subtracts the date to get the time of the next day LocalDateTime now = LocalDateTime.now(); // Current time plus one day LocalDateTime plusDays = now.plusDays(1l); // Current time minus one hour LocalDateTime minusHours = now.minusHours(1l); // There are many other ways
7, Play with CollectionUtils tool class
String[] arrayA = new String[]{"1", "2", "3", "4"}; String[] arrayB = new String[]{"3", "4", "5", "6"}; List<String> listA = Arrays.asList(arrayA); List<String> listB = Arrays.asList(arrayB); // 1. union Union System.out.println(CollectionUtils.union(listA, listB)); // Output: [1, 2, 3, 4, 5, 6] // 2. intersection System.out.println(CollectionUtils.intersection(listA, listB)); // Output: [3, 4] // 3. Complement (disjunction) disjunction of intersection System.out.println(CollectionUtils.disjunction(listA, listB)); // Output: [1, 2, 5, 6] // 4. Difference set (deduction) System.out.println(CollectionUtils.subtract(listA, listB)); // Output: [1, 2] // 1. Intersection List<String> intersectionList = new ArrayList<>(listA); intersectionList.retainAll(listB); System.out.println(intersectionList); // Output: [3, 4] // 2. Difference set List<String> differenceList = new ArrayList<>(listA); differenceList.removeAll(listB); System.out.println(differenceList); // Output: [1, 2] // 3. Union (make difference first, then add all) List<String> unionList = new ArrayList<>(listA); unionList.removeAll(listB); // unionList [1, 2] unionList.addAll(listB); // Add [3,4,5,6] System.out.println(unionList); // Output: [1, 2, 3, 4, 5, 6]
Note: there are two ways to get the intersection, i.e. intersection and retainAll. Let's talk about the differences between them. It should be noted that their return types are different. Intersection returns a new List set, while retainAll returns a bolean type. That means the retainAll method processes the original set and returns the original set, which will change the original set Content of the.
Ideas:
1. From a performance perspective, List comes with a high point because it doesn't have to create new collections.
2. It should be noted that because retainAll will change the original set, it is not suitable to use retainAll if the set needs to be used multiple times.
Note: Arrays.asList cannot perform add and remove operations when converting an array to a collection. Because an exception occurs when calling the add and remove methods of the List produced by Arrays.asList(), which is caused by Arrays.asList() The returned internal class ArrayList of the city Arrays is not java.util.ArrayList. Both the internal classes ArrayList and java.util.ArrayList of the Arrays inherit AbstractList. Remove, add and other methods. The default throw UnsupportedOperationException in the AbstractList does not do any operation. java.util.ArrayList reproduces these methods, but the internal class ArrayList of the Arrays does not, so Will throw an exception.
8, Play with Guava Toolkit
1,Multiset
What is Multiset? As the name suggests, the difference between Multiset and Set is that multiple identical objects can be saved. In JDK, there is a basic difference between List and Set, that is, List can contain multiple identical objects in order, while Set cannot be repeated and order is not guaranteed (some implementations have order, such as LinkedHashSet and SortedSet) Therefore, Multiset occupies a gray area between List and Set: repetition is allowed, but the order is not guaranteed.
Common usage scenarios: a useful function of Multiset is to track the number of each object, so you can use it for digital statistics. Common implementation methods are as follows:
String str = "Zhang San Li Si Li Si Wang Wu Wang Wu Wang Wu"; String[] strArr = str.split(" "); List<String> words = new ArrayList<>(Arrays.asList(strArr)); //Create a HashMultiset set and put the words set data into the Multiset<String> wordMultiset = HashMultiset.create(); wordMultiset.addAll(words); //Put different elements in a set for (String key : wordMultiset.elementSet()) { //View the number of specified elements System.out.println(key + "-->" + wordMultiset.count(key)); //Print result: Li Si -- > 2 sheets of three -- > 1 Wang Wu -- > 3 }
2,Multimap
Multimap can achieve the effect that one key corresponds to multiple values
Multimap<String, String> myMultimap = ArrayListMultimap.create(); myMultimap.put("Fruits", "Bannana"); myMultimap.put("Fruits", "Apple"); myMultimap.put("Fruits", "Pear"); myMultimap.put("Fruits", "Pear"); myMultimap.put("Vegetables", "Carrot"); // Query the number of stored elements in the Multimap System.out.println(myMultimap.size()); // Print result: 5 //The query key is the value corresponding to "Fruits" Collection<String> fruits = myMultimap.get("Fruits"); System.out.println(fruits);//Print results: [Bannana, Apple, Pear, Pear] Collection<String> vegetables = myMultimap.get("Vegetables"); System.out.println(vegetables); //Print result: [Carrot] // Loop iteratively traverses the value stored in the entire Multimap for (String value : myMultimap.values()) { System.out.println(value); //Print results: //Carrot //Bannana //Apple //Pear //Pear } //Remove one of the elements myMultimap.remove("Fruits", "Pear"); System.out.println(myMultimap.get("Fruits"));//Print results: [Bannana, Apple, Pear] //Replace the value content corresponding to the key "Fruits" with the set Arrays.asList("178","910","123") //The returned oldValues are the previous old values Collection<String> oldValues = myMultimap.replaceValues("Fruits", Arrays.asList("178","910","123")); System.out.println("oldValues="+oldValues);//Print result: oldValues=[Bannana, Apple, Pear] System.out.println("myMultimap="+myMultimap);//Print result: mymultimap = {vegetables = [carrot], fruits = [178, 910123]} //Remove all elements with the key "Fruits" in the Multimap myMultimap.removeAll("Fruits"); System.out.println(myMultimap.get("Fruits"));//Print result: []
3,BiMap
Bimap can be used to realize the two-way mapping requirements of key value pairs, so that we can find the corresponding value through the key, or use the value to find the corresponding key. Bimap requires that both key and value are unique. If the key is not unique, the key will be overwritten, and if the value is not unique, an error will be reported directly
//Bidirectional map BiMap<Integer,String> biMap=HashBiMap.create(); biMap.put(1,"Zhang San"); biMap.put(2,"Li Si"); biMap.put(3,"Wang Wu"); biMap.put(4,"Zhao Liu"); biMap.put(5,"Li Qi"); biMap.put(4,"Little"); //Get the value value through the key value (note that the type in the key depends on the pan line String value= biMap.get(1); System.out.println("id Is 1 value value --"+value); //Print result: value value with id 1 -- Zhang San //Get the key value through the value value int key= biMap.inverse().get("Zhang San"); System.out.println("value For Zhang San key value --"+key); //Print result: value is the key value of Zhang San -- 1 //If the key value is repeated, the vakue value will be overwritten. String valuename= biMap.get(4); System.out.println("id For 4 value value --"+valuename);//Print result: value value with id 4 -- small
Common implementations of BiMap include:
1. Hashbimap: both the key set and the value set are implemented by HashMap
2. Enumbimap: both key and value must be enum type
3. ImmutableBiMap: immutable BiMap
9, Play with FileUtils - file manipulation tool class
The file manipulation tool class provides a series of methods that allow us to quickly read and write files.
Fast file / folder copy operation, FileUtils.copyDirectory/FileUtils.copyFile
1. Gets all files in the specified folder
// Find the files in the specified folder according to the specified file suffixes, such as java,txt, etc File directory = new File("E:\\test"); FileUtils.listFiles(directory, new String[]{"txt"}, false);
2. Read all lines of the file
// To read all lines of the specified file, you do not need to use the while loop to read the stream List<String> lines = FileUtils.readLines(fileA)
3. Write file
// 1. Write a text to a file FileUtils.write(new File("D:/a/1.txt"), "File content", "UTF-8", true); // 2. Write as append FileUtils.writeStringToFile(new File("D:/a/1.txt"), "author: apple", "UTF-8", true); //3. Write multiple lines List<String> list= new ArrayList<String>(); list.add("first line"); list.add("Second line"); FileUtils.writeLines(new File("D:/a/1.txt"), list, true);
4. Read file
//read file System.out.println(FileUtils.readFileToString(new File("D:/a/1.txt"), "UTF-8")); //Return a list System.out.println(FileUtils.readLines(new File("D:/a/1.txt"), "UTF-8"));
5. Delete file / folder
// remove folders FileUtils.deleteDirectory(new File("D:/a")); // If the folder is not empty, it can still be deleted and will never throw an exception FileUtils.deleteQuietly(new File("D:/a"));
6. Copy file
//The result is that a and a1 are in the same directory FileUtils.copyDirectory(new File("D:/a"), new File("D:/a1")); //The result is to copy a to a2 FileUtils.copyDirectoryToDirectory(new File("D:/a"), new File("D:/a2")); //Copy file mode 1 FileUtils.copyFile(new File("d:/1.xml"), new File("d:/1.xml.bak")); //Copy file mode 2 Writer write = new FileWriter("D: /abc_bak.txt"); InputStream ins = new FileInputStream(new File("D: /abc.txt")); IOUtils.copy(ins, write); write.close(); IOUtils.closeQuietly(ins); //Copy files to directory FileUtils.copyFileToDirectory(new File("d:/1.xml"), new File("d:/a")); //Copy url to file FileUtils.copyURLToFile(new URL("http://www.a.com/1.xml"), new File("d:/1.xml")); //Fast download URL url = new URL("http://hzf-image-test.oss-cn-beijing.aliyuncs.com/hr_image/HF306268301810/1513632067664AbIB40pv_defalut.JPG?x-oss-process=image/resize,h_400"); File file = new File("/Users/jjs/Desktop/pic.jpg"); FileUtils.copyURLToFile(url, file);
7. Move file
//Move files or folders //static void moveDirectory(File srcDir, File destDir) FileUtils.moveDirectory(new File("D:/a1"), new File("D:/a2")); //Note that an exception will be thrown if the second parameter file does not exist //static void moveDirectoryToDirectory(File src, File destDir, boolean createDestDir) FileUtils.moveDirectoryToDirectory(new File("D:/a2"), new File("D:/a3"), true); /* The difference between the above two methods is: * moveDirectory: D:/a2 The content in is the content of D:/a1. * moveDirectoryToDirectory: D:/a2 Move folder to D:/a3 */
8. Realize fast file download
//Download method 1 URL url = new URL("http://www.baidu.com/img/baidu_logo.gif"); File file = new File("/Users/jjs/Desktop/baidu1.gif"); FileUtils.copyURLToFile(url, file); //Download method 2 InputStream in = new URL("http://www.baidu.com/img/baidu_logo.gif").openStream(); byte[] gif = IOUtils.toByteArray(in); FileUtils.writeByteArrayToFile(new File("D: /baidu2.gif"), gif); IOUtils.closeQuietly(in); //Download method 3 InputStream in3 = new URL("http://www.baidu.com/img/baidu_logo.gif").openStream(); byte[] gif3 = IOUtils.toByteArray(in3); IOUtils.write(gif3, new FileOutputStream(new File("D: /baidu3.gif"))); IOUtils.closeQuietly(in3);
Focus on advanced Java technology, system design, computer network, data structure algorithm, operating system, design mode, computer composition principle and more wonderful contents, and look forward to it
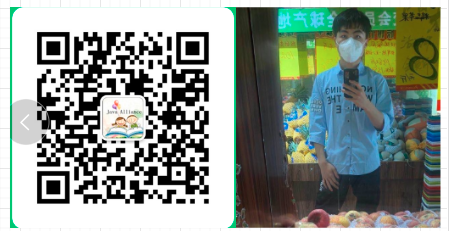