Instance - implement a simple java Web container
Technology stack
- java.net.Socket
- java.net.ServerSocket
Execution process
- Create a ServerSocket object;
- Call the accept method of the ServerSocket object and wait for the connection. If the connection succeeds, a Socket object will be returned. Otherwise, the wait will be blocked all the time.
- Get InputStream and OutputStream byte stream from Socket object, which correspond to request request and response respectively;
- Processing request: read the InputStream byte stream information, convert it into string form, and parse it. The parsing here is relatively simple, only getting the URI (Uniform Resource Identifier) information;
- Processing response: according to the parsed uri information, find the requested resource file from the web root directory, read the resource file, and write it to the OutputStream byte stream;
- Close the Socket object;
- Go to step 2 and continue to wait for the connection request;
code implementation
The server
package com.kunlinr.lifealien.servlet.server;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
public class Response {
private static final int BUFFER_SIZE = 1024;
private Request request;
private OutputStream output;
public Response(OutputStream output) {
this.output = output;
}
public void setRequest(Request request) {
this.request = request;
}
/**
* Send static resources
* @throws IOException
*/
public void sendStaticResource() throws IOException {
byte[] bytes = new byte[BUFFER_SIZE];
FileInputStream fis = null;
try {
//Write the web file to the OutputStream byte stream
File file = new File(HttpServer.WEB_ROOT, request.getUri());
if (file.exists()) {
fis = new FileInputStream(file);
List<byte[]> contentbytes = new ArrayList<>();
int ch = fis.read(bytes, 0, BUFFER_SIZE);
int size = 0;
while (ch != -1) {
contentbytes.add(bytes);
ch = fis.read(bytes, 0, BUFFER_SIZE);
size += BUFFER_SIZE;
}
String head = "HTTP/1.1 200 OK\r\n" + "Content-Type: text/html\r\n"
+ "Content-Length: "+size+"\r\n" + "\r\n";
byte[] headbytes = head.getBytes();
output.write(headbytes,0,headbytes.length);
for(byte[] contentbyte:contentbytes) {
output.write(contentbyte);
}
} else {
// file not found
String errorMessage = "HTTP/1.1 404 File Not Found\r\n" + "Content-Type: text/html\r\n"
+ "Content-Length: 23\r\n" + "\r\n" + "<h1>File Not Found</h1>";
output.write(errorMessage.getBytes());
}
} catch (Exception e) {
// thrown if cannot instantiate a File object
System.out.println(e.toString());
} finally {
if (fis != null)
fis.close();
}
}
}
Request
package com.kunlinr.lifealien.servlet.server;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
@SuppressWarnings("unused")
public class Request {
private InputStream input;
private String uri;
public Request(InputStream input) {
this.input = input;
parse();
}
/**
* Read the request information from the inputStream to get the uri value.
*/
public void parse() {
StringBuffer request = new StringBuffer(2048);
int i;
byte[] buffer = new byte[2048];
try {
i = input.read(buffer);
}catch (Exception e) {
e.printStackTrace();
i = -1;
}
for(int j=0;j<i;j++) {
request.append((char)buffer[j]);
}
uri = parseUri(request.toString());
}
/**
* requestString The form is as follows:
* GET /index.html HTTP/1.1
* Host: localhost:8080
* Connection: keep-alive
* Cache-Control: max-age=0
* ...
* Get / index.html string
* @param string
* @return
*/
private String parseUri(String string) {
int index1,index2;
index1 = string.indexOf(' ');
if (index1 != -1) {
index2 = string.indexOf(' ', index1 + 1);
if (index2 > index1)
return string.substring(index1 + 1, index2);
}
return "";
}
public String getUri() {
return uri;
}
}
Response
package com.kunlinr.lifealien.servlet.server;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
public class Response {
private static final int BUFFER_SIZE = 1024;
private Request request;
private OutputStream output;
public Response(OutputStream output) {
this.output = output;
}
public void setRequest(Request request) {
this.request = request;
}
/**
* Send static resources
* @throws IOException
*/
public void sendStaticResource() throws IOException {
byte[] bytes = new byte[BUFFER_SIZE];
FileInputStream fis = null;
try {
//Write the web file to the OutputStream byte stream
File file = new File(HttpServer.WEB_ROOT, request.getUri());
if (file.exists()) {
fis = new FileInputStream(file);
List<byte[]> contentbytes = new ArrayList<>();
int ch = fis.read(bytes, 0, BUFFER_SIZE);
int size = 0;
while (ch != -1) {
contentbytes.add(bytes);
ch = fis.read(bytes, 0, BUFFER_SIZE);
size += BUFFER_SIZE;
}
String head = "HTTP/1.1 200 OK\r\n" + "Content-Type: text/html\r\n"
+ "Content-Length: "+size+"\r\n" + "\r\n";
byte[] headbytes = head.getBytes();
output.write(headbytes,0,headbytes.length);
for(byte[] contentbyte:contentbytes) {
output.write(contentbyte);
}
} else {
// file not found
String errorMessage = "HTTP/1.1 404 File Not Found\r\n" + "Content-Type: text/html\r\n"
+ "Content-Length: 23\r\n" + "\r\n" + "<h1>File Not Found</h1>";
output.write(errorMessage.getBytes());
}
} catch (Exception e) {
// thrown if cannot instantiate a File object
System.out.println(e.toString());
} finally {
if (fis != null)
fis.close();
}
}
}
Simple page
<html>
<head>
<title>Hello,this is your own Page!</title>
</head>
<body>
Everything is Simple!
</body>
</html>
Effect
- Startup service
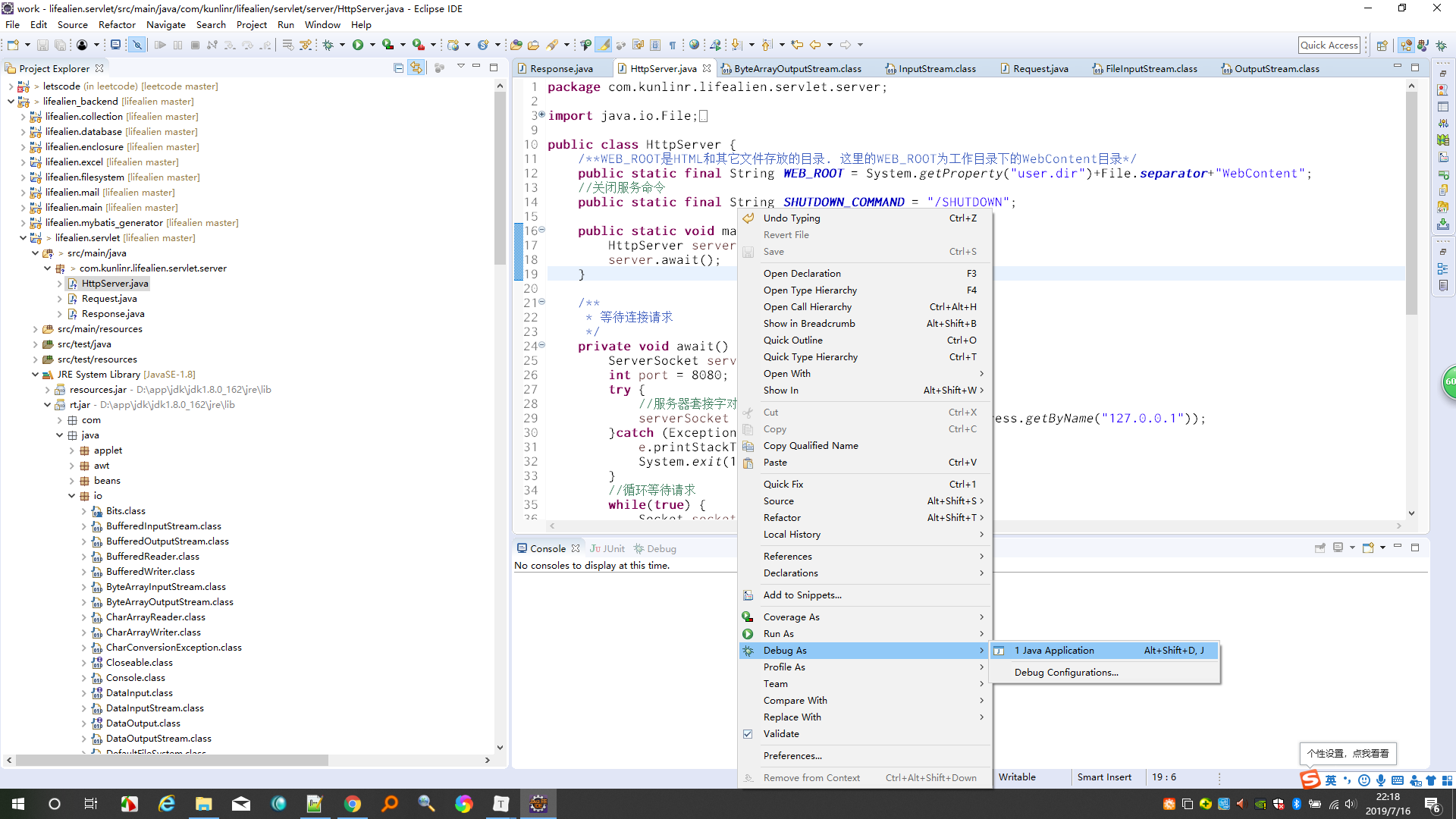
- Send request
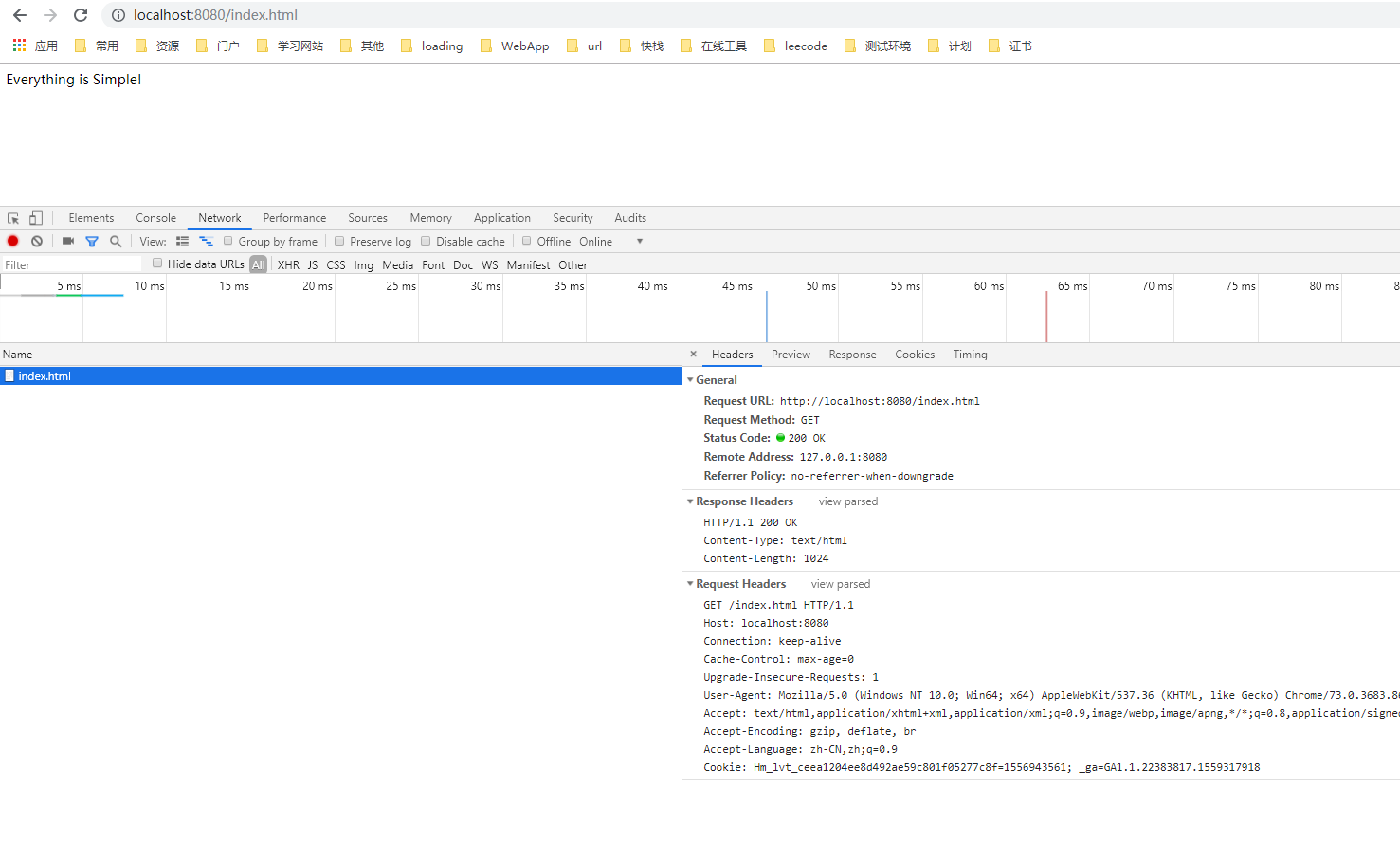
- Return to main text
<html>
<head>
<title>Hello,this is your own Page!</title>
</head>
<body>
Everything is Simple!
</body>
</html>
Reference document