Basic type packing class
There are eight basic data types in Java, but these data are basic data. It becomes difficult to perform complex operations on them. What shall I do?
In the actual program use, the data entered by the user on the program interface is stored in string type. In the process of program development, we need to convert the string data into the specified basic data type according to the needs, such as the age needs to be converted into int type, the test score needs to be converted into double type, etc. So, what do you want to do to realize the conversion between string and basic data?
Java provides corresponding objects to solve this problem. Basic data type object wrapper class: Java encapsulates basic data type values into objects. What are the benefits of encapsulating into objects? It can provide more functions to operate basic values. The packaging classes corresponding to the eight basic types are as follows:
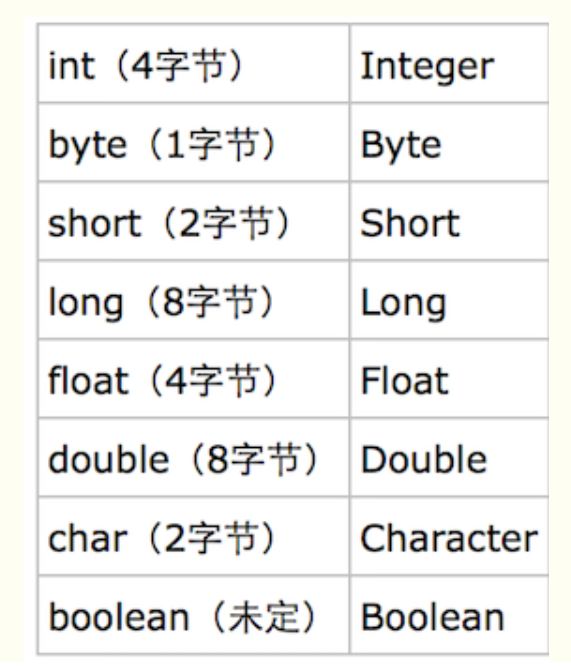
Note that int corresponds to Integer, char corresponds to Character, and the other six are basic types, which can be capitalized with the first letter.
Basic data type object wrapper class features: used to convert between basic data and string.
Boxing is to automatically convert the basic data type to the wrapper type; Unpacking is to automatically convert the wrapper type to the basic data type.
Convert string to base type
parseXXX(String s); Where XXX represents the basic type, and the parameter is a string that can be converted to the basic type. If the string cannot be converted to the basic type, the number conversion problem NumberFormatException will occur
System.out.println(Integer.parseInt("123") + 2); //The print result is 125
Convert basic values to strings
There are three ways:
-
The basic type can be directly connected with ""; 34+""
-
Call the valueof method of string; String.valueOf(34) ;
-
Call the toString method in the wrapper class; Integer.toString(34) ;
Basic type and object conversion
Use int type and Integer object conversion for demonstration, and other basic types are converted in the same way.
Basic value - > packing object
Integer i = new Integer(4);//Using constructor functions Integer ii = new Integer("4");//A numeric string can be passed in the constructor Integer iii = Integer.valueOf(4);//Use the valueOf method in the wrapper class Integer iiii = Integer.valueOf("4");//Use the valueOf method in the wrapper class
Packing object ----- > basic value
int num = i.intValue();
Usually we use it directly
public class Main { public static void main(String[] args) { //Automatic packing Integer total = 99; //Custom unpacking int totalprim = total; } }
Automatic packing and unpacking
If necessary, the basic type and packaging type can be common. Sometimes when we have to use a reference data type, we can pass in the basic data type.
For example:
Basic types can be evaluated directly using operators, but reference types cannot. The basic type wrapper class, as a reference type, can be calculated because Java "secretly" automatically converts objects to basic data types.
Correspondingly, the value of the reference data type variable must be the memory space address value from new, and we can assign the value of a basic type to the reference of a basic type wrapper class. The same reason is that Java automatically converts basic data types to objects "secretly".
Automatic unpacking: convert objects to basic values
Auto boxing: convert basic values to objects
Integer i = 4;//Automatic packing. Equivalent to Integer i = Integer.valueOf(4); i = i + 5;//Right of the equal sign: convert the I object to the basic value (automatic unpacking) i.intValue() + 5; After the addition operation is completed, box again and convert the basic value into an object.
be careful
When the value is within the byte range, it is automatically boxed. Instead of creating a new object space, the existing space is used.
Integer a = new Integer(3); Integer b = new Integer(3); System.out.println(a==b);//false System.out.println(a.equals(b));//true System.out.println("---------------------"); Integer x = 127; Integer y = 127; //In jdk1.5 auto boxing, if the value is within the byte range, the existing object space is used instead of creating a new object space. System.out.println(x==y); //true System.out.println(x.equals(y)); //true
Let's analyze its source code:
First, let's look at the Integer.valueOf function
public static Integer valueOf(int i) { if (i >= IntegerCache.low && i <= IntegerCache.high) return IntegerCache.cache[i + (-IntegerCache.low)]; return new Integer(i); } //IntegerCache.low=128
It will first judge the size of i: if i is less than - 128 or greater than or equal to 128, an Integer object will be created. Otherwise, it will not be created in the returned cache.
Integer i1 = 100; Integer i2 = 100; Integer i3 = 200; Integer i4 = 200; System.out.println(i1==i2); //true System.out.println(i3==i4); //false
At the back of the code, we can see that their execution results are different. Why? Look at our instructions above.
1. i1 and i2 will be automatically boxed and the valueOf function is executed. Their values are in the range (- 128128). They will get the same object in the Cache array. They refer to the same Integer object, so they must be equal.
2. i3 and i4 will also be automatically boxed and execute the valueOf function. Their value is greater than 128, so they will execute new Integer(200), that is, they will create two different objects respectively, so they must be different.
Integer integer100=null; int int100=integer100;
These two lines of code are completely legal and can be compiled, but null pointer exceptions will be thrown at run time. Of course, integer100 is an object of Integer type, which can point to null. However, in the second line, integer100 will be unpacked, that is, intValue() will be executed on a null object Method, of course, will throw a null pointer exception. Therefore, when unpacking, you must pay special attention to whether the encapsulated class object is null.
Summary:
1. You need to know when it will cause packing and unpacking
2. Boxing operations will create objects. Frequent boxing operations will consume a lot of memory and affect performance. Therefore, boxing can be avoided as much as possible.
3. equals(Object o) because the parameter type in the original equals method is the encapsulation type and the passed in parameter type (a) is the original data type, it will be boxed automatically. On the contrary, it will be unpacked
4. When two different types are compared, the wrapper needs to be unpacked. When the same type is compared, it will be unpacked or boxed automatically