The essence of the simple factory pattern is that a factory class dynamically determines which instances of a product class should be created (these product classes inherit from a parent class or interface) according to the parameters passed in. ——Baidu Encyclopedia
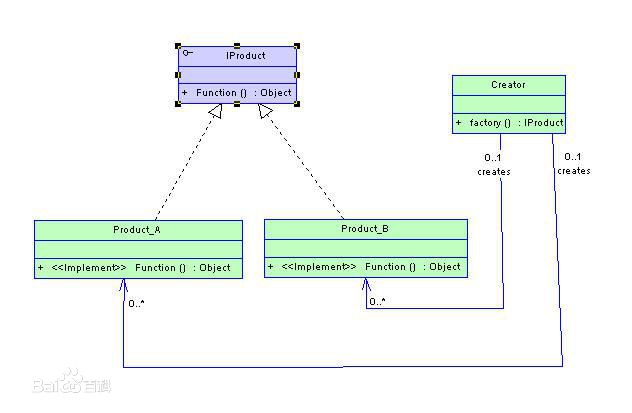
For example:
For example, there is an automobile factory that can produce Benz and BMW car s. With simple factories:
- First, there should be a factory:
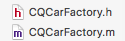
#import <Foundation/Foundation.h> #import "CQCar.h" typedef NS_ENUM(NSUInteger, CQCarType) { /** Benz */ CQCarTypeBenz, /** BMW */ CQCarTypeBMW }; @interface CQCarFactory : NSObject /** Production of car of corresponding type according to type @param type car type @return Specific car examples */ + (CQCar *)produceCarWithType:(CQCarType)type; @end
- The factory produces two kinds of cars, Benz and BMW, based on the incoming type (both inherited from car):
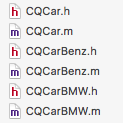
@implementation CQCarFactory /** Production of car of corresponding type according to type @param type car type @return Specific car examples */ + (CQCar *)produceCarWithType:(CQCarType)type { switch (type) { case CQCarTypeBenz: // Benz { CQCarBenz *benz = [[CQCarBenz alloc] init]; return benz; } break; case CQCarTypeBMW: // BMW { CQCarBMW *bmw = [[CQCarBMW alloc] init]; return bmw; } break; default: // General (e.g. Alto) { CQCar *car = [[CQCar alloc] init]; return car; } break; } } @end
- Use of the plant:
// Type of car to be produced NSArray *typeArray = @[@(CQCarTypeBenz), @(CQCarTypeBMW), @(CQCarTypeBenz)]; for (int i = 0; i < typeArray.count; i++) { CQCarType carType = [typeArray[i] integerValue]; // Pass in type and create car CQCar *car = [CQCarFactory produceCarWithType:carType]; // Print car information [car printInfo]; }
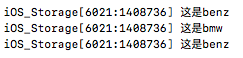
Summarize the simple factory:
Encapsulates a factory class (CarFactory), which can create corresponding instances (Benz and BMW) according to the passed in parameters (CarType), and the parent classes of these instances are the same (all belong to car).
Simple factories are so simple.
Note: simple factory is not one of the 23 GOF design patterns.
demo
https://github.com/CaiWanFeng/iOS_Storage
This demo location:
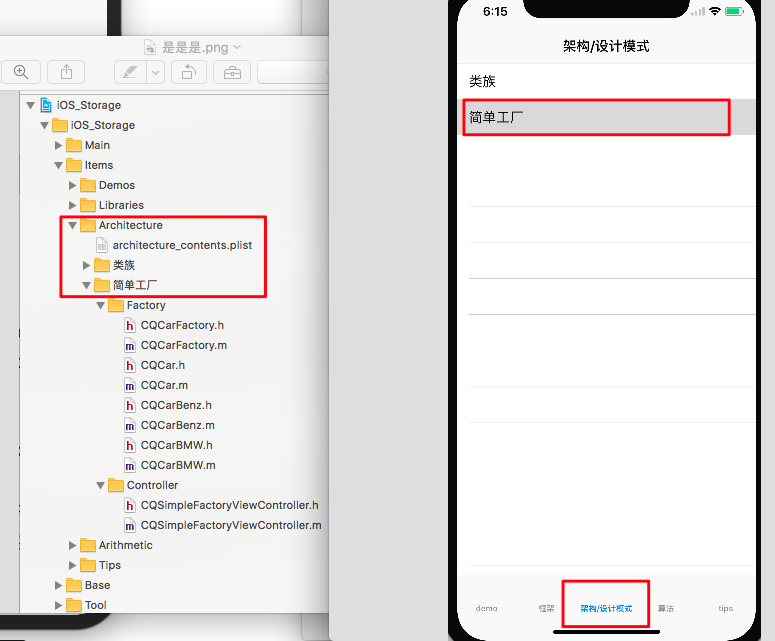