There are four ways to store data in iOS:
plist storage, preferences, NSCoding archives, databases
The first three are stored in the sandbox of the iPhone and the second in the database.
Before learning about iOS storage methods, first learn about iOS storage mechanism - sandbox
Application sandbox mechanism: Each iOS application has its own application sandbox (file system directory) isolated from other file systems.Each application must run in its own sandbox, which is not accessible by other applications.
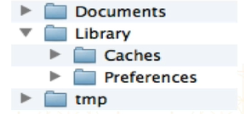
- Sandbox structure analysis:
- Application package: Contains all resource files and executables.
- Documents: Save data made by the application when it runs that needs to be persisted, which is backed up when iTunes synchronizes the device.(For example, game apps can save games in this directory.Large data cannot be stored here, once stored, iOS audits will not pass)
- tmp: Save the temporary data needed for the application to run and delete the files from the directory after use.If the application is not running, the system may also clean up the files in that directory.The directory is not backed up when iTunes synchronizes devices.
- Library/Caches: Saves data generated by the application runtime that needs to be persisted and does not back up when iTunes synchronizes the device.Generally, non-essential data that is large and does not require backup is stored.(Files downloaded from the cache are usually placed here)
- Library/Preference: Save all preferences for the application, where the iOS settings application looks for the application's settings.The iTunes synchronization device backs up the directory.
- How to print sandbox paths:
NSLog(@"%@",NSHomeDirectory);
1. XML attribute list (plist) archive:
The data store is stored on the phone
Plist file storage is generally about accessing dictionaries and arrays, writing them directly into plist files, and storing them in application sandboxes.
Only in IOS is plist storage, which is unique to ios.
// Store data - (IBAction)saveBtnClick:(id)sender { //Model: NSArray *dataArr = @[@"BQ",@10]; NSDictionary *dataDict = @{ @"name" : @"BQ",@"age" : @20}; /* Parameters: 1.Parameters of the search 2.Scope of search 3.Whether to expand the path (iOS does not recognize ~) This parameter must be YES for iOS applications */ NSString *path = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory,NSUserDomainMask, YES)[0]; NSLog(@"%@",path); // Stitching a file name: automatically add a slash, stitching files exclusively NSString *filePath = [path stringByAppendingPathComponent:@"data.plist"]; NSString *dictPath = [path stringByAppendingPathComponent:@"dict.plist"]; // Path is the path to the sandbox [dataArr writeToFile:filePath atomically:YES]; [dataDict writeToFile:dictPath atomically:YES]; } // Read Data - (IBAction)readBtnClick:(id)sender { NSString *path = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory,NSUserDomainMask, YES)[0]; // array NSString *filePath = [path stringByAppendingPathComponent:@"data.plist"]; NSArray *dataArr = [NSArray arrayWithContentsOfFile:filePath]; NSLog(@"%@",dataArr); // Dictionaries NSString *dictPath = [path stringByAppendingPathComponent:@"dict.plist"]; NSDictionary *dict = [NSDictionary dictionaryWithContentsOfFile:dictPath]; NSLog(@"%@",dict); }
2. Preference:
Preference Settings NSUserDefaults:
The bottom level is to close a dictionary and use it to generate plist files
Benefit: You don't need to care about file names (which are generated automatically) for fast key-value pair storage.
Usually used to store information such as account passwords.
Note: What key is used for saving and what key value is used for reading
What type to use when saving and what type to use when taking.
// Store data - (IBAction)save:(id)sender { // NSUserDefaults It also saves a plist file NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults]; [defaults setObject:@"BQ" forKey:@"name"]; [defaults setInteger:20 forKey:@"age"]; // Synchronous call, immediately written to file, not writing this method will be asynchronous, with delay [defaults synchronize]; NSLog(@"%@",NSHomeDirectory()); } // Read Data - (IBAction)read:(id)sender { NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults]; NSString *name = [defaults objectForKey:@"name"]; NSInteger *age = [defaults integerForKey:@"age"]; NSLog(@"%@-------%ld",name,age); }
3. NSKeyedArchiver Archives (NSCoding): Save Custom Objects
Archiving is generally used when saving custom objects, because plist files cannot save custom objects.
If a dictionary contains a custom object, if the dictionary is written to a file, it will not generate a plist file.
// Store data: - (IBAction)save:(id)sender { Person *per = [[Person alloc] init]; per.name = @"LSQ"; per.age = 10; Cat *cat = [[Cat alloc] init]; cat.name = @"wangCai"; per.cat = cat; // Get Sandbox Directory NSString *tempPath = NSTemporaryDirectory(); NSString *filePath = [tempPath stringByAppendingPathComponent:@"Person.data"]; NSLog(@"%@",tempPath); // Archive: Note: archiveRootObject calls encodeWithCoder: method has to write itself [NSKeyedArchiver archiveRootObject:per toFile:filePath]; } // Read data: - (IBAction)read:(id)sender { // Get Sandbox Directory NSString *tempPath = NSTemporaryDirectory(); NSString *filePath = [tempPath stringByAppendingPathComponent:@"Person.data"]; // unarchiveObjectWithFile calls initWithCoder Person *per = [NSKeyedUnarchiver unarchiveObjectWithFile:filePath]; NSLog(@"%@-----%@",per.name,per.cat.name); }
4. SQLite3 & Core Data:
SQL statement + server database
//... not yet completed