iOS reflection mechanism: the use of objc property
Objc ﹣ property ﹣ t is often used in actual development, for example, it is necessary to obtain the property of a registered class, or judge the type of property, or automatically assign a value to the property after obtaining the property, etc
Here is an example of obtaining all the properties of a registered class. The code is as follows:
//Get all property names of a class
- (NSArray*)getPropertieNamesByObject:(id)object
{
unsigned int outCount, i;
// Obtain the property list of the registered class. The first parameter is the class, and the second parameter is the variable that receives the number of class properties
objc_property_t *properties = class_copyPropertyList([object class], &outCount);
//Define an array to receive the acquired property name
NSMutableArray *nameArray = [[NSMutableArray alloc] initWithCapacity:outCount];
for (i = 0; i < outCount; i++) {
//Loop to get a single attribute
objc_property_t property = properties[i];
//Get attribute name
NSString *propertyName = [[NSString alloc] initWithCString:property_getName(property) encoding:NSUTF8StringEncoding];
//Put the resulting property name into the array
[nameArray addObject:propertyName];
}
free(properties);
return nameArray;
}
If you want to determine the type of the property, you only need to get the value of the property of the object through valueForKey:, and then determine the type of the value.
And if you want to get the member variables of a class, how to do it?
The method is as follows:
//Get the member variable name of the class
- (NSArray *)getVariableNamesByObject:(id)object
{
unsigned int numIvars = 0;
//Get all member variables of a class
Ivar * ivars = class_copyIvarList([object class], &numIvars);
//Define an array to receive the acquired property name
NSMutableArray *nameArray = [[NSMutableArray alloc] initWithCapacity:numIvars];
for(int i = 0; i < numIvars; i++) {
//Get a single member variable
Ivar thisIvar = ivars[i];
//Get the type of this member variable
const char *type = ivar_getTypeEncoding(thisIvar);
NSString *stringType = [NSString stringWithCString:type encoding:NSUTF8StringEncoding];
//Skip when judging non object-c type here
if (![stringType hasPrefix:@"@"]) {
continue;
}
//Get member variable name
NSString *variableName = [NSString stringWithUTF8String:ivar_getName(thisIvar)];
[nameArray addObject:variableName];
//This function can get the value of the member variable
//object_getIvar(object, thisIvar)
}
free(ivars);
return nameArray;
}
At this point, we create a Person class as shown in the figure
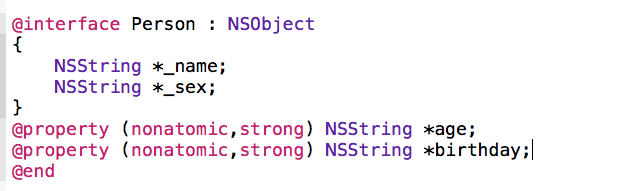
The results of calling these two methods are as follows
