preface
In the process of iOS development, de reprocessing is sometimes used, that is, removing duplicate elements or numbers, and then sorting. This uses the set (NSSet). In fact, it is a hash table that uses the hash algorithm to find the elements in the set.
The similarities between sets and arrays are the addresses where different elements are stored. The differences are that the elements in NSSet are automatically filtered and will not be repeated, but the elements in NSArray are allowed to be repeated; NSSet is an unordered set, and NSArray is an ordered set. Relatively speaking, the processing efficiency of NSSet is faster than that of NSArray.
This section will briefly introduce the use of NSSet and give an actual use case to show it.
1, Basic common methods
(1) NSSet
1. Collection initialization:
NSSet During initialization and assignment, if two identical elements are put in, one of them will be deleted automatically. eg: NSSet *set1 = [[NSSet alloc] initWithObjects:@"a",@"b",@"c",@"d", nil]; //Initialize collection [set1 count]; //Returns the number of in the collection
2. Element handling of NSSet
NSMutableSet *mSet1 = [[NSMutableSet alloc] initWithObjects:@"1",@"2",@"3", nil]; NSMutableSet *mSet2 = [[NSMutableSet alloc] initWithObjects:@"1",@"4",@"5", nil]; [mSet1 unionSet:mSet2]; //Take out union 1, 2, 3, 4, 5 [mSet1 intersectSet:mSet2]; //Take intersection 1 [mSet1 minusSet:mSet2]; //Delete the same elements as mSet2 in mSet1. The results are: 2, 3, 4, 5
3. Determine whether the collection contains an element
BOOL ret1 = [set1 containsObject:@"Apple"]; //Determine whether the collection contains @ "Apple"
4. Judge whether two sets are equal
NSSet *set2 = [[NSSet alloc] initWithObjects:@"a",@"b",@"c",@"d", nil]; BOOL ret1 = [set isEqualToSet:set2]; //Judge whether two sets are equal 5,judge set2 Is it set3 Subset of NSSet *set3 = [[NSSet alloc] initWithObjects:@"a",@"b",@"c",@"d",@"e", nil]; BOOL ret2 = [set2 isSubsetOfSet:set3]; //Determine whether set2 is a subset of set3
6. The collection is traversed by an enumerator
NSEnumerator *enumerator = [set objectEnumerator]; NSString *str; while (str = [enumerator nextObject]) { ...... }
7. Initializes a collection based on an array, that is, an array is converted to a collection
NSArray *array1 = [[NSArray alloc] initWithObjects:@"1",@"2",@"3",@"4", nil]; NSSet *set = [[NSSet alloc] initWithArray:array1];
8. Convert collection to array
NSArray *array2 = [set allObjects];
(2) NSMutableSet
1. Variable set initialization
NSMutableSet *mSet1 = [NSMutableSet setWithObjects:@"1", @"2", @"3", @"4", nil]; //Variable set initialization NSMutableSet *mSet2 = [NSMutableSet setWithCapacity:0]; //Variable set
2. Add element
[mSet1 addObject:@"5"]; [mSet1 addObject:@"6"]; [mSet1 addObject:@"6"]; //If there is a duplicate element added, only one identical element will be retained
3. Delete element
[mSet1 removeObject:@"3"]; [mSet1 removeAllObjects];
4. NSIndexSet: index set (index set)
NSIndexSetNSIndexSet *indexSet1 = [[NSIndexSet alloc] initWithIndexesInRange:NSMakeRange(1,3)]; //The number in the set is 1 2 3 //Extracts the element at the specified position in the array according to the collection NSArray *array1 = [[NSArray alloc] initWithObjects:@"1",@"2",@"3",@"4", nil]; NSArray *array2 = [array1 objectsAtIndexes:indexSet]; //Return 2 3 4
5. NSMutableIndexSet: variable index set
NSMutableIndexSet *indexSet2 =[[NSMutableIndexSet alloc] init]; [indexSet2 addIndex:0]; [indexSet2 addIndex:2]; [indexSet2 addIndex:4]; //Gets the specified element in the array through the collection NSArray *array3 = [[NSArray alloc] initWithObjects:@"1",@"2",@"3",@"4",@"5",@"6", nil]; NSArray *array4 = [array3 objectsAtIndexes:indexSet]; //Return 1 3 5
2, Case display
The case here is to perform a de duplication operation on the duplicate data before local data storage, and then store these data locally. A tool class is encapsulated for systematic processing. The specific code and place of use are as follows:
1. Tool class. h file:
#import <Foundation/Foundation.h> @interface JSHistoryTool : NSObject (instancetype)sharedHistoryTool; // Store * * operation history (void)writeTemp:(NSString *)temp time:(NSString *)time; // Take out * * operation history (NSArray *)getValue; @end
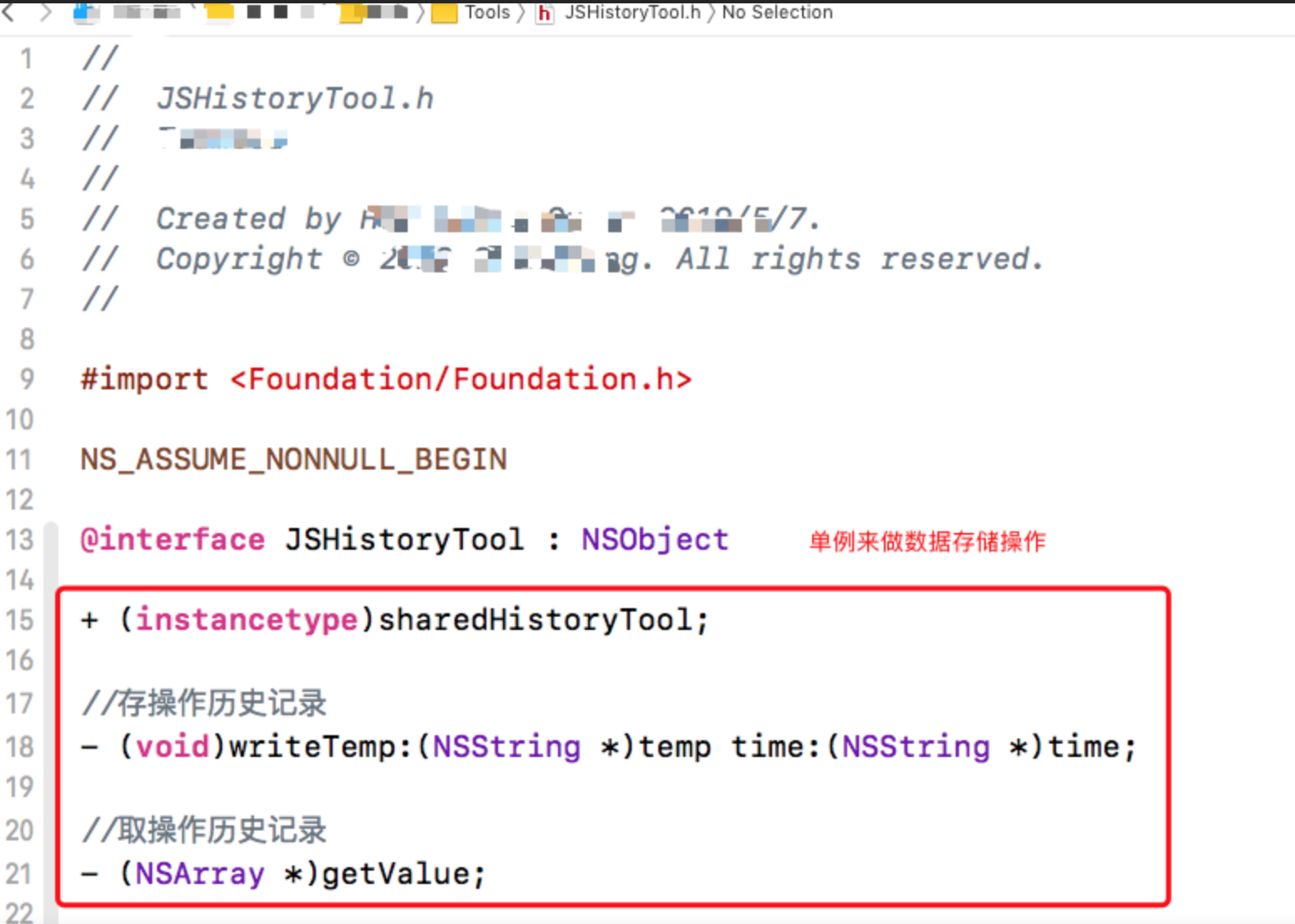
2. Tool class. m file:
#import "JSHistoryTool.h" @implementation JSHistoryTool (instancetype)sharedHistoryTool { static id instance = nil; static dispatch_once_t onceToken; dispatch_once(&onceToken, ^{ instance = [[self alloc]init]; }); return instance; } (void)writeTemp:(NSString *)temp time:(NSString *)time { NSArray *arr = [[NSUserDefaults standardUserDefaults] valueForKey:@"set"]; NSMutableSet *sSet = [[NSMutableSet alloc] initWithArray:arr]; //First take out the existing stored array, then de duplicate it according to the array elements, and finally store it [sSet addObject:@{@"Temp":temp, @"Time":time}]; NSArray *aa = [sSet allObjects]; NSMutableArray *marr = [[NSMutableArray alloc] initWithArray:aa]; if (marr.count > 20) { [marr removeObjectAtIndex:0]; } [[NSUserDefaults standardUserDefaults] setObject:marr forKey:@"set"]; } (NSArray *)getValue { NSArray *arr = [[NSUserDefaults standardUserDefaults] valueForKey:@"set"]; return [[arr reverseObjectEnumerator] allObjects]; } @end

3. Code of specific calling place in the controller:
(void)sendClick { if (![JSBLECenterManager sharedInstance].characteristic) { return; } _tempValue = [NSString stringWithFormat:@"%.0f", _centigradeDegree]; _timeValue = [NSString stringWithFormat:@"%.0ld", (long)_timeDegree]; _waterValue = [NSString stringWithFormat:@"%.0f", _waterDegree]; NSString *ttData = [NSString stringWithFormat:@"TEA%@,%@,%@U", _tempValue, _timeValue, _waterValue]; NSMutableSet *set1 = [[NSMutableSet alloc] initWithObjects:_tempValue, _timeValue, nil]; //Initialize variable set [[JSHistoryTool sharedHistoryTool] writeTemp:_tempValue time:_timeValue]; //Store through tool classes NSData *data = [ttData dataUsingEncoding:NSUTF8StringEncoding]; // Write data according to the above feature self.characteristic [[JSBLECenterManager sharedInstance].peripheral writeValue:data forCharacteristic:[JSBLECenterManager sharedInstance].characteristic type:CBCharacteristicWriteWithResponse]; }
