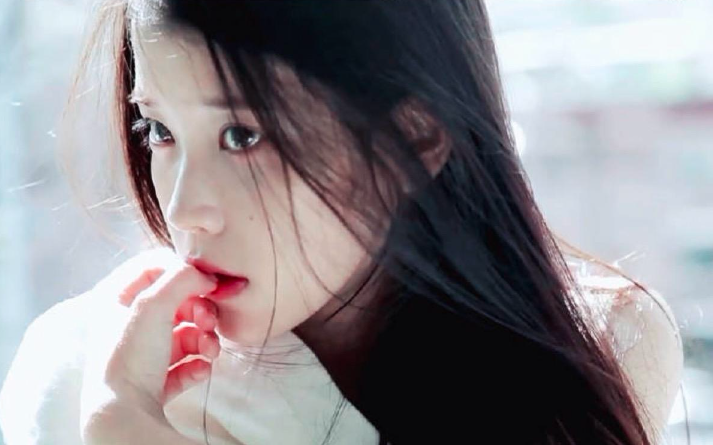
preface
UIScrollView has contentInset, but UILabel doesn't; Android does, iOS doesn't.
Recently, I need to use a label with inner margin, so I encapsulated it with the code of my predecessors on the Internet. Originally, I wanted to create a category, but it failed.
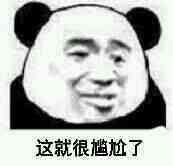
The code is as follows, inherited from UILabel:
. h file:
#import <UIKit/UIKit.h> @interface EdgeInsetsLabel : UILabel @property (nonatomic) UIEdgeInsets contentInset; @end
. m file:
#import "EdgeInsetsLabel.h" @implementation EdgeInsetsLabel - (void)setContentInset:(UIEdgeInsets)contentInset { _contentInset = contentInset; NSString *tempString = self.text; self.text = @""; self.text = tempString; } -(void)drawTextInRect:(CGRect)rect { [super drawTextInRect:UIEdgeInsetsInsetRect(rect, self.contentInset)]; } @end
use
self.label.contentInset = UIEdgeInsetsMake(0, 20, 0, 20);
Code description
The inset of label is changed in this method
-(void)drawTextInRect:(CGRect)rect { [super drawTextInRect:UIEdgeInsetsInsetRect(rect, self.contentInset)]; }
This is the method provided by the system:
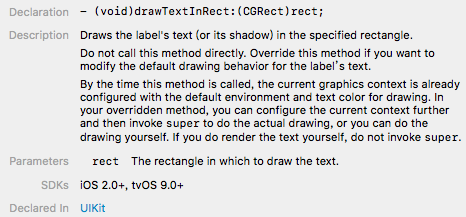
This method can not be called actively, but we need to do processing in this method. What should we do?
I've experimented with this method when I change the text of the label.
In other words, we can call this method indirectly by changing the text of the label.
So we have this wonderful code of assigning contentInset:
- (void)setContentInset:(UIEdgeInsets)contentInset { _contentInset = contentInset; NSString *tempString = self.text; self.text = @""; self.text = tempString; }
First clear the label text, and then re assign text to change the text of the label. Finally, call back the drawTextInRect: method.
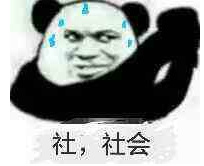
In this way, we can immediately see the effect when we assign contentInset to the label.
Effect GIF:
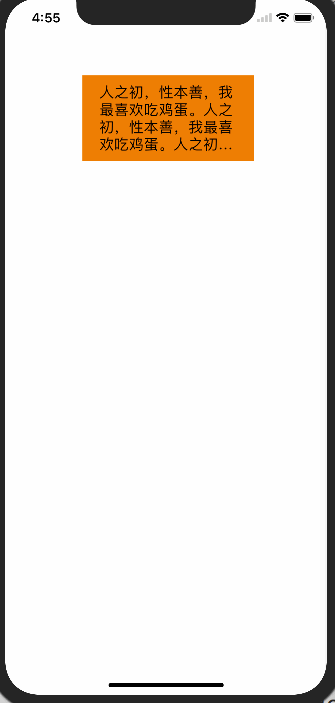
Corresponding code:
- (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view, typically from a nib. self.label = [[EdgeInsetsLabel alloc] initWithFrame:CGRectMake(90, 90, 200, 100)]; [self.view addSubview:self.label]; self.label.text = @"At the beginning of human life, I like to eat eggs best. At the beginning of human life, I like to eat eggs best. At the beginning of human life, I like to eat eggs best. At the beginning of human life, I like to eat eggs best."; self.label.numberOfLines = 0; self.label.backgroundColor = [UIColor orangeColor]; self.label.contentInset = UIEdgeInsetsMake(0, 20, 0, 20); // Change contentInset after 3 seconds dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(3 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{ self.label.contentInset = UIEdgeInsetsMake(20, 50, 10, 20); }); } - (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { self.label.contentInset = UIEdgeInsetsMake(0, 80, 0, 20); self.label.text = @"Because of the lack of thinking Hall"; }
demo
last
If you have a better way, don't grudge sharing.