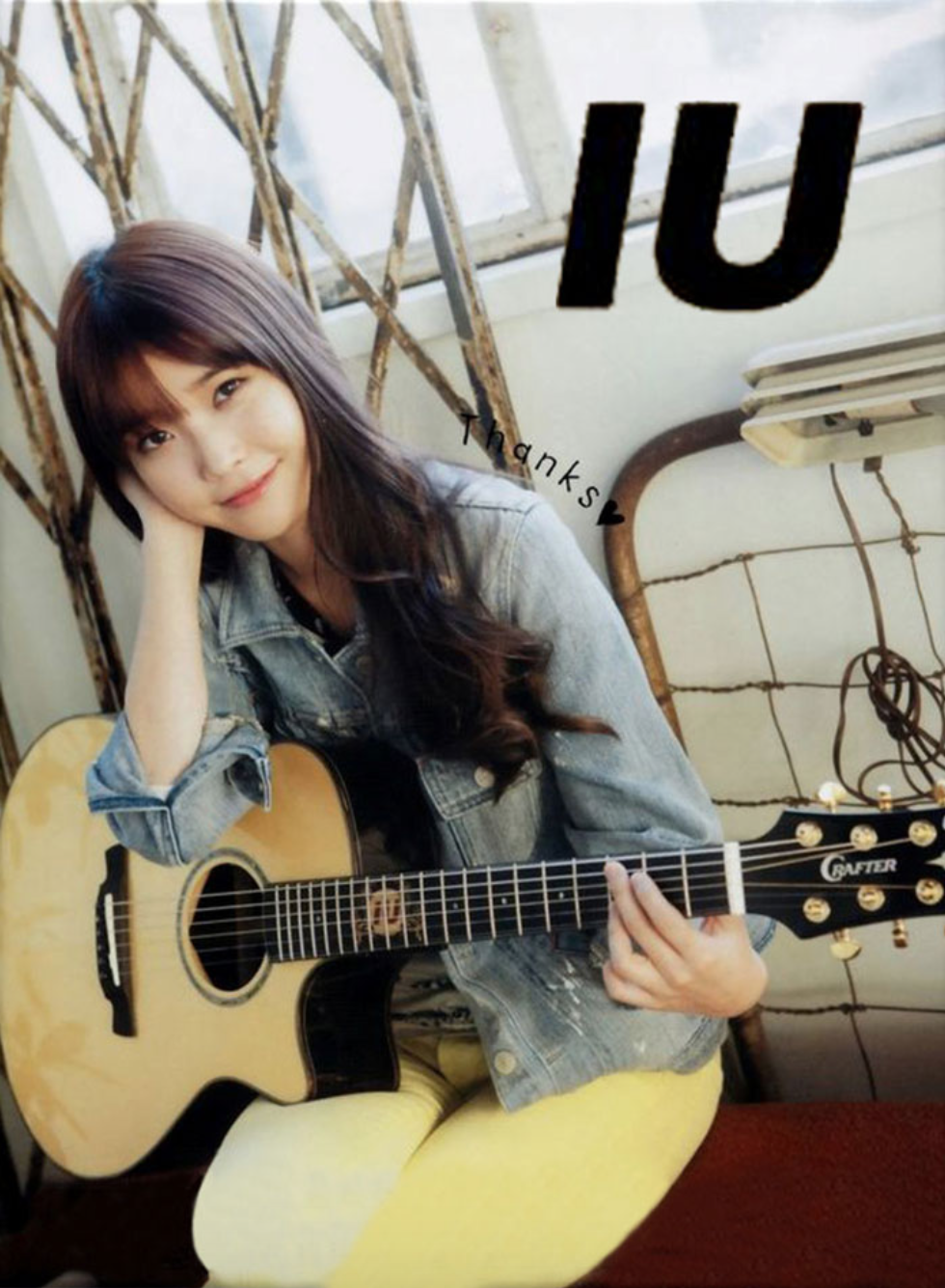
scene
Recently, the app has been revised. Here are some of the UI screenshots:
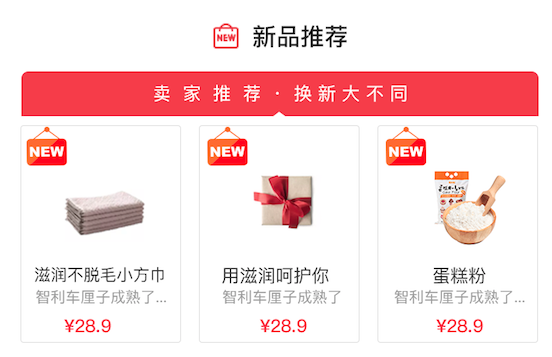
At the top, I think of it as the group header of UICollectionView:
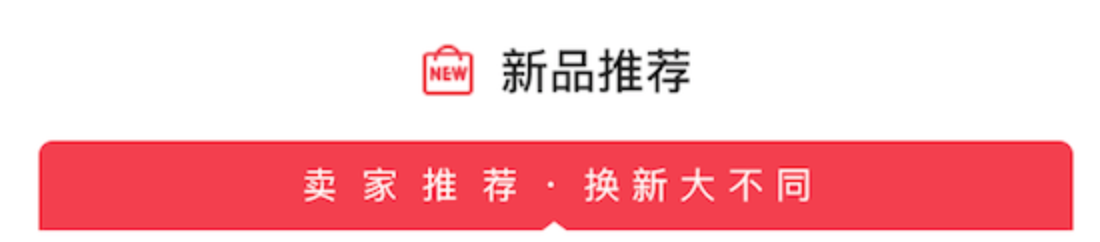
There is an irregular label:

The top two corners of the label are fillets, the bottom two corners are right angles, and there is a small triangle at the bottom.
thinking
CAShapeLayer and UIBezierPath draw an irregular layer as the mask of label.layer.
Concrete realization
1. Customize an iregularlabel inherited from UILabel
#import "IrregularLabel.h" @interface IrregularLabel () /** Mask */ @property (nonatomic, strong) CAShapeLayer *maskLayer; /** Route */ @property (nonatomic, strong) UIBezierPath *borderPath; @end
2. Initialize and set in the initialization method
- (instancetype)initWithFrame:(CGRect)frame { if (self = [super initWithFrame:frame]) { // Initialize mask self.maskLayer = [CAShapeLayer layer]; // Set Matte [self.layer setMask:self.maskLayer]; // Initialization path self.borderPath = [UIBezierPath bezierPath]; } return self; }
3. Set the path in the layoutSubviews method
- (void)layoutSubviews { [super layoutSubviews]; // Mask layer frame self.maskLayer.frame = self.bounds; // Set path start point [self.borderPath moveToPoint:CGPointMake(0, 10)]; // Fillet at top left [self.borderPath addQuadCurveToPoint:CGPointMake(10, 0) controlPoint:CGPointMake(0, 0)]; // Line, to top right [self.borderPath addLineToPoint:CGPointMake(self.bounds.size.width - 10, 0)]; // Fillet at top right [self.borderPath addQuadCurveToPoint:CGPointMake(self.bounds.size.width, 10) controlPoint:CGPointMake(self.bounds.size.width, 0)]; // Straight line, to bottom right [self.borderPath addLineToPoint:CGPointMake(self.bounds.size.width, self.bounds.size.height)]; // Small triangle at the bottom [self.borderPath addLineToPoint:CGPointMake(self.bounds.size.width/2+5, self.bounds.size.height)]; [self.borderPath addLineToPoint:CGPointMake(self.bounds.size.width/2, self.bounds.size.height-5)]; [self.borderPath addLineToPoint:CGPointMake(self.bounds.size.width/2 - 5, self.bounds.size.height)]; // Straight line, to lower left corner [self.borderPath addLineToPoint:CGPointMake(0, self.bounds.size.height)]; // Straight line, back to start [self.borderPath addLineToPoint:CGPointMake(0, 10)]; // Assign this path to the path of the maskLayer self.maskLayer.path = self.borderPath.CGPath; }
The coordinates of the upper left corner are (0,0)
The coordinates of the lower right corner are (maxX,maxY)
The fillet is a quadratic Bezier curve. As for the quadratic Bezier curve, I see an image on the Internet:
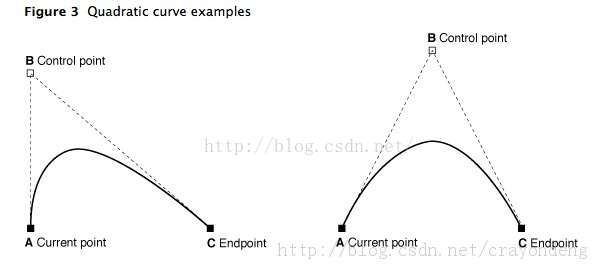
Final effect
- (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view, typically from a nib. self.label = [[IrregularLabel alloc] initWithFrame:CGRectMake(90, 200, 200, 40)]; [self.view addSubview:self.label]; self.label.text = @"It's an irregularity label"; self.label.textAlignment = NSTextAlignmentCenter; self.label.backgroundColor = [UIColor redColor]; self.label.textColor = [UIColor whiteColor]; self.label.font = [UIFont boldSystemFontOfSize:16]; }
Design sketch:
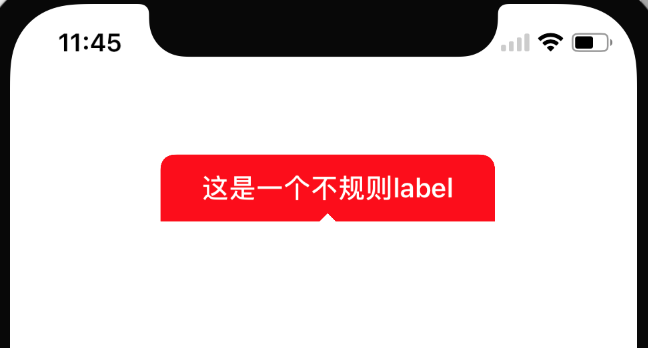
demo
https://github.com/CaiWanFeng/IrregularLabel
summary
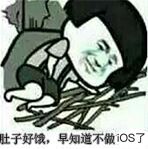