Link: https://www.jianshu.com/p/b9bba621b295
Look at the example first
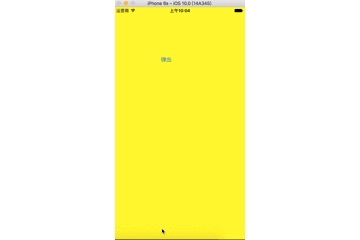
Final result. gif
First set up the basic interface
- (void)viewDidLoad {
[super viewDidLoad];
//Set Background Color
self.view.backgroundColor = [UIColor yellowColor];
//Popup Button
UIButton *alertBt = [UIButton buttonWithType:UIButtonTypeSystem];
alertBt.frame = CGRectMake(100, 100, 100, 100);
[alertBt setTitle:@"Eject" forState:UIControlStateNormal];
[alertBt addTarget:self action:@selector(alertAction) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:alertBt];
}
//Pop-up Button Click Event
- (void)alertAction{
//Here I've created an AlertViewController that inherits UIViewController, which is our most basic way to pop up. As you can imagine, I'll leave it alone.
[self presentViewController:[AlertViewController new] animated:YES completion:nil];
}
And then we're going to do something in AlertViewController
ps: Most of it is layout code, so look at the first few sentences to skip the next step
- (void)viewDidLoad {
[super viewDidLoad];
//For the pop-up effect, of course, we need to make the background transparent
self.view.backgroundColor = [UIColor clearColor];
//This is where the layout code can be read based on the interface effect, and it doesn't matter if you don't read it. I've defined two macros here, one bigWid and one bigHei, which is the width and height of the screen, respectively.
UIView *myView = [UIView new];
myView.frame = CGRectMake(15, bigHei-175, bigWid-30, 160);
myView.backgroundColor = [UIColor whiteColor];
myView.layer.cornerRadius = 5;
[self.view addSubview:myView];
//Share WeChat and Circle of Friends button
UIButton *bt1 = [UIButton buttonWithType:UIButtonTypeSystem];
[bt1 setBackgroundImage:[UIImage imageNamed:@"weChat"] forState:UIControlStateNormal];
[bt1 setTitle:@"Share with friends" forState:UIControlStateNormal];
bt1.titleLabel.font = [UIFont systemFontOfSize:10];
[bt1 setTitleEdgeInsets:UIEdgeInsetsMake(40, 0, -40, 0)];
bt1.tintColor = [UIColor grayColor];
bt1.frame = CGRectMake(40, 30, 50, 50);
[bt1 sizeToFit];
[myView addSubview:bt1];
UIButton *bt2 = [UIButton buttonWithType:UIButtonTypeSystem];
[bt2 setBackgroundImage:[UIImage imageNamed:@"share"] forState:UIControlStateNormal];
[bt2 setTitle:@"Circle of Friends Sharing" forState:UIControlStateNormal];
bt2.titleLabel.font = [UIFont systemFontOfSize:10];
[bt2 setTitleEdgeInsets:UIEdgeInsetsMake(40, 0, -40, 0)];
bt2.tintColor = [UIColor grayColor];
bt2.frame = CGRectMake(bigWid/2-35, 30, 50, 50);
[bt2 sizeToFit];
[myView addSubview:bt2];
UIButton *bt3 = [UIButton buttonWithType:UIButtonTypeSystem];
[bt3 setBackgroundImage:[UIImage imageNamed:@"box"] forState:UIControlStateNormal];
[bt3 setTitle:@"Collection" forState:UIControlStateNormal];
bt3.titleLabel.font = [UIFont systemFontOfSize:10];
[bt3 setTitleEdgeInsets:UIEdgeInsetsMake(40, 0, -40, 0)];
bt3.tintColor = [UIColor grayColor];
bt3.frame = CGRectMake(bigWid - 110, 30, 50, 50);
[myView addSubview:bt3];
//Split Line
UIView *deView = [[UIView alloc]initWithFrame:CGRectMake(0, 120, bigWid - 30, 1)];
deView.backgroundColor = [UIColor colorWithRed:225/255.0 green:225/255.0 blue:225/255.0 alpha:1.0];
[myView addSubview:deView];
//Cancel button
UIButton *cancellBt = [UIButton buttonWithType:UIButtonTypeSystem];
[cancellBt setTitle:@"cancel" forState:UIControlStateNormal];
cancellBt.frame = CGRectMake(15, 120, bigWid - 60, 40);
[myView addSubview:cancellBt];
[cancellBt addTarget:self action:@selector(backAction) forControlEvents:UIControlEventTouchUpInside];
}
//Return button
- (void)backAction{
[self dismissViewControllerAnimated:YES completion:nil];
}
Then what happens, of course
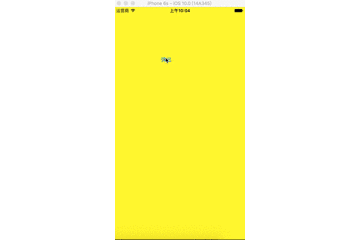
gif.gif
Because the default press effect is not override, it is toggle. The black background displayed is the background color of Window.
So next we'll modify modalPresentStyle
Go back to ViewController first
//Setting the jump mode in the pop-up method
- (void)alertAction{
AlertViewController *vc = [AlertViewController new];
//The mode for setting the destination view controller here is not self
vc.modalPresentationStyle = UIModalPresentationOverCurrentContext;
[self presentViewController:vc animated:YES completion:nil];
}
//For realism, add a shadow
//This can be written in viewDidLoad
self.shadowView = [[UIView alloc] initWithFrame:self.view.bounds];
self.shadowView.backgroundColor = [UIColor blackColor];
[self.view addSubview:self.shadowView];
---------
//Before present, first
self.shadowView .alpha = 0.5;
//Then return with its alpha set back to 0, which cannot be used in viewWillApear because his view is always visible, so return does not trigger this method. Here you can use a block or proxy to implement shadowVIew.alpha = 0 on return;
Final Effect Chart
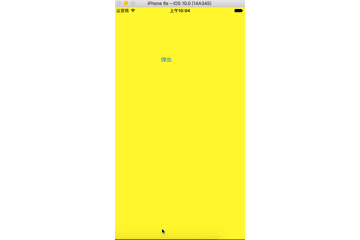
! [Uploading Final Effect _165272.gif..]