List: ion-list
Listing is a common way of organizing information. In ionic, list elements are declared using ion-list instructions, and member elements are declared using ion-item instructions:
-
<ion-list>
-
<ion-item>...</ion-item>
-
<ion-item>...</ion-item>
-
...
-
</ion-list>
The ion-list Directive provides the following attributes to customize the look of the list:
- Type - List type. The type attribute is optional and can be used to set the type of list: list-inset | card. Both lists have built-in effects, but the difference is that the card list has a border shadow effect.
- Show-delete - Whether to display the delete button within a member. The show-delete attribute is optional. If there is an ion-delete-button in a member, use this attribute to notify the list whether the element delete button is displayed. The allowable value is: true | false.
- Show-reorder - Displays the reorder button within a member. The show-reorder attribute is optional. If there is an ion-reorder-button within a member, use this attribute to notify the list whether the element reorder button is displayed. The allowable value is: true | false.
- can-swipe - Whether sliding mode is supported to display member option buttons. The can-switch attribute is optional. If there is an option button in a member, use this property to allow or prohibit opening the option button by sliding the member to the left. The allowable value is: true | false, which defaults to true.
-
<!DOCTYPE html>
-
<html ng-app="myApp">
-
<head>
-
<meta charset="UTF-8">
-
<meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no,width=device-width,height=device-height">
-
<script src="../lib/js/ionic.bundle.min.js"></script>
-
<link rel="stylesheet" type="text/css" href="../lib/css/ionic.min.css">
-
-
</head>
-
<body ng-controller="myCtrl">
-
<ion-header-bar class="bar-positive">
-
<h1 class="title">ion-list</h1>
-
</ion-header-bar>
-
<ion-content>
-
<ion-list can-swipe="true">
-
<ion-item ng-repeat="item in items">
-
{{item}}
-
<ion-option-button class="button-calm icon ion-edit"></ion-option-button>
-
<ion-option-button class="button-energized icon ion-share"></ion-option-button>
-
</ion-item>
-
</ion-list>
-
</ion-content>
-
</body>
-
</html>
-
-
<script>
-
angular.module("myApp",["ionic"])
-
.controller("myCtrl",function($scope){
-
$scope.items = [];
-
for(var i=0;i<10;i++)
-
$scope.items.push(["item list ",i+1].join(""));
-
});
-
-
</script>
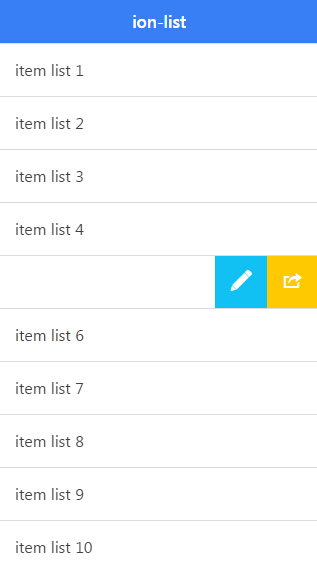
II. ion-list ion-item members
ion-item has three predefined buttons:
-
ion-option-button-option button. An ion-item can contain multiple option buttons. The option button is hidden and requires the user to slide members to the left to display the option button. You can use the can-swipe attribute of ion-list to allow or prohibit sliding open option buttons. Using the ng-click instruction to hook the click event listener function, the prototype is as follows:
-
var optionListener = function(item){...}
When ionic captures a user's click event, it calls this function and passes it into the current item object.
-
ion-delete-button-Delete button There is at most one Delete button in an ion-item. The Delete button is always on the left-most end of the member when it is displayed. The show-delete attribute of ion-list can be used to display or hide the delete button and the ng-click instruction can be used to hook the click event listener function. The prototype is as follows:
-
var deleteListener = function(item){...}
When ionic captures a user's click event, it calls this function and passes it into the current item object.
-
ion-reorder-button-rearrangement button There is at most one rearrangement button in an ion-item. The rearrangement button is always at the right end of the member when it is displayed. You can use the show-reorder attribute of ion-list to display or hide the rearrangement button and use the on-reorder attribute to hook the rearrangement event listener function. Its prototype is as follows:
-
var reorderListener = function(item,$fromIndex,$toIndex){...}
When ionic captures a user's rearrangement event, it calls this function and passes in the current item object, the original serial number and the target serial number.
-
<!DOCTYPE html>
-
<html ng-app="myApp">
-
<head>
-
<meta charset="UTF-8">
-
<meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no,width=device-width,height=device-height">
-
<script src="../lib/js/ionic.bundle.min.js"></script>
-
<link rel="stylesheet" type="text/css" href="../lib/css/ionic.min.css">
-
</head>
-
<body ng-controller="myCtrl">
-
<ion-header-bar class="bar-positive">
-
<a class="button button-clear icon ion-ios-minus-outline" ng-click="flag.showDelete=!flag.showDelete;flag.showReorder=false;"></a>
-
<h1 class="title">Member button</h1>
-
<a class="button" ng-click="flag.showReorder=!flag.showReorder;flag.showDelete=false;">Rescheduling</a>
-
</ion-header-bar>
-
<ion-content>
-
<ion-list show-delete="flag.showDelete" show-reorder="flag.showReorder">
-
<ion-item ng-repeat="item in items">
-
{{item}}!
-
<ion-option-button class="button-calm">...</ion-option-button>
-
-
<ion-delete-button class="ion-minus-circled" ng-click="delete_item(item)"></ion-delete-button>
-
<ion-reorder-button class="ion-navicon" on-reorder="move_item(item,$fromIndex,$toIndex)"></ion-reorder-button>
-
</ion-item>
-
</ion-list>
-
</ion-content>
-
</body>
-
</html>
-
-
<script>
-
angular.module("myApp",["ionic"])
-
.controller("myCtrl",function($scope){
-
$scope.flag={showDelete:false,showReorder:false};
-
$scope.items=["Chinese","English","German","Italian","Janapese","Sweden","Koeran","Russian","French"];
-
$scope.delete_item=function(item){
-
var idx = $scope.items.indexOf(item);
-
$scope.items.splice(idx,1);
-
};
-
$scope.move_item = function(item, fromIndex, toIndex) {
-
-
console.log(fromIndex);
-
console.log(toIndex);
-
-
console.log(item);
-
-
$scope.items.splice(fromIndex, 1);
-
$scope.items.splice(toIndex, 0, item);
-
console.log(item);
-
};
-
});
collection-repeat: High Performance ng-repeat
collection-repeat instructions are similar to ng-repeat instructions, but they are more suitable for large amount of list data because they only render data in the visual area to DOM:
-
<any collection-repeat="...">...</any>
The collection-repeat instruction accepts an enumeration expression and can attach the following attributes:
- item-width-optional. Declare the width of duplicate elements
- item-height-optional. Declare the height of duplicate elements
- item-render-buffer-optional. The number of visual data before and after loading the rendering buffer. The default is 3.
- force-refresh-images-optional. Whether the image is forced to refresh when scrolling. Permissible value: true | false
-
<!DOCTYPE html>
-
<html ng-app="myApp">
-
<head>
-
<meta charset="UTF-8">
-
<meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no,width=device-width,height=device-height">
-
<script src="../lib/js/ionic.bundle.min.js"></script>
-
<link rel="stylesheet" type="text/css" href="../lib/css/ionic.min.css">
-
</head>
-
<body ng-controller="myCtrl">
-
<ion-header-bar class="bar-positive">
-
<h1 class="title">collection-repeat</h1>
-
</ion-header-bar>
-
<ion-content>
-
<ion-list>
-
<ion-item collection-repeat="item in items">
-
{{item}}
-
<ion-option-button class="button-positive">...</ion-option-button>
-
</ion-item>
-
</ion-list>
-
</ion-content>
-
<script type="text/javascript">
-
angular.module("myApp",["ionic"])
-
.controller("myCtrl",function($scope){
-
$scope.items = [];
-
for(var i=0;i<5000;i++)
-
$scope.items.push(["item",i+1].join(""));
-
});
-
</script>
-
</body>
-
</html>
IV. Script Interface: $ionicListDelegate
If you need to control list elements from scripts, you can use the $ionicListDelegate service:
- showReorder([showReorder]) - Display/close the sort button showReorder is allowed to be true | false. You can use an expression in scope.
- showDelete([showDelete]) - Display/close the delete button showDelete with the allowable value of true | false. You can use an expression in scope.
- Can Swipe Items ([can Swipe Items]) - Whether the allowable value of the member option button can Swipe Items is allowed to be displayed by sliding: true | false. You can use a scoped expression closeOptionButtons() - close all options buttons.
-
<!DOCTYPE html>
-
<html ng-app="ezApp">
-
<head>
-
<meta charset="UTF-8">
-
<meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no,width=device-width,height=device-height">
-
<script src="../lib/js/ionic.bundle.min.js"></script>
-
<link rel="stylesheet" type="text/css" href="../lib/css/ionic.min.css">
-
</head>
-
<body ng-controller="myCtrl">
-
<ion-header-bar align-title="left" class="bar-positive">
-
<h1 class="title">$ionicListDelegate</h1>
-
<a class="button" ng-click="close();">choosing close</a>
-
</ion-header-bar>
-
<ion-content>
-
<ion-list>
-
<ion-item ng-repeat="item in items">
-
{{item}}!
-
<ion-option-button class="button-calm icon ion-share"></ion-option-button>
-
</ion-item>
-
</ion-list>
-
</ion-content>
-
</body>
-
</html>
-
-
-
<script>
-
angular.module("ezApp",["ionic"])
-
.controller("myCtrl",["$scope","$ionicListDelegate",function($scope,$ionicListDelegate){
-
$scope.items = ["Chinese","English","Russian","Japanese"];
-
var flag = false;
-
$scope.close = function(){
-
$ionicListDelegate.closeOptionButtons();
-
};
-
}]);
-
-
</script>