This article is reproduced. Introduction to Vue Learning Notes-Class and Style Binding
Binding HTML Class
A common requirement for data binding is the class list of operational elements and its inline style. Because they are attributes, we can deal with them with v-bind: we just need to compute the final string of the expression. However, string splicing is troublesome and error-prone. Therefore, when v-bind is used for classes and styles, Vue.js specifically enhances it. The result type of an expression can be an object or an array in addition to a string.
In the example, we write two simple styles to test:
<style> .active{ background-color: lightskyblue; } .text-danger{ color: red; } </style>
This style note is simple, active is to set the background color, text-danger is to set the text color.
The next step is to implement Class binding.
Object Grammar
We can pass an object to v-bind:class to dynamically switch classes. For example:
<div id="app"> <label v-bind:class="{active:isActive, 'text-danger':hasError}">test</label> </div> var app = new Vue({ el:'#app', data:{ isActive:true, hasError : false, } })
The results are as follows:

If you change the values of isActive and hasError in the console, you will find that the interface style will change accordingly according to the result of the modification.
You can also directly bind an object in the data:
<div id="app"> <label v-bind:class="classObj">test</label> </div> var app = new Vue({ el:'#app', data:{ classObj:{ active:true, 'text-danger':true } } })
The results are as follows:
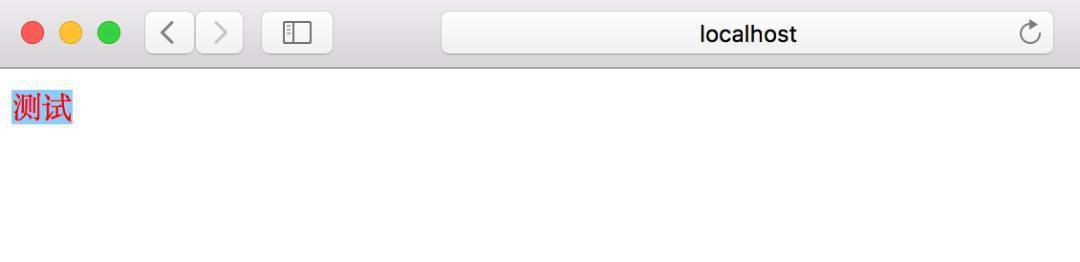
This effect is consistent with the previous one. As for why text-danger needs quotation marks, it is because the'-'sign written in js is considered minus sign, so quotation marks need to be added.
If you agree, it is also possible to use computational attributes, as follows:
<div id="app"> <label v-bind:class="classObj">test</label> </div> var app = new Vue({ el:'#app', computed:{ classObj:function () { return { 'active':true, 'text-danger':true } } } })
Operation results:
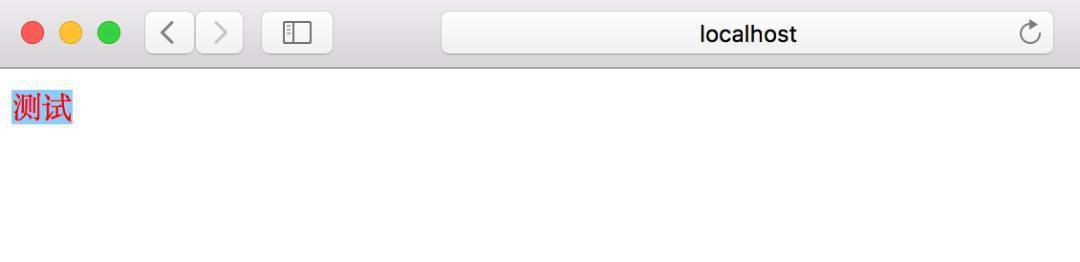
Array grammar
They can pass an array to v-bind:class to apply a list of classes:
<div id="app"> <label v-bind:class="[activeClass, errorClass]">test</label> </div> var app = new Vue({ el:'#app', data:{ activeClass:'active', errorClass:'text-danger' } })
Operation results:
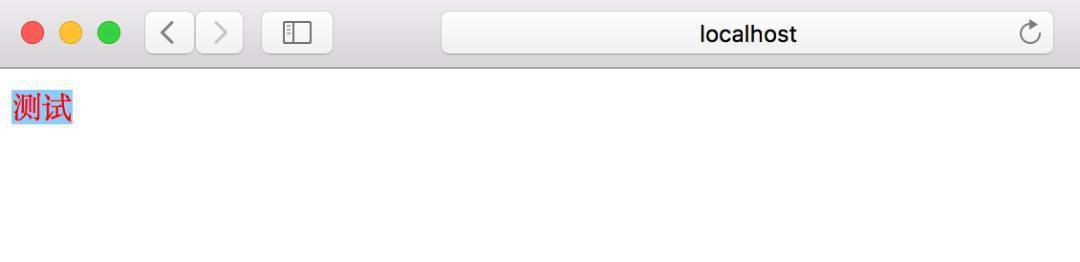
If you also want to switch class es in the list according to conditions, you can use ternary expressions:
<div id="app"> <label v-bind:class="[isActive ? activeClass : '', errorClass]">test</label> </div> var app = new Vue({ el:'#app', data:{ isActive:false, activeClass:'active', errorClass:'text-danger' } })
The results are as follows:

This example always adds errorClass, but only if isActive is true.
However, when there are multiple conditional class es, it is a bit cumbersome to write like this. Object grammar can be used in array grammar:
<div id="app"> <label v-bind:class="[{active:isActive}, errorClass]">test</label> </div> var app = new Vue({ el:'#app', data:{ isActive:false, activeClass:'active', errorClass:'text-danger' } })
Operation results:

Used on components
When you use class attributes on a custom component, these classes will be added to the root element, and the existing classes on that element will not be overwritten. The method used is the same as that used in the above elements, so it will not be repeated.
Binding Inline Styles
Object Grammar
V-bind: The object syntax of style is intuitive -- it looks very much like CSS, but it's actually a JavaScript object. CSS attribute names can be named by camelCase or kebab-case with quotation marks:
<div id="app"> <label v-bind:style="{color:color, fontSize:fontSize + 'px'}">test</label> </div> var app = new Vue({ el:'#app', data:{ color:'red', fontSize:30 } })
The results are as follows:
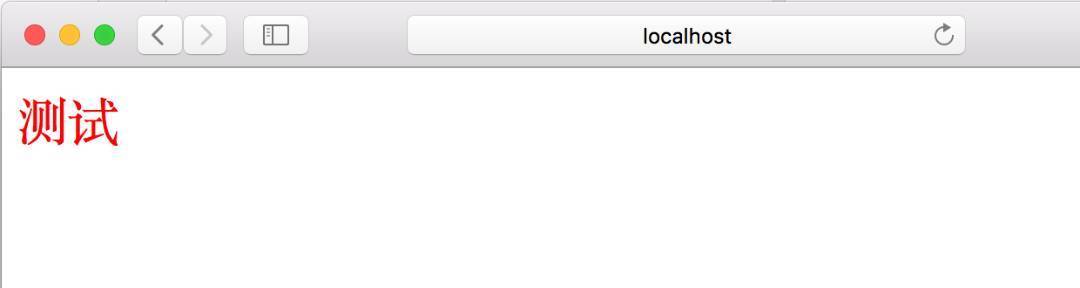
It is usually better to bind directly to a style object to make the template clearer:
<div id="app"> <label v-bind:style="classObj">test</label> </div> var app = new Vue({ el:'#app', data:{ classObj: { color: 'red', fontSize: '30px' } } })
The results are as follows:
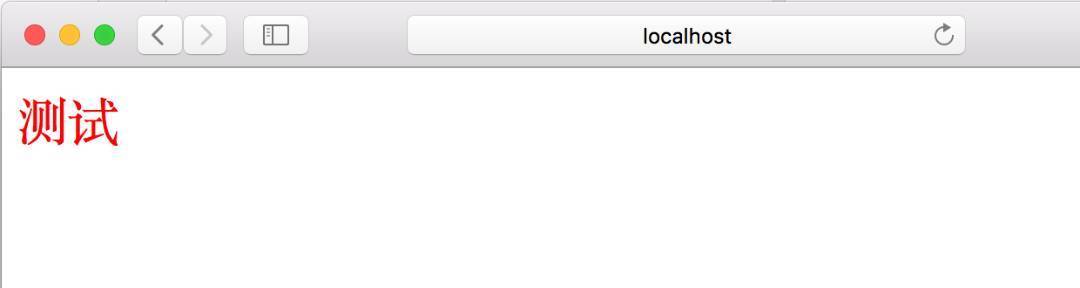
Similarly, object grammar is often used in conjunction with the computational properties of the returned object.
Array grammar
- The array syntax of bind:style can apply multiple style objects to an element:
<div v-bind:style="[baseStyles, overridingStyles]">
Automatically adding prefixes
When v-bind:style uses CSS attributes that require a specific prefix, such as transform, Vue.js automatically detects and adds the corresponding prefix.
Multiple values
Starting with 2.3, you can provide an array of multiple values for attributes in style bindings, often used to provide multiple prefixed values:
<div :style="{ display: ['-webkit-box', '-ms-flexbox', 'flex'] }">
This renders the last value supported by the browser in the array. In this example, if the browser supports flexbox without browser prefix, the rendering result will be display: flex.
finish
Original blog, reprinted please indicate the source
Last section: Introduction to Vue Learning Notes - Data and DOM
Return to the directory
Next section: Introduction to Vue Learning Notes - Use of Components