Array introduction
Array -An array is also an object-It is similar to our ordinary object function and is also used to store some values
-The difference is that ordinary objects use strings as attribute values, while arrays use numbers as index operation elements.
-Index:
Integer starting from 0
-The storage performance of array is better than that of ordinary objects. In development, we often use array to store some data Create array: var arr = new Array();
use When typeof checks an array, it returns object
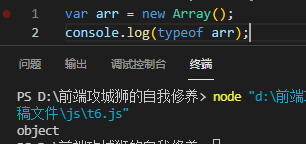
If you read a nonexistent index, it will not report an error, but return undefined Gets the length of the array have access to Length property to get the length of the array (number of elements)
Syntax: Array.length
For contiguous arrays, use Length can get the length of the array (number of elements)
For non contiguous arrays, use length will get the maximum index of the array + 1
Try not to create discontinuous arrays. modify length If modified If the length is less than the original length, the extra part will be empty
If modified If the length is less than the original length, the extra elements will be deleted Adds an element to the last position of the array Syntax: Array [array. length] = value
arr[arr.length] = 70; arr[arr.length] = 80; arr[arr.length] = 90;
array literal
Create an array using array literals
Syntax: []
var arr = [] ;
When you create an array with literals, you can specify the elements in the array at creation time
var arr = [1,2,3,4,5];
When using the build function to create an array, you can also add elements at the same time. The elements to be added are passed as parameters of the build function. The elements are separated by
var arr = new Array(1,2,3,4,5);
be careful:
Create an array with [] an element in the array 10
var arr = [10];
When you use the build function to create an array parameter, you create an empty array with a length of 10;
var arr = new Array(10); console.log(arr); console.log("arr.length="+arr.length);
Array can be any data type
var arr = ["Sun WuKong", 1, true, null, undefined]; console.log(arr);
Can be an object
var arr = [{name:"Sun WuKong"}, {name:"Zhu Bajie"}, {name:"Sha Wujing"}]; console.log(arr[0].name);
Can be a function
var arr = [ function () { alert(1) }, function () { alert(2) }];
Transfer function through arr[0] ()
Two dimensional array
establish:
Use []
var arr = [[1,2,3],[4,5,6],[7,8,9]]; //Two dimensional array with 3 rows and 3 columns
Using new Array
Access to elements Array name [row subscript] [column subscript]var a = new Array( new Array(10,20,30), new Array(11,22,33), new Array(45,56,67) )
(1) Transpose of two-dimensional array:
var a = [ ['a','b','c'], ['d','e','f'], ['g','h','i'], ['i','k','I'] ] var str = '' for(var i=0;i<a.length;i++){ for(var j=0;j<a[i].length;j++){ str += a[i][j]+'\t'; } str += '\n'; } console.log("Before transpose:\n",str); var res = [] for(var i=0;i<a[0].length;i++){ res[i] = [] for(var j=0;j<a.length;j++){ res[i][j] = a[j][i]; } } console.log("After transpose:",res);
var str = '' for(var i=0;i<a.length;i++){ //Outer loop: a.length indicates the number of rows of the two-dimensional array for(var j=0;j<a[i].length;j++){ //Inner loop: a[i].length indicates the number of elements (columns) in row I str += a[i][j]+'\t' } str += '\n'; //Add a newline at the end of each line } console.log(str); for(var i=0;i<a.length;i++){ var max = a[i][0] for(var j=1;j<a[i].length;j++){ if(max<a[i][j]){ max = a[i][j]; } } console.log("The first"+(i+1)+"The maximum value of the row is:"+max) }