abnormal
- Concept: an unexpected condition that occurs during program operation, rather than a compilation error.
1 Classification of anomalies
- For example:
All subclasses of Exception are checked exceptions and compile time exceptions.1. Throwable: The parent class of all exceptions. 2. Error: Throwable Subclass, indicating error, JVM collapse,Hardware problems or operating system related problems;Features: can not be avoided, can not be handled. 3. Exception:Throwable Subclass representing exception, compile time exception or checked exception. 4. RuntimeException: Exception Subclass, representing exception, runtime exception or unchecked exception.
All subclasses of RuntimeException are unchecked exceptions and runtime exceptions.It is a known exception of a program, which must be handled manually to solve the problem, otherwise the compilation error will occur. Features: unavoidable and must be handled.
It is because programmers do not check the exceptions well. They can provide solutions or not, and the compiler does not intervene. Features: can be avoided, can be handled or not.
2. Generation mode of abnormality
2.1 auto throw exception
- When writing the program, it is not rigorous, it is not well checked, and the exception occurs automatically in the wrong way of use.
Demonstration: array subscript out of bounds exception.String str = "ABC"; char[] cs = str.toCharArray(); System.out.println( cs[3] );
2.2 throw exception manually
- The syntax is as follows:
demonstration:throw Exception object;
NullPointerException npe = new NullPointerException(); throw npe; throw new NullPointerException(); throw new NullPointerException("Custom exception description information");
2.3 impact of abnormality on procedure
- The program will terminate due to the occurrence of an exception.
- Exception transfer process:
The demo code is as follows:Similar to the return value of a method, the exception object is returned layer by layer along the method call chain.
package com.txw.test; public class TestException { public static void main(String[] args) { System.out.println("main---start"); m1( 0 ); System.out.println("main---end"); } public static void m1(int n){ System.out.println("m1---start"); // Exception object // NullPointerException npe = new NullPointerException(); // if(n==0) throw npe; m2( n ); System.out.println("m1---end"); } public static void m2(int n){ System.out.println("m2---start"); if(n==0)throw new NullPointerException("Null pointer exception"); System.out.println("m2---end"); } }
3. Handling methods of exceptions [ key ]
3.1 statement throwing [negative handling]
- Syntax: add a declaration throw to the checked method.
Declare the impact of throwing on the program: who does not handle it, who calls who handles it.Access modifier return value type method name(Parameter table) throws abnormal,Exception 2...{ }
demonstration:
You can declare to throw a parent exception and handle all child exceptions (polymorphic).public static void main(String[] args) throws Exception{ System.out.println("main---start"); m1( 2 ); System.out.println("main---end"); } public static void m1(int n)throws IOException,SQLException { System.out.println("m1---start"); m2( n ); System.out.println("m1---end"); } public static void m2(int n)throws EOFException,FileNotFoundException,SQLException{ System.out.println("m2---start"); if(n==0)throw new NullPointerException("Null pointer exception"); if(n==1)throw new EOFException(); if(n==2)throw new FileNotFoundException(); if(n==3)throw new SQLException(); System.out.println("m2---end"); }
The demo code is as follows:
package com.txw.test; import java.io.EOFException; import java.io.FileNotFoundException; import java.io.IOException; import java.sql.SQLException; public class TestThrows { public static void main(String[] args) throws Exception{ System.out.println("main---start"); m1( 2 ); System.out.println("main---end"); } public static void m1(int n)throws IOException,SQLException { System.out.println("m1---start"); m2( n ); System.out.println("m1---end"); } public static void m2(int n)throws EOFException,FileNotFoundException,SQLException{ System.out.println("m2---start"); if(n==0)throw new NullPointerException("Null pointer exception"); if(n==1)throw new EOFException(); if(n==2)throw new FileNotFoundException(); if(n==3)throw new SQLException(); System.out.println("m2---end"); } }
3.2 capture processing [ active processing ]
- When an exception occurs in the method, you can capture the exception object and jump to the code block dedicated to handling the exception. After processing, the program will automatically convert from exception to normal. The program will not terminate unexpectedly due to the occurrence of exception, and can be used together with throws.
- The syntax is as follows:
The demo code is as follows:try{ // Possible exception codes // Used to catch exceptions }catch(Declared exception 1 | Claim handling exception 2 e){ [Optional]e.printStackTrace(); // Print exception stack information (exception source) [Optional]String message = e.getMessage(); // Print description information System.out.println(message); [Optional]throw e; // Declare again and throw an exception }catch(Declared exception e){ }...finally{ // No matter whether there is an exception, it must be executed // Usually used to free (close) resources }
package com.txw.test; import java.io.EOFException; import java.io.FileNotFoundException; import java.sql.SQLException; import java.util.zip.DataFormatException; public class TestTryCatch2 { public static void main(String[] args) { try { m1(1); }catch(SQLException e){ }catch(Exception e){ } } public static void m1(int n) throws SQLException,DataFormatException{ // Otorhinolaryngology try { System.out.println("m1------1"); m2( n ); System.out.println("m1------2"); }catch(FileNotFoundException | EOFException e) { e.printStackTrace(); } /*catch(IOException e){ // You can handle IOException or any subclass exception }*/ System.out.println("m1------3"); } public static void m2(int n)throws EOFException,FileNotFoundException,SQLException,DataFormatException{ System.out.println("m2---start"); if(n==0)throw new NullPointerException("Null pointer exception"); if(n==1)throw new EOFException("The file has been read!!!!"); // sneeze if(n==2)throw new FileNotFoundException(); // have a sore throat if(n==3)throw new SQLException(); // Toothache if(n==4)throw new DataFormatException(); // headache System.out.println("m2---end"); } }
You can declare to handle parent exceptions. When multiple catch es are required, follow the order from child to parent.
The demo code is as follows:
package com.txw.test; import java.io.EOFException; import java.io.FileNotFoundException; import java.sql.SQLException; public class TestTryCatch1 { public static void main(String[] args) { System.out.println("main---start"); m1( 1 ); System.out.println("main---end"); } public static void m1(int n) { try { // Catch exception objects and assign values to exception references in catch System.out.println("m1---start"); m2(n); }catch(EOFException e){ // Execute when EOFException occurs // System.out.println("catch EOFException"); // e.printStackTrace(); // Print exception information String message = e.getMessage(); System.out.println(message); }catch(FileNotFoundException e){ // System.out.println("catch FileNotFoundException"); }catch(SQLException e){ // System.out.println("catch SQLException"); } System.out.println("m1---end"); } public static void m2(int n)throws EOFException,FileNotFoundException,SQLException{ System.out.println("m2---start"); if(n==0)throw new NullPointerException("Null pointer exception"); if(n==1)throw new EOFException("The file has been read!!!!"); if(n==2)throw new FileNotFoundException(); if(n==3)throw new SQLException(); System.out.println("m2---end"); } }
demonstration:
try { }catch(SQLException e){ }catch(Exception e){ }
Note: the code in the try box may not be executed. When the method needs to return a value, the return statement should be written in the outer layer as much as possible.
try{ 1. Log in and enter the card number and password 2. Enter transfer amount 3. Verify balance 4. Deduction from original account -2000 An exception occurred 5. Increase balance for opposite account }catch(Exception e){ Original account balance +2000 }finally{ Return card }
- Common try catch structure.
If there is a return statement in the finally code box, it will be returned as the final result of the method.1. try{ }catch(Exception e){ } 2. try{ }catch(Exception e){ }finally{ } 3. try{ }finally{ }
About exceptions: generally, programmers will not throw exceptions manually because it is too expensive. Usually, they are dealing with checked exceptions thrown by the framework or other classes.
The demo code is as follows:
package com.txw.test; import java.io.EOFException; import java.sql.SQLException; public class TestTryCatchFinally { public static void main(String[] args)throws SQLException{ System.out.println("main---start"); m1( 2 ); System.out.println("main---end"); } public static void m1(int n) throws SQLException{ try { System.out.println("m1---1"); m2(n); System.out.println("m1---2"); }catch(EOFException e){ e.printStackTrace(); }finally{ System.out.println(" finally { }"); } System.out.println("m1---3"); } public static void m2(int n)throws EOFException,SQLException{ System.out.println("m2---start"); if(n==0)throw new NullPointerException("Null pointer exception"); if(n==1)throw new EOFException(); if(n==2)throw new SQLException(); System.out.println("m2---end"); } }
4 custom exception
- Custom checked exception.
// Inherit Exception class MyException1 extends Exception{ public MyException1(){} public MyException1(String message){ super(message); // Use the parent class construction method to add description information } } // Throw exception throw new MyException1("Custom checked exception");
- Custom unchecked exception [recommended].
// Inherit RuntimeException class MyException2 extends RuntimeException{ public MyException2(){} public MyException2(String message){ super(message); // Use the parent class construction method to add description information } } // Throw exception throw new MyException2("Custom checked exception");
The demo code is as follows:
package com.txw.test; public class TestMyException { public static void main(String[] args) throws MyException1{ m1(2); } public static void m1(int n)throws MyException1{ if(n==1)throw new MyException1("Custom checked exception"); // Throw custom checked exception if(n==2)throw new MyException2("Custom unchecked exception"); } } // Custom checked exception class MyException1 extends Exception{ public MyException1(){} public MyException1(String message){ super(message); // Use parent class construction method } } // Custom unchecked exception class MyException2 extends RuntimeException{ public MyException2(){} public MyException2(String message){ super(message); } }
5 syntax requirements for method coverage
- Syntax requirements:
The demo code is as follows:The access modifier is the same or wider, and the return value type, method name and parameter table are consistent with the parent class. You cannot throw a broader exception than the parent class.
class Super{ public void m1()throws IOException {} } class Sub extends Super{ @Override public void m1()throws FileNotFoundException, EOFException {} // Although the m1 method throws a large number of exceptions, they are subclasses of IOException and are not broader than those thrown by the parent method }
- Interview questions
The demo code is as follows:1. final,finally,finalize The difference between the three final It's a modifier finally Is a code block finalize yes Object Class
package com.txw.test; import java.io.EOFException; import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; public class TestOverride { public static void main(String[] args){ // Can represent a file on your computer try { File f = new File("D:/abc.txt"); f.createNewFile(); }catch(Exception e){ e.printStackTrace(); } } } class Super{ public void m1()throws IOException {} } class Sub extends Super{ @Override public void m1()throws FileNotFoundException, EOFException {} }
package com.txw.test; public class TestReturnResult { public static void main(String[] args) { System.out.println(method()); } public static int method(){ // The return value is usually declared outside the try box int count = 0; try { // Define count variables for (int i = 1; i <= 999; i++) { if(i%2==0)count++; } return 1; }catch(Exception e){ e.printStackTrace(); return 2; }finally{ return 2000; } } }
exercises
1. All errors in Java inherit from Throwable class; In the subclass of this class, Error class represents serious underlying errors, for which the program code cannot handle; Exception indicates exception and exception.
2. Consult API and complete the following blanks:
(1) The exception class java.rmi.AlreadyBoundException belongs to checked (checked | runtime) exceptions in terms of classification. In terms of handling methods, such exceptions must be handled.
(2) The exception class java.util.regex.PatternSyntaxException belongs to | runtime (checked | runtime) exception in terms of classification. In terms of handling method, this kind of exception can be handled or not handled.
3. The keyword used to throw exceptions in Java is (C).
A. try
B. catch
C. throw
D. finally
4. In exception handling, releasing resources, closing files, etc____ Process (C).
A. try statement
B. catch statement
C. finally statement
D. throw statement
5. Code in finally statement block (A).
A. Always executed
B. When there is no catch after the try statement block, the code in finally will be executed
C. It is executed only when an exception occurs
D. It is executed only when the exception does not occur
6. When customizing exception classes, the classes that can be inherited are (C)
A. Error
B. ArrayList
C. Exception
D. NullPointerException
7. For the arrangement of try {...} catch... Statements, the following description is correct (A).
A. Subclass exceptions precede and parent exceptions follow
B. The parent class exception precedes the child class exception
C. Only subclass exceptions are allowed
D. A parent exception cannot occur at the same time as a child exception
8. Read the following codes carefully and complete them.
package com.txw.test; public class TestThrow { public static void main(String[] args) { throwException(10); } public static void throwException(int n){ if (n == 0) { // Throw a NullPointerException }else { // Throw a ClassCastException // And set the details to type "conversion error" } } }
Answer: the code is as follows:
package com.txw.test; public class TestThrow { public static void main(String[] args) { throwException(10); } public static void throwException(int n){ if (n == 0) { // Throw a NullPointerException throw new NullPointerException(); }else { // Throw a ClassCastException // And set the details to "type conversion error" throw new ClassCastException("Type conversion error"); } } }
- Code error correction: carefully read the following procedures to correct the wrong code.
package com.txw.test; class MyException{ } public class TestException { public static void main(String[] args) { ma(); } public static int ma(){ try { m(); return 100; } catch (Exception e) { System.out.println("Exception"); }catch (ArithmeticException e){ System.out.println("ArithmeticException"); } } public static void m(){ throw new MyException(); } }
A: the corrected code is as follows:
package com.txw.test; // The MyException class must inherit from a subclass of Exception class MyException extends RuntimeException{ } public class TestException { public static void main(String[] args) { ma(); } public static int ma(){ try { m(); } catch (ArithmeticException e) { System.out.println("ArithmeticException"); }catch (Exception e){ // Statements that catch exceptions should be placed at the end of all catch statements. System.out.println("Exception"); } return 100; } // Depending on whether MyException inherits from RuntimeException // Consider whether to declare throw here public static void m(){ throw new MyException(); } }
- Read the following code carefully. When the read n is 1, 2, 3, 4 and 5 respectively, what are the output results?
Answer:
1. When n = 1, output main1 ma1 mb1 Catch EOFException In finally main2
2. When n = 2, main1 ma1 mb1 Catch IOException In finally main2 is output
3. When n = 3, main1 ma1 mb1 Catch SQLException In finally main2 is output
4. When n = 4, main1 ma1 mb1 Catch Exception In finally main2 is output
5. When n = 5, main1 ma1 mb1 mb2 ma2 In finally main2 is output. Note: the In finally statement will be printed regardless of whether or not an exception occurs and what exception occurs. - Read the following code carefully:
At / / 1, fill in the following__ A B____ The code can be compiled and filled in at / / 2____ D__ The code can be compiled.
A. throws java.io.IOException
B. throws java.io.FileNotFoundException, java.io.EOFException
C. throws java.sql.SQLException
D. No exceptions can be thrown
reason:
1. According to the requirements of method coverage, the coverage method of a child class cannot throw a broader exception than the covered method of the parent class.
2. If the Ma method throws IOException, the subclass can throw IOException or subclass exception of IOException.
3. If the MB method does not throw any exceptions, the subclass cannot throw any exceptions. - Read the following code carefully. The correct description of the program is (A).
A. Compilation failed
B. Compile passed, output - 1
C. Compile and output 0
D. None of the above descriptions is correct
Reason: after an exception occurs in the assignment statement of N, find the code in the catch execution, and finally return n. at this time, because the assignment statement of n is abnormal, the assignment fails. N has no value, and the local variable must have a value before it can be used. - Read the following code carefully. In the ma method, when the read in b is 100, the output result is___ 100___, When the read in b is 0, the output result is 100.
Reason: the code in Finally must be executed. - Read the following code carefully. In the Ma method, read in the integer b. if the read value is 10, the output is_ ma1 ma2 1 In Finally ; If the read value is 0, ma1 In Finally is output.
- Read the following code carefully. Can it be compiled? If not, how should I modify it?
A: it cannot be compiled. Since MySub2 inherits from the MySub class, you cannot throw more exceptions than the methods in MySub. Because the m method in MySub class throws EOFException, while the FileNotFoundException thrown by the m method of MySub2 class is not a subclass of EOFException, this method throws more exceptions of the m method in MySub class, so the compilation fails. - Read the following code carefully. The correct description of the program is (A).
A. Compilation error
B. Compile normally and output main1 ma1 In Catch
C. Compilation is normal, error occurred while running
D. None of the above descriptions is correct
Reason: the second output statement in the ma method. Since the previous statement is a throw statement, the second output statement can never be executed, so there is a compilation error. - Read the following program carefully. Which of the following codes can be compiled at / 1 /.
A. catch(NullPointerException npe){}
B. catch(IOException ioe){}
C. catch(SQLException sqle){}
reason:
1. At / 1 /, because the ma method declaration may throw I OException, B compiles and passes.
2. Since the NullPointerException exception is A runtime exception, even if it is not declared to be thrown in the throws statement of the ma method, it may occur during the call. Therefore, A compiles and passes;
3. Because SQLException is not declared to be thrown and is a checked exception, it is impossible to catch this kind of exception at / 1 /, so C compilation does not pass. - Briefly describe the differences between final, finalize and finally.
A: final: modify variables, methods and classes.
The modification variable is a constant and can only be assigned a modification method once, which cannot be overwritten, and the modification class cannot be inherited. Finally: it is a part of exception handling, and the code in finally will be executed. Most of it is used to release resources in the database and io stream.
finalize: is a method in the Ojbect class for garbage collection. - There is a return statement in try. Will the statement in finally be executed? Why?
A: it will execute, and the code placed in finally will execute. - Read the following code carefully and write the result of program execution.
Answer:
StepB StepE
Cause: if s is null, calling the method will cause null pointer exception. Find the corresponding catch and execute the code in finally after execution. - Programming: create two custom exception classes MyException1 and MyException2. The requirements are as follows:
(1) MyException1 is the checked exception, and MyException2 is the runtime exception;
(2) Both exceptions have two constructors: one with no parameters and the other with string parameters. The parameters represent the information that causes the exception.
Demo code, you can refer to question 22. - On the basis of the previous question, complete the following code.
The demo code is as follows:
package com.txw.test; import java.util.Scanner; class MyException1 extends Exception { public MyException1() { super(); } public MyException1(String message) { super(message); } } class MyException2 extends RuntimeException { public MyException2() { super(); } public MyException2(String message) { super(message); } } public class TestMyException { public static void main(String args[]) { int n; // Read in n Scanner sc = new Scanner(System.in); n = sc.nextInt(); try { m(n); } catch (MyException1 ex1) { // Output ex1 detailed method call stack information ex1.printStackTrace(); } catch (MyException2 ex2) { // Output ex2 details System.out.println(ex2.getMessage()); // And throw ex2 back out of throw ex2; } } public static void m(int n) throws MyException1 { // Declaration throws MyException1 if (n == 1) { // Throw MyException1 // And set its details to "n == 1" throw new MyException1("n == 1"); } else { // Throw MyException2 // And set its details to "n == 2" throw new MyException2("n == 2"); } } }
summary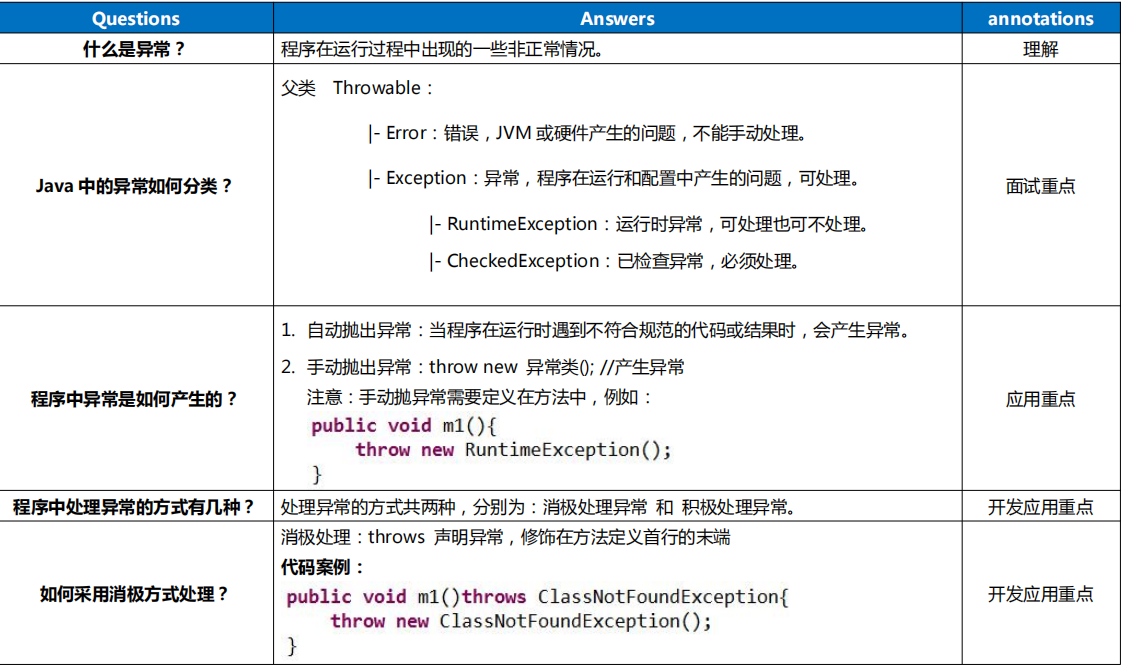