Intermediary model of design model
Keywords:
Design Pattern
Smart home project
- The family can only include all kinds of equipment: alarm clock, coffee machine, TV, curtains, etc.
- When the host wants to watch TV, various devices can work together to automatically complete the preparation for watching TV. For example: the alarm clock rings - > the coffee machine starts making coffee - > the curtains fall automatically - > the TV starts playing.
Intermediary model
Basic introduction
- The mediator pattern uses a mediation object to encapsulate the interaction of some columns of objects. Mediators make objects do not need to explicitly refer to each other, so that they are loosely coupled, and their interaction can be changed independently.
- The mediator pattern is a behavioral design pattern, which makes the code easy to maintain.
- For example, in MVC mode, the Controller acts as an intermediary between the Model and View view, and acts as an intermediary in front and back-end interaction.
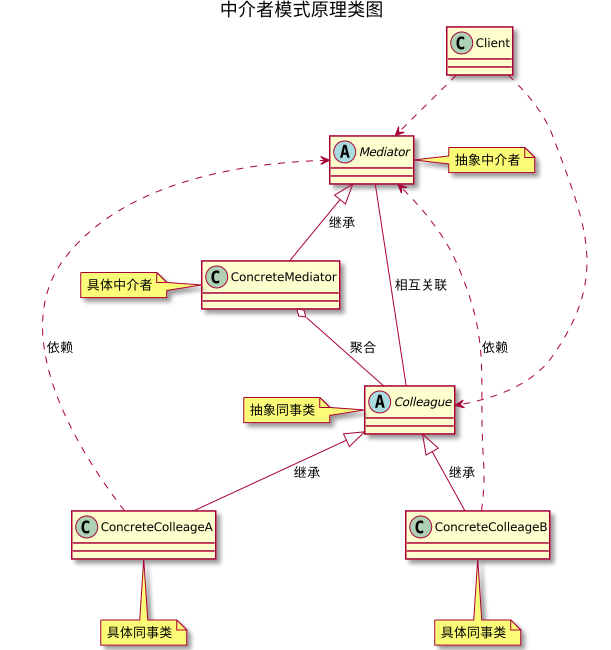
Solving the problem of smart home project with intermediary model
- UML class diagram
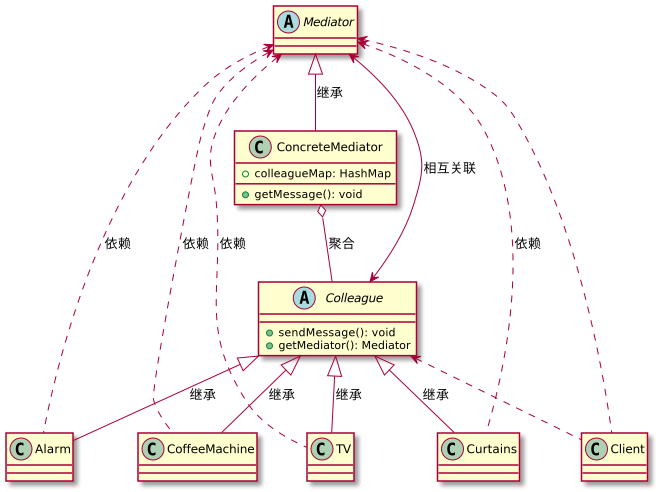
- code implementation
Abstract Mediator class:public abstract class Mediator {
// Add the mediator object to the collection
public abstract void register(String colleagueName, Colleague colleague);
// Receive the message sent by the specific colleague object
public abstract void getMessage(int stateChange, String colleague);
public abstract void sendMessage();
}
Abstract Colleague class collague class:public abstract class Colleague {
private Mediator mediator;
public String name;
public Colleague(Mediator mediator, String name) {
this.mediator = mediator;
this.name = name;
}
public Mediator getMediator() {
return this.mediator;
}
public abstract void sendMessage(int stateChange);
}
Specific TV category:public class TV extends Colleague {
public TV(Mediator mediator, String name) {
super(mediator, name);
// TODO Auto-generated constructor stub
mediator.register(name, this);
}
@Override
public void sendMessage(int stateChange) {
// TODO Auto-generated method stub
this.getMediator().getMessage(stateChange, this.name);
}
public void StartTv() {
System.out.println("It's time to StartTv!");
}
public void StopTv() {
// TODO Auto-generated method stub
System.out.println("StopTv!");
}
}
Specific colleagues: Curtains:public class Curtains extends Colleague {
public Curtains(Mediator mediator, String name) {
super(mediator, name);
// TODO Auto-generated constructor stub
mediator.register(name, this);
}
@Override
public void sendMessage(int stateChange) {
// TODO Auto-generated method stub
this.getMediator().getMessage(stateChange, this.name);
}
public void UpCurtains() {
System.out.println("I am holding Up Curtains!");
}
}
Specific Alarm class:public class Alarm extends Colleague {
public Alarm(Mediator mediator, String name) {
super(mediator, name);
mediator.register(name, this);
}
public void sendAlarm(int stateChange) {
sendMessage(stateChange);
}
@Override
public void sendMessage(int stateChange) {
this.getMediator().getMessage(stateChange, this.name);
}
}
Specific colleague CoffeeMachine class:public class CoffeeMachine extends Colleague {
public CoffeeMachine(Mediator mediator, String name) {
super(mediator, name);
// TODO Auto-generated constructor stub
mediator.register(name, this);
}
@Override
public void sendMessage(int stateChange) {
// TODO Auto-generated method stub
this.getMediator().getMessage(stateChange, this.name);
}
public void StartCoffee() {
System.out.println("It's time to startcoffee!");
}
public void FinishCoffee() {
System.out.println("After 5 minutes!");
System.out.println("Coffee is ok!");
sendMessage(0);
}
}
Concrete mediator class:import java.util.HashMap;
public class ConcreteMediator extends Mediator {
private HashMap<String, Colleague> colleagueMap;
private HashMap<String, String> interMap;
public ConcreteMediator() {
colleagueMap = new HashMap<>();
interMap = new HashMap<>();
}
@Override
public void register(String colleagueName, Colleague colleague) {
colleagueMap.put(colleagueName, colleague);
if (colleague instanceof Alarm) {
interMap.put("Alarm", colleagueName);
} else if (colleague instanceof CoffeeMachine) {
interMap.put("CoffeeMachine", colleagueName);
} else if (colleague instanceof TV) {
interMap.put("TV", colleagueName);
} else if (colleague instanceof Curtains) {
interMap.put("Curtains", colleagueName);
}
}
@Override
public void getMessage(int stateChange, String colleagueName) {
if (colleagueMap.get(colleagueName) instanceof Alarm) {
if (stateChange == 0) {
((CoffeeMachine) (colleagueMap.get(interMap
.get("CoffeeMachine")))).StartCoffee();
((TV) (colleagueMap.get(interMap.get("TV")))).StartTv();
} else if (stateChange == 1) {
((TV) (colleagueMap.get(interMap.get("TV")))).StopTv();
}
} else if (colleagueMap.get(colleagueName) instanceof CoffeeMachine) {
((Curtains) (colleagueMap.get(interMap.get("Curtains"))))
.UpCurtains();
} else if (colleagueMap.get(colleagueName) instanceof TV) {
} else if (colleagueMap.get(colleagueName) instanceof Curtains) {
}
}
@Override
public void sendMessage() {
}
}
Client class:public class Client {
public static void main(String[] args) {
Mediator mediator = new ConcreteMediator();
Alarm alarm = new Alarm(mediator, "alarm");
CoffeeMachine coffeeMachine = new CoffeeMachine(mediator,
"coffeeMachine");
Curtains curtains = new Curtains(mediator, "curtains");
TV tV = new TV(mediator, "TV");
alarm.sendAlarm(0);
coffeeMachine.FinishCoffee();
alarm.sendAlarm(1);
}
}
Considerations and details of the mediator model
- Multiple classes are coupled with each other to form a network structure. The mediator model is used to separate the mesh structure into star structure for decoupling.
- Reduce the dependence between classes and reduce the coupling, which is in line with the Demeter's law.
- Intermediaries assume more responsibilities. Once the intermediaries have problems, the system will be affected.
- If not designed properly, the mediator object itself becomes too complex. This should be paid attention to in actual transportation.
Posted by xmarcusx on Fri, 17 Sep 2021 16:50:55 -0700