Implementation of jQuery fade in and fade out rotation chart
Keywords:
Javascript
JQuery
Hello, I'm QD Xiaobai. This is the first time in my life to write a blog. I'm going to write about jQuery fading in and out of the implementation of the rotation chart. Before that, I didn't feel good about the effect of learning JS writing the rotation chart. After learning the fading in and out effect in jQuery, I wrote another rotation chart, which obviously felt much better. Now I'm going to share my ideas and methods of writing the broadcast chart.
HTML section:

<div id="img-box" style="width:700px; height:350px;">
<img style="opacity: 1;" width="700px" height="350px" id="imgis" alt="<Snowman's fate animated film desktop wallpaper"
src="https://img.ivsky.com/img/bizhi/pre/201909/25/abominable-003.jpg">
<img width="700px" height="350px" id="imgis" alt="Lush bamboo forest pictures"
src="https://img.ivsky.com/img/tupian/pre/201902/13/zhulin.jpg">
<img width="700px" height="350px" id="imgis" alt="Pictures of surging waves"
src="https://img.ivsky.com/img/tupian/pre/201903/14/hailang-001.jpg">
<img width="700px" height="350px" id="imgis" alt="Hourglass picture of timing"
src="https://img.ivsky.com/img/tupian/pre/201903/14/shalou-002.jpg">
<img width="700px" height="350px" id="imgis" alt="Picture of rape flower"
src="https://img.ivsky.com/img/tupian/pre/201902/13/youcaihua-002.jpg">
<img width="700px" height="350px" id="imgis" alt="Zile meaty picture"
src="https://img.ivsky.com/img/tupian/pre/201902/13/zile_duorou.jpg">
<div id="img-left"> < </div>
<div id="img-right"> > </div>
<div id="img-num">
<li class="num-one">0</li>
<li class="num-two">1</li>
<li class="num-three">2</li>
<li class="num-four">3</li>
<li class="num-five">4</li>
<li class="num-six">5</li>
</div>
</div>
HTML:
Part CSS:
<style>
* {
margin: 0;
padding: 0;
}
#img-box {
position: relative;
border: 25px solid #ccc;
margin: 100px auto;
}
#img-box img {
position: absolute;
display: block;
opacity: 0;
float: left;
cursor:pointer;
}
#img-box #img-left,
#img-box #img-right{
position: absolute;
width: 65px;
height: 120px;
border: 4px solid thistle;
border-radius: 6px;
background-color: black;
opacity: 0.6;
cursor: pointer;
color: #fff;
font-size: 30px;
text-align: center;
line-height: 120px;
}
#img-box #img-left {
left: 1px;
top: 31%;
}
#img-box #img-right {
right: 1px;
top: 31%;
}
#img-box #img-num{
position:absolute;
width:500px;
height:50px;
/* border:2px solid red; */
bottom:0px;
left:15%;
display:flex;
flex-direction:row;
justify-content:space-around;
}
#img-box #img-num li{
display:inline-block;
list-style:none;
font-size:14px;
color:#fff;
text-align: center;
line-height: 40px;
cursor: pointer;
width:45px;
height:40px;
border-radius: 50%;
background-color:black;
}
</style>
This is HTML and CSS. The effect of the demonstration is as follows:
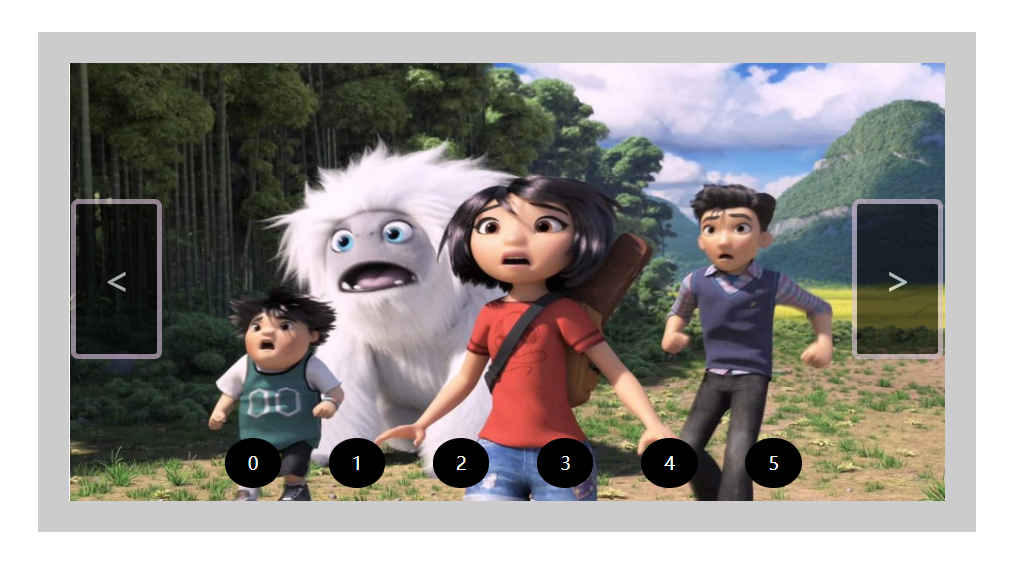
The following is the JS part:
<script src="jqwj/jquery-1.12.4.js"></script>
<script>
$(document).ready(function () {
var imgLeath = $("img").length;
var liLeath = $("li").length;
// Show the subscript of the picture (global variable)
var n = 0;
//set timer timer
var timer = setInterval(time, 4000);
// Rotation function
// index Hide picture for ondex Picture displayed for
function time() {
n++;
if (n == imgLeath) {
n = 0;
}
for(var i=0; i<imgLeath; i++) {
$("#img-box img").eq(i).fadeTo(0,0);
}
$("#img-box img").eq((n)).delay(50).fadeTo(500, 1);
}
//Set Click to switch effect (right)
var rightteum;
$("#img-right").click(function () {
for (var i = 0; i < imgLeath; i++)
{
if ($("#img-box img").eq(i).css("opacity") != '0')
{
// Get the subscript of the current picture display
righteum = i;
}
}
if(righteum == 5)
{
righteum = 5;
}
n = (righteum);
time();
});
// Set Click to switch effect (Left)
var lefteum;
$("#img-left").click(function () {
for(var i=0; i<imgLeath; i++){
if($("#img-box img").eq(i).css("opacity") != '0'){
// Get the subscript of the current picture
lefteum = i;
}
}
if(lefteum == 0){
lefteum = 6;
}
n = (lefteum-2);
time();
});
// When the mouse moves into the frame, the carousel stops
$("#img-box").mouseover(function(){
clearInterval(timer);
});
//When the mouse is moved out of the frame, the carousel chart is executed.
$("#img-box").mouseout(function(){
timer = setInterval(time,4000);
});
//click li The sequence number picture will switch to that one
for(var i=0; i<liLeath; i++) {
//Closure problem
(function(i){
$("#img-num li").eq(i).click(function(){
// i To toggle the subscript of a picture
console.log(i);
//hold (i-1) Pass to n Because in time() Function n++
n = (i-1);
time();
});
})(i)
}
})
</script>
Let me talk about my ideas and ideas for writing this round robin chart:
This time() function is the key of the whole rotation chart. The rotation chart is displayed and hidden through the fadeTo() function of jQuery. The defined n is the subscript of which picture to display. The for loop in time() is to traverse all the pictures before displaying the next picture, so as to prevent the transparency of pictures and displayed pictures from being 1.
Click the button to switch the effect: the purpose of for cycling is to get the subscript of the picture displayed in the six pictures, and then pass the value to n according to the situation of left and right switching and execute the function time().
Mouse in and out function: start timer: timer = setInterval(time,4000); stop timer: clearInterval(timer);
Click the sequence number of li to switch the picture: cycle through the whole li through for(). There is also a closure problem that must use the immediate execution function. The idea is the same as clicking the button to switch the effect.
This is what we need to write the whole broadcast chart. I hope you can adopt it. The blog pictures are from Paradise pictures. Com. Crabs and crabs!
2019-10-16 20:57:14