In practical development, we may encounter this situation: the picture needs to be rotated at an angle.For this purpose, we may use the transform ation of the UIView to rotate, but doing so only rotates the view that hosts the imageView at an angle, and the imageView does not rotate.All this is not good.
If you need to rotate the imageView at an angle, the steps are:
1. Convert image to context.
2. Rotate the context at an angle.
3. Convert the rotated context to an image.
With these three steps, we can really rotate the picture.Okay, go directly to the code:
#import"UIImage+RotateImageTool.h" #import<QuartzCore/QuartzCore.h> #import<Accelerate/Accelerate.h> @implementationUIImage (RotateImageTool) -(UIImage*)rotateImageWithDegree:(CGFloat)degree{ //Convert image to context //Get the width and height of the picture pixels size_t width =self.size.width*self.scale; size_t height =self.size.height*self.scale; //The color channel is 8 because 0-255 undergoes eight color channel changes //Number of bytes per line because we're using ARGB/RGBA so the number of bytes is width * 4 size_t bytesPerRow =width *4; //Transparency channel for pictures CGImageAlphaInfo info =kCGImageAlphaPremultipliedFirst; //Configure the parameters of the context: CGContextRef context =CGBitmapContextCreate(nil, width, height,8, bytesPerRow,CGColorSpaceCreateDeviceRGB(),kCGBitmapByteOrderDefault|info); if(!context) { return nil; } //Render Picture to Graphic Context CGContextDrawImage(context,CGRectMake(0,0, width, height),self.CGImage); uint8_t* data = (uint8_t*)CGBitmapContextGetData(context); //Rotate underdue data vImage_Buffer src = { data,height,width,bytesPerRow}; //Rotated data vImage_Buffer dest= { data,height,width,bytesPerRow}; //background color Pixel_8888 backColor = {0,0,0,0}; //fill color vImage_Flags flags = kvImageBackgroundColorFill; //Rotate context vImageRotate_ARGB8888(&src, &dest,nil, degree *M_PI/180.f, backColor, flags); //Convert conetxt to image CGImageRef imageRef =CGBitmapContextCreateImage(context); UIImage* rotateImage =[UIImageimageWithCGImage:imageRefscale:self.scaleorientation:self.imageOrientation]; returnrotateImage; }
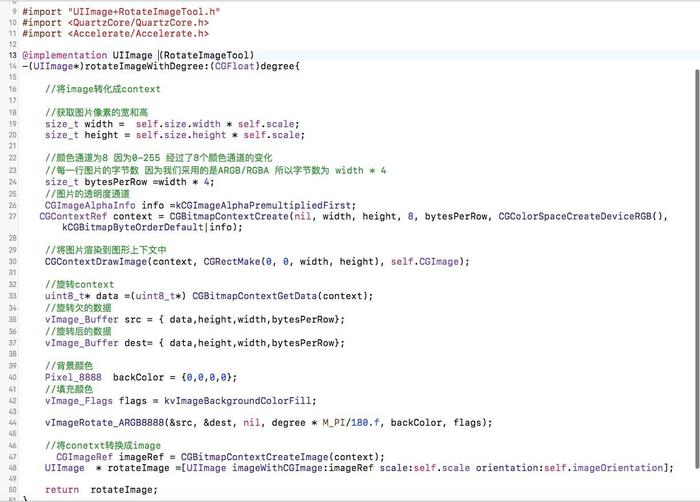
There are detailed comments in the code, and I don't explain much here.If you are interested, you can download it on github.Download address: github.com/15221532825/ImageTool