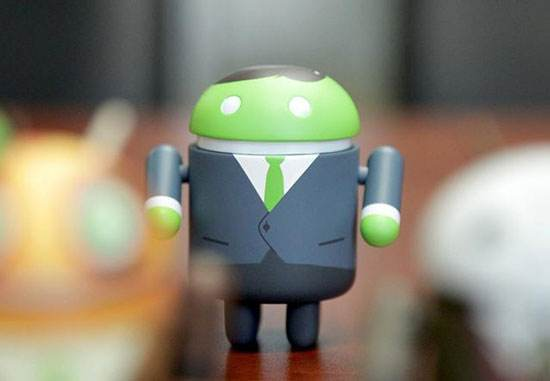
There are two ways to pass objects with Intent: Serializable and Parcelable.
1 Serializable mode
Suppose there is an Account class of POJO, which implements the Serializable interface:
public class Account implements Serializable { //Full name private String name; //Password private String pwd; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPwd() { return pwd; } public void setPwd(String pwd) { this.pwd = pwd; } @Override public String toString() { return "Account{" + "name='" + name + '\'' + ", pwd='" + pwd + '\'' + '}'; } }
Serializable means to convert an object into a state that can be stored or transferred. Serialized objects can be transferred on the network or stored locally. In order to serialize a class, we only need to implement the serializable interface (tag interface) O(∩∩) o~
Set the Account object in MainActivity:
Account account = new Account(); account.setName("deniro"); account.setPwd("ew23408"); Intent intent = new Intent(MainActivity.this, NextActivity.class); intent.putExtra("account", account); startActivity(intent);
Here we pass account directly to the putExtra() method of Intent.
Get the Account object in NextActivity:
Account account=(Account)getIntent().getSerializableExtra("account"); Log.d(TAG, "account: "+account);
Output results:
D/NextActivity: account: Account{name='deniro', pwd='ew23408'}
2 Parcelable mode
The Parcelable method will decompose the object, so that every part after decomposition is the data type supported by Intent. In this way, you can realize the function of passing the object (∩∩∩∩∩∩∩∩∩∩∩∩∩∩) O~
public class Account2 implements Parcelable { //Full name private String name; //Password private String pwd; public Account2() { } public static final Creator<Account2> CREATOR = new Creator<Account2>() { @Override public Account2 createFromParcel(Parcel in) { Account2 entity = new Account2(); entity.name = in.readString(); entity.pwd = in.readString(); return entity; } @Override public Account2[] newArray(int size) { return new Account2[size]; } }; @Override public int describeContents() { return 0; } @Override public void writeToParcel(Parcel dest, int flags) { dest.writeString(name); dest.writeString(pwd); } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPwd() { return pwd; } public void setPwd(String pwd) { this.pwd = pwd; } @Override public String toString() { return "Account{" + "name='" + name + '\'' + ", pwd='" + pwd + '\'' + '}'; } }
The describeContents and writeToParcel methods in the Parcelable must be implemented. The describeContents method is rarely used and can be implemented by default. The writeToParcel method is used to write data to Parcel.
We also need to define a CREATOR constant. Generics are the classes we need to pass. The createFromParcel method in it is used to read data from the Parcel. Note: the read order here must be the same as the write order previously defined in the writeToParcel method!
The code of the passed parameter is the same as that of Serializable:
Account2 entity = new Account2(); entity.setName("deniro"); entity.setPwd("wdour23"); intent.putExtra("account2",entity);
The code to read the parameters is as follows:
Account2 account2= getIntent().getParcelableExtra("account2"); Log.d(TAG, "account2: "+account2);
Output results:
D/NextActivity: account2: Account{name='deniro', pwd='wdour23'}