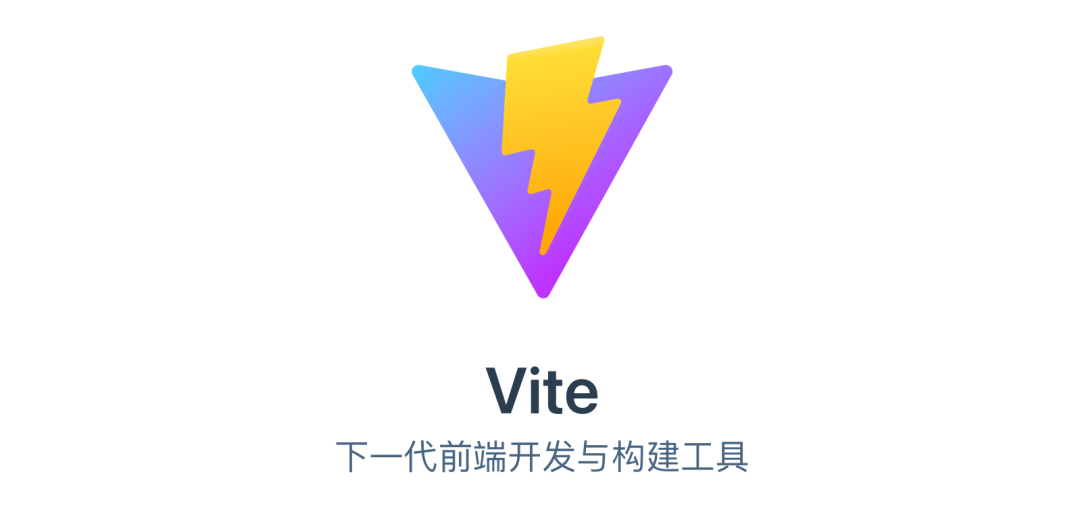
preface
I haven't sent a document for two weeks. I'm a little delayed temporarily. I'm deeply sorry to you here. Today, we use vite2.0+vue3+ts to develop a demo project.
actual combat
Let's open Vite's official website( https://cn.vitejs.dev/ ).
Vite (French for "fast", pronounced / vit /) is a new front-end construction tool that can significantly improve the front-end development experience. It mainly consists of two parts: A development server that provides rich built-in functions based on native ES modules, such as amazing module hot update (HMR). A set of build instructions that use Rollup to package your code, and it is pre configured to output optimized static resources for the production environment. Vite is intended to provide out of the box configuration. At the same time, its plug-in API and JavaScript API bring a high degree of scalability and complete type support.
Here, let's compare Vite with VueCLI.
- Vite can run directly without packaging in the development mode, using the modular loading rules of ES6;
- In VueCLI development mode, the project must be packaged before it can run;
- Vite cache based hot update;
- VueCLI hot update based on webpack;
Construction project
Let's build the first Vite project. I use the Yarn dependency management tool to create the project.
yarn create @vitejs/app
Type the above command on the command line, and then you may wait for a while~
Configure Project name, Select a template
Project name: vite-vue-demo Select a template: vue-ts
Because we use Vue+Ts to develop projects here, we choose Vue TS as the template. After one operation, you will be prompted to type the following commands and fill in them in turn.
cd vite-vue-demo yarn yarn dev
In this way, we have completed the construction project.
Project folder list
We will see the following files and their folders.
node_modules ---Dependent folder public ---Public folder src ---Project main folder .gitignore ---exclude git Submit profile index.html ---Entry file package.json ---Module description file tsconfig.json ---ts configuration file vite.config.ts ---vite configuration file
Pre development configuration
Before development, we need to do the following work.
1. Edit ts configuration file
Configure the required configuration items as needed. In compilerOptions, the configuration items are configured at compile time. The include item is the path included in the configuration effectiveness. On the contrary, the exclude item is the excluded path.
{ "compilerOptions": { "target": "esnext", "module": "esnext", "strict": true, "jsx": "preserve", "importHelpers": true, "moduleResolution": "node", "experimentalDecorators": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "sourceMap": true, "baseUrl": ".", "types": ["vite/client"], "paths": { "@/*": [ "src/*" ] }, "lib": [ "esnext", "dom", "dom.iterable", "scripthost" ] }, "include": [ "src/**/*.ts", "src/**/*.tsx", "src/**/*.vue", "tests/**/*.ts", "tests/**/*.tsx" ], "exclude": [ "node_modules" ] }
2. Configure vite profile
Why do I need to configure this file? This is because we need to introduce the Element Plus UI framework locally to develop this demo project. In addition, let's briefly understand how to configure Vite. When we used VueCLI3.x to create a project, the configuration project was configured under the vue.config.js file in the root directory. This file should export an object that contains options.
module.exports = { // Options }
vite.config.ts is similar.
export default { // configuration option }
Because Vite itself comes with Typescript type, you can make intelligent prompt through the cooperation of IDE and jsdoc. In addition, you can use the defineConfig helper function to get type prompt without jsdoc annotation. Here, we configure vite.config.ts as follows.
import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' // https://vitejs.dev/config/ export default defineConfig({ plugins: [vue()] })
You will find that Vue is introduced here as a built-in plug-in of vite. Just now, we said that we want to introduce the Element Plus UI framework locally. We open the Element Plus UI local introduction website:( https://element-plus.gitee.io/#/zh-CN/component/quickstart), it is found that babel.config.js needs to be configured here, and we use TS, so we subconsciously change the suffix and think it can succeed. However, I was cheated anyway. Therefore, this method is adopted: configure it in vite.config.ts file as follows:
import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' import vitePluginImp from "vite-plugin-imp"; // https://vitejs.dev/config/ export default defineConfig({ plugins: [vue(), vitePluginImp({ libList: [ { libName: 'element-plus', style: (name) => { return`element-plus/lib/theme-chalk/${name}.css` } } ] })], server: { port: 6061 }, })
Vite plugin imp is a vite plug-in that automatically imports component library styles.
List of main project folders Src
The following is the initial project file directory.
assets ---Static folder components ---Component folder App.vue ---pagefile main.ts ---Project entry file shims-vue.d.ts ---Type definition file (description file)
We won't show so many files one by one. We'll mainly look at App.vue, main.ts and ships-vue.d.ts.
App.vue
<template> <img alt="Vue logo" src="./assets/logo.png" /> <HelloWorld msg="Hello Vue 3 + TypeScript + Vite" /> </template> <script lang="ts"> import { defineComponent } from 'vue' import HelloWorld from './components/HelloWorld.vue' export default defineComponent({ name: 'App', components: { HelloWorld } }) </script> <style> #app { font-family: Avenir, Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; margin-top: 60px; } </style>
main.ts
import { createApp } from 'vue' import App from './App.vue' createApp(App).mount('#app')
shims-vue.d.ts
declare module '*.vue' { import { DefineComponent } from 'vue' const component: DefineComponent<{}, {}, any> export default component }
Here, we see defineComponent, a method of Vue3. Why use it here? To quote an official sentence:
In terms of implementation, defineComponent only returns the objects passed to it. However, in terms of type, the returned value has a constructor of composite type for manual rendering function, TSX and IDE tool support.
Introduce Vue router4
After reading the previous basic configuration, we are now ready to introduce Vue3's ecosystem.
Now when we install the Vue router version, the 3.x version is installed by default. Due to the great changes in the update of Vue 3, the Vue router will also release the latest version of 4.x for compatibility.
This is the official website of router4:
https://next.router.vuejs.org/
1. Installation
npm install vue-router@4
2. Configuration file
After installing the dependency, create a router folder under the project folder src, where you can create an index.ts file (because here we are a TS based project).
import { createRouter, createWebHashHistory, RouteRecordRaw } from "vue-router"; import Home from "../views/Home.vue"; const routes: Array<RouteRecordRaw> = [ { path: "/", name: "Home", component: Home }, { path: "/about", name: "About", component: () => import("../views/About.vue") } ]; const router = createRouter({ history: createWebHashHistory(), routes }); export default router;
In addition, you need to import it in the main.ts file and register it.
import { createApp } from "vue"; import App from "./App.vue"; import router from "./router"; createApp(App).use(router).mount("#app");
In order to test the effect, we create a views folder under the src folder, which creates two page files. About.vue and Home.vue, respectively.
home.vue
<template> <p class="txt">home</p> </template> <script lang="ts"> import { Options, Vue } from "vue-class-component"; @Options({ }) export default class Home extends Vue {} </script> <style scoped> .txt{ color: red; } </style>
about.vue
<template> <p>about</p> </template> <script> export default { name: "About" } </script>
Finally, you are in the App.vue file.
<template> <router-link to="/">Home</router-link> | <router-link to="/about">About</router-link> <router-view /> </template> <script lang="ts"> </script>
Thus, Vue router4 was successfully introduced.
Introducing css preprocessing language
Here, we introduce scss. Because we use vite as a build tool to build projects, we only need this.
npm install -D sass
We can see the official explanation:
Vite also provides built-in support for. SCSS,. Sass,. Less,. Style, and. stylus files. There is no need to install a specific vite plug-in for them, but the corresponding preprocessor dependency itself must be installed.
So you can use scss like this.
<template> <p class="txt">home</p> </template> <script lang="ts"> import { Options, Vue } from "vue-class-component"; @Options({ }) export default class Home extends Vue {} </script> <style scoped lang="scss"> .txt{ color: red; } </style>
Using Element Plus UI
We have successfully configured the locally introduced Element Plus framework above, so we can use it in this way.
<template> <p class="txt">home</p> <ElButton>Default button</ElButton> </template> <script lang="ts"> import { Options, Vue } from "vue-class-component"; import { ElButton } from 'element-plus' @Options({ components: { ElButton } }) export default class Home extends Vue {} </script> <style scoped lang="scss"> .txt{ color: red; } </style>
Here, you will find that Vue class component is introduced. What does it do?
Official website:
https://class-component.vuejs.org/
Vue Class Component is a library that lets you make your Vue components in class-style syntax.
Vue class component is a library that allows you to create Vue components in class style syntax.
For vue3, we use Vue Class Component v8, that is, version 8.
https://www.npmjs.com/package/vue-class-component/v/8.0.0-rc.1
You can install it like this.
npm i vue-class-component@8.0.0-rc.1
Introducing vue custom components
We often encapsulate components ourselves, so how did we introduce them in this project? We create a components folder in the src directory, where we create a HelloWorld.vue file.
HelloWorld.vue
<template> <h1>{{ msg }}</h1> </template> <script lang="ts"> import { ref, defineComponent } from 'vue' export default defineComponent({ name: 'HelloWorld', props: { msg: { type: String, required: true } }, setup: () => { const count = ref(0) return { count } } }) </script> <style scoped lang="scss"> a { color: #42b983; } label { margin: 0 0.5em; font-weight: bold; } code { background-color: #eee; padding: 2px 4px; border-radius: 4px; color: #304455; } </style>
Then we introduce it in App.vue.
<template> <HelloWorld msg="Hello Vue 3 + TypeScript + Vite" /> <router-link to="/">Home</router-link> | <router-link to="/about">About</router-link> <router-view /> </template> <script lang="ts"> import { defineComponent } from 'vue' import HelloWorld from './components/HelloWorld.vue' export default defineComponent({ name: 'App', components: { HelloWorld } }) </script> <style > #app { font-family: Avenir, Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; } </style>
Introduce vuex4
Vuex must be indispensable in Vue ecology. In order to be compatible with vue3, vuex has also launched version 4.0.
https://next.vuex.vuejs.org/
You can install it like this.
npm install vuex@next --save
You can create a store folder in the src folder, where you can create an index.ts file.
import { createStore } from "vuex"; export default createStore({ state: {}, mutations: {}, actions: {}, modules: {} });
Then, you use it in the main.ts file.
import { createApp } from "vue"; import App from "./App.vue"; import router from "./router"; import store from "./store"; createApp(App).use(router).use(store) .mount("#app");