When we use vue.js to write a single page application, packaged JavaScript packages can be very large, affecting page loading.
We all know the lazy loading of pictures.We don't load this picture when it doesn't appear in the viewport.So are we able to do that, lazy loading of routes?It is more efficient to load components when a route is used.
To achieve this, we need to combine the asynchronous component of Vue with the code splitting feature of webpack.
Here's how lazy loading of routes works and how it works.
First, let's create a project for a web pack template
vue init webpack my-project cd my-project npm install
Let's add a route and see how a single-page application loads js by default.
Let's first create a file New.vue at src/components/
<template> <div> <h1>vue-router Lazy load</h1> <h2>new doc</h2> </div> </template> <script> export default { } </script> <style> h1 { color: red; } </style>
Add two links to the App.vue file
<template> <div id="app">  <router-link to="/">Go to hello</router-link> <router-link to="/new">Go to new</router-link> <router-view></router-view> </div> </template>
Add Route to src/router/index.js File
import Vue from 'vue' import Router from 'vue-router' import Hello from 'components/Hello' import New from 'components/New' Vue.use(Router) export default new Router({ routes: [{ path: '/', name: 'Hello', component: Hello }, { path: '/new', name: 'New', component: New }] })
Now let's package the files
npm run build
After packaging, open the page (how can't open, please resolve by yourself).I have nginx configured so I can open it directly)
http://localhost/mall/dist/index.htm#/
Let's see what resources are loaded when you open a web page.
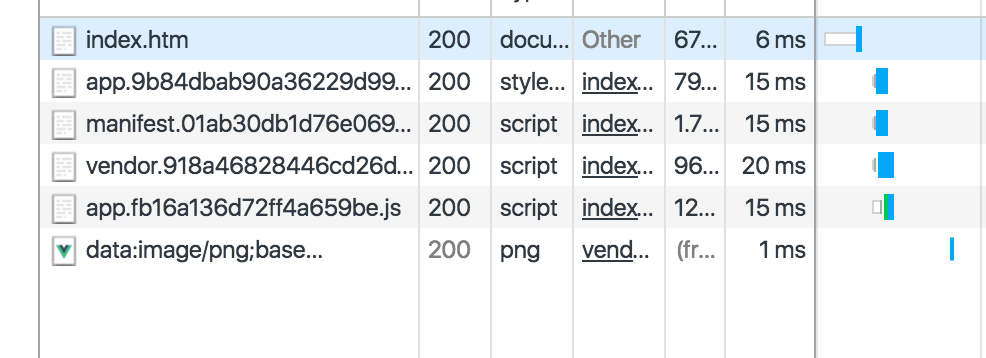
Now let's switch routes
http://localhost/mall/dist/index.htm#/new
We found that no new resources were loaded.
Because all js are loaded at once.If your application is large, it will open very slowly at this time.
Load lazily with routing to see how it works
Modify src/router/index.js file
import Vue from 'vue' import Router from 'vue-router' // import Hello from 'components/Hello' // import New from 'components/New' Vue.use(Router) // Define the component corresponding to the route as an asynchronous component const Hello = resolve => { require.ensure(['components/Hello.vue'], () => { resolve(require('components/Hello.vue')) }) } const New = resolve => { require.ensure(['components/New.vue'], () => { resolve(require('components/New.vue')) }) } export default new Router({ routes: [{ path: '/', name: 'Hello', component: Hello }, { path: '/new', name: 'New', component: New }] })
After successful packaging, open the page
http://localhost/mall/dist/index.htm#/
Look at the resources loaded at this time
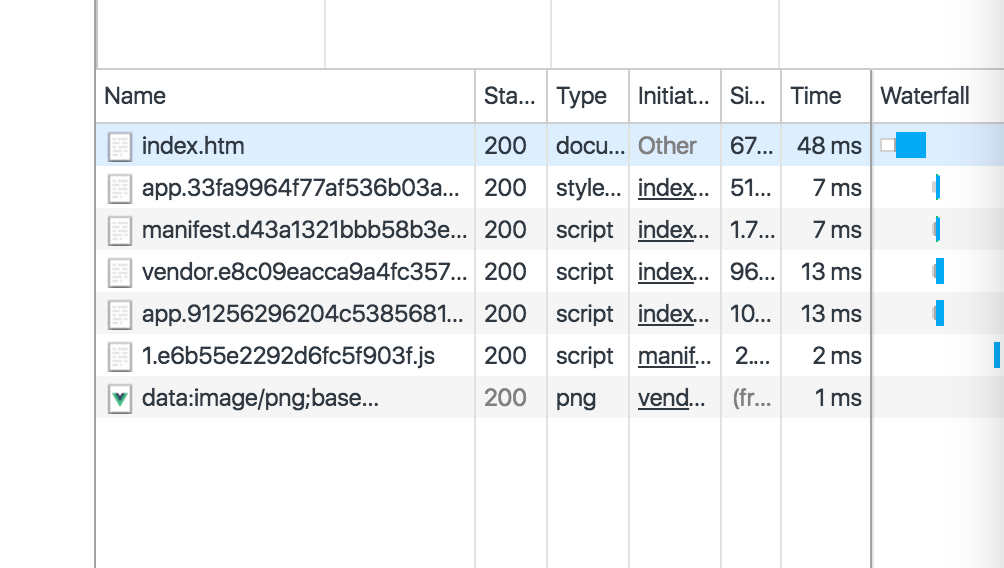
Compared with the last time, I found that the resource loaded at this time had one more js file.The contents of this js file contain all the contents of the Hello.vue file.
Let's switch routes
http://localhost/mall/dist/index.htm#/new
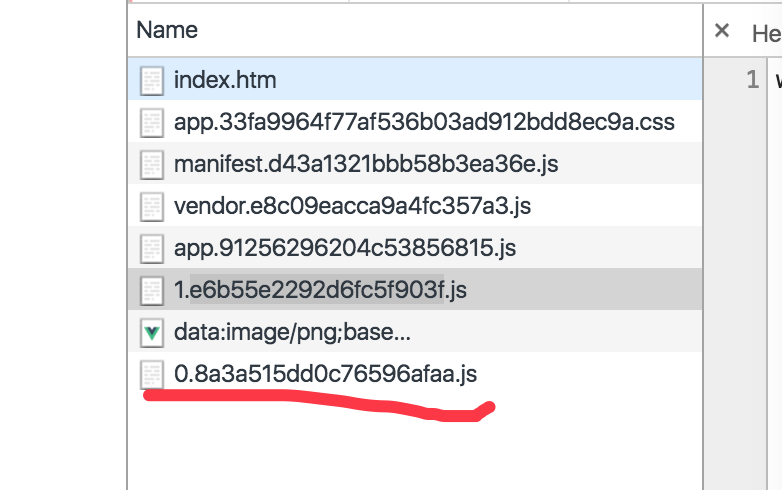
A new js file was found to be loaded.Open this js and find that it contains all the contents of the New.vue file.
Now, instead of loading all the js at once, we have implemented loading components on demand.You don't have to worry about loading all at once, which can delay the page from opening.
Modify New.vue file
<script> //Added reference to axios module import axios from 'axios' console.log(typeof axios, 11) export default { name: 'hello', data() { return { msg: 'Welcome to Your Vue.js App' } } } </script>
After packaging, open the file
http://localhost/mall/dist/index.htm#/new
You'll find that the js used to load the New component asynchronously are much larger because it contains axios code.
Okay, the implementation of routing lazy loading is that simple.See more Official Documents
Author: no_shower
Link: http://www.jianshu.com/p/97f72141bd89
Source: Short Book
Copyright belongs to the author.For commercial reprinting, please contact the author for authorization. For non-commercial reprinting, please indicate the source.