Under resources, create a new META-INF/dubbo/com.alibaba.dubbo.rpc.Filter file (which can be a txt suffix file)
Learn about @ Activate annotation in dubbo before rolling code
@Activate(group = {Constants.PROVIDER}, value = {"token"},order = -998)
- group refers to the common parameters of filter users: Constants.PROVIDER, Constants.CONSUMER
- Value indicates that the request URL contains the content. After the filter is activated to add value, the matching condition becomes more strict. This parameter must be in the URL and the parameter must have a value.
- Order is very simple. The smaller the order value, the higher the priority
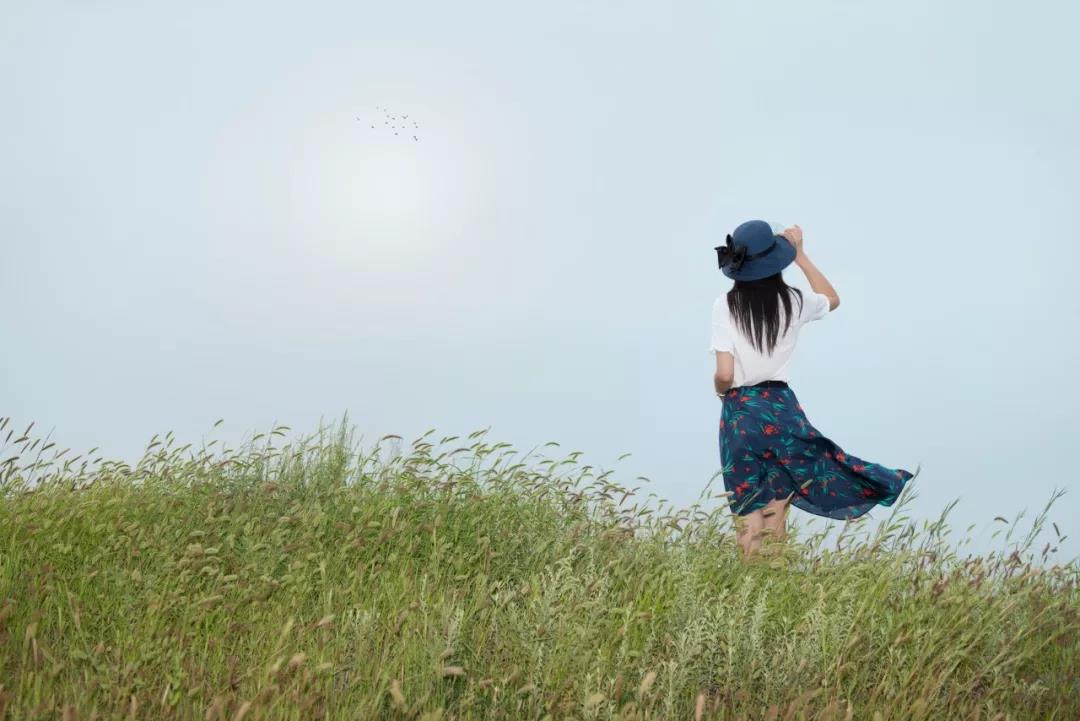
Take a rest with a beautiful picture
Let's code it.
- Building entity
@Data public class DubboLogBean { private long elaspedTime; private String fromAddress; private String toAddress; private String qualifiedName; private String version; private String group; private String args; }
- Building dubbo filters
/** * @Title: * @Auther: hangyu * @Date: 2019/3/4 * @Description * @Version:1.0 */ public abstract class DubboLogFilter implements Filter { public abstract Logger getLogger(); protected DubboLogBean buildDubboLogBean(Invoker invoker, Invocation invocation, String args, long elaspedTime) { DubboLogBean logBean = new DubboLogBean(); RpcContext context = RpcContext.getContext(); logBean.setFromAddress(context.getLocalAddressString()); logBean.setToAddress(context.getRemoteAddressString()); String qualifiedName = invoker.getInterface() + "#" + invocation.getMethodName(); logBean.setQualifiedName(qualifiedName); logBean.setArgs(args); logBean.setVersion(invoker.getUrl().getParameter(Constants.VERSION_KEY)); logBean.setGroup(invoker.getUrl().getParameter(Constants.GROUP_KEY)); logBean.setElaspedTime(elaspedTime); return logBean; } @Override public Result invoke(Invoker invoker, Invocation invocation) throws RpcException { // Start execution time long startTime = System.currentTimeMillis(); Result result = invoker.invoke(invocation); long elaspedTime = System.currentTimeMillis() - startTime; Object[] objs = invocation.getArguments(); StringBuilder sb = new StringBuilder(); for (Object obj : objs) { sb.append(obj).append("|"); } String args = sb.toString().substring(0, sb.length() > 0 ? sb.length() - 1 : 0); DubboLogBean inLogBean = buildDubboLogBean(invoker, invocation, args, elaspedTime); getLogger().info("Participation request: " + JSON.toJSONString(inLogBean)); DubboLogBean outLogBean = buildDubboLogBean(invoker, invocation, result.toString(), elaspedTime); getLogger().info("Ginseng production response: " + JSON.toJSONString(outLogBean)); return result; } }
- Service provider activation method
/** * @Title: * @Auther: hangyu * @Date: 2019/3/4 * @Description * @Version:1.0 */ @Activate(group = {Constants.PROVIDER},order = -998) public class DubboProviderLogFilter extends DubboLogFilter { private static final String DUBBO_PROVIDER_LOG = "dubbo.provider"; private final static Logger LOGGER_PROVIDER = LoggerFactory.getLogger(DUBBO_PROVIDER_LOG); @Override public Logger getLogger() { return LOGGER_PROVIDER; } }
- Service consumer activation method
/** * @Title: * @Auther: hangyu * @Date: 2019/3/4 * @Description * @Version:1.0 */ @Activate(group = {Constants.CONSUMER}, order = -999) public class DubboConsumerLogFilter extends DubboLogFilter { private static final String DUBBO_CONSUMER_LOG = "dubbo.consumer"; private final static Logger LOGGER_CONSUMER = LoggerFactory.getLogger(DUBBO_CONSUMER_LOG); @Override public Logger getLogger() { return LOGGER_CONSUMER; } }
- Identify the filter in the com.alibaba.dubbo.rpc.Filter file
dubboProviderLog=com.bbkmobile.iqoo.vivoshop.core.filter.DubboProviderLogFilter
dubboConsumerLog=com.bbkmobile.iqoo.vivoshop.core.filter.DubboConsumerLogFilter
Start service, call dubbo interface
Viewing console log printing