I. Writing on the front
Before using the WebDriver framework, I used two other automated testing frameworks, IBM Rational Robot and TestComplete, which are powerful payment tools for automated testing of Web, mobile and desktop applications, compared to WebDrIver, they are automated. For script developers, there are not enough "open" (extensible) and "free" (encapsulable), more or less there are some limitations, of course, this does not mean that there are no limitations of WebDriver, for GUI desktop application interface WebDriver is currently at a loss, must use other auxiliary tools, but this does not prevent me. I like WebDriver because it can cover more than 95% of the pages for automated testing on the Web side, and on the basis of it, I can quickly write an automated testing framework that conforms to my project.
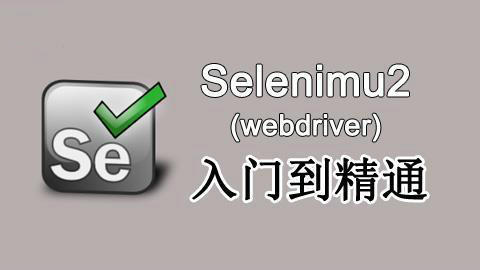
II. Preparations
Before we begin the automated testing of our own project, we'd better have completed the following preparations:
1. Familiarity with the system to be tested
Have a clear understanding of the overall function and business logic of the system to be tested.
2. Writing syllabus of automated test cases for the system
This step mainly lets us divide the parts of the system that can implement automated testing according to priority before coding.
3. Choosing appropriate tools and frameworks
For Web Driver, we can choose to be based on Java or python, browser based on chrome or firefox, Web Driver version, browser version, use case management to choose TestNG or Junit and so on. Here we use selenium-java 2.53.1/firefox 45/TestNG 6.8.8.
III. Implementation steps
1 > Create the parent BaseCase class of the test case:
Each test case class inherits from the BaseCase class, so that the common parts of the use case can be implemented in the BaseCase class, thus simplifying the code structure and reducing code redundancy, such as:
a. Use TestNG framework to manage use cases and implement @BeforeSuite,@BeforeClass,@BeforeTest,@BeforeMethod and its corresponding After method in BaseCase class.
b. Some commonly used methods related to use cases;
c. Common variables, etc.
public class BaseCase { public static String url = ""; public static String username = ""; public static String password = ""; public static WebDriver driver; @BeforeSuite public void initSuite(){ //Initialize the entire project, such as configuration data synchronization } @BeforeClass public void initTest(){ //Initialize test classes, such as opening browsers driver = new FirefoxDriver(); } @AfterClass public void close(){ //Close browsers and other operations, of course, you can also put in @AfterTest or @AfterMethod driver.close(); } ......
2 > TestAction class for creating use cases:
TestAction class mainly encapsulates some interface actions, such as click, input, move, refresh, and so on. User actions related to the interface can be encapsulated in this class.
public class TestAction { private WebDriver driver; public TestAction(WebDriver driver){ this.driver = driver; } public void refresh(){ driver.navigate().refresh(); Log.info("F5 Refresh"); sleep(1); } public void moveToElement(WebElement e){ if(e != null){ Actions action = new Actions(driver); Log.info("Mouse to:" + getText(e)); action.moveToElement(e).perform();//Mouse over the element }else{ Log.error("No object found"); } } public void click(WebElement e){ if(e != null){ Log.info("Click on the object:" + getText(e)); e.click(); }else{ Log.error("No object found"); } } public void setText(WebElement element, Object content, boolean isPrintLog){ if(element != null){ element.clear(); element.sendKeys(String.valueOf(content)); if(isPrintLog){ assertEquals(getText(element), content+"", "input"); } }else{ Log.error("Textbox element not found"); } } }
3 > Handler class encapsulating common basic controls:
This step is not a problem in TestAction, but it is also possible to encapsulate the operations of some common basic controls separately (see personal habits), such as TextHandler for text control, TableHandler for table operation, Date PickerHandler for date selection control, etc. Examples of ndler classes are as follows:
public class DatePickerHandler { public static WebElement getDatePicker(){ By by = By.xpath("//*[@class='mz-datepicker']/input"); return BaseCase.isElementExist(by) ? Page.driver.findElement(by) : null; } public static List<WebElement> getDateLinks(){ By by = By.xpath("//*[@class='mz-calendar-top']/a"); return BaseCase.isElementsExist(by) ? Page.driver.findElements(by) : null; } public static String setDate(String text){ TestAction.click(getDatePicker(), 0.5); if(getDateLinks()==null || getDateLinks().size() < 1){ return ""; } for(WebElement link : getDateLinks()){ if(BaseCase.getText(link).equals(text)){ TestAction.click(link, 0.2); break; } } Log.info("Select the date range:" + BaseCase.getText(getDatePicker())); return BaseCase.getText(getDatePicker()); } public static String setDateText(String dateRange){ ((JavascriptExecutor)Page.driver).executeScript("arguments[0].removeAttribute(\"readOnly\");",getDatePicker()); getDatePicker().clear(); getDatePicker().sendKeys(dateRange); if(! dateRange.equals(BaseCase.getText(getDatePicker()))){ Log.writeInfo("Failed to select the date range. Actually:"+ BaseCase.getText(getDatePicker()) +",Expect:" + dateRange); return ""; } Log.writeInfo("Select the date range:" + BaseCase.getText(getDatePicker())); return BaseCase.getText(getDatePicker()); } }
4 > Element Object Management:
BaseCase and operation classes have been encapsulated before, but how should the element objects of the page be managed? This may depend on the size of the project and the number of elements. Here are some common ways:
a. Write the id, name or xpath of the element location directly in the code;
b. The expression of element location is extracted and stored in text, XML, yaml or json.
c. Extract the expression of element location and store it in database.
Writing element positioning in code has obvious advantages. It's easy to debug, but it's not recommended for tens of thousands of page elements. Element objects will become difficult to manage (it's estimated that there will be a lot of useless objects left in the code), but it's recommended when there are few page elements. Here's PageO. The way bject writes element positioning in code, for example:
public class HomePage { private WebDriver driver; public HomePage (WebDriver driver){ this.driver = driver; } public WebElement getLeftNavHome(){ By by = By.xpath("//a[text()='home page'] "; return BaseCase.isElementExist(by) ? driver.findElement(by) : null; } public WebElement getUser(){ By by = By.xpath("//*[@class='name']/b"); return BaseCase.isElementExist(by) ? driver.findElement(by) : null; } }
5 > Use Case Writing:
Create test case classes according to the page. According to the page function points, one or more @Test can be written in the class, and the granularity of the use case can be controlled by itself.
public class TestHomePage extends BaseCase { private HomePage homePage; @Test public void testHeaderUsername(){ if(isLogged){ TestAction.refresh(); homePage = new HomePage(); if(assertEquals(getText(homePage.getUser()), user_name, "User name")){ TestAction.moveToElement(homePage.getUser()); TestAction.sleep(0.5); if(homePage.getQuit().isDisplayed()){ TestAction.click(homePage.getQuit(), 0.5); if(driver.getTitle().contains("Sign in") || driver.getTitle().contains("Login")){ Log.writeInfo("Successful exit and landing"); }else{ Log.writeErrorInfo("Exit login failure"); } } } } } @Test public void testXXXXX1(){......} @Test public void testXXXXX2(){......} ......
6 > Use Case Management:
Using TestNG's xml file to manage use cases
<suite name="WebAutomationTest" parallel="tests" thread-count="1"> <listeners> <listener class-name="your Listener" /> </listeners> <test name="test case" preserve-order="true"> <classes> <class name="com.alany.testcase.TestHomePage"></class> //Use case classes continue to be added later </classes> </test> </suite>
So far, this automated testing framework based on WebDriver+TestNG framework has been built up. With the increase of project use cases and skills, you can further optimize and adjust...
PS: For more original technical articles and information, please pay attention to the following public numbers:
The "Test Development Stack" public number is jointly managed and operated by veterans with many years of experience in testing and development, aiming at sharing original testing and developing related technologies, including but not limited to:
Testing direction: Web automation testing, mobile automation testing, Web service testing, interface testing, etc.
Development direction: Java development, Android development, front-end development, etc.
We hope that our experience and technology sharing will enable you to grow and progress every day and become a technology bull as soon as possible.~
Welcome to share and forward our articles (share and forward please retain the source of the articles), so that more friends can pay attention to us, but also welcome to join our QQ group exchanges and questions: 427020613
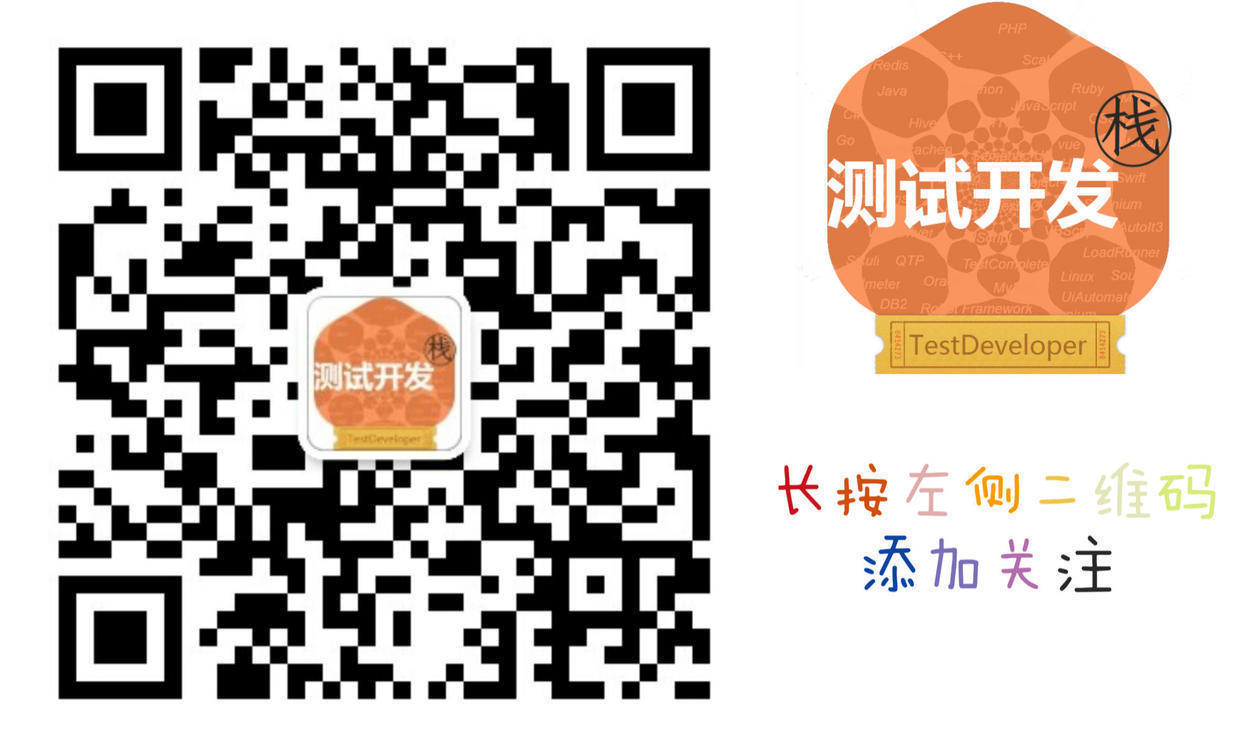