How does python + selenium + chrome operate the scroll bar
Keywords:
Selenium
Python
Pycharm
How does python + selenium + chrome operate the scroll bar
1. background
- When using selenium to simulate browser, you often need to pull down scroll bar, generally for two purposes:
- Anthropomorphic operation, breaking through the sophisticated anti climbing system.
- Some elements don't load out normally. You need to drag the drop-down bar to load out slowly. It's similar to Taobao.
2. environment
- python 3.6.1
- System: win7
- IDE: pycharm
- Installed chrome browser
- Configure the chrome driver
- selenium 3.7.0
3. Operation method
3.1. Drag to the specified element location.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import Select
import time
import random
chrome_options = webdriver.ChromeOptions()
extension_path = 'D:/extension/XPath-Helper_v2.0.2.crx'
chrome_options.add_extension(extension_path)
browser = webdriver.Chrome(chrome_options=chrome_options)
wait = WebDriverWait(browser, 25)
waitPopWindow = WebDriverWait(browser, 25)
browser.get("https://www.amazon.com/s/ref=nb_sb_noss_2?url=search-alias%3Daps&field-keywords=phone")
time.sleep(random.randrange(5, 10, 1))
targetElem = browser.find_element_by_xpath("//a[@id='pagnNextLink']")
browser.execute_script("arguments[0].focus();", targetElem)
print(f"End drag scroll bar....")
time.sleep(random.randrange(5, 10, 1))
browser.quit()
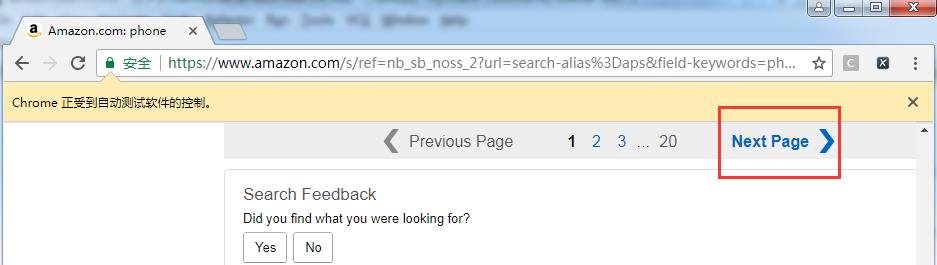
3.2. Specify the pull-down distance
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import Select
import time
import random
chrome_options = webdriver.ChromeOptions()
extension_path = 'D:/extension/XPath-Helper_v2.0.2.crx'
chrome_options.add_extension(extension_path)
browser = webdriver.Chrome(chrome_options=chrome_options)
browser.maximize_window()
wait = WebDriverWait(browser, 25)
waitPopWindow = WebDriverWait(browser, 25)
browser.get("https://www.amazon.com/s/ref=nb_sb_noss_2?url=search-alias%3Daps&field-keywords=phone")
time.sleep(random.randrange(5, 10, 1))
jsCode = "var q=document.documentElement.scrollTop=100000"
browser.execute_script(jsCode)
print("Drag slider to bottom...")
time.sleep(random.randrange(5, 10, 1))
browser.quit()
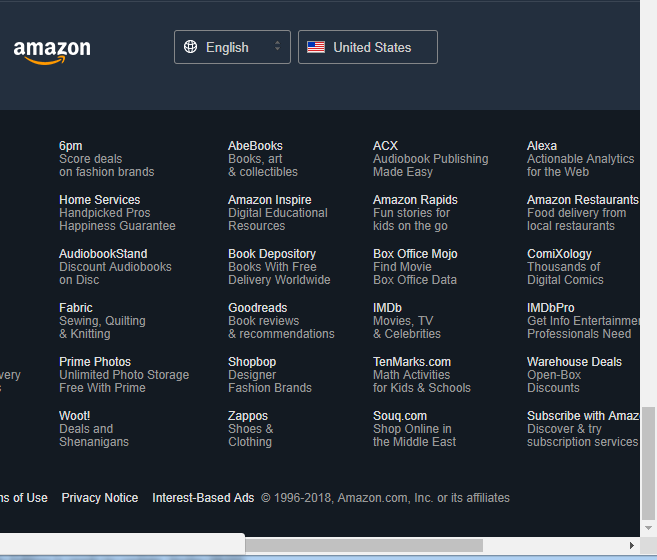
3.3. Send tab key, move to target element
- You can send the tab key to switch the page buttons, so as to pull down the scroll bar. But it must be noted that the specified elements must be able to be selected by tab key, such as input box, hyperlink, Button, etc.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import Select
import time
import random
chrome_options = webdriver.ChromeOptions()
extension_path = 'D:/extension/XPath-Helper_v2.0.2.crx'
chrome_options.add_extension(extension_path)
browser = webdriver.Chrome(chrome_options=chrome_options)
browser.maximize_window()
wait = WebDriverWait(browser, 25)
waitPopWindow = WebDriverWait(browser, 25)
browser.get("https://www.amazon.com/s/ref=nb_sb_noss_2?url=search-alias%3Daps&field-keywords=phone")
time.sleep(random.randrange(5, 10, 1))
targetElem = browser.find_element_by_xpath("//a[@id='pagnNextLink']")
targetElem.send_keys(Keys.TAB)
print(f"End drag scroll bar....")
time.sleep(random.randrange(5, 10, 1))
browser.quit()
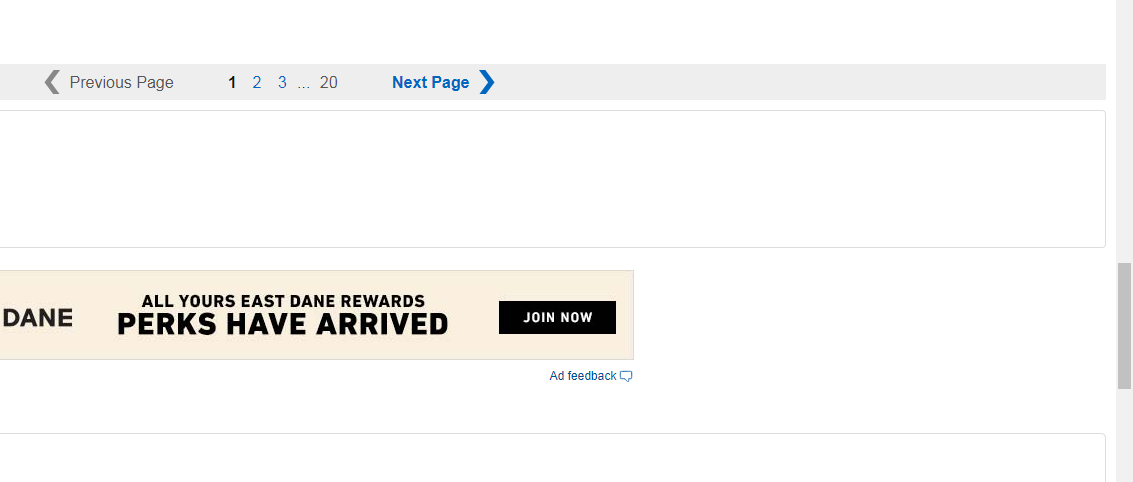
Posted by jbatty on Wed, 15 Apr 2020 08:38:05 -0700