1, Object navigation query
Previous relationships between customers and contacts were one to many: One to many
Scenario: query the customer according to the customer id, and find out all contacts of the customer
@Test public void selectTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Find out the customer according to C ﹣ ID Customer customer = session.get(Customer.class,1); //Then find out the contact person of the customer Set<Salesperson> set = customer.getSalespersonSet(); Iterator<Salesperson> it = set.iterator(); while (it.hasNext()) { Salesperson str = it.next(); System.out.println(str.getS_name()); } transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
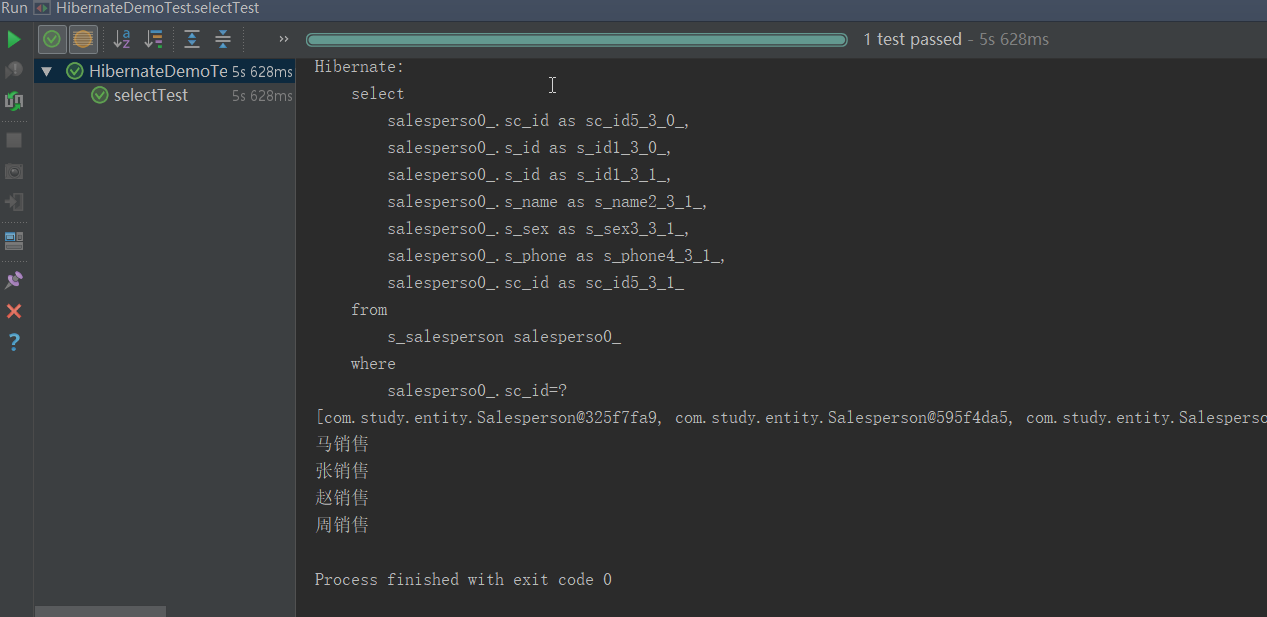
2, HQL query
- Query all customer information
- Create Query object and write out hql statement
- Call the method in the query object to get the result
- Query all from + entity class names
@Test public void selectTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Create query object Query query = session.createQuery("from Customer"); //Call method to get result List<Customer> list = query.list(); for (Customer customer: list) { System.out.println(customer.getC_id()+"-"+customer.getC_name()); } transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
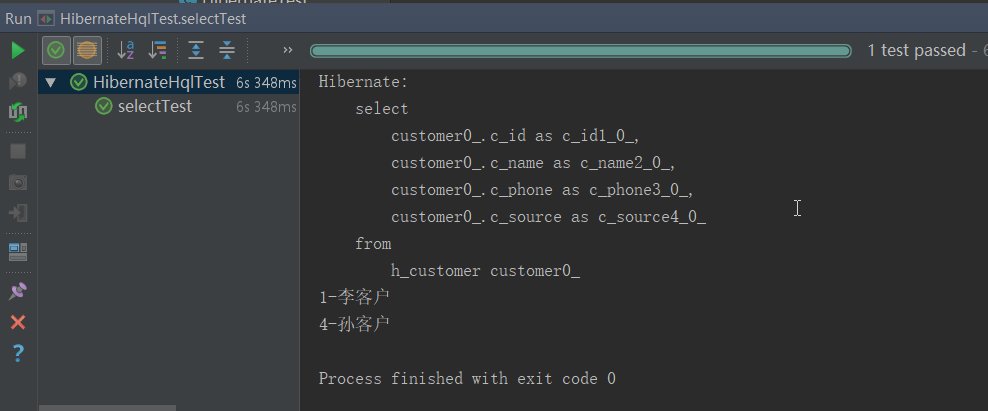
- Condition query
- Statement: from entity class name where entity class property =? and entity class name
@Test public void selectByCidAndCnameTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Create query object Query query = session.createQuery("from Customer where c_id = ? and c_name = ?"); query.setParameter(0,1); query.setParameter(1,"Li customer"); //Call method to get result List<Customer> list = query.list(); for (Customer customer: list) { System.out.println(customer.getC_id()+"-"+customer.getC_name()); } transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
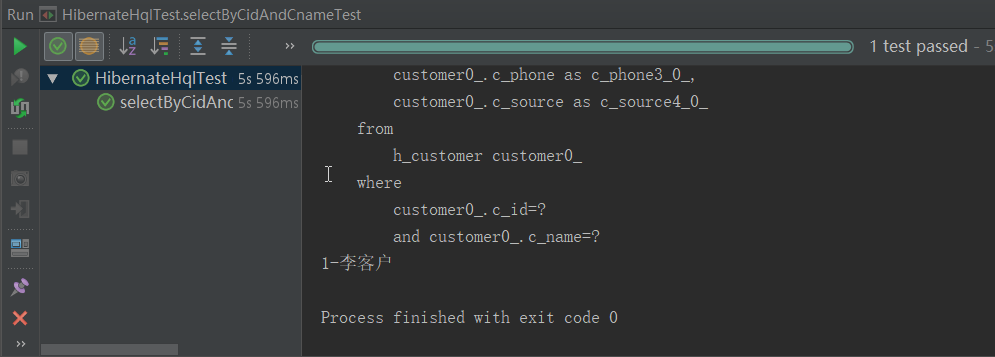
Condition query
- Sort query
- Statement: from entity class name order by entity class name asc/desc
@Test public void selectOrderByTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Create query object Query query = session.createQuery("from Customer order by c_id desc "); //Call method to get result List<Customer> list = query.list(); for (Customer customer: list) { System.out.println(customer.getC_id()+"-"+customer.getC_name()); } transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
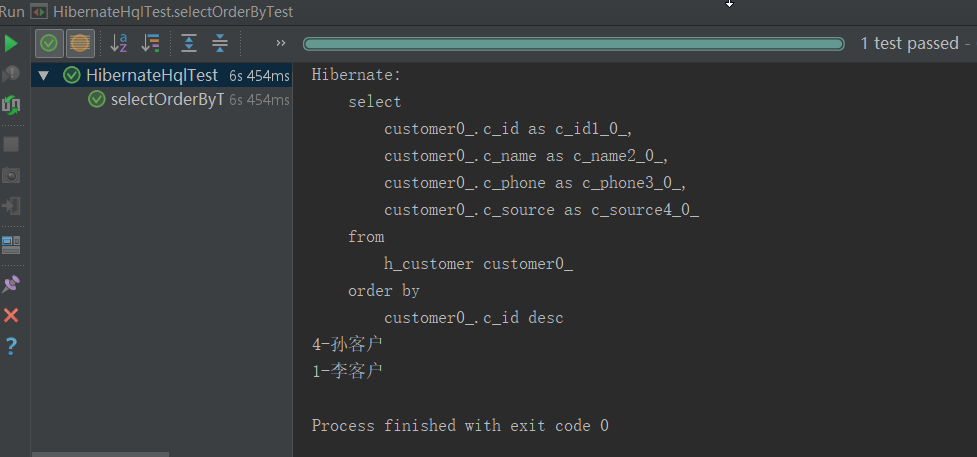
- Paging query
@Test public void selectLimitTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Create query object Query query = session.createQuery("from Customer"); //Set paging data query.setFirstResult(0); query.setMaxResults(2); //Call method to get result List<Customer> list = query.list(); for (Customer customer: list) { System.out.println(customer.getC_id()+"-"+customer.getC_name()); } transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
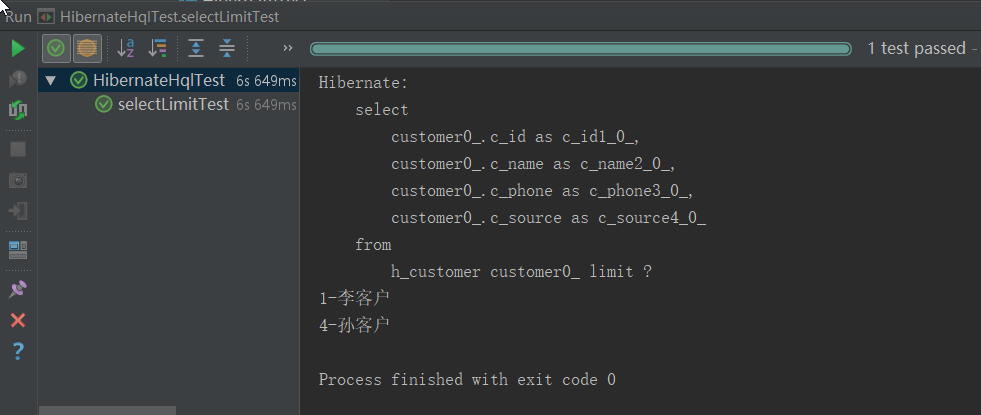
- Projection query
- Statement: select * from entity class attribute name 1, entity class attribute name 2, from entity class name
- Cannot write * after select, not supported
@Test public void selectSomeTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Create query object Query query = session.createQuery("select c_name from Customer"); //Call method to get result List<Object> lists = query.list(); for (Object list: lists) { System.out.println(list); } transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
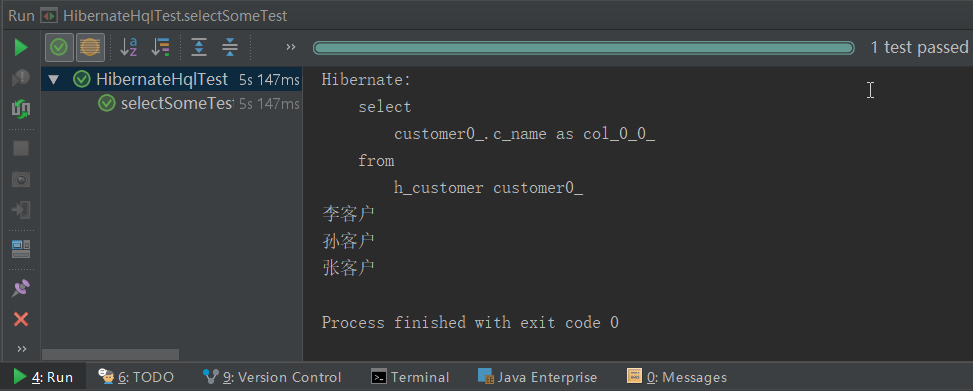
image.png
- Aggregate function use
- count ,sum , avg , mac , min
- Statement: select count (*) from entity class name
@Test public void selectHanshuTest(){ SessionFactory sessionFactory = null; Session session = null; Transaction transaction = null; try{ sessionFactory = HibernateUtils.getSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); //Create query object Query query = session.createQuery("select count(*) from Customer"); //Call method to get result Object count = query.uniqueResult(); //Convert to long, then int Long co = Long.parseLong(count.toString()); System.out.println(co.intValue()); transaction.commit(); }catch (Exception e){ transaction.rollback(); e.printStackTrace(); }finally{ session.close(); sessionFactory.close(); } }
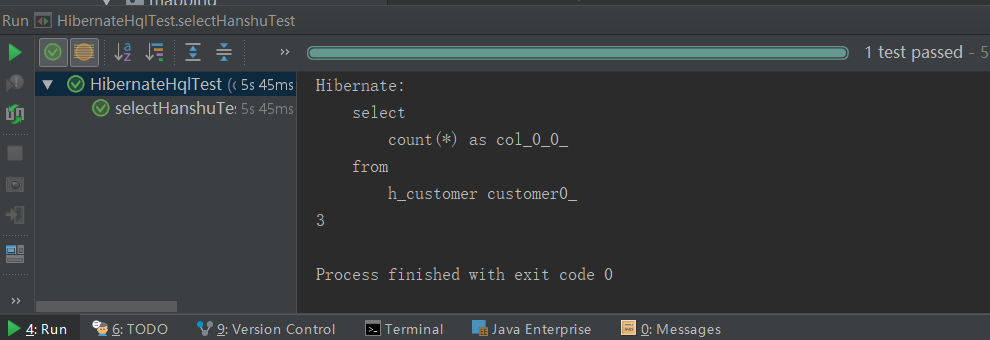