Code for this chapter: https://github.com/MarsOu1998/ForeignKeyHibernate
1. Create connections between PO s
In Hibernate framework, PO object relational layer is registered by mapping file and configuration file, and some operations on database can be completed by using Hibernate API support. So can PO and PO create links and collaborate with each other? The answer is yes.
In databases, there are three kinds of relationships between tables: one-to-one, one-to-many and many-to-many. So PO s can also do this association.
2. One-to-one association: one-to-one
Topic: School and principal is a one-to-one relationship, which requires the realization of: when inquiring the school information, the principal's information can be obtained without additional inquiries.
Steps:
- Create POJO: School.java,Header.java, create Hibernate configuration file, bind two hbm files to it.
- Create a Header type property in Scholl. java.
- Added to Scholl's hbm file
<one-to-one name="header Property name" class="header Attribute class" cascade="all|none(Cascade or not)/>
Assuming that the primary key of the school table in the database is school ID, and the primary key in the header table is headerId, when using Hibernate to query the school, the principal's information can't pop up.
Query1.java:
import PO.School; import org.hibernate.Session; public class Query1 { public static void main(String[] args) { Session session=util.HibernateSessionFactory.getSession(); School school=(School)session.get(School.class,1); System.out.println(school.getSchoolName()); System.out.println(school.getHeader()); util.HibernateSessionFactory.closeSession(); } }
The output will find that the principal's name is null.
The reasons are as follows:
The system does not look for schoolId=schoolId, but for schoolId=headerId, so it cannot find it.
The reason is that in the header mapping file, the primary key is headerId, which can be solved by changing the primary key to schoolId.
Query.java:
package Test; import PO.School; import org.hibernate.Session; public class Query1 { public static void main(String[] args) { Session session=util.HibernateSessionFactory.getSession(); School school=(School)session.get(School.class,1); System.out.println(school.getSchoolName()); System.out.println(school.getHeader().getHeaderName()); util.HibernateSessionFactory.closeSession(); } }
Header.hbm.xml:
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="PO.Header" table="header"> <id name="schoolId" column="schoolId" > <generator class="assigned"></generator> </id> <property name="headerName" column="headerName" ></property> <property name="headerId" column="headerId"></property> </class> </hibernate-mapping>
Student.hbm.xml
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="PO.School" table="school"> <id name="schoolId" column="schoolId" > <generator class="assigned"></generator> </id> <property name="schoolName" column="schoolName" ></property> <one-to-one name="header" class="PO.Header" cascade="all"></one-to-one> </class> </hibernate-mapping>
Student.java:
package PO; public class School {//If you want to know the principal, you need to set the principal's attributes here. private int schoolId; private String schoolName; private Header header; public int getSchoolId() { return schoolId; } public void setSchoolId(int schoolId) { this.schoolId = schoolId; } public String getSchoolName() { return schoolName; } public void setSchoolName(String schoolName) { this.schoolName = schoolName; } public Header getHeader() { return header; } public void setHeader(Header header) { this.header = header; } }
Header.java:
package PO;
public class Header {
private int headerId,schoolId;
private String headerName;
public int getHeaderId() { return headerId; } public void setHeaderId(int headerId) { this.headerId = headerId; } public int getSchoolId() { return schoolId; } public void setSchoolId(int schoolId) { this.schoolId = schoolId; } public String getHeaderName() { return headerName; } public void setHeaderName(String headerName) { this.headerName = headerName; }
}
//The results are as follows: 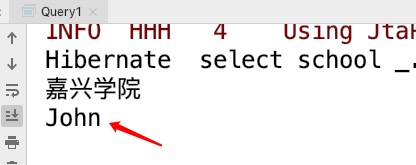 //Similarly, the principal's information can also be modified: Update.java: ~~~java package Test; import PO.School; import org.hibernate.Session; import org.hibernate.Transaction; public class Update { public static void main(String[] args) { Session session=util.HibernateSessionFactory.getSession(); School school=(School)session.get(School.class,3); school.getHeader().setHeaderName("Oshile"); Transaction transaction=session.beginTransaction(); session.update(school); transaction.commit(); util.HibernateSessionFactory.closeSession(); } }
Similarly, information can also be deleted, and the contents of the two tables will be deleted together.
Delete.java
package Test; import PO.School; import org.hibernate.Session; import org.hibernate.Transaction; public class Delete { public static void main(String[] args) { Session session=util.HibernateSessionFactory.getSession(); School school=(School)session.get(School.class,2); session.delete(school); Transaction transaction=session.beginTransaction(); transaction.commit(); util.HibernateSessionFactory.closeSession(); } }
Similarly, you can add:
Insert.java:
package Test; import PO.Header; import PO.School; import org.hibernate.Session; import org.hibernate.Transaction; public class Insert { public static void main(String[] args) { Session session=util.HibernateSessionFactory.getSession(); School school=new School(); school.setSchoolId(4); school.setSchoolName("Tongji University"); Header header=new Header(); header.setHeaderName("Zhang Hua"); header.setHeaderId(7); header.setSchoolId(4); school.setHeader(header); Transaction transaction=session.beginTransaction(); session.save(school); transaction.commit(); util.HibernateSessionFactory.closeSession(); } }
Data information is inserted into two tables, which is a one-to-one relationship.