1. Review of the relationship between tables (emphasis)
1.1. One-to-many
(1) Classification and commodity relationship. There are many commodities in a classification. A commodity can only belong to one classification.
(2) One-to-many relationship between customers and contacts
- Customer: With the company business contacts, Baidu, Sina, 360
- Contacts: employees in the company, there are many employees in Baidu, contact employees
** The relationship between the company and its employees
- One customer, many contacts
- There are many contacts in a customer. A contact can only belong to one customer.
(3) One-to-many table building: establishing relationships through foreign keys
1.2. Many-to-many
(1) Order and commodity relationship. There are more than one commodity in an order, and one commodity belongs to more than one order.
(2) Many-to-many relationships between users and roles
- Users: Xiaowang, Xiaoma, Xiaosong
- Roles: General Manager, Secretary, Driver, Security
For example, Xiao Wang can be a general manager or a driver.
** For example, Xiao Song can be a driver, a secretary and a security guard.
** For example, a pony can be a secretary or a general manager.
- There can be multiple roles in a user and multiple users in a role.
(3) Multi-to-multi table building: creating a third table maintenance relationship
1.3. One-to-one
(1) In China, a man can only have one wife and a woman can only have one husband.
2. One-to-many operations of Hibernate (emphasis)
2.1. One-to-many mapping configuration (emphasis)
Take customers and contacts for example: customers are one, contacts are many
2.1.1. The first step is to create two entity classes, customers and contacts.
2.1.2. Step 2 lets two entity classes represent each other
(1) Represent multiple contacts in the client entity class
- There are multiple contacts in a customer
(2) Represent the customer in the contact entity class
- A contact can only belong to one customer
2.1.3. The third step is to configure mapping relationships
(1) A general entity class corresponds to a mapping file
(2) Complete the basic configuration of mapping
(3) Configure one-to-many relationships in mapping files
- In the customer mapping file, all contacts are represented
<! - The relationship between tables and tables - > <! - Represents all contacts in the customer mapping file Use the set tag to represent all contacts There is a name attribute in the set tag: Property values are written in the client entity class to represent the set collection name of the contact Default value of inverse attribute: false does not abandon relationship maintenance true denotes abandonment of relationship maintenance --> <set name="setLinkMan"table="t_linkman" inverse="false"lazy="true"> <! - One-to-many table building, with foreign keys hibernate mechanism: two-way maintenance of foreign keys, one or more sides are configured with foreign keys column attribute value: foreign key name --> <key> <column name="CID"/> </key> <! - All the contacts of the customer, the full path of the contact entity class is written in the class - >. <one-to-many class="hibernate.entity.LinkMan"/> </set>
- In the contact mapping file, represent the customer to which you belong
<! - The relationship between tables and tables - > <! - Indicates the customer to which the contact belongs. Name attribute: because the contact entity class is represented by the customer object and writes the customer name class attribute: customer full path column attribute: foreign key name --> <many-to-one name="customer"class="hibernate.entity.Customer" fetch="join"> <column name="CID"/> </many-to-one>
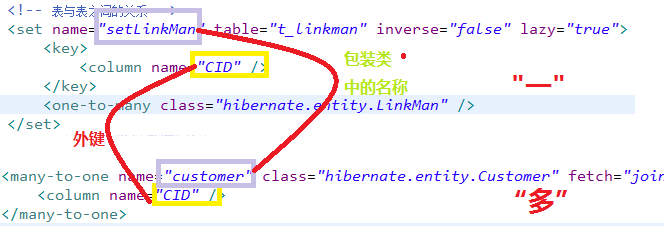
2.1.4. Step 4: Create the core configuration file and introduce the mapping file into the core configuration file
Test:
2.2. One-to-many cascade operation
cascade
1 cascade storage
(1) Add a customer, add multiple contacts for the customer
2 cascade deletion
(1) Delete a customer, and all the contacts in that customer are deleted.
2.2.1. One-to-many cascade storage
1 Add a customer, add a contact for this customer
(1) Complex writing:
//One-to-many cascade storage @Test public void testAddDemo1(){ SessionFactorysessionFactory = null; Sessionsession = null; Transactiontx = null; try { sessionFactory= HibernateUtils.getSessionFactory(); session= sessionFactory.openSession(); tx= session.beginTransaction(); // Add a customer, add a contact for that customer //1 Create customer and contact objects Customercustomer = newCustomer(); customer.setCustName("Panzhihua"); customer.setCustLevel("vip"); customer.setCustSource("network"); customer.setCustPhone("110"); customer.setCustMobile("999"); LinkManlinkman = newLinkMan(); linkman.setLkm_name("lucy"); linkman.setLkm_gender("male"); linkman.setLkm_phone("911"); //2 Represent all contacts at the customer and customers at the contact // Establishing the relationship between customer and contact objects //2.1 Put the contact object in the set set set of the client object customer.getSetLinkMan().add(linkman); //2.2 Put customer objects in contacts linkman.setCustomer(customer); //3 Save to database session.save(customer); session.save(linkman); tx.commit(); }catch(Exception e) { tx.rollback(); }finally { session.close(); sessionFactory.close(); } }

(2) Simplified Writing
- Generally add contacts based on customers
The first step is to configure in the client mapping file
- Configure the set tag in the client mapping file
The second step is to create the customer and contact object, just put the contact in the customer, and ultimately just save the customer.
//One-to-many cascade storage @Test public void testAddDemo2(){ SessionFactorysessionFactory = null; Sessionsession = null; Transactiontx = null; try { sessionFactory= HibernateUtils.getSessionFactory(); session= sessionFactory.openSession(); tx= session.beginTransaction(); // Add a customer, add a contact for that customer //1 Create customer and contact objects Customercustomer = newCustomer(); customer.setCustName("Baidu"); customer.setCustLevel("Member"); customer.setCustSource("People love you"); customer.setCustPhone("110121"); customer.setCustMobile("999458"); LinkManlinkman = newLinkMan(); linkman.setLkm_name("Old orchid"); linkman.setLkm_gender("female"); linkman.setLkm_phone("120"); //2. Put the contact object in the set set set of the client object customer.getSetLinkMan().add(linkman); //3 Save to database session.save(customer); tx.commit(); }catch(Exception e) { tx.rollback(); }finally { session.close(); sessionFactory.close(); } }
Add multiple contact set sets
2.2.2. One-to-many cascade deletion
1 Delete a customer, delete all contacts in the customer
2. Realization
The first step is to configure the set tag of the client mapping file
(1) Use attribute cascade attribute value delete
The second step is to delete the customer directly from the code
(1) according to id query object, call delete method in session to delete
3. Implementation process:
(1) Query customers according to id
(2) Query contacts based on foreign key id values
(3) Set the contact foreign key to null
(4) Delete contacts and customers
2.2.3. One-to-many modification operation (inverse attribute)
1. Let lucy contact belong to Baidu instead of Panzhihua.
2 inverse attribute
(1) Because hibernate maintains foreign keys in both directions, both customers and contacts need to maintain foreign keys. When modifying customers, it modifies foreign keys once, and when modifying contacts, it modifies foreign keys once, which causes efficiency problems.
(2) Solution: Let one of them not maintain foreign keys
One-to-many inside, let one of them give up foreign key maintenance, let one give up
There is a president in a country, and there are many people in the country. The President can't know all the people in the country, and all the people in the country can know the president.
(3) Specific realization:
In the abandoned relational maintenance mapping file, configure and use the inverse attribute on the set tag
3. Hibernate multi-to-multi operation
3.1. Multi-to-multi mapping configuration
Demonstrate with examples of users and roles
Step 1: Create Entity Classes, Users and Roles
3.1.2. Step 2 lets two entity classes represent each other
(1) All roles are represented in a user, using set sets
(2) A role has multiple users, using set sets
3.1.3. The third step is to configure mapping relationships
(1) Basic configuration
(2) Configuring many-to-many relationships
- Represent all roles in the user, using the set tag
- Represent all users in roles, using the set tag
,
3.1.4. Step 4: Introduce a mapping file into the core configuration file
Test:
3.2. Multi-to-Multi Cascade Operation
3.2.1. Multi-pair and multi-cascade storage
Save roles according to users
The first step is to configure the set tag in the user profile with the cascade value save-update
Step 2 Coding Implementation
(1) Create users and role objects, put roles in users, and finally save users.
//Cascaded multi-to-many storage @Test public void testSave(){ SessionFactorysessionFactory = null; Sessionsession = null; Transactiontx = null; try { sessionFactory= HibernateUtils.getSessionFactory(); session= sessionFactory.openSession(); tx= session.beginTransaction(); //Add 2 users and 2 roles for 2 users //1 Create Objects Useruser1 = newUser(); user1.setUser_name("Tom"); user1.setUser_password("123"); Useruser2 = newUser(); user2.setUser_name("Jerry"); user2.setUser_password("456"); Rolerole1 = newRole(); role1.setRole_name("General manager"); role1.setRole_memo("General manager"); Rolerole2 = newRole(); role2.setRole_name("secretary"); role2.setRole_memo("secretary"); Rolerole3 = newRole(); role3.setRole_name("Security staff"); role3.setRole_memo("Security staff"); //2. Establishing relationships and putting roles in users // user1 -- r1/r2 user1.getSetRole().add(role1); user1.getSetRole().add(role2); // user2 -- r2/r3 user2.getSetRole().add(role2); user2.getSetRole().add(role3); //3 Save Users session.save(user1); session.save(user2); tx.commit(); }catch(Exception e) { tx.rollback(); }finally{ session.close(); sessionFactory.close(); } }
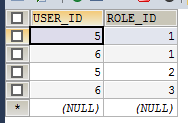
3.2.2. Multi-cascade deletion (understanding) -- basically useless
The first step is to configure the set tag with the cascade value delete
Step 2 Delete Users
3.3. Maintain the third table relationship
Users and roles have many-to-many relationships. Maintenance relationships are maintained through the third table
3.3.1. Give a user a role
Let lucy play the role of a broker
The first step is to query users and roles based on id
Step 2: Place roles in users
Place role objects in user set sets
3.3.2. Let a user not have a role
Let Tom lose his security role
The first step is to query users and roles based on id
The second step is to remove the role from the user
Remove roles from set sets
Source code file: http://download.csdn.net/detail/qq_26553781/9776244