1. Create a project with the name hibernatedemo25 and the directory structure as shown in the figure.
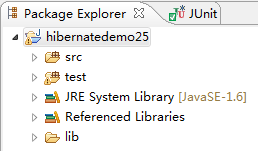
2. Create a lib directory in the project to store jar files. The directory structure is shown in the figure.
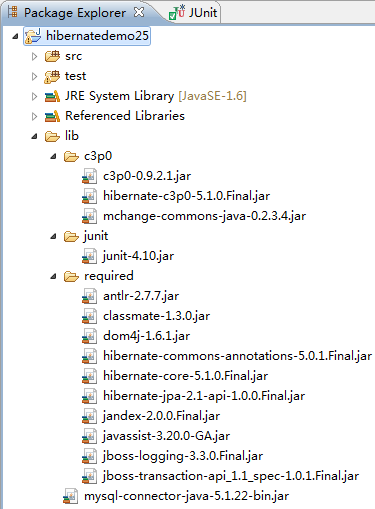
3. Create the entity class Forum, package name (com.mycompany.demo.bean) in the src directory, as shown in the figure
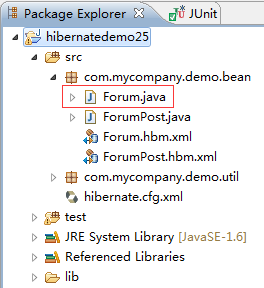
4. The content of the entity class Forum is as follows
package com.mycompany.demo.bean;
import java.util.Set;
public class Forum {
private int fid;
private String name;
private Set<ForumPost> forumPosts;
public int getFid() {
return fid;
}
public void setFid(int fid) {
this.fid = fid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set<ForumPost> getForumPosts() {
return forumPosts;
}
public void setForumPosts(Set<ForumPost> forumPosts) {
this.forumPosts = forumPosts;
}
}
5. Create the mapping file Forum.hbm.xml, package name (com.mycompany.demo.bean) of the entity class Forum in the src directory, as shown in the figure.
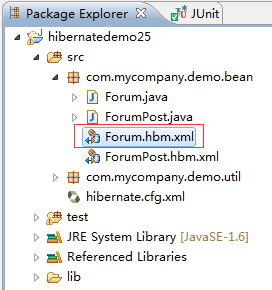
6. The mapping file Forum.hbm.xml is as follows
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<!--
package:Appoint<class/>The package
-->
<hibernate-mapping package="com.mycompany.demo.bean">
<!--
name:Class name
table:Table name
catalog:Database name,Default is hibernate.cfg.xml The name of the database configured in
-->
<class name="Forum" table="forum">
<meta attribute="class-description">
This class contains the forum detail.
</meta>
<!--
name:Attribute name
colum:Column names
-->
<id name="fid" type="int" column="fid">
<!--
increment:hibernate Maintaining primary key values
identity:Database self-growth
sequence:sequence
native:Select generation strategies based on different databases
uuid:adopt UUID Algorithm generation,More practical use
assigned:Manual setting
-->
<generator class="native"/>
</id>
<!--
length:Byte length
type:Field type,Support java and hibernate type
not-null:Non empty constraint
unique:Uniqueness constraints
-->
<property name="name" column="name" />
<!--
lazy:
false:Direct loading
true:Delayed loading
extra:Special Delayed Loading
fetch
join:Urgent Left External Connection
select:ordinary select query
subselect :Subquery mode
-->
<set name="forumPosts" cascade="all" fetch="select" lazy="extra">
<key column="fid"/>
<one-to-many class="ForumPost"/>
</set>
</class>
</hibernate-mapping>
7. Create the entity class ForumPost, package name (com.mycompany.demo.bean) in the src directory, as shown in the figure
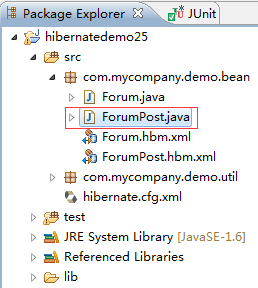
8. The content of the entity class ForumPost is as follows
package com.mycompany.demo.bean;
public class ForumPost {
private int pid;
private String subject;
private Forum forum;
public int getPid() {
return pid;
}
public void setPid(int pid) {
this.pid = pid;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public Forum getForum() {
return forum;
}
public void setForum(Forum forum) {
this.forum = forum;
}
}
9. Create the mapping file ForumPost.hbm.xml and package name (com.mycompany.demo.bean) of the entity class ForumPost in the src directory, as shown in the figure.
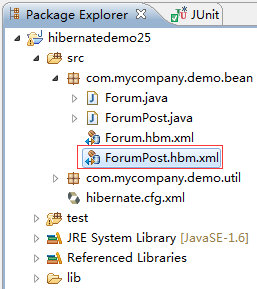
10. The mapping file ForumPost.hbm.xml is as follows
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<!--
package:Appoint<class/>The package
-->
<hibernate-mapping package="com.mycompany.demo.bean">
<!--
name:Class name
table:Table name
catalog:Database name,Default is hibernate.cfg.xml The name of the database configured in
-->
<class name="ForumPost" table="forumpost">
<meta attribute="class-description">
This class contains the forumpost detail.
</meta>
<!--
name:Attribute name
colum:Column names
-->
<id name="pid" type="int" column="pid">
<!--
increment:hibernate Maintaining primary key values
identity:Database self-growth
sequence:sequence
native:Select generation strategies based on different databases
uuid:adopt UUID Algorithm generation,More practical use
assigned:Manual setting
-->
<generator class="native"/>
</id>
<!--
length:Byte length
type:Field type,Support java and hibernate type
not-null:Non empty constraint
unique:Uniqueness constraints
-->
<property name="subject" column="subject" type="string"
length="50" not-null="true" unique="false"/>
<!--
name:Association attribute
column:The fields corresponding to the associated attributes in the database
class:Types corresponding to associated attributes
-->
<many-to-one name="forum" class="Forum" column="fid"/>
</class>
<query name="queryAll">from Forum f left outer join fetch f.forumPosts</query>
</hibernate-mapping>
11. Create the tool class HbnUtil in the src directory with the package name (com.mycompany.demo.util), as shown in the figure.
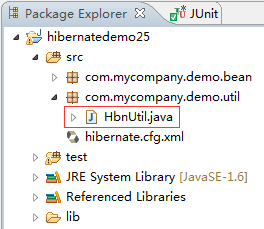
12. The tool class HbnUtil is as follows
package com.mycompany.demo.util;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HbnUtil {
private static SessionFactory sessionFactory;
public static Session getSession(){
if(sessionFactory == null || sessionFactory.isClosed()){
sessionFactory = new Configuration().configure().buildSessionFactory();
}
return sessionFactory.getCurrentSession();
}
}
13. Create Hibernate configuration file hibernate.cfg.xml in the src directory, as shown in the figure
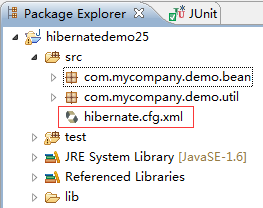
14.Hibernate's configuration file, hibernate.cfg.xml, reads as follows
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration SYSTEM
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- dialect,Can from Hibernate core jar(hibernate-core-x.x.x.Finall.jar)
Document or.hibernate.dialect Find the corresponding class in the package,The full name of a class is -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- drive -->
<property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property>
<!-- Database Connection Address -->
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/test</property>
<!-- User name -->
<property name="hibernate.connection.username">
root
</property>
<!-- Password -->
<property name="hibernate.connection.password"></property>
<!--
create:Every time it's created,Delete if it exists
create-drop:Create new table,sessionFactory Close,Table delete
update :Table field addition,Synchronization,Field Reduction Asynchronism,Data changes are synchronized
-->
<property name="hibernate.hbm2ddl.auto">update</property>
<!-- output sql -->
<property name="hibernate.show_sql">true</property>
<!-- Format sql -->
<property name="hibernate.format_sql">true</property>
<!-- Transaction environment A thread to a transaction
thread:Local transaction environment
jta:Distributed Transaction Environment
SpringSessionContext:Be used for ssh integration
-->
<property name="hibernate.current_session_context_class">thread</property>
<!-- Use c3p0 data source -->
<property name="hibernate.connection.provider_class">
org.hibernate.c3p0.internal.C3P0ConnectionProvider</property>
<!-- List of XML mapping files -->
<mapping resource="com/mycompany/demo/bean/Forum.hbm.xml"/>
<mapping resource="com/mycompany/demo/bean/ForumPost.hbm.xml"/>
</session-factory>
</hibernate-configuration>
15. Create a test directory in the project to store test files, the file name TestApp, the package name (com.mycompany.demo.bean), and the directory structure is shown in the figure.
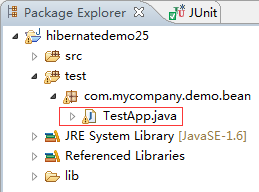
16. The TestApp test class is as follows
package com.mycompany.demo.bean;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.hibernate.Criteria;
import org.hibernate.Session;
import org.hibernate.sql.JoinType;
import org.junit.Before;
import org.junit.Test;
import com.mycompany.demo.util.HbnUtil;
public class TestApp {
private Session session;
@Before
public void init(){
session = HbnUtil.getSession();
}
/*
* One-to-many bidirectional association-add
* Set property in Forum.hbm.xml needs to be set to cascade="all"
*/
@Test
public void testOneToManyAdd(){
try {
session.beginTransaction();
ForumPost forumPost1 = new ForumPost();
forumPost1.setSubject("A");
ForumPost forumPost2 = new ForumPost();
forumPost2.setSubject("B");
Set<ForumPost> forumPosts = new HashSet<ForumPost>();
forumPosts.add(forumPost1);
forumPosts.add(forumPost2);
Forum forum = new Forum();
forum.setName("foruma");
forum.setForumPosts(forumPosts);
session.save(forum);
session.getTransaction().commit();
} catch (Exception e) {
session.getTransaction().rollback();
e.printStackTrace();
}
}
/*
* One-to-many bidirectional association-fetch="select",lazy="extra"
*/
@Test
public void testFetchForSelect(){
try {
session.beginTransaction();
Forum forum = session.get(Forum.class, 10);
Set<ForumPost> forumPosts = forum.getForumPosts();
System.out.println(forumPosts.size());
for (ForumPost forumPost : forumPosts) {
System.out.println(forumPost.getSubject());
}
session.getTransaction().commit();
} catch (Exception e) {
session.getTransaction().rollback();
e.printStackTrace();
}
}
}
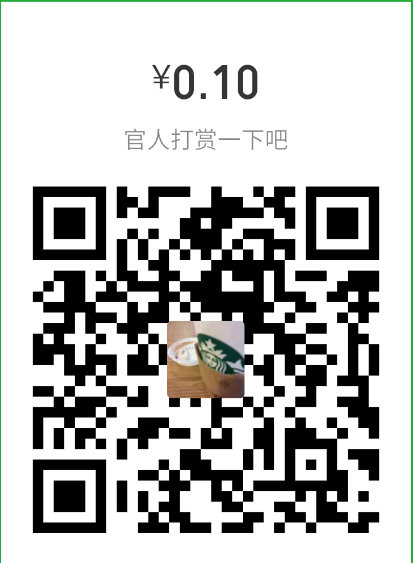