Resources
stm32_usb_cubemx.md
hal Software Architecture Principle of usb of Yan Gang's stm32
I. usb Foundation
The usb of stm32 is also the interface that many companies are using. The usb can reach 12M/s at full speed. As a virtual serial interface, it's still good. The whole protocol of usb is very complex. I have sorted out the usb usage in the next few years, explained the usb software architecture, and some important knowledge points.
2. demo Engineering of cubemx Generating usb Virtual Serial Port
Download Warehouse:
git clone https://github.com/yangang123/usb_vcp_demo.git
Step 2.1:
- The selection board is stm32f4 discovery
- Selection of external crystal oscillator
- Setting the system clock is 168MHz
- Setting USB as device
- The class that sets up the slave device of USB is vcp
- Setting the stack is 0x600 [Note]
- Set Reset
- View Port
- View the results
1. Selection board is stm32f4 discovery
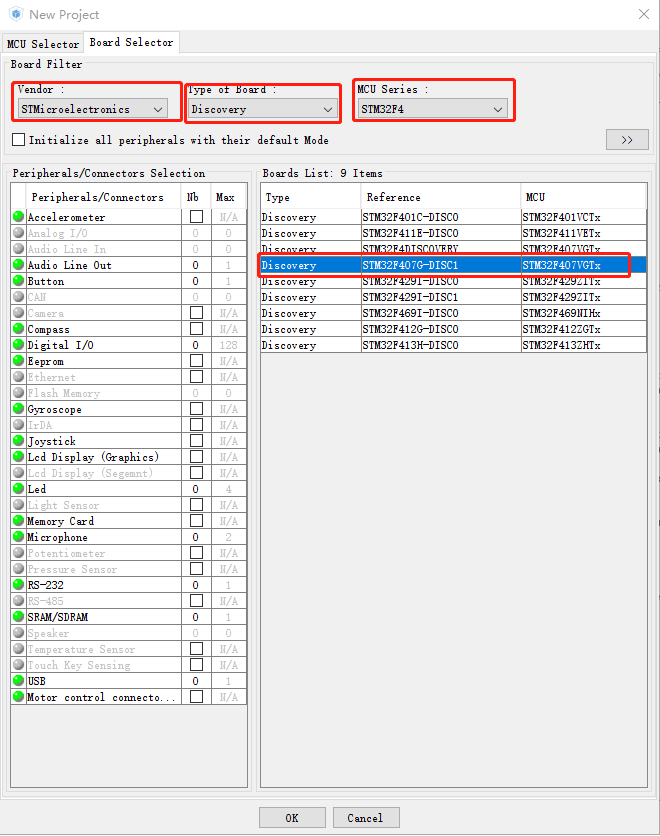
2. Selection of external crystal oscillators
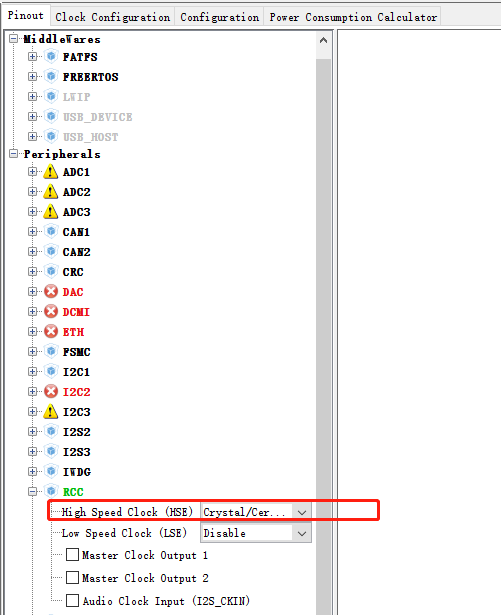
3. Setting the system clock is 168MHz
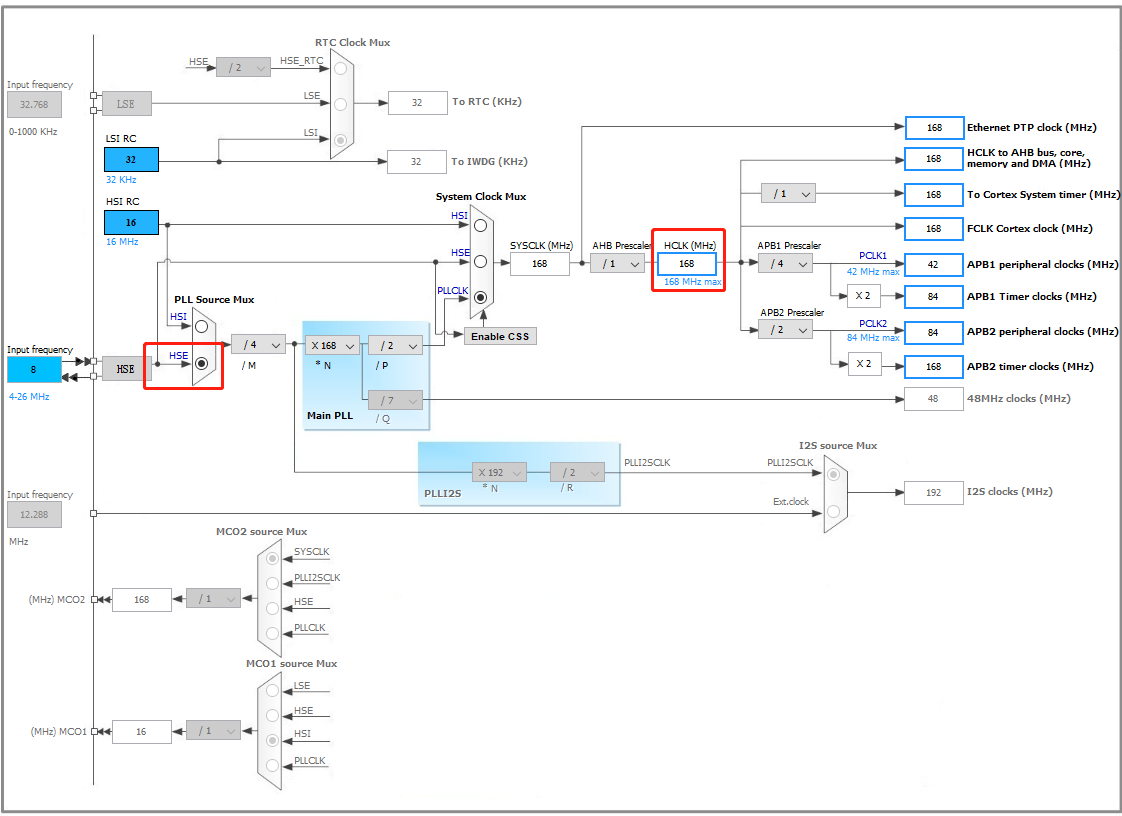
4. Setting USB as device
5. The class of setting USB slave device is vcp
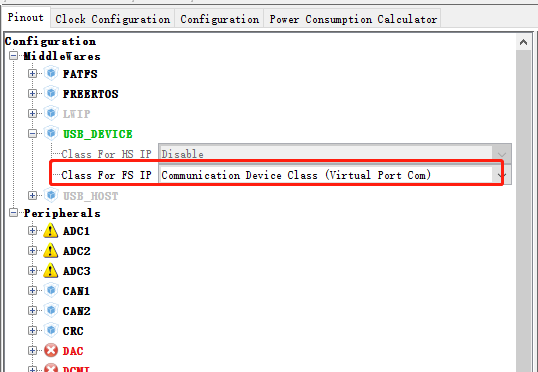
6. Set the stack to 0x600
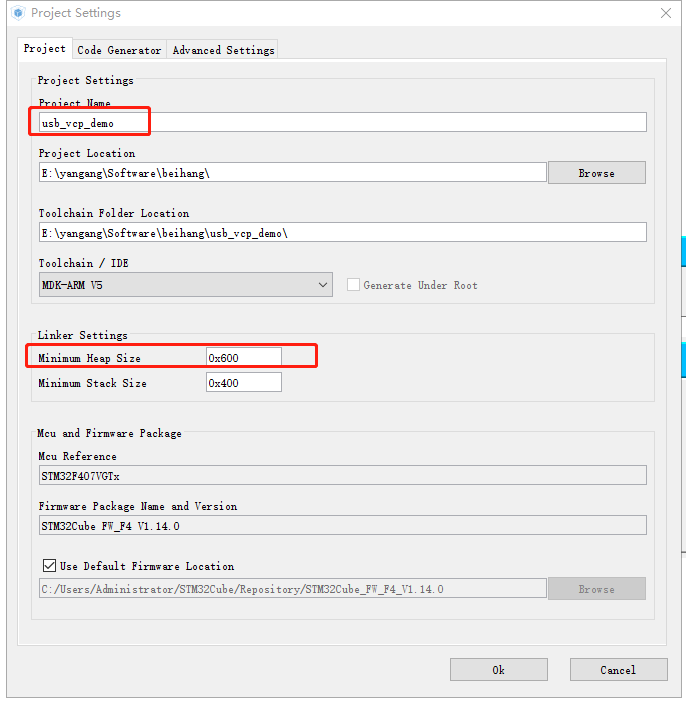
7. Setting Reset
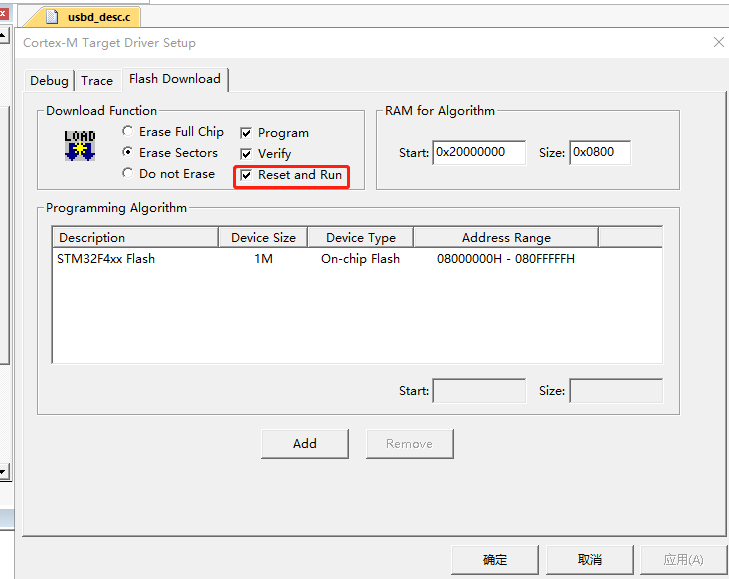
8. View ports
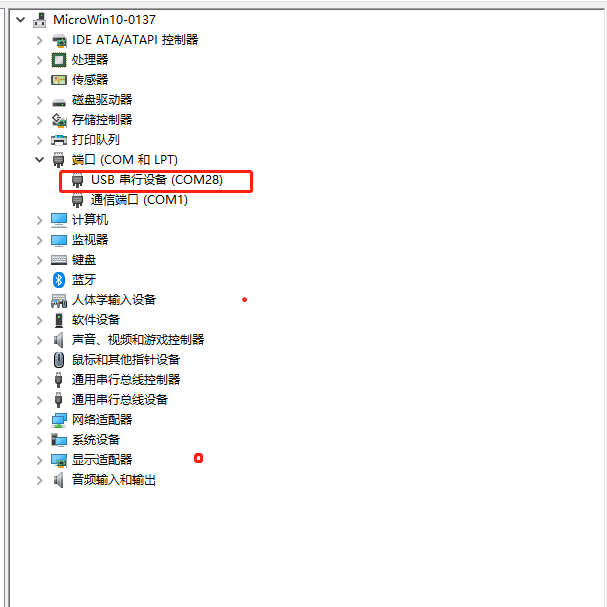
9. View the results
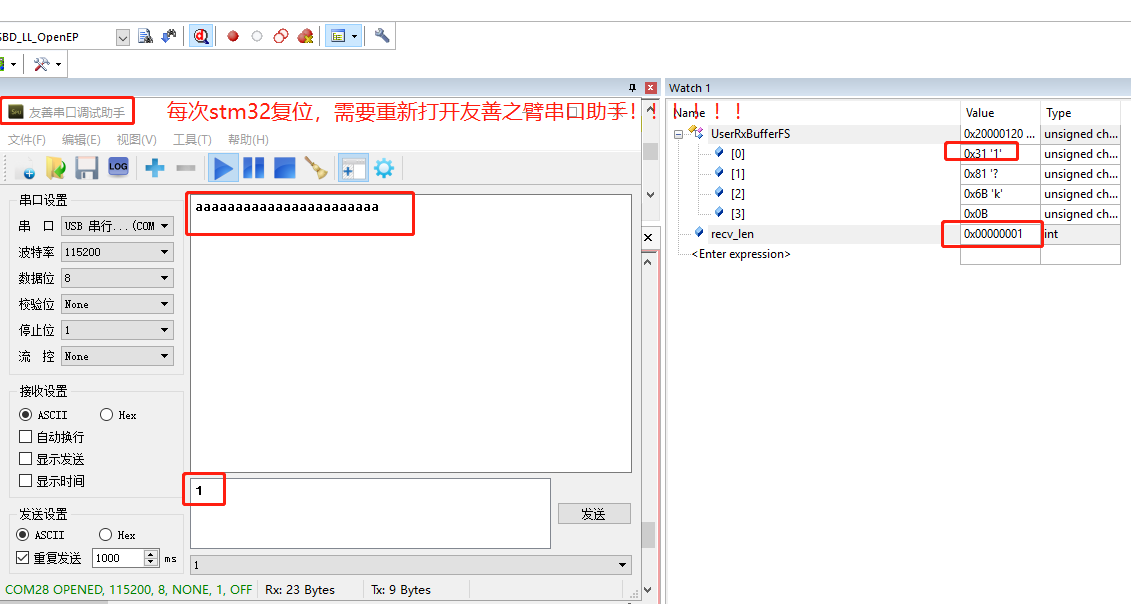
2.2 Testing:
PC : WIN10
Software: Friendly Arm Serial Port Assistant
Keil: MDK5
Veneer: stm32f4 discory
2.3 Testing process:
- KEIL to set up after downloading, automatic reset
- Check which COM port is on the computer
- The program sends one character'a'per second.
- The serial assistant sends 1 character'a'per second
The entire project has been submitted to github
Address:
How to deal with sending and receiving
Both the receiving and sending interfaces are in usbd_cdc_if.c.
3.1 Data buffer size configuration is as follows
#define APP_RX_DATA_SIZE 4 #define APP_TX_DATA_SIZE 4
3.2 Send Data
To send data, use the following api
uint8_t CDC_Transmit_FS(uint8_t* Buf, uint16_t Len)
Here is the 1s cycle for sending "a" data
HAL_Delay(1000); char *string = "a"; CDC_Transmit_FS((uint8_t*)string, 1);
3.3 Receiving Data
The receiving buffer uses uint8_t UserRxBufferFS[APP_RX_DATA_SIZE];
The length of received data int recv_len = 0;
The interface for receiving data is static int8_t CDC_Receive_FS (uint8_t*Buf, uint32_t*Len), which receives data.
static int8_t CDC_Receive_FS (uint8_t* Buf, uint32_t *Len) { recv_len = *Len; USBD_CDC_SetRxBuffer(&hUsbDeviceFS, &Buf[0]); USBD_CDC_ReceivePacket(&hUsbDeviceFS); return (USBD_OK); }
IV. Principles of Sending and Receiving
arrangement | function | Main documents |
---|---|---|
APP | application layer | usbd_cdc_if.c |
calss | Different devices, different data | usb_cdc.c |
core | Processing of USB Data Buffer | usb_core.c |
HAL | Hardware interrupt processing | stm32f4_ll_usb.c |
4.1 Send Data
USB_EPStartXfer: Start an Endpoint Transfer
USBx_INEP: Enabling EP
USB_WritePacket: Data Writing to fifo
CDC_Transmit_FS((uint8_t*)string, 15); -> USBD_CDC_TransmitPacket(&hUsbDeviceFS); -> USBD_LL_Transmit(pdev, CDC_IN_EP, hcdc->TxBuffer,hcdc->TxLength); -> hal_status = HAL_PCD_EP_Transmit(pdev->pData, ep_addr, pbuf, size); -> HAL_StatusTypeDef USB_EPStartXfer(USB_OTG_GlobalTypeDef *USBx , USB_OTG_EPTypeDef *ep, uint8_t dma) ->USBx_INEP(ep->num)->DIEPCTL |= (USB_OTG_DIEPCTL_CNAK | USB_OTG_DIEPCTL_EPENA); ->USB_WritePacket(USBx, ep->xfer_buff, ep->num, ep->xfer_len, dma);
4.2 Receiving Data
HAL_PCD_IRQHandler(&hpcd_USB_OTG_FS); -> HAL_PCD_DataOutStageCallback(hpcd, epnum); -> USBD_LL_DataOutStage((USBD_HandleTypeDef*)hpcd->pData, epnum, hpcd->OUT_ep[epnum].xfer_buff); -> pdev->pClass->DataOut(pdev, epnum); -> ((USBD_CDC_ItfTypeDef *)pdev->pUserData)->Receive(hcdc->RxBuffer, &hcdc->RxLength);
4.2.1 Interface DataOut
Interface Architecture
typedef struct _Dev ice_cb{ uint8_t (*DataOut)(struct _USBD_HandleTypeDef *pdev , uint8_t epnum);
Register the DataOut interface
USBD_ClassTypeDef USBD_CDC = { USBD_CDC_DataOut,
4.2.2 Interface Receive
Interface Architecture
typedef struct _USBD_CDC_Itf { int8_t (* Init) (void); int8_t (* DeInit) (void); int8_t (* Control) (uint8_t, uint8_t * , uint16_t); int8_t (* Receive) (uint8_t *, uint32_t *); }USBD_CDC_ItfTypeDef;
Registration (* Receive) This interface function
USBD_CDC_ItfTypeDef USBD_Interface_fops_FS = { CDC_Init_FS, CDC_DeInit_FS, CDC_Control_FS, CDC_Receive_FS };