From: http://www.jianshu.com/p/4986100eff90
On Green Dao
greenDao is a lightweight and fast ORM solution that maps objects to SQLite databases.
The concept of green DAO can be seen on the official website greenDAO
Green DAO Advantages
1. A streamlined Library
2. Maximizing performance
3. Minimizing memory overhead
4. Easy to use APIs
5. Highly Optimize Android
GreenDao 3.0
GreenDao 3.0 uses annotations to define entity classes and generates corresponding code through gradle plug-ins.
First, import related packages in as
compile'org.greenrobot:greendao:3.0.1'
compile'org.greenrobot:greendao-generator:3.0.0'
Second, configure in build.gradle:
apply plugin: 'org.greenrobot.greendao'
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'org.greenrobot:greendao-gradle-plugin:3.0.0'
}
}
Add the above code to the root module of gradle.
Third, customize the path
greendao {
schemaVersion 1
daoPackage 'com.anye.greendao.gen'
targetGenDir 'src/main/java'
}
Adding the above code to the root module of gradle completes our basic configuration.
Attribute introduction:
SchemaVersion - > Specify database schema version number, migration and other operations will be used;
DaoPackage - > Dao's package name, which defaults to entity's package;
TagetGenDir - > The directory where the database files are generated;
Fourth, create an entity class of User
@Entity
public class User {
@Id
private Long id;
private String name;
@Transient
private int tempUsageCount; // not persisted
}
Make Project
To compile the project, the User entity class compiles automatically, generates get and set methods, and generates three files in the com.anye.greendao.gen directory.
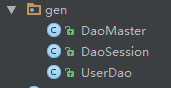
GreenDao uses
public class MyApplication extends Application {
private DaoMaster.DevOpenHelper mHelper;
private SQLiteDatabase db;
private DaoMaster mDaoMaster;
private DaoSession mDaoSession;
public static MyApplication instances;
@Override public void onCreate() {
super.onCreate();
instances = this;
setDatabase();
}
public static MyApplication getInstances(){
return instances;
}
/**
* Setting up green Dao
*/
private void setDatabase() {
// Through the internal class DevOpenHelper of DaoMaster, you can get a convenient SQLiteOpenHelper object.
// As you may have noticed, you don't need to write SQL statements like "CREATE TABLE" because green DAO has already helped you.
// Note: The default DaoMaster.DevOpenHelper deletes all tables when the database is upgraded, meaning that this will result in data loss.
// Therefore, in a formal project, you should also do a layer of encapsulation to achieve database security upgrade.
mHelper = new DaoMaster.DevOpenHelper(this, "notes-db", null);
db = mHelper.getWritableDatabase();
// Note: This database connection belongs to DaoMaster, so multiple sessions refer to the same database connection.
mDaoMaster = new DaoMaster(db);
mDaoSession = mDaoMaster.newSession();
}
public DaoSession getDaoSession() {
return mDaoSession;
}
public SQLiteDatabase getDb() {
return db;
}
}
Get the UserDao object:
mUserDao = MyApplication.getInstances().getDaoSession().getUserDao();
Simple additions, deletions and modifications are implemented:
1. increase
mUser = new User((long)2,"anye3");
mUserDao.insert(mUser);//Add one
2. delete
mUserDao.deleteByKey(id);
3. changes
mUser = new User((long)2,"anye0803");
mUserDao.update(mUser);
4. check
List<User> users = mUserDao.loadAll();
String userName = "";
for (int i = 0; i < users.size(); i++) {
userName += users.get(i).getName()+",";
}
mContext.setText("Query all data==>"+userName);
More operations will not be introduced one by one, you can search for information according to need;
Annotations in greendao
(i) Entity Definition Entity
@ Name of nameInDb in database, class name in entity if not written
@ indexes index
@ Whether or not createInDb creates tables, default to true,false does not create
@ schema specifies the schema name as entity
@ active refreshes both update generation
(two) @Id
(3) NotNull is not null
(4) Unique Unique Constraints
(v) @ToMany one-to-many
(6) OrderBy sort
(7) @ToOne-to-One
(8) Transient is not stored in the database
(9) constructors or methods generated by greendao @generated
Concluding remarks
Overall, GreenDao 3.0 is much simpler in configuration than 2.0.
This article Demo download link: https://github.com/anye0803/GreenDao/ If you like, you can star t.
The purpose of this tutorial is to introduce the basic usage and configuration of green DAO 3.0, advanced and detailed usage, see Official website If you have any questions in this article, please correct them.