preface
Create a new project directly under Android Studio and you can use it!
I. Gradle settings file
settings.gradle is used to indicate which modules Gradle should include when building an application. Only app module is included here
include ':app'
II. Top level construction documents
Look at build.gradle of the project under the project, which is located in the root directory of the project
//buildscript configures repositories and dependencies for gradle itself buildscript{ //ext add custom attribute ext.kotlin_version = '1.2.50' //gradle uses this repository to search or download dependencies repositories { google() jcenter() mavenCentral() } //The dependencies that gradle needs to use to build projects dependencies { classpath 'com.android.tools.build:gradle:3.1.4' classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" } } //Dependencies used by all modules in the project, such as third-party plug-ins or libraries, // Not all modules will use the dependency, should be placed in the module's build.gradle. //Here, only the warehouses used are put here, and no dependency is configured. allprojects { repositories { google() jcenter() } }
III. module level construction documents
Used to configure the build settings of the module
//Application plug-in apply plugin: 'com.android.application' apply plugin: 'kotlin-android' apply plugin: 'kotlin-android-extensions' //Configure build items android { //Compile version, fill in Android version number compileSdkVersion 27 //Build tool version number. Android system needs to use new tools to build every time new features are added // Build toolsversion requires > = compilesdkversion buildToolsVersion "28.0.3" //The default configuration can also override some properties of Android manifest.xml //Can be overridden when multiple versions are required defaultConfig { //applicationId uniquely identifies the published package name. Of course, the source code still uses the package name defined in Android manifest.xml applicationId "com.example.crossroads.aa" //The minimum sdk version, i.e. the minimum supported mobile phone version of APP minSdkVersion 21 //Specify the API target version to be < = compilesdkversion. // If you don't have time to configure new features and systems, set them down a bit. For example, if you don't want to request permissions dynamically, write targetSdkVersion below 23, //If it is > = 23, you need to apply for dynamic permission. targetSdkVersion 26 //APP version number versionCode 1 //Version name of APP versionName "1.0" //If the number of methods is more than 64K and minsdk is less than 21, you need to add a multidex dependency. I will not explain it here multiDexEnabled true //Testing with Android JUnit runner testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } // Configure multiple build types, including release and debug by default //Debug is not explicitly shown in the default configuration we generated, but it already contains debugging related tools and is signed with debug key //release uses proguard's settings. There is no signature by default. buildTypes { release { //Resource compression or not shrinkResources true //Confusion or not minifyEnabled false //Obfuscate file location proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } //There can be more than one flavor dimension flavorDimensions ('money','package') //Product flavors generates multiple apk s of different versions for a set of code, // When a set of code is required to generate multiple apps, you can use this //The configuration here can override defaultConfig productFlavors { //A APP productA { //Configure the applicationId of product A applicationIdSuffix ".A" dimension 'package' } //B APP productB { //Configure the applicationId of product B, which is equivalent to two apps. applicationIdSuffix ".B" dimension 'package' } free{ dimension 'money' } vip{ dimension 'money' } } //APK splitting only supports splitting according to screen density and ABI, // Using this requires each APK to have a different version code //This development period has not been used, do not do detailed explanation, interested in can search APK split. // splits { // //Split by screen density // density { // enable false / / enable the screen density splitting mechanism // } // abi { // enable false / / enable ABI splitting mechanism // } // } } //Module level dependency, further details dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version" implementation 'com.android.support:appcompat-v7:27.1.1' implementation 'com.android.support:design:27.1.1' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' }
Here is a dimension, which means dimension. Packages with the same dimension are mutually exclusive, and different packages can be combined with each other, as shown in the following figure
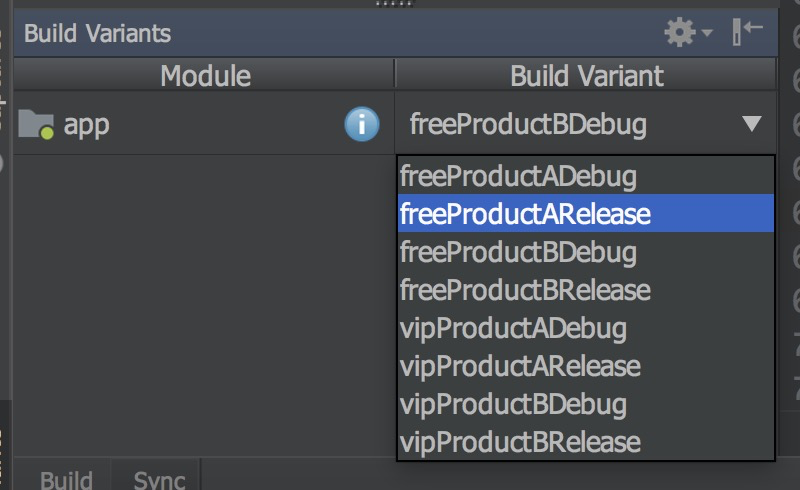
IV. new construction type
buildTypes { ...... beta { // Copy debug properties and debug signatures initWith debug versionNameSuffix 'b' minifyEnabled true proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' //Specify an alternative match. The beta build type has no response configuration in the library dependency. It will use the first configuration available in matchingFallbacks. matchingFallbacks = ['release', 'debug'] } }
V. filter variants
Filter out freeProductADebug and freeProductARelease
variantFilter{variant-> def names =variant.flavors*.name if(names.contains("freeProductA")){ setIgnore(true) } }
Vi. rewrite some properties of Android manifest.xml
Override access? ID in Android manifest.xml
<meta-data android:name="ACCESS_ID" android:value="${ACCESS_ID}"/>
Write as follows in build.gradle:
android { defaultConfig { manifestPlaceholders = [ ACCESS_ID:123456 ] } ... }
If for different build types, write
buildTypes { release { ...... manifestPlaceholders=[ ACCESS_ID:123456 ] } }