23. Getting started with servlet - user registration case
Case - Registration
1. Demand
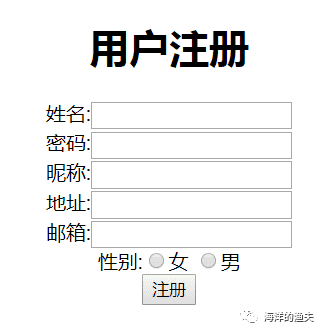
1571732869531
2. Three tier architecture
- Layering in software: it is divided into different layers according to different functions. It is usually divided into three layers: presentation layer (web layer), business layer and persistence (database) layer.

img
- Naming of package names at different levels
layered | Package name (inverted company domain name) |
---|---|
Presentation layer (web layer) | com.web |
Business layer (service layer) | com.service |
Persistence layer (database access layer) | com.dao |
JavaBean | com.bean |
Tool class | com.utils |
- Meaning of stratification:
- Decoupling: reduce the coupling between layers.
- Maintainability: improve the maintainability of the software, and the modification and update of existing functions will not affect the original functions.
- Scalability: improve the scalability of the software. Adding new functions will not affect the existing functions.
- Reusability: when calling functions between different layers, the same functions can be reused.
- Purpose of program design:
- High cohesion and low coupling
- Strong scalability
- Strong maintainability
- Strong reusability
3. Complete the registration case
3.1 registration case ideas
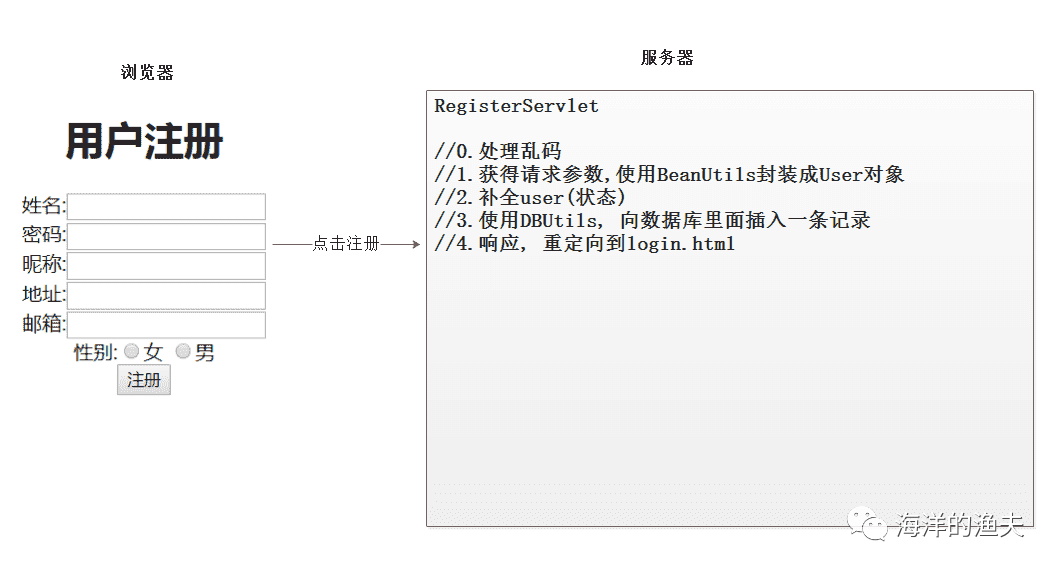
image-20191209154418825
3.2 preparation
3.2.1 creating database
create database userdemo; use userdemo; DROP TABLE IF EXISTS `user`; CREATE TABLE `user` ( `id` int(11) NOT NULL AUTO_INCREMENT, `username` varchar(40) DEFAULT NULL, `password` varchar(40) DEFAULT NULL, `address` varchar(40) DEFAULT NULL, `nickname` varchar(40) DEFAULT NULL, `gender` varchar(10) DEFAULT NULL, `email` varchar(20) DEFAULT NULL, `status` varchar(10) DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8
3.2.2 creating engineering structures
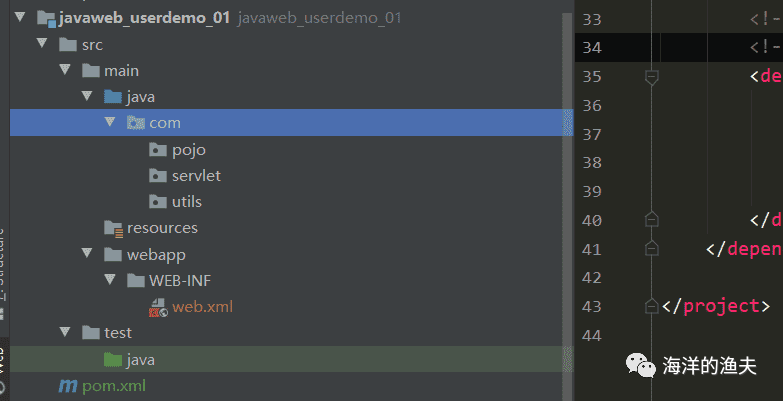
image-20210112083937875
3.2.3 creating JavaBean s
Under the pojo package, create the User class as follows:
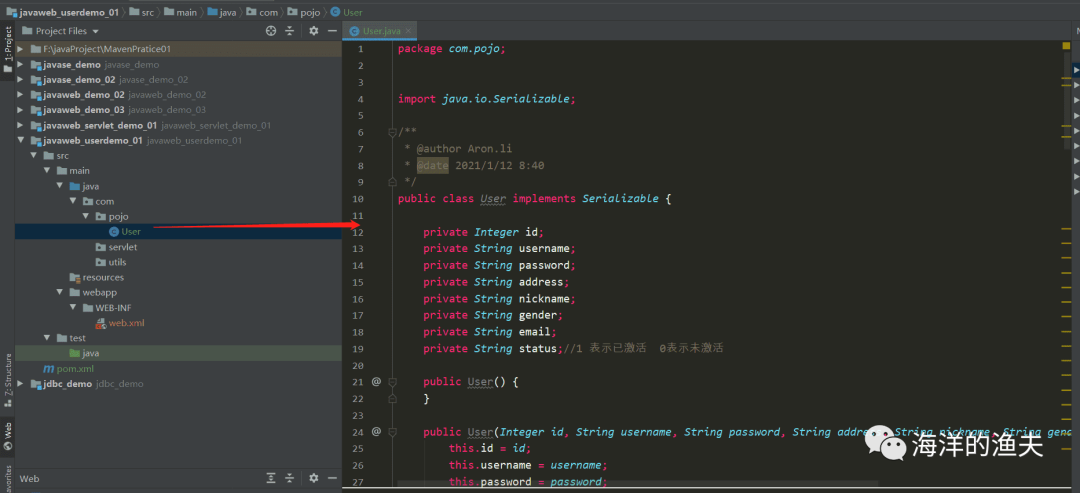
image-20210112084156225
public class User implements Serializable{ private Integer id; private String username; private String password; private String address; private String nickname; private String gender; private String email; private String status;//1 means activated, 0 means inactive //... }
3.3 configuring Maven dependencies
3.3.1 configuration database related dependencies
- mysql driver
- druid
- dbutils
- beanutils
Set the dependency in pom.xml of the project as follows:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.lijw</groupId> <artifactId>javaweb_userdemo_01</artifactId> <version>1.0-SNAPSHOT</version> <!-- Packaging method --> <packaging>war</packaging> <!-- Project properties, setting jdk And coding --> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <!-- Set dependency --> <dependencies> <!-- dbutils rely on --> <!-- https://mvnrepository.com/artifact/commons-dbutils/commons-dbutils --> <dependency> <groupId>commons-dbutils</groupId> <artifactId>commons-dbutils</artifactId> <version>1.7</version> </dependency> <!-- druid rely on --> <!-- https://mvnrepository.com/artifact/com.alibaba/druid --> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.2.4</version> </dependency> <!-- mysql Connection dependency --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.49</version> </dependency> <!-- Import BeanUtils rely on --> <dependency> <groupId>commons-beanutils</groupId> <artifactId>commons-beanutils</artifactId> <version>1.9.3</version> </dependency> <!-- Import commons-io --> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.6</version> </dependency> <!--introduce junit Dependence of --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> <!--introduce servlet Dependence of--> <!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency> </dependencies> </project>
3.4 required tool classes and configuration files
- DruidUtil
- druid.properties

image-20210215171518750
3.4.2 druid.properties
Configure the parameters related to druid connection to the database.
url=jdbc:mysql://localhost:3306/userdemo?rewriteBatchedStatements=true&serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf8&useSSL=false username=root password=Lijw******password**********0 driverClassName=com.mysql.jdbc.Driver initialSize=10 maxActive=10
3.4.1 DruidUtil: used to obtain database connection pool
package com.utils; import com.alibaba.druid.pool.DruidDataSourceFactory; import javax.sql.DataSource; import java.io.InputStream; import java.util.Properties; public class DruidUtil { private static DataSource dataSource; static { try { //1. Create Properties object Properties properties = new Properties(); //2. Convert the configuration file into byte input stream InputStream is = DruidUtil.class.getClassLoader().getResourceAsStream("druid.properties"); //3. Use the properties object to load is properties.load(is); //The druid bottom layer uses the factory design pattern to load the configuration file and create the DruidDataSource object dataSource = DruidDataSourceFactory.createDataSource(properties); } catch (Exception e) { e.printStackTrace(); } } public static DataSource getDataSource(){ return dataSource; } }
3.4.3 DruidUtilTest: Test DruidUtil to obtain database connection
public class DruidUtilTest { @Test public void getDataSource() throws SQLException { // Get database connection pool for druid DataSource dataSource = DruidUtil.getDataSource(); //Test insert data QueryRunner queryRunner = new QueryRunner(DruidUtil.getDataSource()); String sql = "insert into user(`username`, `password`, `address`, `nickname`, `gender`, `email`) values (?,?,?,?,?,?)"; //Insert the data stored by user into the database int i = queryRunner.update(sql, "testuser05", "483212", "Shenzhen", "DevOps Fisherman of the sea", "male", "123@qq.com"); System.out.println("Number of data rows inserted: " + i); } }
The implementation is as follows:
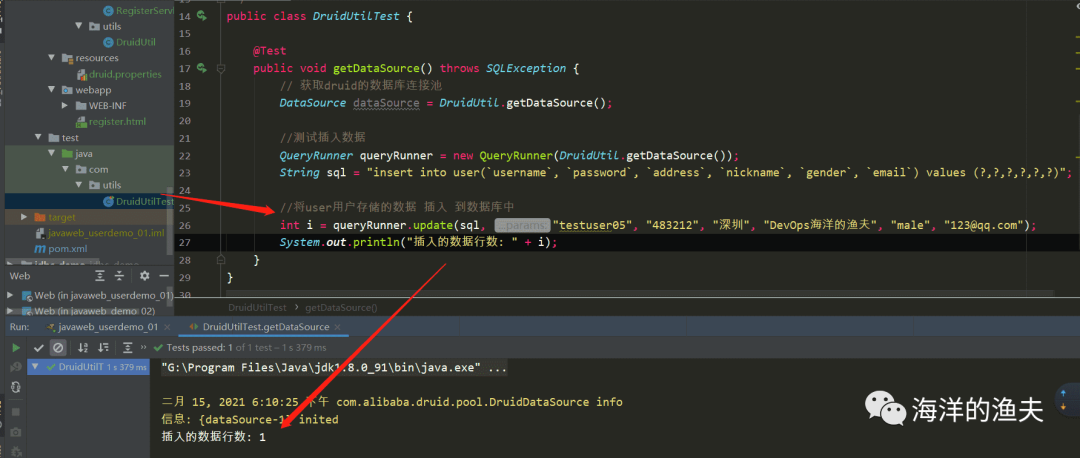
image-20210215181045692
Well, here we are ready for the database connection code. Let's gradually and simply implement the case of user registration.
4. Registration case realization
4.1 registration page
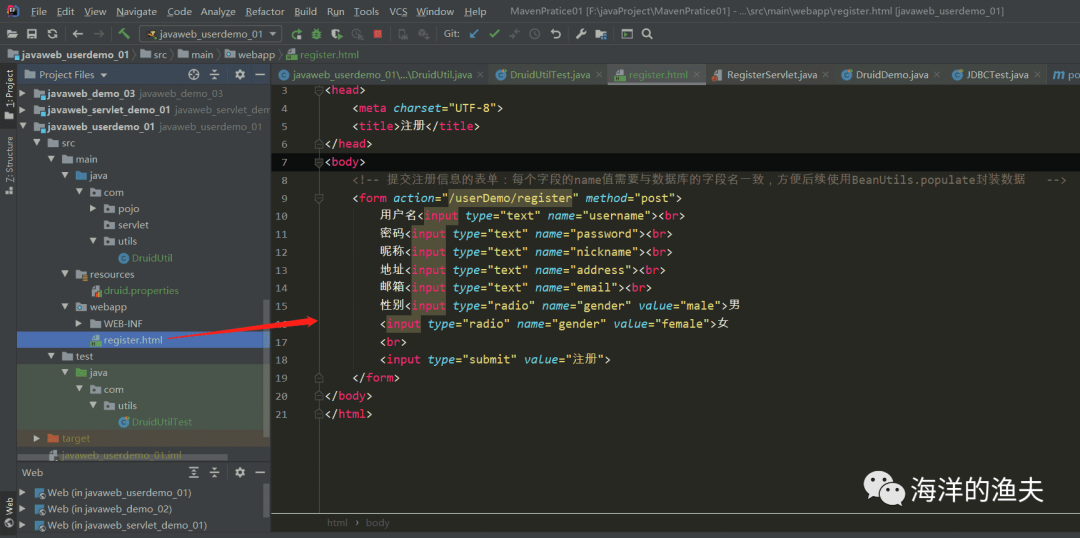
image-20210215174146646
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>register</title> </head> <body> <!-- Form for submitting registration information: for each field name The value needs to be consistent with the field name of the database for subsequent use BeanUtils.populate Encapsulate data --> <form action="/userDemo/register" method="post"> user name<input type="text" name="username"><br> password<input type="text" name="password"><br> nickname<input type="text" name="nickname"><br> address<input type="text" name="address"><br> mailbox<input type="text" name="email"><br> Gender<input type="radio" name="gender" value="male">male <input type="radio" name="gender" value="female">female <br> <input type="submit" value="register"> </form> </body> </html>
Start tomcat to view this page, as follows:
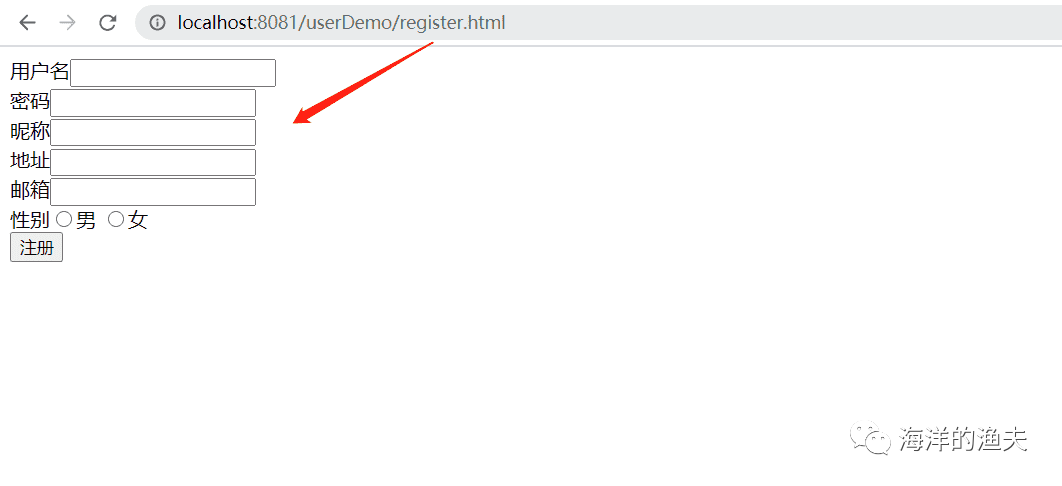
image-20210215174350090
OK, the registration page has been written. Let's continue to write the Servlet of registration business
4.2 code of registerservlet
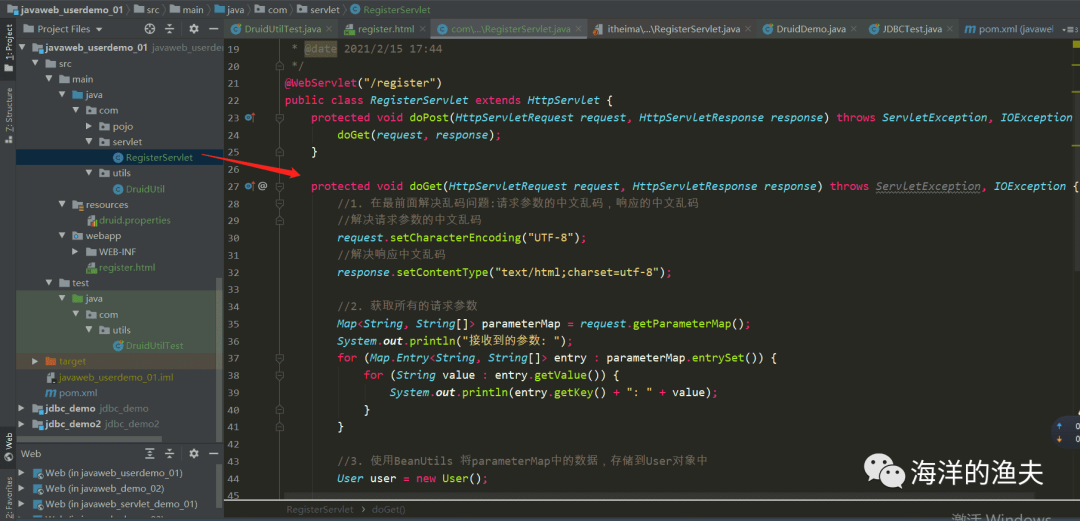
image-20210215181311490
package com.servlet; import com.pojo.User; import com.utils.DruidUtil; import org.apache.commons.beanutils.BeanUtils; import org.apache.commons.dbutils.QueryRunner; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.lang.reflect.InvocationTargetException; import java.util.Map; /** * @author Aron.li * @date 2021/2/15 17:44 */ @WebServlet("/register") public class RegisterServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //1. Solve the garbled code problem at the front: the Chinese garbled code of the request parameter and the Chinese garbled code of the response //Solve the Chinese garbled code of request parameters request.setCharacterEncoding("UTF-8"); //Solve Chinese garbled code in response response.setContentType("text/html;charset=utf-8"); //2. Get all request parameters Map<String, String[]> parameterMap = request.getParameterMap(); System.out.println("Received parameters: "); for (Map.Entry<String, String[]> entry : parameterMap.entrySet()) { for (String value : entry.getValue()) { System.out.println(entry.getKey() + ": " + value); } } //3. Use BeanUtils to store the data in the parameterMap into the User object User user = new User(); //Set the default status to "0" user.setStatus("0"); try { // Use BeanUtils.populate to save the received parameters to the user object BeanUtils.populate(user,parameterMap); //4. Use DBUtils to store user information in the database //The jar packages of mysql driver, druid and dbutils are required here QueryRunner queryRunner = new QueryRunner(DruidUtil.getDataSource()); String sql = "insert into user values (null,?,?,?,?,?,?,?)"; //Insert the data stored by user into the database queryRunner.update(sql,user.getUsername(),user.getPassword(),user.getAddress(), user.getNickname(),user.getGender(),user.getEmail(),user.getStatus()); //If the registration is successful, respond to the browser with the sentence "registration is successful" response.getWriter().write("login was successful"); } catch (Exception e) { e.printStackTrace(); //If the registration fails, respond to the browser with "registration failed" response.getWriter().write("login has failed"); } } }
Perform registration on the registration page as follows:
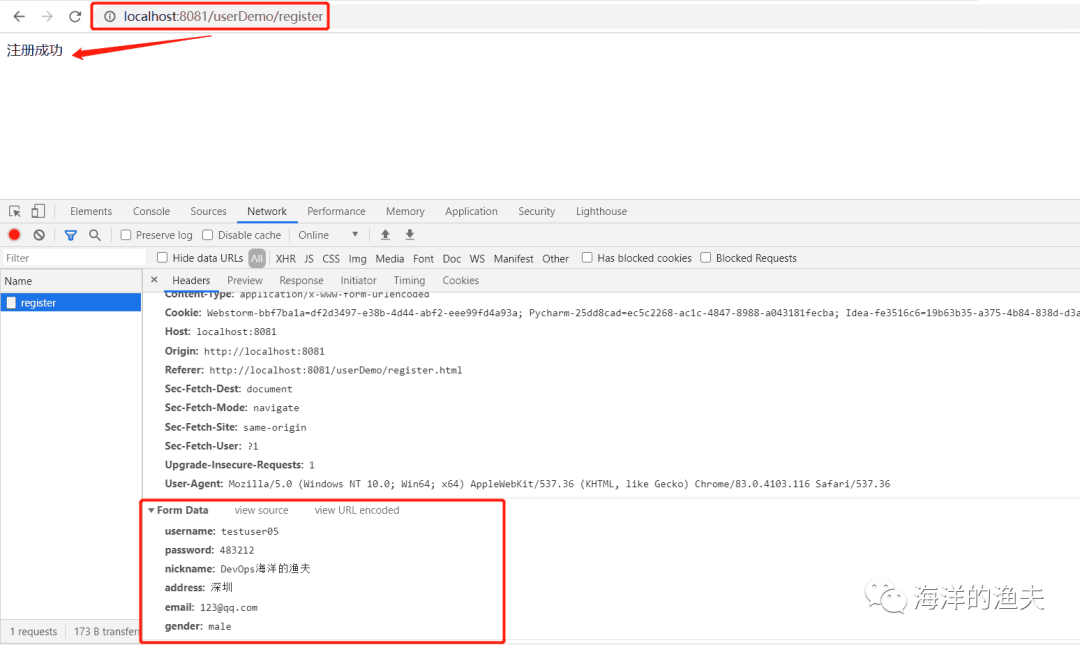
image-20210215181420271
4.3 viewing the inserted registration data in the database
mysql> select * from user \G *************************** 1. row *************************** id: 1 username: testuser05 password: 483212 address: Shenzhen nickname: DevOps Fisherman of the sea gender: male email: 123@qq.com status: 0 1 row in set (0.00 sec) mysql>
4.4 create a successful login homepage index.html
After the above RegisterServlet is registered successfully, only the information of successful registration is returned in the page. Let's write a successful login page to provide page redirection.
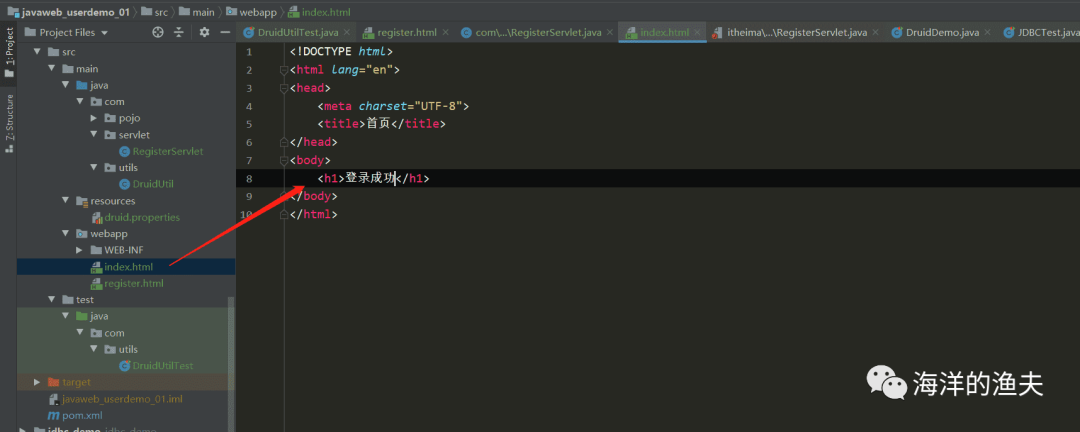
image-20210215181705163
4.5 modify the RegisterServlet. After successful registration, jump to index.html
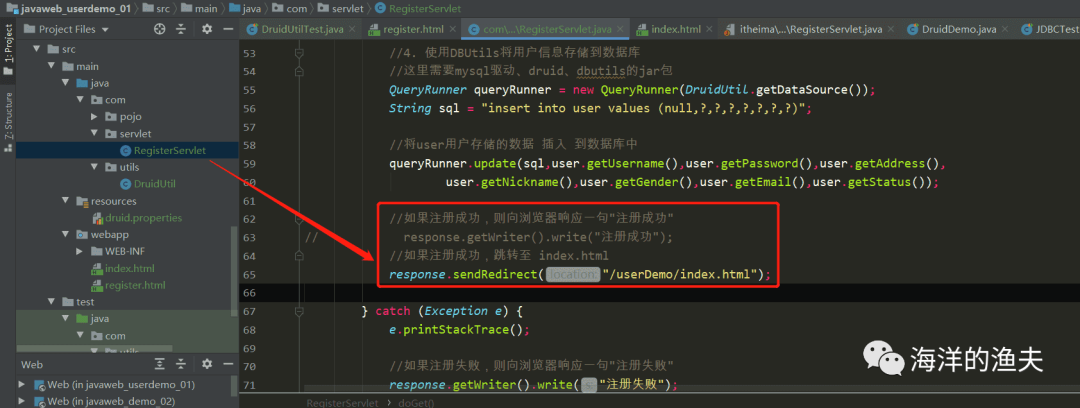
image-20210215182016293
package com.servlet; import com.pojo.User; import com.utils.DruidUtil; import org.apache.commons.beanutils.BeanUtils; import org.apache.commons.dbutils.QueryRunner; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.lang.reflect.InvocationTargetException; import java.util.Map; /** * @author Aron.li * @date 2021/2/15 17:44 */ @WebServlet("/register") public class RegisterServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //1. Solve the garbled code problem at the front: the Chinese garbled code of the request parameter and the Chinese garbled code of the response //Solve the Chinese garbled code of request parameters request.setCharacterEncoding("UTF-8"); //Solve Chinese garbled code in response response.setContentType("text/html;charset=utf-8"); //2. Get all request parameters Map<String, String[]> parameterMap = request.getParameterMap(); System.out.println("Received parameters: "); for (Map.Entry<String, String[]> entry : parameterMap.entrySet()) { for (String value : entry.getValue()) { System.out.println(entry.getKey() + ": " + value); } } //3. Use BeanUtils to store the data in the parameterMap into the User object User user = new User(); //Set the default status to "0" user.setStatus("0"); try { // Use BeanUtils.populate to save the received parameters to the user object BeanUtils.populate(user,parameterMap); //4. Use DBUtils to store user information in the database //The jar packages of mysql driver, druid and dbutils are required here QueryRunner queryRunner = new QueryRunner(DruidUtil.getDataSource()); String sql = "insert into user values (null,?,?,?,?,?,?,?)"; //Insert the data stored by user into the database queryRunner.update(sql,user.getUsername(),user.getPassword(),user.getAddress(), user.getNickname(),user.getGender(),user.getEmail(),user.getStatus()); //If the registration is successful, respond to the browser with the sentence "registration is successful" // response.getWriter().write("registration succeeded"); //If the registration is successful, go to index.html response.sendRedirect("/userDemo/index.html"); } catch (Exception e) { e.printStackTrace(); //If the registration fails, respond to the browser with "registration failed" response.getWriter().write("login has failed"); } } }
4.6 test registration page
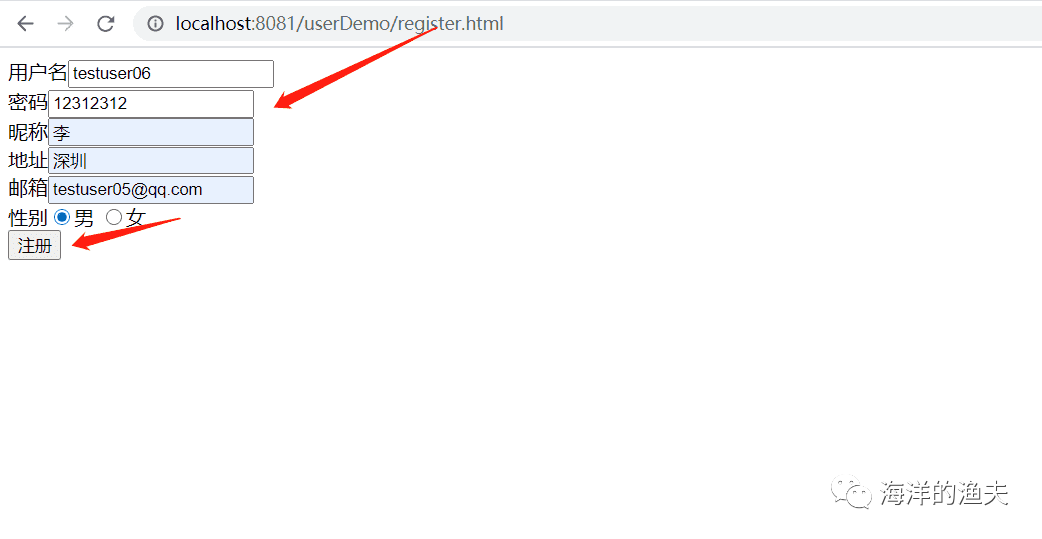
image-20210215181915592
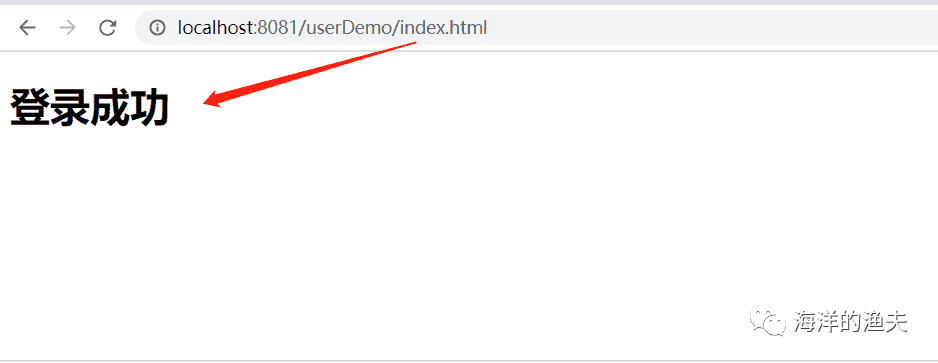
image-20210215181933542
5. Summary
- Registration essence: insert a record into the database
- Idea (in RegisterServlet)
- Obtain the data submitted by the User and encapsulate it into a User object using BeanUtils
- Complete User object (status)
- Use DBUtils to insert a record into the database
- response