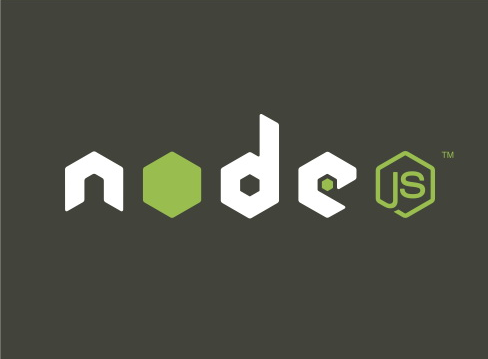
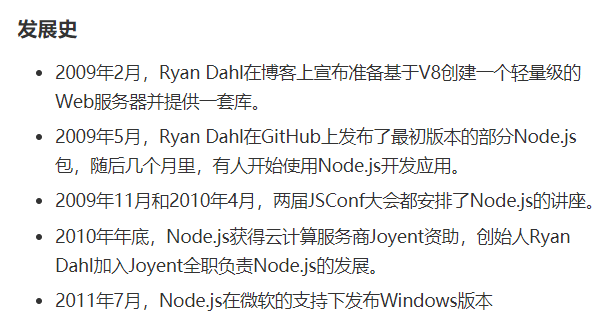
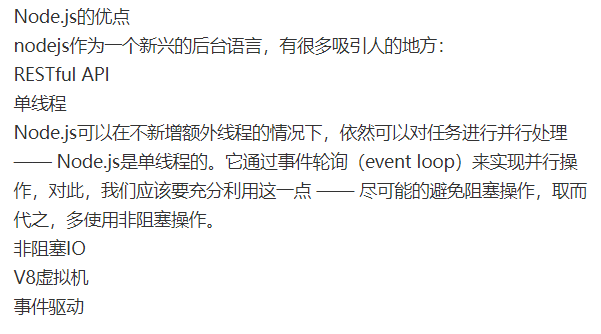
Node.js uses Module module to divide different functions to simplify application development.
var myModule = require('./myModule.js');
Some methods and variables are exposed outside the module, which can be implemented using the exports object.
install
Installation of Node.js under Linux
sudo apt-get update sudo apt-get install node //Or: sudo aptitude update sudo aptitude install node

var http = require('http'); server = http.createServer(function (req, res) { res.writeHeader(200, {"Content-Type": "text/plain"}); res.end("Hello"); }); server.listen(8000); console.log("httpd start @8000");

console.log("Hello World"); node helloworld.js
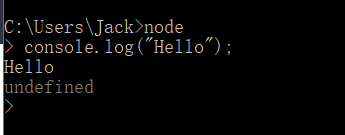
Node.js installation configuration
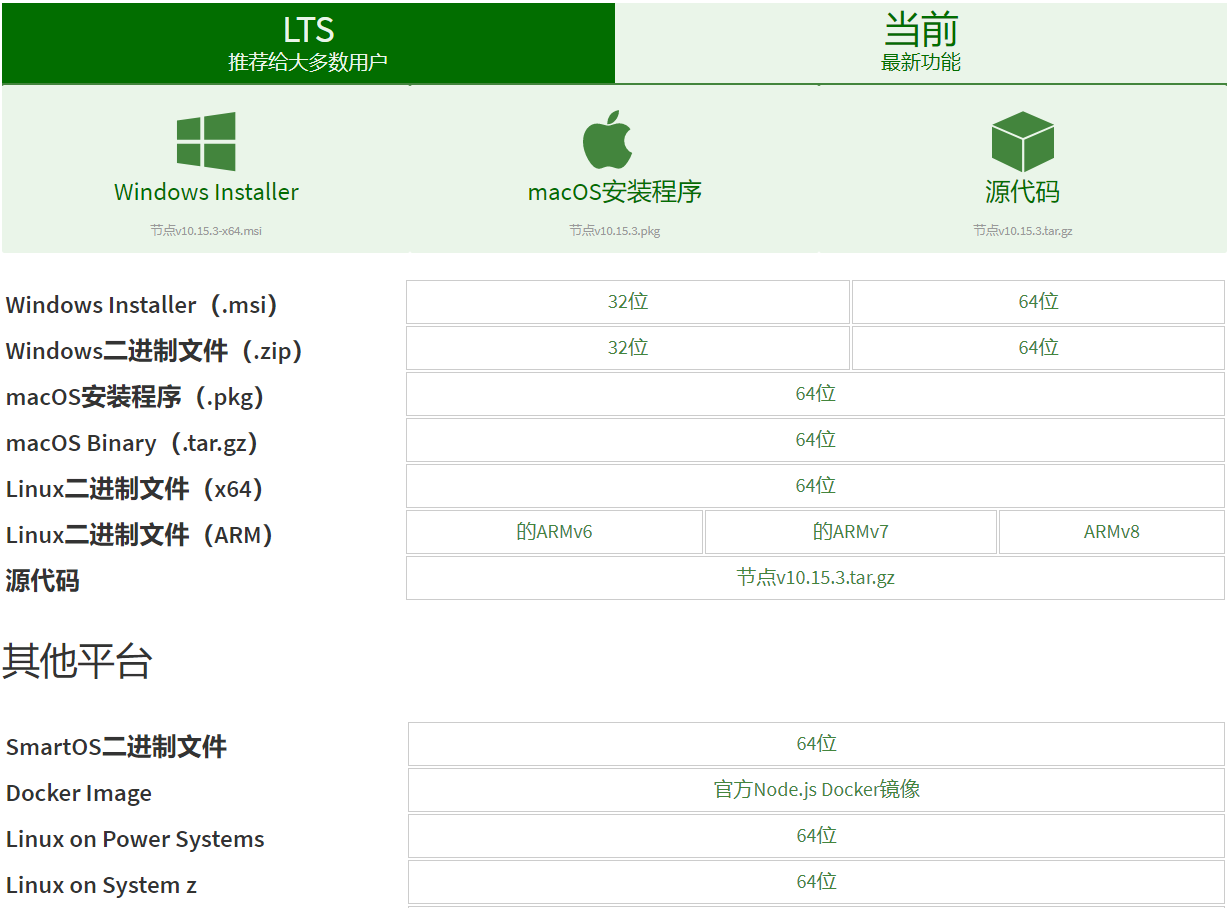

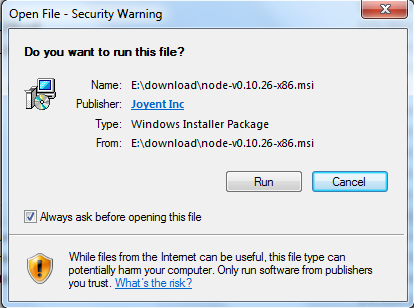
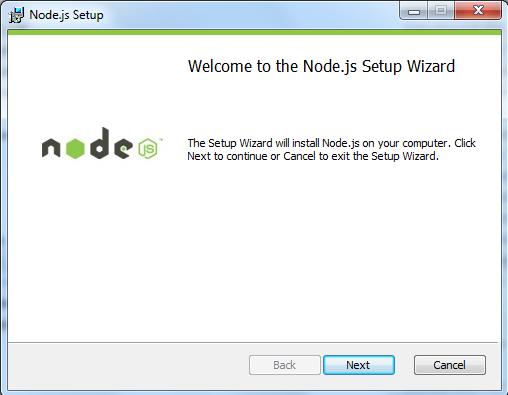
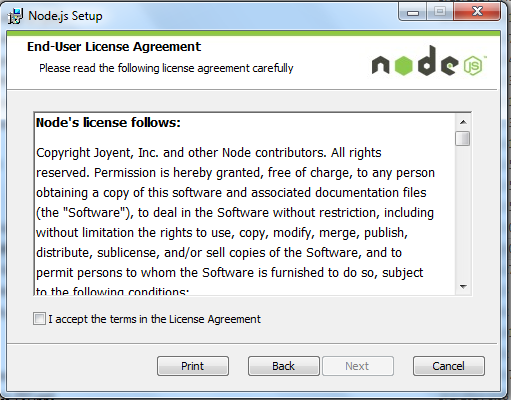
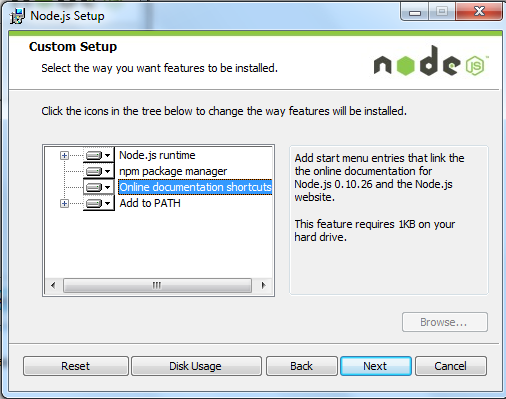
Introduce required module
Create server
Receiving and responding to requests
Step 1: introduce the required module
var http = require("http");
Step 1. Create a server
Use the http.createServer() method to create the server, and use the listen method to bind port 8888 The function receives and responds to data through the request and response parameters.
double-click
// Touch start time touchStartTime: 0, // Touch end time touchEndTime: 0, // Last click event Click time lastTapTime: 0, // Function to trigger after clicking event lastTapTimeoutFunc: null,
///Event triggered by button touch touchStart: function(e) { this.touchStartTime = e.timeStamp }, ///Event triggered by button touch end touchEnd: function(e) { this.touchEndTime = e.timeStamp },
/// Click tap: function(e) { var that = this wx.showModal({ title: 'Tips', content: 'Click event triggered', showCancel: false }) },
//Double click doubleTap: function(e) { var that = this // Control the click event to be triggered within 350ms, and add this layer of judgment to prevent the click event from being triggered in a long time if (that.touchEndTime - that.touchStartTime < 350) { // Current click time var currentTime = e.timeStamp var lastTapTime = that.lastTapTime // Update last click time that.lastTapTime = currentTime // If the time of two clicks is within 300 milliseconds, it is considered as double-click event if (currentTime - lastTapTime < 300) { console.log("double tap") // Cancel the execution of the double click event when the double click event is triggered successfully clearTimeout(that.lastTapTimeoutFunc); wx.showModal({ title: 'Tips', content: 'Double click event triggered', showCancel: false }) } } }, //Click, double-click and long press simultaneously: //Long press longTap: function(e) { console.log("long tap") wx.showModal({ title: 'Tips', content: 'Long press event triggered', showCancel: false }) }, ///Click, double-click multipleTap: function(e) { var that = this // Control the click event to be triggered within 350ms, and add this layer of judgment to prevent the click event from being triggered in a long time if (that.touchEndTime - that.touchStartTime < 350) { // Current click time var currentTime = e.timeStamp var lastTapTime = that.lastTapTime // Update last click time that.lastTapTime = currentTime // If the time of two clicks is within 300 milliseconds, it is considered as double-click event if (currentTime - lastTapTime < 300) { console.log("double tap") // Cancel the execution of the double click event when the double click event is triggered successfully clearTimeout(that.lastTapTimeoutFunc); wx.showModal({ title: 'Tips', content: 'Double click event triggered', showCancel: false }) } else { // The click event is executed with a delay of 300 ms, which is similar to that of the original browser. that.lastTapTimeoutFunc = setTimeout(function () { console.log("tap") wx.showModal({ title: 'Tips', content: 'Click event triggered', showCancel: false }) }, 300); } } },
var http = require('http'); http.createServer(function (request, response) { // Send HTTP header // HTTP status value: 200: OK // Content type: text/plain response.writeHead(200, {'Content-Type': 'text/plain'}); // Send response data "Hello World" response.end('Hello World\n'); }).listen(8888); // The terminal prints the following information console.log('Server running at http://127.0.0.1:8888/');
Please praise! Because your encouragement is the biggest driving force of my writing!
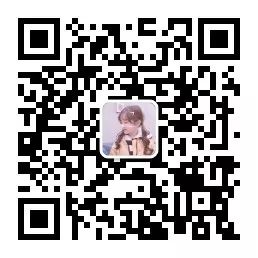
Forced communication group: 711613774
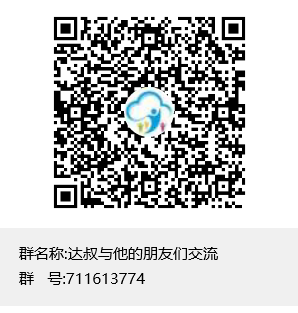