relative_layout
Plug-in Address: https://pub.dev/packages/relative_layout
Corresponding github address: https://github.com/CCY0122/flutter_relative_layout
Relative Layout on Flutter
Usage
Dependence:
relative_layout: ^0.0.1
Import:
import 'package:relative_layout/relative_layout.dart';
Then you can use Relative Layout happily. See Example for specific available attributes.
Requirement:
- children must be LayoutId, and the id of LayoutId must be RelativeId;
- The id of RelativeId, as the identity of the child looking for the relative relationship, must be unique in value.
- Dependent children must be declared before relying on them. If a child B is to be laid out on the left side of child A, A is to be declared before B, and then B is to use toLeft Of:'A'
Example
1. Relative relationship: to Left Of, to Right Of, above, below
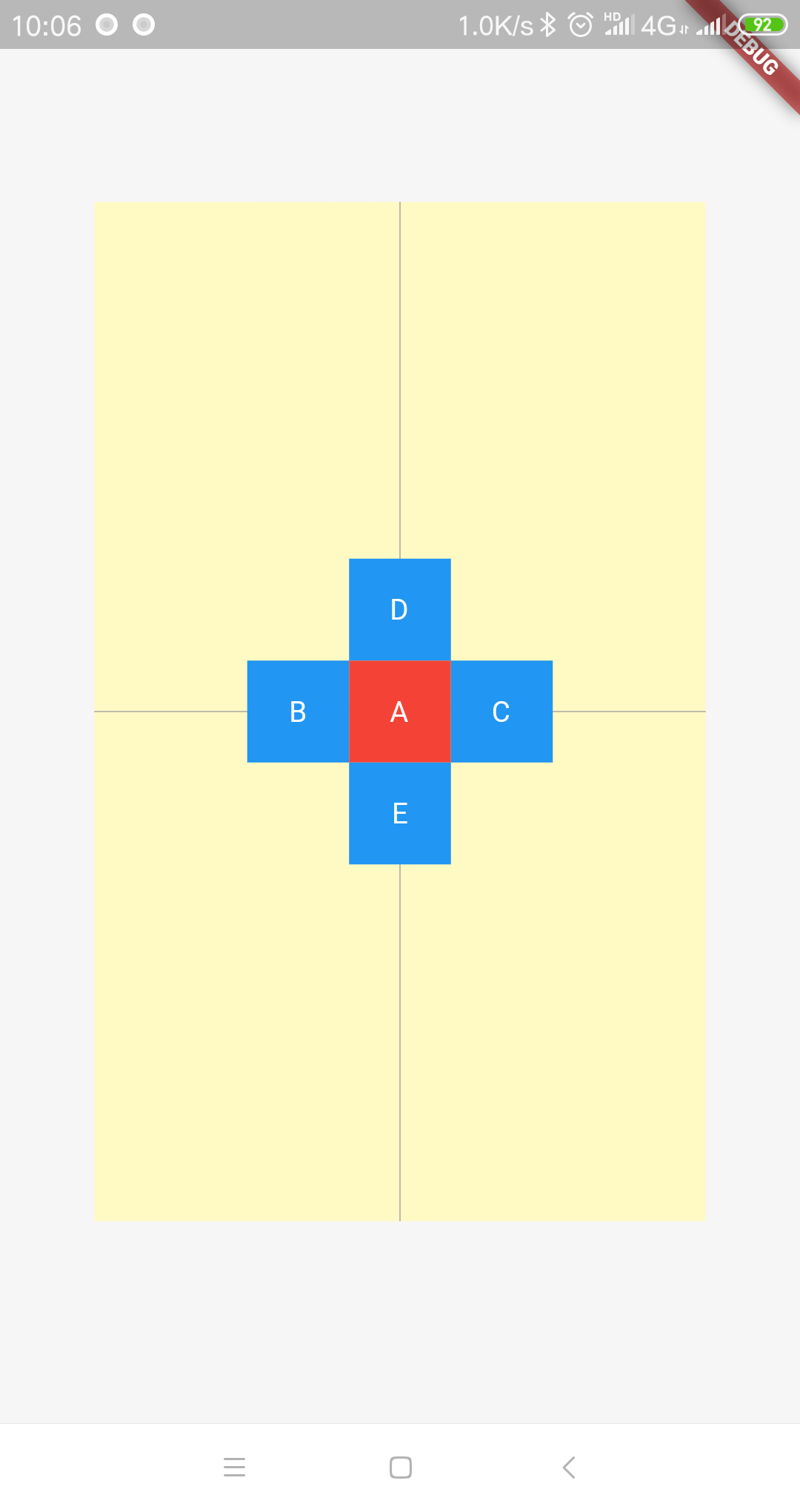
RelativeLayout( children: <LayoutId>[ LayoutId( id: RelativeId('A'), child: simpleContatiner(text: 'A', color: Colors.red), ), LayoutId( id: RelativeId('B', toLeftOf: 'A'), child: simpleContatiner(text: 'B', color: Colors.blue), ), LayoutId( id: RelativeId('C', toRightOf: 'A'), child: simpleContatiner(text: 'C', color: Colors.blue), ), LayoutId( id: RelativeId('D', above: 'A'), child: simpleContatiner(text: 'D', color: Colors.blue), ), LayoutId( id: RelativeId('E', below: 'A'), child: simpleContatiner(text: 'E', color: Colors.blue), ), ], ),
Combination use:
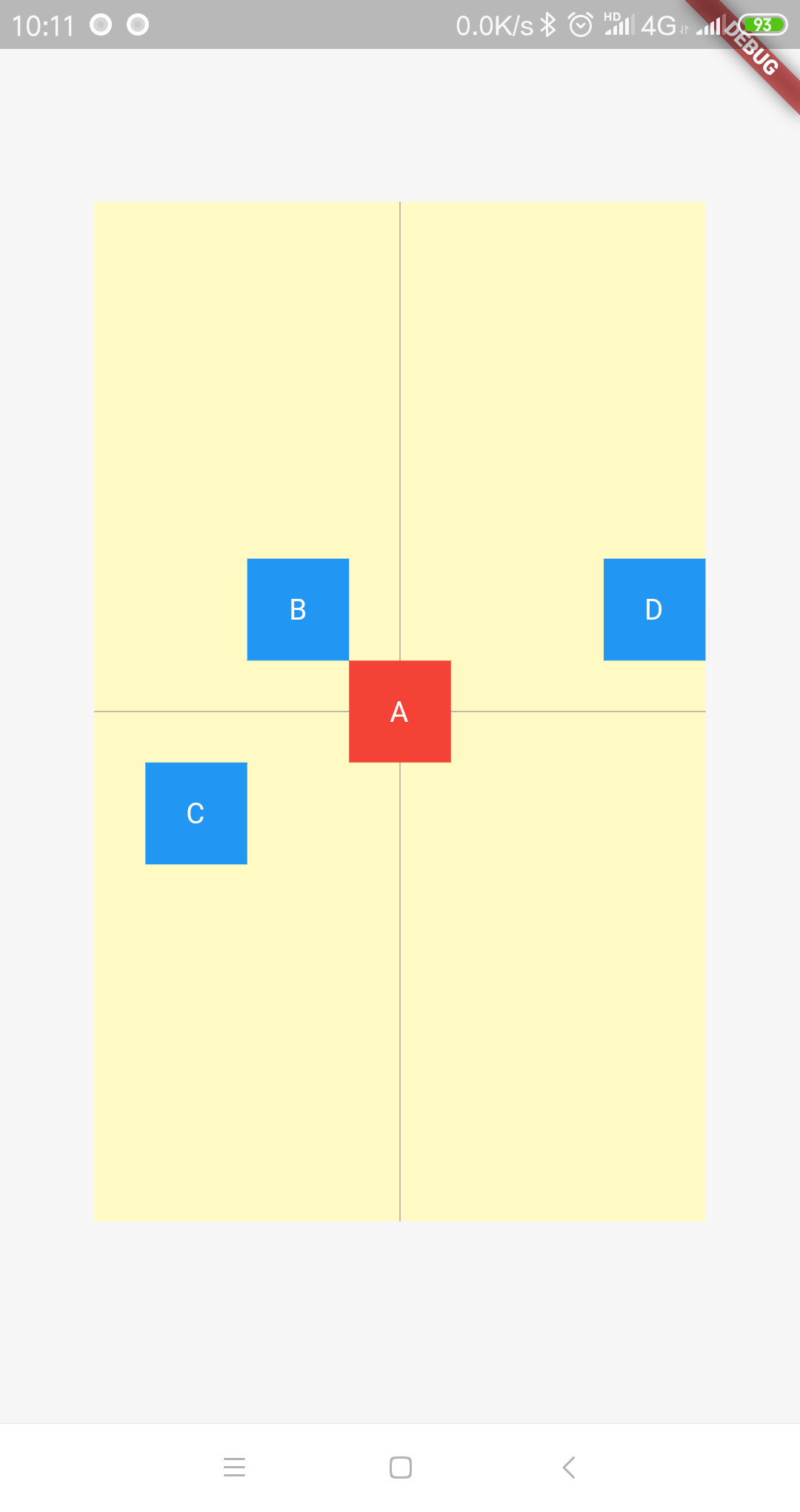
RelativeLayout( children: <LayoutId>[ LayoutId( id: RelativeId('A'), child: simpleContatiner(text: 'A', color: Colors.red), ), LayoutId( id: RelativeId('B', toLeftOf: 'A',above: 'A'), child: simpleContatiner(text: 'B', color: Colors.blue), ), LayoutId( id: RelativeId('C', toLeftOf: 'B',below: 'A'), child: simpleContatiner(text: 'C', color: Colors.blue), ), LayoutId( //Because above has specified the position on the y axis, alignment takes effect only on the x axis. id: RelativeId('D', above: 'A',alignment: Alignment.centerRight), child: simpleContatiner(text: 'D', color: Colors.blue), ), ], ),
2. Axis alignment: align left, align right, align top, align bottom
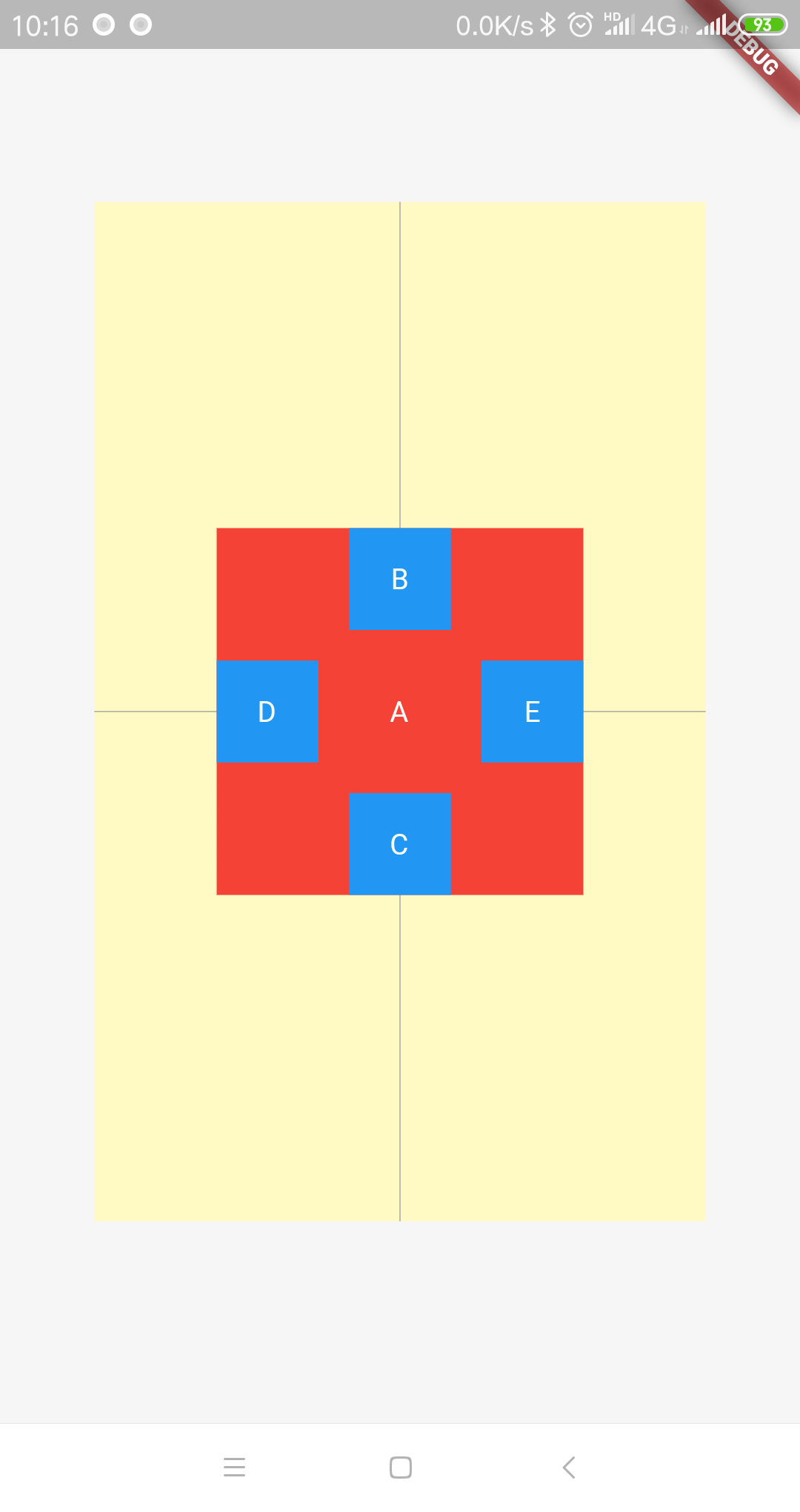
RelativeLayout( children: <LayoutId>[ LayoutId( id: RelativeId('A'), child: simpleContatiner(text: 'A', color: Colors.red,width: 180,height: 180), ), LayoutId( id: RelativeId('B', alignTop: 'A'), child: simpleContatiner(text: 'B', color: Colors.blue), ), LayoutId( id: RelativeId('C', alignBottom: 'A'), child: simpleContatiner(text: 'C', color: Colors.blue), ), LayoutId( id: RelativeId('D', alignLeft: 'A'), child: simpleContatiner(text: 'D', color: Colors.blue), ), LayoutId( id: RelativeId('E', alignRight: 'A'), child: simpleContatiner(text: 'E', color: Colors.blue), ), ], ),
Combination use:
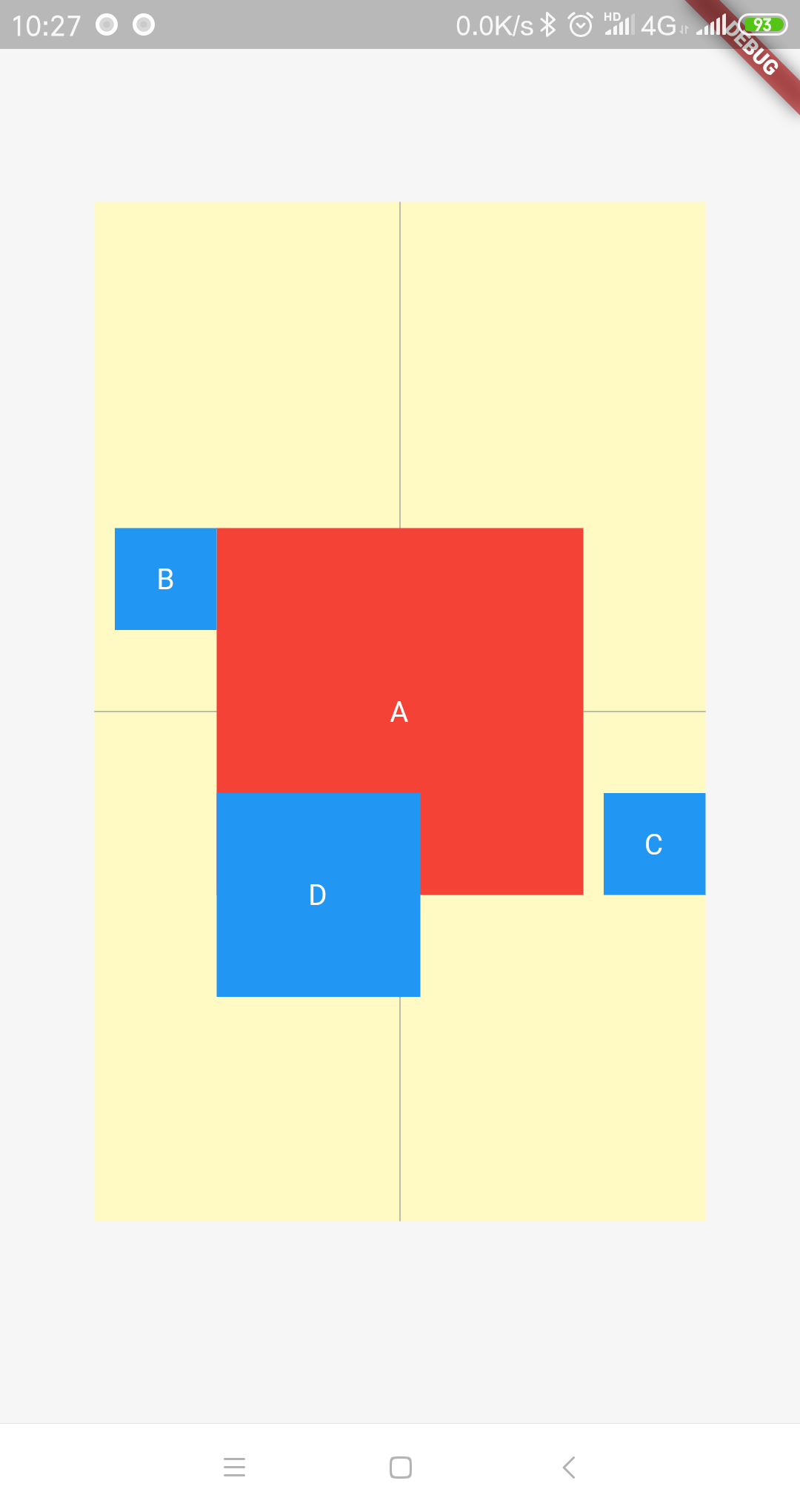
RelativeLayout( children: <LayoutId>[ LayoutId( id: RelativeId('A'), child: simpleContatiner(text: 'A', color: Colors.red,width: 180,height: 180), ), LayoutId( id: RelativeId('B', alignTop: 'A',toLeftOf: 'A'), child: simpleContatiner(text: 'B', color: Colors.blue), ), LayoutId( //Because alignment Bottom has specified the position on the y axis, alignment only takes effect on the x axis. id: RelativeId('C', alignBottom: 'A',alignment: Alignment.centerRight), child: simpleContatiner(text: 'C', color: Colors.blue), ), LayoutId( id: RelativeId('D', alignLeft: 'A',alignTop: 'C',), child: simpleContatiner(text: 'D', color: Colors.blue,height: 100,width: 100), ), ], ),
3. Spillover Model
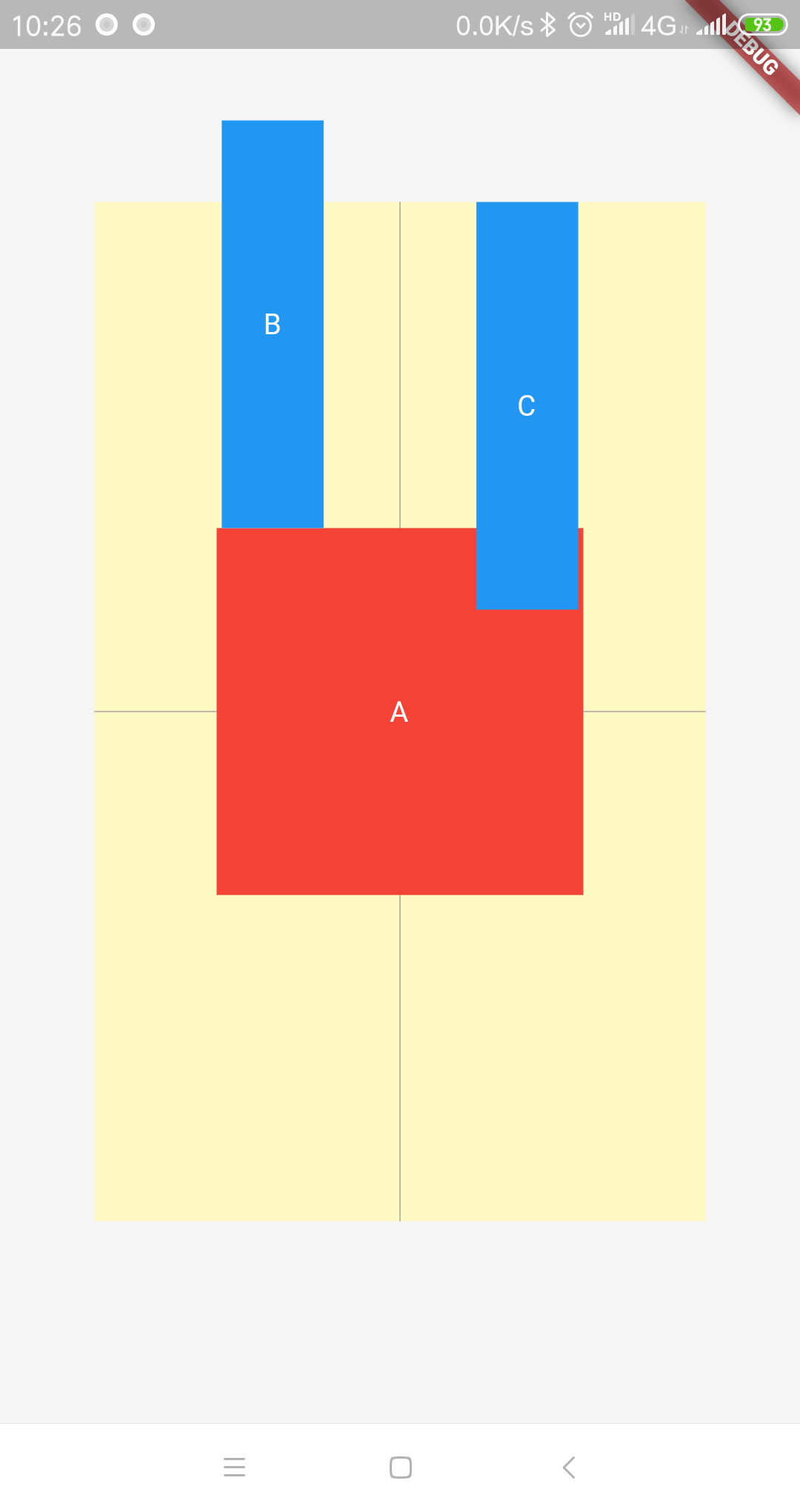
RelativeLayout( children: <LayoutId>[ LayoutId( id: RelativeId('A'), child: simpleContatiner(text: 'A', color: Colors.red,width: 180,height: 180), ), LayoutId( //Can overflow parent layout id: RelativeId('B', above: 'A',alignment: Alignment(-0.5,0),overFlow: RelativeOverFlow.overflow), child: simpleContatiner(text: 'B', color: Colors.blue,height: 200), ), LayoutId( //Do not overflow parent layout id: RelativeId('C', above: 'A',alignment: Alignment(0.5,0),overFlow: RelativeOverFlow.inside), child: simpleContatiner(text: 'C', color: Colors.blue,height: 200), ), ], ),
(clip overflow mode is not supported (that is, the part of the overflow parent layout is tailored), which can be implemented by nesting a layer of ClipRect into the layout.)
simpleContatiner:
Widget simpleContatiner({ Color color = Colors.red, double width = 50, double height = 50, String text = 'A', }) { return Container( width: width, height: height, color: color, alignment: Alignment.center, child: Text( text, style: TextStyle(color: Colors.white), ), ); }