Due to other delays these days, the part about getting to know C language (3) has not been updated (which is also the last part). Today, take time to update the last part of getting to know C language.
@[top] [first knowledge of C language (3)]
1. Common keywords
⚠️:
1) Common keywords cannot be created by yourself.
2) Keyword cannot be a variable name.
Next, introduce the usage of several keywords to further deepen the understanding of keywords.
1.1 keyword typedef:
typedef is a type definition, which should be understood as type renaming.
For example:
//Rename unsigned int uint_ thirty-two typedef unsigned int unit_32; int main() { //At this time, the types of num1 and num2 variables are the same, which is the usage of typedef. unsigned int num1 = 0; uint_32 num2 = 0; return 0; }
1.2 keyword static – static
In C language, static is used to modify variables and functions.
1) Decorated local variables – called static local variables
2) Decorated global variables – called static global variables
3) Modifier functions – called static functions
Before understanding these variables, let's first understand which block static variables are in memory. -- > Memory is a relatively large storage space, which will be divided into different functional areas when using memory. In the current learning stage, you only need to understand: stack area, heap area and static area.
1) static modifies local variables:
Through the output of the following code, let's compare the difference between not adding static and adding static to modify local variables.
//code 1 without static #include <stdio.h> //There is no need for the function to return any value, so use void void test() { int a = 1; a++; printf("%d ", a); } int main() { int i = 0; while(i < 10) { test(); i++; } return 0; } //code 2 modify local variables with static #include <stdio.h> //There is no need for the function to return any value, so use void void test() { static 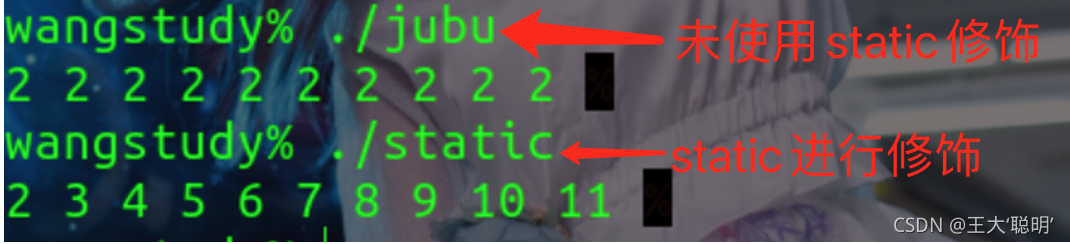 int a = 1; a++; printf("%d ", a); } int main() { int i = 0; while(i < 10) { test(); i++; } return 0; }
It can be inferred from the results of the two codes that if static is used to modify the local variable, each time the test function is called, the (a) used is (a) left by the last function call, indicating that the scope of the local variable has not been destroyed.
According to the results, it can be concluded that:
- When static modifies a local variable, it actually changes the storage type of the variable, from stack storage to static storage.
- Static local variables will not be destroyed when they are out of their scope, which is equivalent to changing the life cycle of local variables.
2) static modifies global variables:
Create two. c files under one source file. If you want to use the global variables of code 2 or other files (external files) in current code 1, you need to declare them first.
//code 1 test.c #include <stdio.h> //extern is a keyword specifically used to declare external symbols. extern int g_val; int main() { printf("%d\n", g_val); return 0; } //code 2 Add.c //g_val is a global variable int g_val = 2021;
Through the running of the code, we can see that the global variables in code 2 can be used after being declared in code 1.
//code 1 test.c #include <stdio.h> //extern is a keyword specifically used to declare external symbols. extern int g_val; int main() { printf("%d\n", g_val); return 0; } //code 2 Add.c //g_val is a global variable static int g_val = 2021;
In the same code, when we use static to modify the global variable, we find that code 1 cannot use the global variable in code 2 again.
By running the code, we can get:
Global variables can be used inside other files in the whole project because they have external link properties. However, when a global variable is modified by static, the external link attribute of the global variable becomes an internal link attribute (no one can see it except the source file). This makes this global variable can only be used inside its own source file, and cannot be used inside other source files. It gives us the feeling that the scope has become smaller.
3) static modifier function:
Modifying functions with static is very similar to modifying global variables.
Functions also have external link properties. However, when it is modified by static, it becomes an internal link attribute. This function can only be used inside its own source file, not inside other files. The scope becomes smaller.
2.#define defines constants and macros
//#define constant #include <stdio.h> //#Define defines constants as: #define + name + parameter #define X 100 #define SKY "sky" int main() { printf("%d\n", X); printf("%s\n", SKY); return 0; }
//#define macro #include <stdio.h> //#Define defines a macro as: #define + macro name (parameter) + macro body #define ADD(X, Y) ((X) + (Y)) int main() { int a = 10; int b = 20; int ret = ADD(a, b); printf("%d\n", ret); return 0; }
In the beginning, you only need to know the two usages of #define now. We'll learn the details later.
3. Pointer
3.1 understanding of memory
Memory is a particularly important memory on the computer. The operation of programs in the computer is carried out in memory.
Therefore, in order to use memory effectively, the memory is divided into small memory units, and the size of each memory unit is 1 byte.
In order to effectively access each unit of memory, the memory unit is numbered. These numbers are called the address of the memory unit.
Because variables are created in memory, each memory unit has an address, so variables also have addresses.
Let's use the following code to identify how to get the address of the variable:
#include <stdio.h> int main() { int num = 10; # //Fetch num address, & -- > is the address character. printf("%p\n", &num); //Print address,% p is printed as an address return 0; }
Because num is a shaping variable, shaping is 4 bytes, and a small piece of memory is 1 byte. Each byte has an address, so it occupies 4 small pieces of memory.
The address of the first byte taken out is the print address of num.
If there is an address, there must be a storage method. The storage of the address needs to be realized by using pointer variables.
#include <stdio.h> int main() { int num = 10; int *p = # //int *p is a pointer variable, which is specially used to store addresses *p = 20; //*Is a dereference operator, * p means to find the object pointed to by p through the value in p, * p is num, and assigning 20 is to change the value of num to 20 printf("%d\n", num); return 0; }
From the results, we can see that * p finds the address of num, then assigns 20 to num, and changes the original value of num = 10.
3.2 pointer variable size
The size of the pointer variable depends on the platform.
In the 32-bit platform, the address is 32 bits (i.e. 4 bytes).
On a 64 bit platform, the address is 64 bits (8 bytes).
#include <stdio.h> int main() { printf("%d\n", sizeof(char *)); printf("%d\n", sizeof(short *)); printf("%d\n", sizeof(int *)); printf("%d\n", sizeof(double *)); printf("%d\n", sizeof(float *)); return 0; }
Because I use a 64 bit platform, the output pointer size is 8 bytes.
In terms of pointers, we learned from our first knowledge. Later, in the process of advanced learning of pointers, we will review and summarize the major aspects of pointers.
4. Structure
Structure is a particularly important knowledge point in C language. Structure makes C language capable of describing complex types.
Next, let's take a brief look at the structure.
For example, to describe a student, the student includes: Name + age + gender + student number, which can only be described by a structure here.
For example: //Describe a student's structure struct stu { char name[20]; //name int age; //Age char sex[5]; //Gender char id[15]; //Student number }; //There is a semicolon here, which cannot be omitted!!!
Operators accessed by structure members are: (.) and (- >).
4.1 (.) operator
//The. Operator is an operator accessed by structure members //The expression form is: structure variable. Structure member #include <stdio.h> //structural morphology struct stu { //Structure member char name[20]; int age; char sex[10]; char id[15]; }; int main() { //Create a structure variable and initialize it struct stu zhangsan = {"Zhang San", 22, "man", "202110216"}; //Structure printed data printf("nanme = %s age = %d sex = %s id = %s\n", zhangsan.name, zhangsan.age, zhangsan.sex, zhangsan.id); return 0; }
4.2 (- >) operator
//->It is also a struct member access operator //The expression is: structure pointer - > structure member #include <stdio.h> //structural morphology struct stu { //Structure member char name[20]; int age; char sex[10]; char id[15]; }; int main() { //Create a structure variable and initialize it struct stu zhangsan = {"Zhang San", 22, "man", "202110216"}; //->Operator struct stu *p = &zhangsan; //Structure printed data //P - > name means that the object pointed to by pl is the member name of the structural variable zhangsan printf("nanme = %s age = %d sex = %s id = %s\n", p->name, p->age, p->sex, p->id); return 0; }
Through the results, we can find that using the - > struct member access operator can also print out the data of the struct, which is simpler than the. Operator.
After learning here, the process of getting to know the C language is over. Through the study of 1, 2 and 3, you can roughly understand all aspects of the C language. The next study is the content of all aspects of advanced learning. If you feel helpful, you can pay attention. I will update the learning process of C language from 0 to 1 from time to time.