1: Execution environment and scope and variable object
var color = "blue";
function changeColor(){
var anotherColor = "red";
// Here you can visit color and another color
}
// Only color can be accessed here
changeColor();
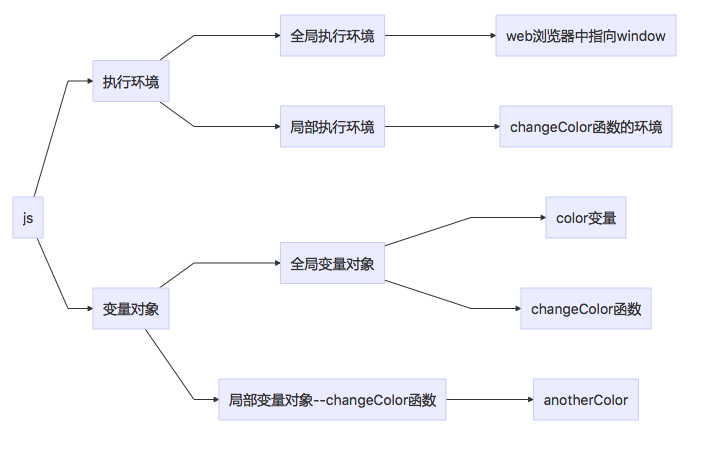
Execution environment is the most important concept in javascript. Each execution environment has a variable object associated with it (saving all defined variables and functions in the execution environment).
2: Scope chain
-
When code is executed in the execution environment, a scope chain is created. Scope chain is essentially a list of pointers to variable objects.
-
If the execution environment is a function, its active object (containing only one variable - > argument object at the beginning) is used as the variable object. ps:argument object does not exist in the global environment
-
The next variable object in the scope chain (based on condition 2) comes from the external environment, and the next variable object comes from the next external environment. In this way, it continues to the global execution environment; the variable object of the global execution environment is always the last object in the scope chain.
3: js has no block level scope
if (true) { var color = "blue"; } alert(color); //"blue" Note: the variable declaration in the if statement in js will add the variable to the execution environment outside of if (currently refers to the window variable); at this time, a value in the window variable object is color = 'blue'
for (var i=0; i < 10; i++){ doSomething(i); } alert(i); //10 Note: in jsinforAfter the end of the loop, it still exists in the execution environment outside the loop, i.ewindowVariable objects have i = 10
Four:closureA closure is a function that has access to variables in the scope of another function. A common way to create a closure is to create another function within one function.
eg: function createComparisonFunction(propertyName) { return function(object1, object2){ var value1 = object1[propertyName]; var value2 = object2[propertyName]; if (value1 < value2){ return -1; } else if (value1 > value2){ return 1; } else { return 0; } }; } //Create function var compareNames = createComparisonFunction("name"); //Calling function var result = compareNames({ name: "Nicholas" }, { name: "Greg" }); //Note: after the createComparisonFunction() function returns, the scope chain of its execution environment will be destroyed, but its active object will remain in memory, and the scope chain of anonymous function still references the active object
The above configuration mechanism of scope chain leads to a side effect, that is, closure can only obtain the last value of any variable of external function
function createFunctions(){
var result = new Array();
for (var i=0; i < 10; i++){
result[i] = function(){
return i;
};
}
console.log(i) // i = 10
for (var j = 0; j < 10; j++){
console.log(result[j]()); // Print 10 10
}
return result;
}
createFunctions();
//Amendment:
function createFunctions(){
var result = new Array();
for (var i = 0; i < 10; i++){
result[i] = function(num){
return num;
}(i);
}
console.log(result); // [ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 ]
return result;
}
createFunctions();
------ in view of the high-level programming of javascript, you are welcome to give some suggestions