






Copyright Statement: This article is the original article of Evankaka, blogger Lin Bingwen, reproduced at http://blog.csdn.net/evankaka
Evankaka Lin Bingwen Original works. Reprinted please indicate the source http://blog.csdn.net/evankaka
Summary: This article mainly talks about how to use Maven to build. spring+Mybatis+SpringMVC+MySQL The article is very detailed, with code and pictures, and finally with the effect of running.
I. Preparations
1. First create a table:
- CREATE TABLE `t_user` (
- `USER_ID` int(11) NOT NULL AUTO_INCREMENT,
- `USER_NAME` char(30) NOT NULL,
- `USER_PASSWORD` char(10) NOT NULL,
- `USER_EMAIL` char(30) NOT NULL,
- PRIMARY KEY (`USER_ID`),
- KEY `IDX_NAME` (`USER_NAME`)
- ) ENGINE=InnoDB AUTO_INCREMENT=11 DEFAULT CHARSET=utf8
CREATE TABLE `t_user` ( `USER_ID` int(11) NOT NULL AUTO_INCREMENT, `USER_NAME` char(30) NOT NULL, `USER_PASSWORD` char(10) NOT NULL, `USER_EMAIL` char(30) NOT NULL, PRIMARY KEY (`USER_ID`), KEY `IDX_NAME` (`USER_NAME`) ) ENGINE=InnoDB AUTO_INCREMENT=11 DEFAULT CHARSET=utf8
Insert some data randomly:
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (1,'Lin Bingwen','1234567','ling20081005@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (2, 'evan', '123', 'fff@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (3, 'kaka', 'cadg', 'fwsfg@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (4, 'simle', 'cscs', 'fsaf@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (5, 'arthur', 'csas', 'fsaff@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (6,'Xiaode','yuh78','fdfas@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (7,'Small','cvff','fsaf@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (8,'Forest House','gvv','lin@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (9,'Lin Bingwen Evankaka','dfsc','ling2008@126.com');
- INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (10, 'apple', 'uih6', 'ff@qq.com');
INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (1, 'Pin-Wen Lin', '1234567@', 'ling20081005@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (2, 'evan', '123', 'fff@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (3, 'kaka', 'cadg', 'fwsfg@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (4, 'simle', 'cscs', 'fsaf@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (5, 'arthur', 'csas', 'fsaff@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (6, 'Xiao De', 'yuh78', 'fdfas@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (7, 'Little', 'cvff', 'fsaf@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (8, 'Lin Lin's home', 'gvv', 'lin@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (9, 'Pin-Wen Lin Evankaka', 'dfsc', 'ling2008@126.com'); INSERT INTO t_user (USER_ID, USER_NAME, USER_PASSWORD, USER_EMAIL) VALUES (10, 'apple', 'uih6', 'ff@qq.com');
II. Engineering Creation
1. Maven Project Creation
(1) New construction
(2) Choosing a Fast Framework
(3) Output project name, package, remember to select war (for web project, spingMVC can be used later)
(4) After creation.
The catalogue is as follows:
(5) Check
The JDK versions in these three places must be the same!!!
3. sping+mybatis configuration
1. The whole project catalogue is as follows:
2. POM files
- <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
- <modelVersion>4.0.0</modelVersion>
- <groupId>com.lin</groupId>
- <artifactId>ssm_project</artifactId>
- <version>0.0.1-SNAPSHOT</version>
- <packaging>war</packaging>
- <properties>
- <!-- spring version number -->
- <spring.version>3.2.8.RELEASE</spring.version>
- <!-- log4j Log File Management Package Version -->
- <slf4j.version>1.6.6</slf4j.version>
- <log4j.version>1.2.12</log4j.version>
- <!-- junit version number -->
- <junit.version>4.10</junit.version>
- <!-- mybatis version number -->
- <mybatis.version>3.2.1</mybatis.version>
- </properties>
- <dependencies>
- <!-- Add to Spring rely on -->
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-core</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-webmvc</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-context</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-context-support</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-aop</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-aspects</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-tx</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-jdbc</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-web</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <!--Unit test dependencies -->
- <dependency>
- <groupId>junit</groupId>
- <artifactId>junit</artifactId>
- <version>${junit.version}</version>
- <scope>test</scope>
- </dependency>
- <!-- Log File Management Package -->
- <!-- log start -->
- <dependency>
- <groupId>log4j</groupId>
- <artifactId>log4j</artifactId>
- <version>${log4j.version}</version>
- </dependency>
- <dependency>
- <groupId>org.slf4j</groupId>
- <artifactId>slf4j-api</artifactId>
- <version>${slf4j.version}</version>
- </dependency>
- <dependency>
- <groupId>org.slf4j</groupId>
- <artifactId>slf4j-log4j12</artifactId>
- <version>${slf4j.version}</version>
- </dependency>
- <!-- log end -->
- <!--spring Unit test dependencies -->
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-test</artifactId>
- <version>${spring.version}</version>
- <scope>test</scope>
- </dependency>
- <!--mybatis rely on -->
- <dependency>
- <groupId>org.mybatis</groupId>
- <artifactId>mybatis</artifactId>
- <version>${mybatis.version}</version>
- </dependency>
- <!-- mybatis/spring package -->
- <dependency>
- <groupId>org.mybatis</groupId>
- <artifactId>mybatis-spring</artifactId>
- <version>1.2.0</version>
- </dependency>
- <!-- mysql Driving package -->
- <dependency>
- <groupId>mysql</groupId>
- <artifactId>mysql-connector-java</artifactId>
- <version>5.1.29</version>
- </dependency>
- </dependencies>
- </project>
3. Java Code - --- src/main/java<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.lin</groupId> <artifactId>ssm_project</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <properties> <!-- spring version number --> <spring.version>3.2.8.RELEASE</spring.version> <!-- log4j Log File Management Package Version --> <slf4j.version>1.6.6</slf4j.version> <log4j.version>1.2.12</log4j.version> <!-- junit version number --> <junit.version>4.10</junit.version> <!-- mybatis version number --> <mybatis.version>3.2.1</mybatis.version> </properties> <dependencies> <!-- Add to Spring rely on --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-tx</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <!--Unit test dependencies --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> <!-- Log File Management Package --> <!-- log start --> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>${log4j.version}</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>${slf4j.version}</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>${slf4j.version}</version> </dependency> <!-- log end --> <!--spring Unit test dependencies --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>${spring.version}</version> <scope>test</scope> </dependency> <!--mybatis rely on --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>${mybatis.version}</version> </dependency> <!-- mybatis/spring package --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>1.2.0</version> </dependency> <!-- mysql Driving package --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.29</version> </dependency> </dependencies> </project>
The catalogue is as follows:
(1)User.Java
Corresponding data base The fields of the middle table, the package com.lin.domain under src/main/java
- package com.lin.domain;
- /**
- * User Mapping class
- *
- * @author linbingwen
- * @time 2015.5.15
- */
- public class User {
- private Integer userId;
- private String userName;
- private String userPassword;
- private String userEmail;
- public Integer getUserId() {
- return userId;
- }
- public void setUserId(Integer userId) {
- this.userId = userId;
- }
- public String getUserName() {
- return userName;
- }
- public void setUserName(String userName) {
- this.userName = userName;
- }
- public String getUserPassword() {
- return userPassword;
- }
- public void setUserPassword(String userPassword) {
- this.userPassword = userPassword;
- }
- public String getUserEmail() {
- return userEmail;
- }
- public void setUserEmail(String userEmail) {
- this.userEmail = userEmail;
- }
- @Override
- public String toString() {
- return "User [userId=" + userId + ", userName=" + userName
- + ", userPassword=" + userPassword + ", userEmail=" + userEmail
- + "]";
- }
- }
package com.lin.domain; /** * User Mapping class * * @author linbingwen * @time 2015.5.15 */ public class User { private Integer userId; private String userName; private String userPassword; private String userEmail; public Integer getUserId() { return userId; } public void setUserId(Integer userId) { this.userId = userId; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getUserPassword() { return userPassword; } public void setUserPassword(String userPassword) { this.userPassword = userPassword; } public String getUserEmail() { return userEmail; } public void setUserEmail(String userEmail) { this.userEmail = userEmail; } @Override public String toString() { return "User [userId=" + userId + ", userName=" + userName + ", userPassword=" + userPassword + ", userEmail=" + userEmail + "]"; } }
(2)UserDao.java
Dao interface class, which corresponds to mapper files. The package com.lin.dao placed under src/main/java is as follows:
- package com.lin.dao;
- import com.lin.domain.User;
- /**
- * Functional summary: User's DAO class
- *
- * @author linbingwen
- * @since 2015 September 28th 2013
- */
- public interface UserDao {
- /**
- *
- * @author linbingwen
- * @since 2015 September 28th 2013
- * @param userId
- * @return
- */
- public User selectUserById(Integer userId);
- }
package com.lin.dao; import com.lin.domain.User; /** * Functional summary: User's DAO class * * @author linbingwen * @since 2015 September 28th 2013 */ public interface UserDao { /** * * @author linbingwen * @since 2015 September 28th 2013 * @param userId * @return */ public User selectUserById(Integer userId); }
(2) UserService.java and UserService Impl. Java
Service interface class and implementation class, the package com.lin.service under src/main/java, are as follows:
UserService.java
- package com.lin.service;
- import org.springframework.stereotype.Service;
- import com.lin.domain.User;
- /**
- * Functional summary: UserService interface class
- *
- * @author linbingwen
- * @since 2015 28 September
- */
- public interface UserService {
- User selectUserById(Integer userId);
- }
package com.lin.service; import org.springframework.stereotype.Service; import com.lin.domain.User; /** * Functional summary: UserService interface class * * @author linbingwen * @since 2015 September 28th 2013 */ public interface UserService { User selectUserById(Integer userId); }
UserServiceImpl.java
- package com.lin.service;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.stereotype.Service;
- import com.lin.dao.UserDao;
- import com.lin.domain.User;
- /**
- * Functional summary: UserService implementation class
- *
- * @author linbingwen
- * @since 2015 28 September
- */
- @Service
- public class UserServiceImpl implements UserService{
- @Autowired
- private UserDao userDao;
- public User selectUserById(Integer userId) {
- return userDao.selectUserById(userId);
- }
- }
package com.lin.service; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.lin.dao.UserDao; import com.lin.domain.User; /** * Functional summary: UserService implementation class * * @author linbingwen * @since 2015 September 28th 2013 */ @Service public class UserServiceImpl implements UserService{ @Autowired private UserDao userDao; public User selectUserById(Integer userId) { return userDao.selectUserById(userId); } }
(4) mapper file
Used to correspond to dao files, under the com.lin.mapper package under src/main/java
- <?xml version="1.0" encoding="UTF-8"?>
- <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
- "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
- <mapper namespace="com.lin.dao.UserDao">
- <!--Set up domain Class corresponds to the fields of the tables in the database one by one. Pay attention to the fields in the database and the fields in the database. domain The field names in the classes are different, so be sure to do it here! uuuuuuuuuu-->
- <resultMap id="BaseResultMap" type="com.lin.domain.User">
- <id column="USER_ID" property="userId" jdbcType="INTEGER" />
- <result column="USER_NAME" property="userName" jdbcType="CHAR" />
- <result column="USER_PASSWORD" property="userPassword" jdbcType="CHAR" />
- <result column="USER_EMAIL" property="userEmail" jdbcType="CHAR" />
- </resultMap>
- <!-- Query single record -->
- <select id="selectUserById" parameterType="int" resultMap="BaseResultMap">
- SELECT * FROM t_user WHERE USER_ID = #{userId}
- </select>
- </mapper>
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.lin.dao.UserDao"> <!--Set up domain Class corresponds to the fields of the tables in the database one by one. Pay attention to the fields in the database and the fields in the database. domain The field names in the classes are different, so be sure to do it here! uuuuuuuuuu--> <resultMap id="BaseResultMap" type="com.lin.domain.User"> <id column="USER_ID" property="userId" jdbcType="INTEGER" /> <result column="USER_NAME" property="userName" jdbcType="CHAR" /> <result column="USER_PASSWORD" property="userPassword" jdbcType="CHAR" /> <result column="USER_EMAIL" property="userEmail" jdbcType="CHAR" /> </resultMap> <!-- Query single record --> <select id="selectUserById" parameterType="int" resultMap="BaseResultMap"> SELECT * FROM t_user WHERE USER_ID = #{userId} </select> </mapper>
4. Resource Allocation--src/main/resources
The catalogue is as follows:
(1)mybatis configuration file
There's nothing here, because it's all in application.xml, under the mybatis folder under src/main/resources
mybatis-config.xml reads as follows:
- <?xml version="1.0" encoding="UTF-8"?>
- <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
- "http://mybatis.org/dtd/mybatis-3-config.dtd">
- <configuration>
- </configuration>
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> </configuration>
(2) Data source configuration jdbc.properties
Put it in the properties folder under src/main/resources
- jdbc_driverClassName=com.mysql.jdbc.Driver
- jdbc_url=jdbc:mysql://localhost:3306/learning
- jdbc_username=root
- jdbc_password=christmas258@
(3) Spring configurationjdbc_driverClassName=com.mysql.jdbc.Driver jdbc_url=jdbc:mysql://localhost:3306/learning jdbc_username=root jdbc_password=christmas258@
This is the most important: application.xml is as follows
- <?xml version="1.0" encoding="UTF-8"?>
- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
- xmlns:aop="http://www.springframework.org/schema/aop"
- xsi:schemaLocation="
- http://www.springframework.org/schema/beans
- http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
- http://www.springframework.org/schema/aop
- http://www.springframework.org/schema/aop/spring-aop-3.0.xsd
- http://www.springframework.org/schema/context
- http://www.springframework.org/schema/context/spring-context-3.0.xsd">
- <!-- Introduce jdbc configuration file -->
- <bean id="propertyConfigurer" class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
- <property name="locations">
- <list>
- <value>classpath:properties/*.properties</value>
- <!--If you have more than one configuration file, you just need to add it here - >
- </list>
- </property>
- </bean>
- <!-- Configure Data Source - > Configure Data Source -> Configure Data Source -> Configure Data Source -> Configure Data Source -> Configure Data Source
- <bean id="dataSource"
- class="org.springframework.jdbc.datasource.DriverManagerDataSource">
- <!-- Do not use properties to configure --> uuuuuuuuuuuu
- <!-- <property name="driverClassName" value="com.mysql.jdbc.Driver" />
- <property name="url" value="jdbc:mysql://localhost:3306/learning" />
- <property name="username" value="root" />
- <property name="password" value="christmas258@" /> -->
- <!-- Use properties to configure --> uuuuuuuuuuuu
- <property name="driverClassName">
- <value>${jdbc_driverClassName}</value>
- </property>
- <property name="url">
- <value>${jdbc_url}</value>
- </property>
- <property name="username">
- <value>${jdbc_username}</value>
- </property>
- <property name="password">
- <value>${jdbc_password}</value>
- </property>
- </bean>
- <!-- All mapper interface files corresponding to XxxxMapper.xml are scanned automatically, so there is no need to configure the mapping of Mpper manually one by one, as long as the Mapper interface class corresponds to the Mapper mapping file. >
- <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
- <property name="basePackage"
- value="com.lin.dao" />
- </bean>
- <!-- Configure Mybatis files, mapperLocations ** Mapper.xml file locations, configLocation s mybatis-config file locations - >.
- <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
- <property name="dataSource" ref="dataSource" />
- <property name="mapperLocations" value="classpath*:com/lin/mapper/**/*.xml"/>
- <property name="configLocation" value="classpath:mybatis/mybatis-config.xml" />
- <!-- <property name="typeAliasesPackage" value="com.tiantian.ckeditor.model"
- /> -->
- </bean>
- <!-- Automatic Scanning Annotation bean -->
- <context:component-scan base-package="com.lin.service" />
- </beans>
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <!-- Introduce jdbc configuration file --> <bean id="propertyConfigurer" class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"> <property name="locations"> <list> <value>classpath:properties/*.properties</value> <!--If you have more than one configuration file, you just need to add it here - > </list> </property> </bean> <!-- Configuring data sources - > <bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <!-- Configuration without using properties - > uuuuuuuuuuuu <!-- <property name="driverClassName" value="com.mysql.jdbc.Driver" /> <property name="url" value="jdbc:mysql://localhost:3306/learning" /> <property name="username" value="root" /> <property name="password" value="christmas258@" /> --> <!-- Use properties to configure - > <property name="driverClassName"> <value>${jdbc_driverClassName}</value> </property> <property name="url"> <value>${jdbc_url}</value> </property> <property name="username"> <value>${jdbc_username}</value> </property> <property name="password"> <value>${jdbc_password}</value> </property> </bean> <!-- All mapper interface files corresponding to XxxxMapper.xml are scanned automatically, so there is no need to configure the mapping of Mpper manually one by one, as long as the Mapper interface class corresponds to the Mapper mapping file. > <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="com.lin.dao" /> </bean> <!-- Configure Mybatis file, mapperLocations ** Mapper.xml file location, configLocation configuration mybatis-config file location - > <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSource" /> <property name="mapperLocations" value="classpath*:com/lin/mapper/**/*.xml"/> <property name="configLocation" value="classpath:mybatis/mybatis-config.xml" /> <!-- <property name="typeAliasesPackage" value="com.tiantian.ckeditor.model" /> --> </bean> <!-- Automatic Scanning Annotation bean --> <context:component-scan base-package="com.lin.service" /> </beans>
(4) Log printing log4j.properties
Just put it in src/main/resources
- log4j.rootLogger=DEBUG,Console,Stdout
- #Console
- log4j.appender.Console=org.apache.log4j.ConsoleAppender
- log4j.appender.Console.layout=org.apache.log4j.PatternLayout
- log4j.appender.Console.layout.ConversionPattern=%d [%t] %-5p [%c] - %m%n
- log4j.logger.java.sql.ResultSet=INFO
- log4j.logger.org.apache=INFO
- log4j.logger.java.sql.Connection=DEBUG
- log4j.logger.java.sql.Statement=DEBUG
- log4j.logger.java.sql.PreparedStatement=DEBUG
- log4j.appender.Stdout = org.apache.log4j.DailyRollingFileAppender
- log4j.appender.Stdout.File = E://logs/log.log
- log4j.appender.Stdout.Append = true
- log4j.appender.Stdout.Threshold = DEBUG
- log4j.appender.Stdout.layout = org.apache.log4j.PatternLayout
- log4j.appender.Stdout.layout.ConversionPattern = %-d{yyyy-MM-dd HH:mm:ss} [ %t:%r ] - [ %p ] %m%n
log4j.rootLogger=DEBUG,Console,Stdout #Console log4j.appender.Console=org.apache.log4j.ConsoleAppender log4j.appender.Console.layout=org.apache.log4j.PatternLayout log4j.appender.Console.layout.ConversionPattern=%d [%t] %-5p [%c] - %m%n log4j.logger.java.sql.ResultSet=INFO log4j.logger.org.apache=INFO log4j.logger.java.sql.Connection=DEBUG log4j.logger.java.sql.Statement=DEBUG log4j.logger.java.sql.PreparedStatement=DEBUG log4j.appender.Stdout = org.apache.log4j.DailyRollingFileAppender log4j.appender.Stdout.File = E://logs/log.log log4j.appender.Stdout.Append = true log4j.appender.Stdout.Threshold = DEBUG log4j.appender.Stdout.layout = org.apache.log4j.PatternLayout log4j.appender.Stdout.layout.ConversionPattern = %-d{yyyy-MM-dd HH:mm:ss} [ %t:%r ] - [ %p ] %m%n
Unit Testing
The above configuration is in good condition, and the next step is the success of the test.
The entire catalogue is as follows:
(1)test base class
- package com.lin.baseTest;
- import org.junit.runner.RunWith;
- import org.slf4j.Logger;
- import org.slf4j.LoggerFactory;
- import org.springframework.test.context.ContextConfiguration;
- import org.springframework.test.context.junit4.AbstractJUnit4SpringContextTests;
- import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
- /**
- * Functional summary:
- *
- * @author linbingwen
- * @since 2015 28 September
- */
- //Specify the configuration file for bean injection
- @ContextConfiguration(locations = { "classpath:application.xml" })
- //Use the standard JUnit@RunWith annotation to tell JUnit to use Spring TestRunner
- @RunWith(SpringJUnit4ClassRunner.class)
- public abstract class SpringTestCase extends AbstractJUnit4SpringContextTests{
- protected Logger logger = LoggerFactory.getLogger(getClass());
- }
package com.lin.baseTest; import org.junit.runner.RunWith; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.AbstractJUnit4SpringContextTests; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; /** * Functional summary: * * @author linbingwen * @since 2015 September 28th 2013 */ //Specify the configuration file for bean injection @ContextConfiguration(locations = { "classpath:application.xml" }) //Use the standard JUnit@RunWith annotation to tell JUnit to use Spring TestRunner @RunWith(SpringJUnit4ClassRunner.class) public abstract class SpringTestCase extends AbstractJUnit4SpringContextTests{ protected Logger logger = LoggerFactory.getLogger(getClass()); }
(2) Test class
- package com.lin.service;
- import org.apache.log4j.Logger;
- import org.junit.Test;
- import org.springframework.beans.factory.annotation.Autowired;
- import com.lin.baseTest.SpringTestCase;
- import com.lin.domain.User;
- /**
- * Functional summary: UserService unit testing
- *
- * @author linbingwen
- * @since 2015 28 September
- */
- public class UserServiceTest extends SpringTestCase {
- @Autowired
- private UserService userService;
- Logger logger = Logger.getLogger(UserServiceTest.class);
- @Test
- public void selectUserByIdTest(){
- User user = userService.selectUserById(10);
- logger.debug("Finding results" + user);
- }
- }
Select UserByIdTest, and then right-click to run as followspackage com.lin.service; import org.apache.log4j.Logger; import org.junit.Test; import org.springframework.beans.factory.annotation.Autowired; import com.lin.baseTest.SpringTestCase; import com.lin.domain.User; /** * Functional summary: UserService unit testing * * @author linbingwen * @since 2015 September 28th 2013 */ public class UserServiceTest extends SpringTestCase { @Autowired private UserService userService; Logger logger = Logger.getLogger(UserServiceTest.class); @Test public void selectUserByIdTest(){ User user = userService.selectUserById(10); logger.debug("Finding results" + user); } }
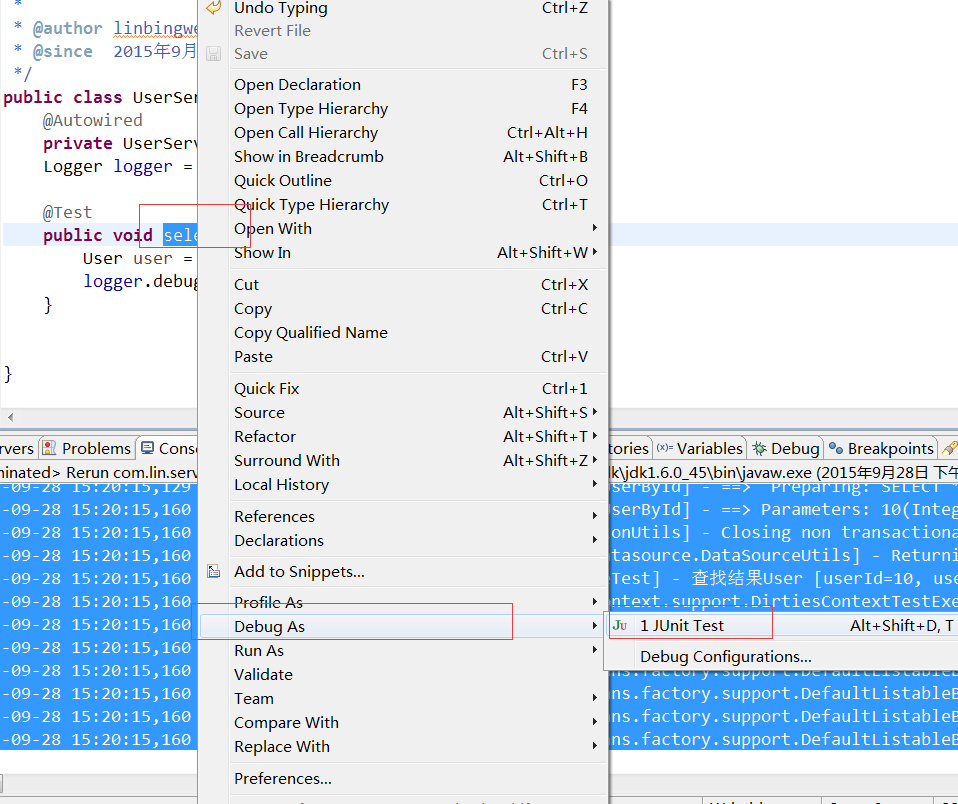
Output results:
Here
- 2015-09-28 15:20:15,129 [main] DEBUG [com.lin.dao.UserDao.selectUserById] - ==> Preparing: SELECT * FROM t_user WHERE USER_ID = ?
- 2015-09-28 15:20:15,160 [main] DEBUG [com.lin.dao.UserDao.selectUserById] - ==> Parameters: 10(Integer)
- 2015-09-28 15:20:15,160 [main] DEBUG [org.mybatis.spring.SqlSessionUtils] - Closing non transactional SqlSession [org.apache.ibatis.session.defaults.DefaultSqlSession@6b64bff9]
- 2015-09-28 15:20:15,160 [main] DEBUG [org.springframework.jdbc.datasource.DataSourceUtils] - Returning JDBC Connection to DataSource
- 2015-09-28 15:20:15,160 [main] DEBUG [com.lin.service.UserServiceTest] - Finding results User [userId=10, userName=apple, userPassword=uih6, userEmail=ff@qq.com]
2015-09-28 15:20:15,129 [main] DEBUG [com.lin.dao.UserDao.selectUserById] - ==> Preparing: SELECT * FROM t_user WHERE USER_ID = ? 2015-09-28 15:20:15,160 [main] DEBUG [com.lin.dao.UserDao.selectUserById] - ==> Parameters: 10(Integer) 2015-09-28 15:20:15,160 [main] DEBUG [org.mybatis.spring.SqlSessionUtils] - Closing non transactional SqlSession [org.apache.ibatis.session.defaults.DefaultSqlSession@6b64bff9] 2015-09-28 15:20:15,160 [main] DEBUG [org.springframework.jdbc.datasource.DataSourceUtils] - Returning JDBC Connection to DataSource 2015-09-28 15:20:15,160 [main] DEBUG [com.lin.service.UserServiceTest] - Finding results User [userId=10, userName=apple, userPassword=uih6, userEmail=ff@qq.com]
Database:
5. Conversion of web Engineering
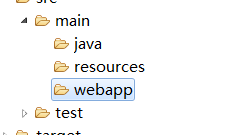
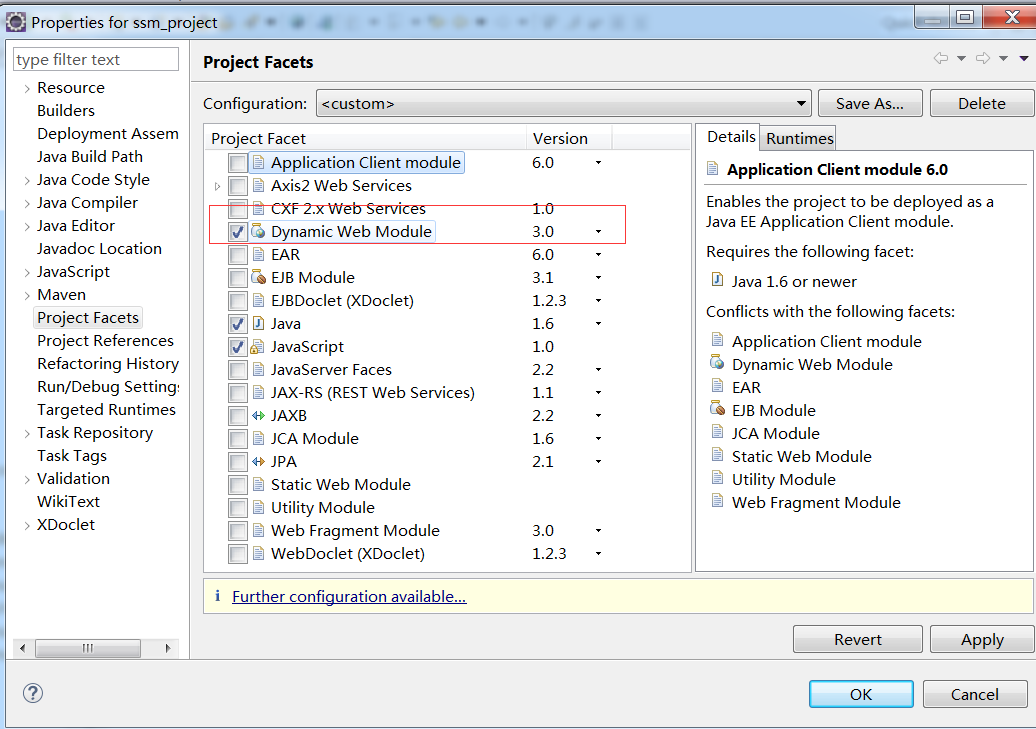
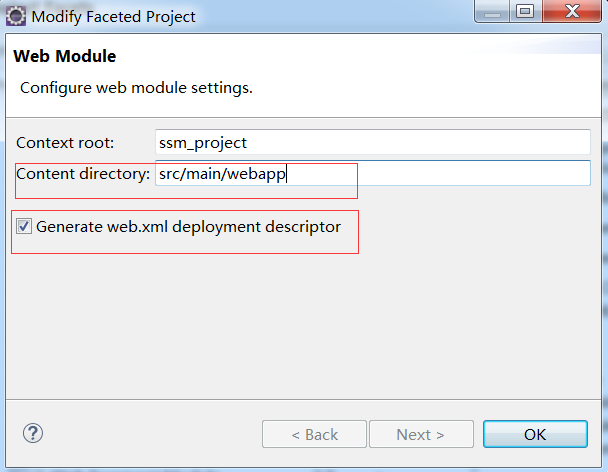
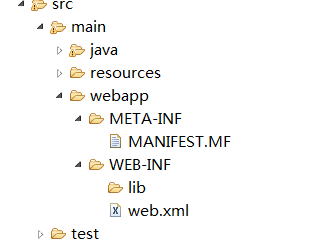
Configuration of Spring MVC
- <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
- <modelVersion>4.0.0</modelVersion>
- <groupId>com.lin</groupId>
- <artifactId>ssm_project</artifactId>
- <version>0.0.1-SNAPSHOT</version>
- <packaging>war</packaging>
- <properties>
- <!-- spring version number -->
- <spring.version>3.2.8.RELEASE</spring.version>
- <!-- log4j Log File Management Package Version -->
- <slf4j.version>1.6.6</slf4j.version>
- <log4j.version>1.2.12</log4j.version>
- <!-- junit version number -->
- <junit.version>4.10</junit.version>
- <!-- mybatis version number -->
- <mybatis.version>3.2.1</mybatis.version>
- </properties>
- <dependencies>
- <!-- Add to Spring rely on -->
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-core</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-webmvc</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-context</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-context-support</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-aop</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-aspects</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-tx</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-jdbc</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-web</artifactId>
- <version>${spring.version}</version>
- </dependency>
- <!--Unit test dependencies -->
- <dependency>
- <groupId>junit</groupId>
- <artifactId>junit</artifactId>
- <version>${junit.version}</version>
- <scope>test</scope>
- </dependency>
- <!-- Log File Management Package -->
- <!-- log start -->
- <dependency>
- <groupId>log4j</groupId>
- <artifactId>log4j</artifactId>
- <version>${log4j.version}</version>
- </dependency>
- <dependency>
- <groupId>org.slf4j</groupId>
- <artifactId>slf4j-api</artifactId>
- <version>${slf4j.version}</version>
- </dependency>
- <dependency>
- <groupId>org.slf4j</groupId>
- <artifactId>slf4j-log4j12</artifactId>
- <version>${slf4j.version}</version>
- </dependency>
- <!-- log end -->
- <!--spring Unit test dependencies -->
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-test</artifactId>
- <version>${spring.version}</version>
- <scope>test</scope>
- </dependency>
- <!--mybatis rely on -->
- <dependency>
- <groupId>org.mybatis</groupId>
- <artifactId>mybatis</artifactId>
- <version>${mybatis.version}</version>
- </dependency>
- <!-- mybatis/spring package -->
- <dependency>
- <groupId>org.mybatis</groupId>
- <artifactId>mybatis-spring</artifactId>
- <version>1.2.0</version>
- </dependency>
- <!-- mysql Driving package -->
- <dependency>
- <groupId>mysql</groupId>
- <artifactId>mysql-connector-java</artifactId>
- <version>5.1.29</version>
- </dependency>
- <!-- javaee-api package Attention and project usage JDK Version correspondence -->
- <dependency>
- <groupId>javax</groupId>
- <artifactId>javaee-api</artifactId>
- <version>6.0</version>
- <scope>provided</scope>
- </dependency>
- <!-- javaee-web-api package Attention and project usage JDK Version correspondence -->
- <dependency>
- <groupId>javax</groupId>
- <artifactId>javaee-web-api</artifactId>
- <version>6.0</version>
- <scope>provided</scope>
- </dependency>
- </dependencies>
- </project>
In fact, it adds the following two<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.lin</groupId> <artifactId>ssm_project</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <properties> <!-- spring version number --> <spring.version>3.2.8.RELEASE</spring.version> <!-- log4j Log File Management Package Version --> <slf4j.version>1.6.6</slf4j.version> <log4j.version>1.2.12</log4j.version> <!-- junit version number --> <junit.version>4.10</junit.version> <!-- mybatis version number --> <mybatis.version>3.2.1</mybatis.version> </properties> <dependencies> <!-- Add to Spring rely on --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-tx</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <!--Unit test dependencies --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> <!-- Log File Management Package --> <!-- log start --> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>${log4j.version}</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>${slf4j.version}</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>${slf4j.version}</version> </dependency> <!-- log end --> <!--spring Unit test dependencies --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>${spring.version}</version> <scope>test</scope> </dependency> <!--mybatis rely on --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>${mybatis.version}</version> </dependency> <!-- mybatis/spring package --> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>1.2.0</version> </dependency> <!-- mysql Driving package --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.29</version> </dependency> <!-- javaee-api Pack attention and project usage JDK Version correspondence --> <dependency> <groupId>javax</groupId> <artifactId>javaee-api</artifactId> <version>6.0</version> <scope>provided</scope> </dependency> <!-- javaee-web-api Pack attention and project usage JDK Version correspondence --> <dependency> <groupId>javax</groupId> <artifactId>javaee-web-api</artifactId> <version>6.0</version> <scope>provided</scope> </dependency> </dependencies> </project>
- <!-- javaee-api package Attention and project usage JDK Version correspondence -->
- <dependency>
- <groupId>javax</groupId>
- <artifactId>javaee-api</artifactId>
- <version>6.0</version>
- <scope>provided</scope>
- </dependency>
- <!-- javaee-web-api package Attention and project usage JDK Version correspondence -->
- <dependency>
- <groupId>javax</groupId>
- <artifactId>javaee-web-api</artifactId>
- <version>6.0</version>
- <scope>provided</scope>
- </dependency>
<!-- javaee-api Pack attention and project usage JDK Version correspondence --> <dependency> <groupId>javax</groupId> <artifactId>javaee-api</artifactId> <version>6.0</version> <scope>provided</scope> </dependency> <!-- javaee-web-api Pack attention and project usage JDK Version correspondence --> <dependency> <groupId>javax</groupId> <artifactId>javaee-web-api</artifactId> <version>6.0</version> <scope>provided</scope> </dependency>
- <?xml version="1.0" encoding="UTF-8"?>
- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:p="http://www.springframework.org/schema/p"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns:context="http://www.springframework.org/schema/context"
- xmlns:mvc="http://www.springframework.org/schema/mvc"
- xsi:schemaLocation="
- http://www.springframework.org/schema/beans
- http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
- http://www.springframework.org/schema/context
- http://www.springframework.org/schema/context/spring-context-3.2.xsd
- http://www.springframework.org/schema/mvc
- http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd">
- <!-- scanning controller(controller Layer injection) -->
- <context:component-scan base-package="com.lin.controller"/>
- <!-- Adding prefixes and suffixes to model views -->
- <bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"
- p:prefix="/WEB-INF/view/" p:suffix=".jsp"/>
- </beans>
(3) configure web,xml<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:p="http://www.springframework.org/schema/p" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd"> <!-- scanning controller(controller Layer injection) --> <context:component-scan base-package="com.lin.controller"/> <!-- Adding prefixes and suffixes to model views --> <bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver" p:prefix="/WEB-INF/view/" p:suffix=".jsp"/> </beans>
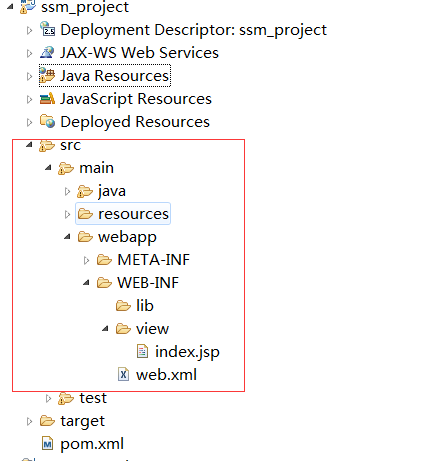
- <?xml version="1.0" encoding="UTF-8"?>
- <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
- xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
- id="WebApp_ID" version="2.5">
- <display-name>Archetype Created Web Application</display-name>
- <!-- Initial Welcome Interface -->
- <welcome-file-list>
- <welcome-file>index.jsp</welcome-file>
- </welcome-file-list>
- <!-- read spring configuration file -->
- <context-param>
- <param-name>contextConfigLocation</param-name>
- <param-value>classpath:application.xml</param-value>
- </context-param>
- <!-- Design Path Variable Value -->
- <context-param>
- <param-name>webAppRootKey</param-name>
- <param-value>springmvc.root</param-value>
- </context-param>
- <!-- Spring Character Set Filter -->
- <filter>
- <filter-name>SpringEncodingFilter</filter-name>
- <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
- <init-param>
- <param-name>encoding</param-name>
- <param-value>UTF-8</param-value>
- </init-param>
- <init-param>
- <param-name>forceEncoding</param-name>
- <param-value>true</param-value>
- </init-param>
- </filter>
- <filter-mapping>
- <filter-name>SpringEncodingFilter</filter-name>
- <url-pattern>/*</url-pattern>
- </filter-mapping>
- <!-- Log record -->
- <context-param>
- <!-- Log Profile Path -->
- <param-name>log4jConfigLocation</param-name>
- <param-value>classpath:log4j.properties</param-value>
- </context-param>
- <context-param>
- <!-- Refresh intervals for log pages -->
- <param-name>log4jRefreshInterval</param-name>
- <param-value>6000</param-value>
- </context-param>
- <listener>
- <listener-class>org.springframework.web.util.Log4jConfigListener</listener-class>
- </listener>
- <listener>
- <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
- </listener>
- <!-- springMVC Core configuration -->
- <servlet>
- <servlet-name>dispatcherServlet</servlet-name>
- <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
- <init-param>
- <param-name>contextConfigLocation</param-name>
- <!--spingMVC Configuration path -->
- <param-value>classpath:springmvc/spring-mvc.xml</param-value>
- </init-param>
- <load-on-startup>1</load-on-startup>
- </servlet>
- <!-- Interception settings -->
- <servlet-mapping>
- <servlet-name>dispatcherServlet</servlet-name>
- <url-pattern>/</url-pattern>
- </servlet-mapping>
- <!-- Error jump page -->
- <error-page>
- <!-- Path incorrect -->
- <error-code>404</error-code>
- <location>/WEB-INF/errorpage/404.jsp</location>
- </error-page>
- <error-page>
- <!-- Access is prohibited without access rights -->
- <error-code>405</error-code>
- <location>/WEB-INF/errorpage/405.jsp</location>
- </error-page>
- <error-page>
- <!-- internal error -->
- <error-code>500</error-code>
- <location>/WEB-INF/errorpage/500.jsp</location>
- </error-page>
- </web-app>
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"> <display-name>Archetype Created Web Application</display-name> <!-- Initial Welcome Interface --> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <!-- read spring configuration file --> <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:application.xml</param-value> </context-param> <!-- Design Path Variable Value --> <context-param> <param-name>webAppRootKey</param-name> <param-value>springmvc.root</param-value> </context-param> <!-- Spring Character Set Filter --> <filter> <filter-name>SpringEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> <init-param> <param-name>forceEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>SpringEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!-- Log record --> <context-param> <!-- Log Profile Path --> <param-name>log4jConfigLocation</param-name> <param-value>classpath:log4j.properties</param-value> </context-param> <context-param> <!-- Refresh intervals for log pages --> <param-name>log4jRefreshInterval</param-name> <param-value>6000</param-value> </context-param> <listener> <listener-class>org.springframework.web.util.Log4jConfigListener</listener-class> </listener> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <!-- springMVC Core configuration --> <servlet> <servlet-name>dispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <!--spingMVC Configuration path --> <param-value>classpath:springmvc/spring-mvc.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <!-- Interception settings --> <servlet-mapping> <servlet-name>dispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> <!-- Error jump page --> <error-page> <!-- Path incorrect --> <error-code>404</error-code> <location>/WEB-INF/errorpage/404.jsp</location> </error-page> <error-page> <!-- Access is prohibited without access rights --> <error-code>405</error-code> <location>/WEB-INF/errorpage/405.jsp</location> </error-page> <error-page> <!-- internal error --> <error-code>500</error-code> <location>/WEB-INF/errorpage/500.jsp</location> </error-page> </web-app>
(4) Add index.jsp
- <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
- <html>
- <body>
- <h2>Hello World!</h2>
- ${user.userId}<br>
- ${user.userName}<br>
- ${user.userPassword}<br>
- ${user.userEmail}<br>
- </body>
- </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <html> <body> <h2>Hello World!</h2> ${user.userId}<br> ${user.userName}<br> ${user.userPassword}<br> ${user.userEmail}<br> </body> </html>
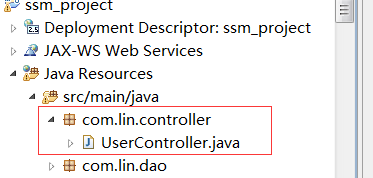
- package com.lin.controller;
- import javax.annotation.Resource;
- import org.springframework.stereotype.Controller;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.servlet.ModelAndView;
- import com.lin.domain.User;
- import com.lin.service.UserService;
- /**
- * Functional summary: User Controller
- *
- * @author linbingwen
- * @since 2015 28 September
- */
- @Controller
- public class UserController {
- @Resource
- private UserService userService;
- @RequestMapping("/")
- public ModelAndView getIndex(){
- ModelAndView mav = new ModelAndView("index");
- User user = userService.selectUserById(1);
- mav.addObject("user", user);
- return mav;
- }
- }
package com.lin.controller; import javax.annotation.Resource; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.servlet.ModelAndView; import com.lin.domain.User; import com.lin.service.UserService; /** * Functional summary: User Controller * * @author linbingwen * @since 2015 September 28th 2013 */ @Controller public class UserController { @Resource private UserService userService; @RequestMapping("/") public ModelAndView getIndex(){ ModelAndView mav = new ModelAndView("index"); User user = userService.selectUserById(1); mav.addObject("user", user); return mav; } }
(6) Final operation!
Finally, it's the result. It's all configured. We can start running as a web project!
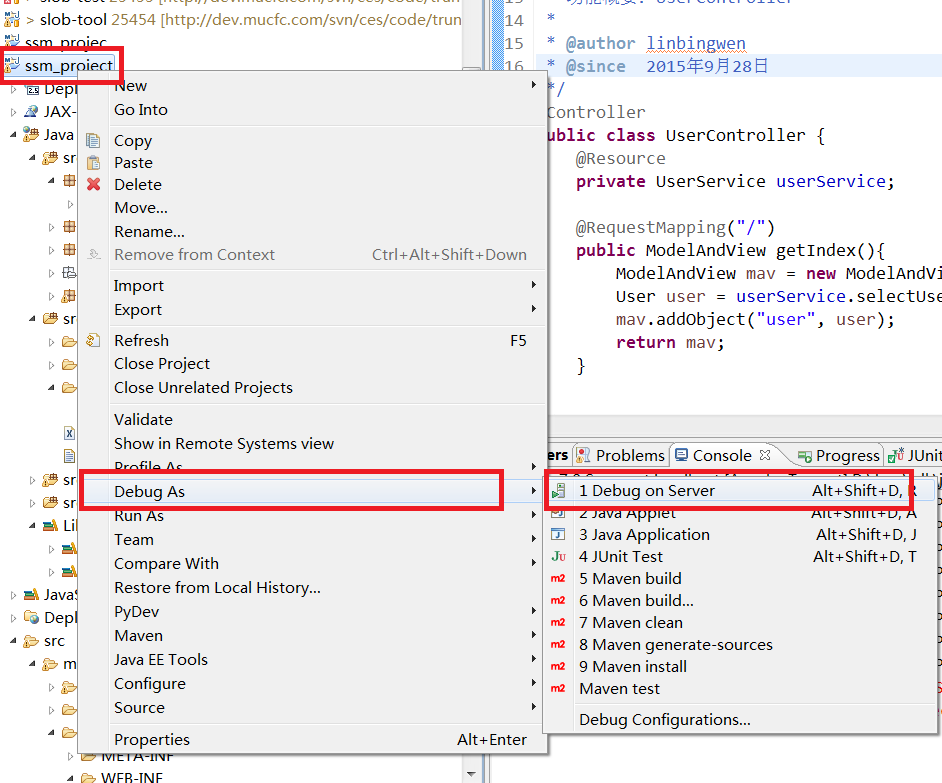
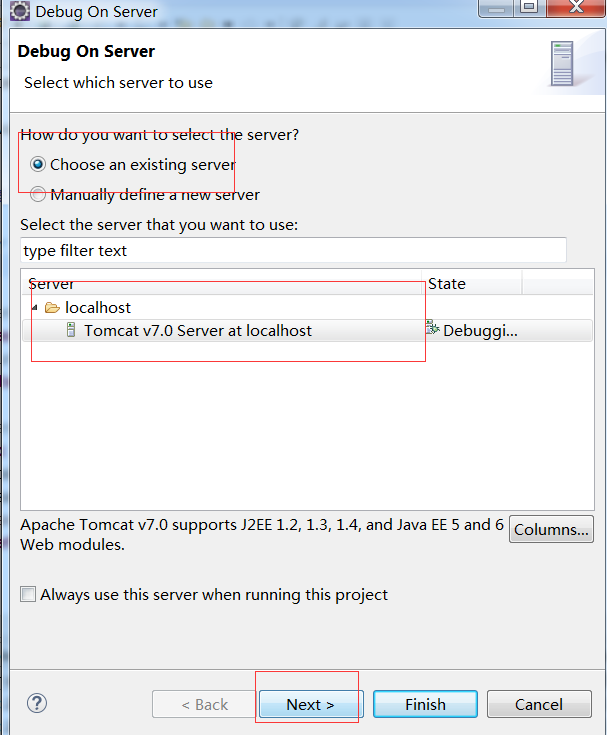
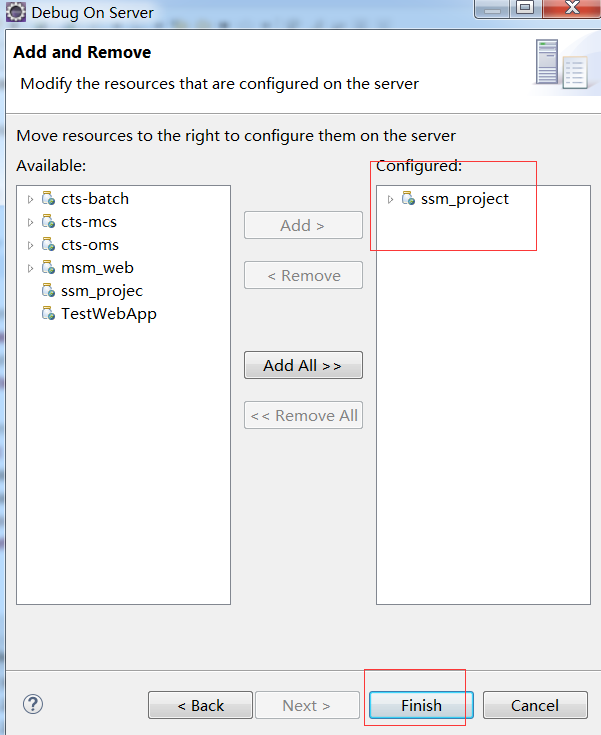
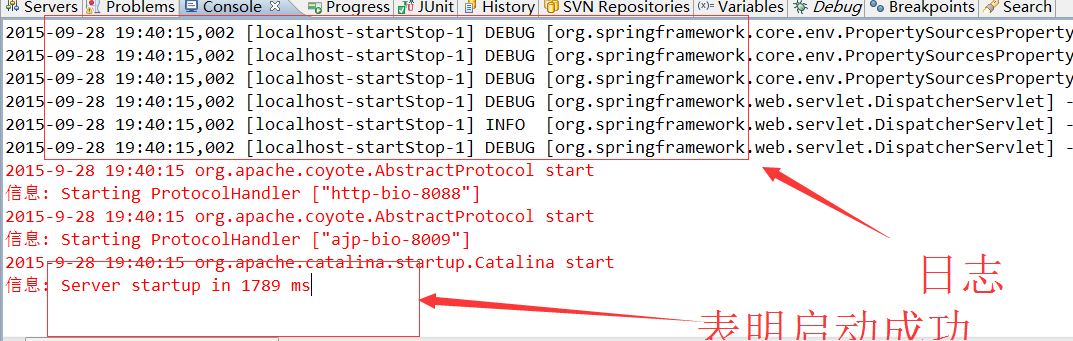
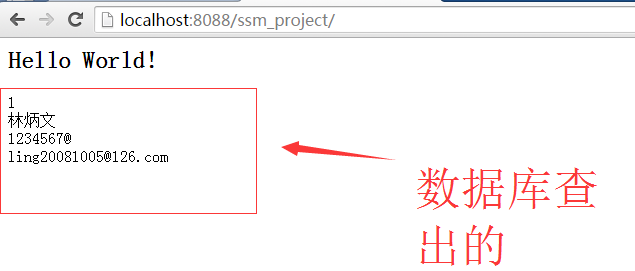
- Last article An Example of Spring+Mybatis+Maven+MySql Construction
- Next article Jquery introductory tutorial
My Similar Articles
- •Detailed Interpretation of Shrio Landing Verification Examples 2015-12-06 Reading 5539
- •An Example of Spring+Mybatis+Maven+MySql Construction 2015-09-29 Reading 4377
- •Mybatis automatically generates code 2015-07-23 Reading 3729
- •Mybatis+Spring Integration Creating Web Projects 2015-05-15 Reading 4533
- •One-to-one and one-to-many implementation of Mybatis associated query 2015-05-13 Reading 8137
- •Spring+Mybatis+Spring MVC Background and Front Page Display Example (attached project) 2015-10-27 Reading 14104
- •Ajax+Spring MVC+Spring+Mybatis+MySql+js User Registration Example 2015-07-23 Reading 7371
- •MyBatis dynamic Sql statement 2015-07-09 Reading 5420
- •Mybatis additions, deletions, modifications and multi-parameter list queries based on annotations 2015-05-15 Reading 4695
- •Implementation of Mybatis Multi-parameter Query and List Query in Different Ways 2015-05-13 Reading 4617