1, Purpose
The purpose of encapsulating request parameters is for unification and convenience. To put it bluntly, if only one attribute is queried and one input parameter is passed, it is no problem. If an object has 100 attributes and multiple parameter associations are required for query, it needs to be unified and convenient for management. Simply put, passing the object is over, ha ha ha.
2, Actual case
For example, I want to make a fuzzy query and query by name.
1. Interface modification
The query interface is transformed. The example code is as follows:
package com.rongrong.wiki.controller; import com.rongrong.wiki.domain.EBook; import com.rongrong.wiki.resp.CommonResp; import com.rongrong.wiki.service.EBookService; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import javax.annotation.Resource; import java.util.List; /** * @author longrong.lang * @version 1.0 * @description */ @RestController @RequestMapping("/ebook") public class EBookController { @Resource private EBookService eBookService; @GetMapping("/list") public CommonResp list(String name) { CommonResp<List<EBook>> resp = new CommonResp<>(); List<EBook> list = eBookService.list(name); resp.setMessage("Query executed successfully!"); resp.setContent(list); return resp; } }
2. Transformation from Service layer
First, I'll add an input parameter from Service, such as Name, which is what we often say about querying by keyword. From the perspective of Sql, it's like. I won't talk nonsense. It's a little inky. The example code is as follows:
package com.rongrong.wiki.service; import com.rongrong.wiki.domain.EBook; import com.rongrong.wiki.domain.EBookExample; import com.rongrong.wiki.mapper.EBookMapper; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.List; /** * @author rongrong * @version 1.0 * @description * @date 2021/10/13 10:09 */ @Service public class EBookService { @Resource private EBookMapper eBookMapper; public List<EBook> list(String name) { EBookExample eBookExample = new EBookExample(); //The meaning of the code here is equivalent to making a Sql where condition EBookExample.Criteria criteria = eBookExample.createCriteria(); criteria.andNameLike("%"+name+"%"); return eBookMapper.selectByExample(eBookExample); } }
3. Interface modification test
Query results:
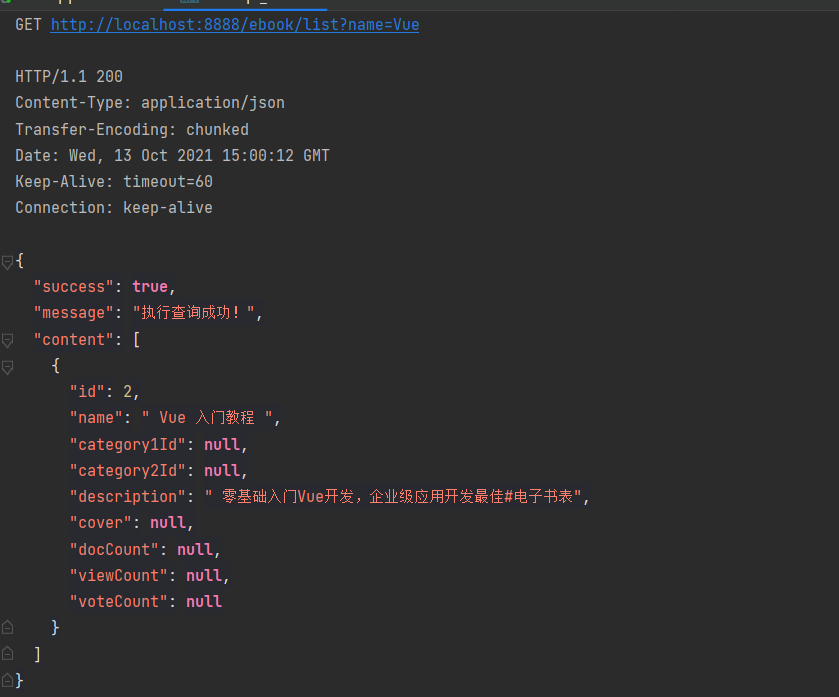
3, Demand change
Now I want to query through ID and Name, or multiple parameters. What should I do?
1. Structure unified input parameter
It's very simple. Just pass a class (object). Let's start with a unified input parameter structure. Take two parameters as input parameters as an example. The example code is as follows:
package com.rongrong.wiki.req; public class EBookReq { private Long id; private String name; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Override public String toString() { StringBuilder sb = new StringBuilder(); sb.append(getClass().getSimpleName()); sb.append(" ["); sb.append("Hash = ").append(hashCode()); sb.append(", id=").append(id); sb.append(", name=").append(name); sb.append("]"); return sb.toString(); } }
2. Construct unified return
Why construct uniform return information?
For example, if we log in successfully, we can't return the password field to the user. I don't think it's professional. Normally, we can only return a few fields, so we have a unified return information. The example code is as follows:
package com.rongrong.wiki.resp; public class EBookResp { private Long id; private String name; private Long category1Id; private Long category2Id; private String description; private String cover; private Integer docCount; private Integer viewCount; private Integer voteCount; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Long getCategory1Id() { return category1Id; } public void setCategory1Id(Long category1Id) { this.category1Id = category1Id; } public Long getCategory2Id() { return category2Id; } public void setCategory2Id(Long category2Id) { this.category2Id = category2Id; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public String getCover() { return cover; } public void setCover(String cover) { this.cover = cover; } public Integer getDocCount() { return docCount; } public void setDocCount(Integer docCount) { this.docCount = docCount; } public Integer getViewCount() { return viewCount; } public void setViewCount(Integer viewCount) { this.viewCount = viewCount; } public Integer getVoteCount() { return voteCount; } public void setVoteCount(Integer voteCount) { this.voteCount = voteCount; } @Override public String toString() { StringBuilder sb = new StringBuilder(); sb.append(getClass().getSimpleName()); sb.append(" ["); sb.append("Hash = ").append(hashCode()); sb.append(", id=").append(id); sb.append(", name=").append(name); sb.append(", category1Id=").append(category1Id); sb.append(", category2Id=").append(category2Id); sb.append(", description=").append(description); sb.append(", cover=").append(cover); sb.append(", docCount=").append(docCount); sb.append(", viewCount=").append(viewCount); sb.append(", voteCount=").append(voteCount); sb.append("]"); return sb.toString(); } }
3. Interface modification
The example code is as follows:
package com.rongrong.wiki.controller; import com.rongrong.wiki.req.EBookReq; import com.rongrong.wiki.resp.CommonResp; import com.rongrong.wiki.resp.EBookResp; import com.rongrong.wiki.service.EBookService; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import javax.annotation.Resource; import java.util.List; /** * @author longrong.lang * @version 1.0 * @description */ @RestController @RequestMapping("/ebook") public class EBookController { @Resource private EBookService eBookService; @GetMapping("/list") public CommonResp list(EBookReq eBookReq) { CommonResp<List<EBookResp>> resp = new CommonResp<>(); List<EBookResp> list = eBookService.list(eBookReq); resp.setMessage("Query executed successfully!"); resp.setContent(list); return resp; } }
4. Service layer transformation
The example code is as follows:
package com.rongrong.wiki.service; import com.rongrong.wiki.domain.EBook; import com.rongrong.wiki.domain.EBookExample; import com.rongrong.wiki.mapper.EBookMapper; import com.rongrong.wiki.req.EBookReq; import com.rongrong.wiki.resp.EBookResp; import org.springframework.beans.BeanUtils; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.ArrayList; import java.util.List; /** * @author rongrong * @version 1.0 * @description * @date 2021/10/13 10:09 */ @Service public class EBookService { @Resource private EBookMapper eBookMapper; public List<EBookResp> list(EBookReq eBookReq) { EBookExample eBookExample = new EBookExample(); //The meaning of the code here is equivalent to making a Sql where condition EBookExample.Criteria criteria = eBookExample.createCriteria(); criteria.andNameLike("%"+eBookReq.getName()+"%"); List<EBook> eBookList = eBookMapper.selectByExample(eBookExample); List<EBookResp> eBookRespList = new ArrayList<>(); for (EBook eBook: eBookList) { EBookResp eBookResp = new EBookResp(); //The BeanUtils provided with spring boot completes the copy of the object BeanUtils.copyProperties(eBook, eBookResp); eBookResp.setId(12345L); eBookRespList.add(eBookResp); } return eBookRespList; } }
5. Test after interface modification
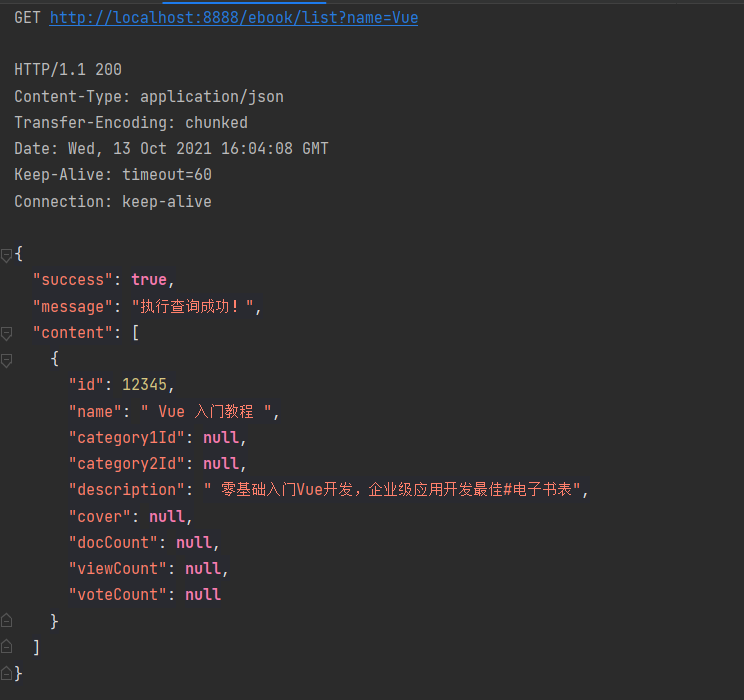
6. Reconstruction of Service floor
CopyUtil tool class. The example code is as follows:
package com.rongrong.wiki.util; import org.springframework.beans.BeanUtils; import org.springframework.util.CollectionUtils; import java.util.ArrayList; import java.util.List; public class CopyUtil { /** * Monomer replication */ public static <T> T copy(Object source, Class<T> clazz) { if (source == null) { return null; } T obj = null; try { obj = clazz.newInstance(); } catch (Exception e) { e.printStackTrace(); return null; } BeanUtils.copyProperties(source, obj); return obj; } /** * List copy */ public static <T> List<T> copyList(List source, Class<T> clazz) { List<T> target = new ArrayList<>(); if (!CollectionUtils.isEmpty(source)){ for (Object c: source) { T obj = copy(c, clazz); target.add(obj); } } return target; } }
Remodel the Service layer. The example code is as follows:
package com.rongrong.wiki.service; import com.rongrong.wiki.domain.EBook; import com.rongrong.wiki.domain.EBookExample; import com.rongrong.wiki.mapper.EBookMapper; import com.rongrong.wiki.req.EBookReq; import com.rongrong.wiki.resp.EBookResp; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.List; import static com.rongrong.wiki.util.CopyUtil.copyList; /** * @author rongrong * @version 1.0 * @description * @date 2021/10/13 10:09 */ @Service public class EBookService { @Resource private EBookMapper eBookMapper; public List<EBookResp> list(EBookReq eBookReq) { EBookExample eBookExample = new EBookExample(); //The meaning of the code here is equivalent to making a Sql where condition EBookExample.Criteria criteria = eBookExample.createCriteria(); criteria.andNameLike("%"+eBookReq.getName()+"%"); List<EBook> eBookList = eBookMapper.selectByExample(eBookExample); //List<EBookResp> eBookRespList = new ArrayList<>(); //for (EBook eBook: eBookList) { // //EBookResp eBookResp = new EBookResp(); // ////The BeanUtils provided with spring boot completes the copy of the object // //BeanUtils.copyProperties(eBook, eBookResp); // //eBookResp.setId(12345L); // //Monomer replication // EBookResp copy = copy(eBook, EBookResp.class); // eBookRespList.add(copy); //} //List copy List<EBookResp> respList = copyList(eBookList, EBookResp.class); return respList; } }
7. Test after interface modification
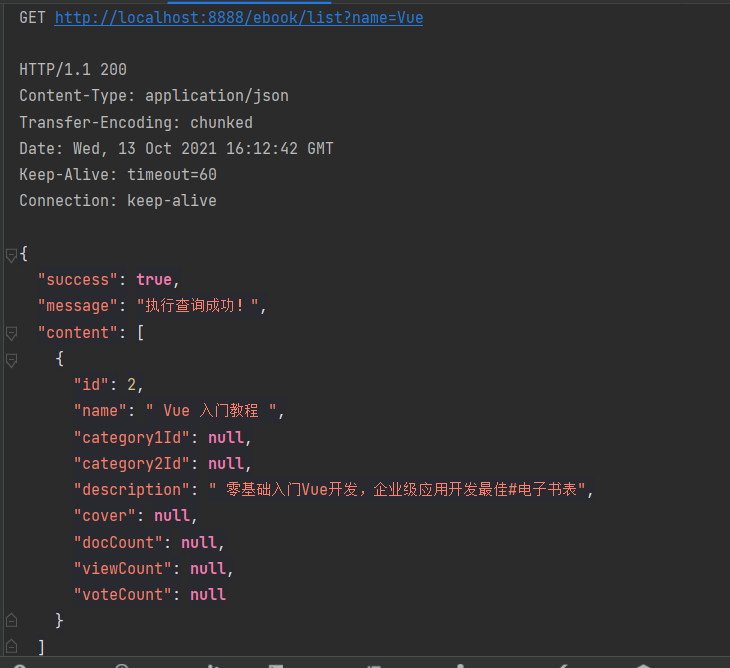