1 Introduction to this chapter
2 create a SpringBoot project
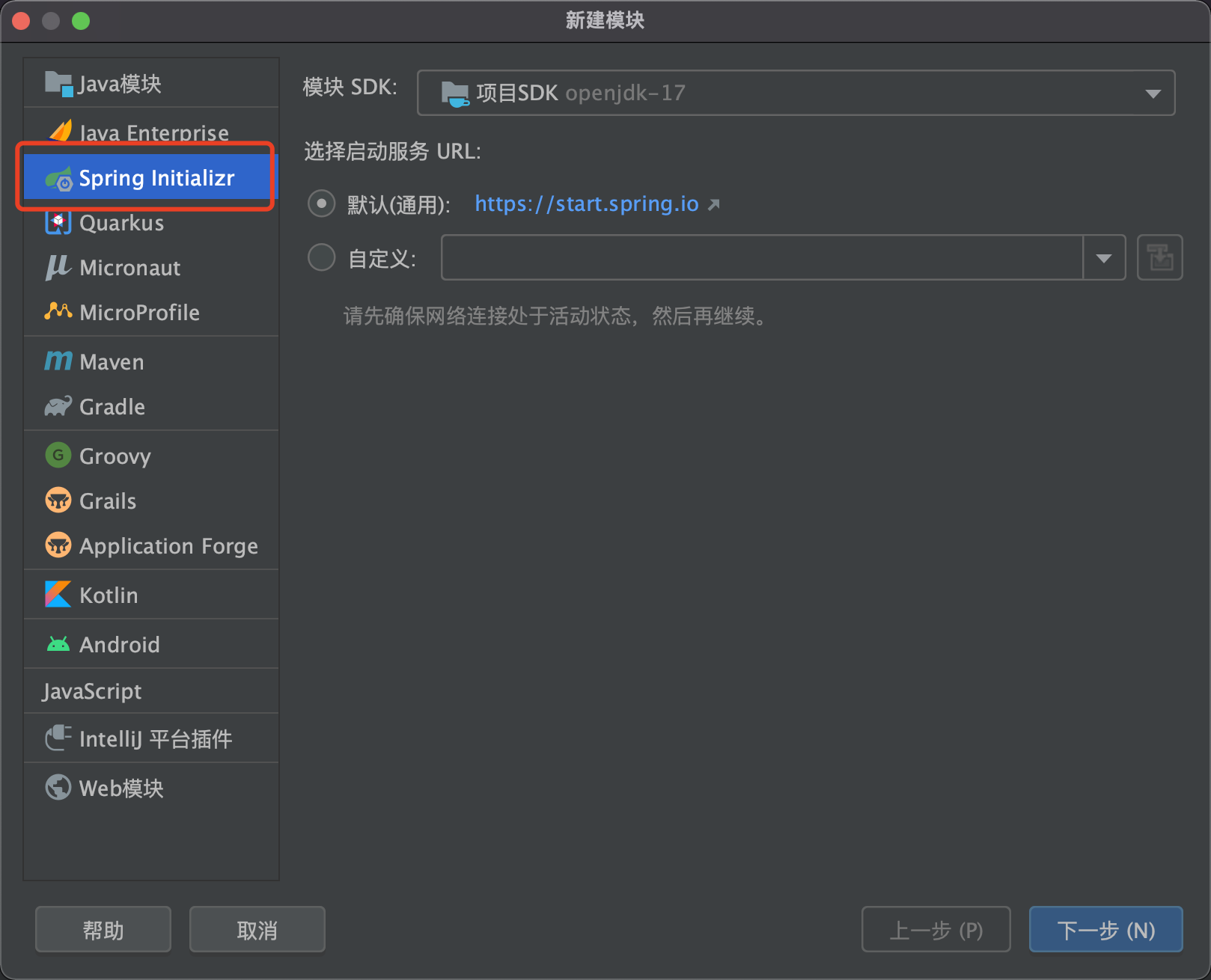
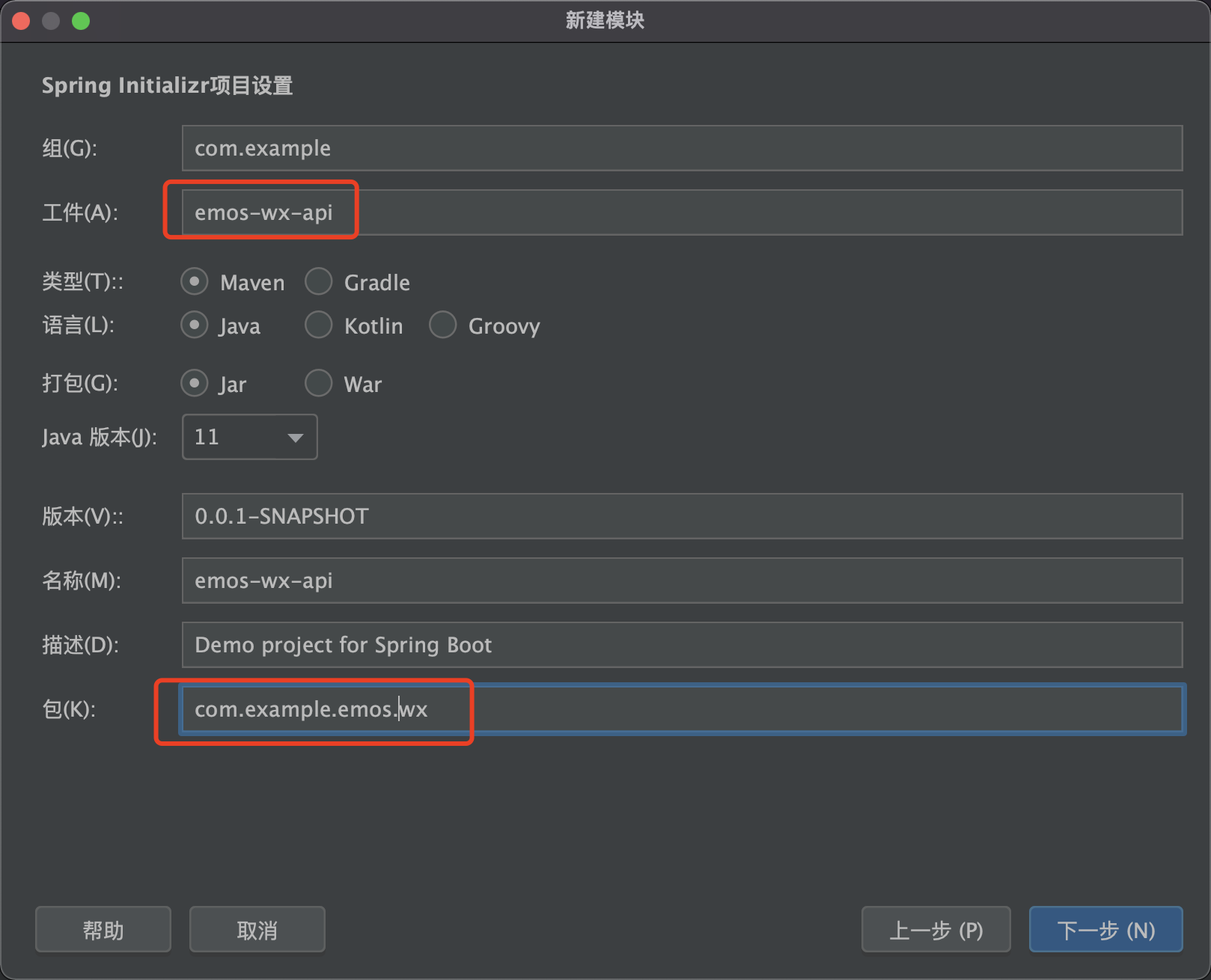
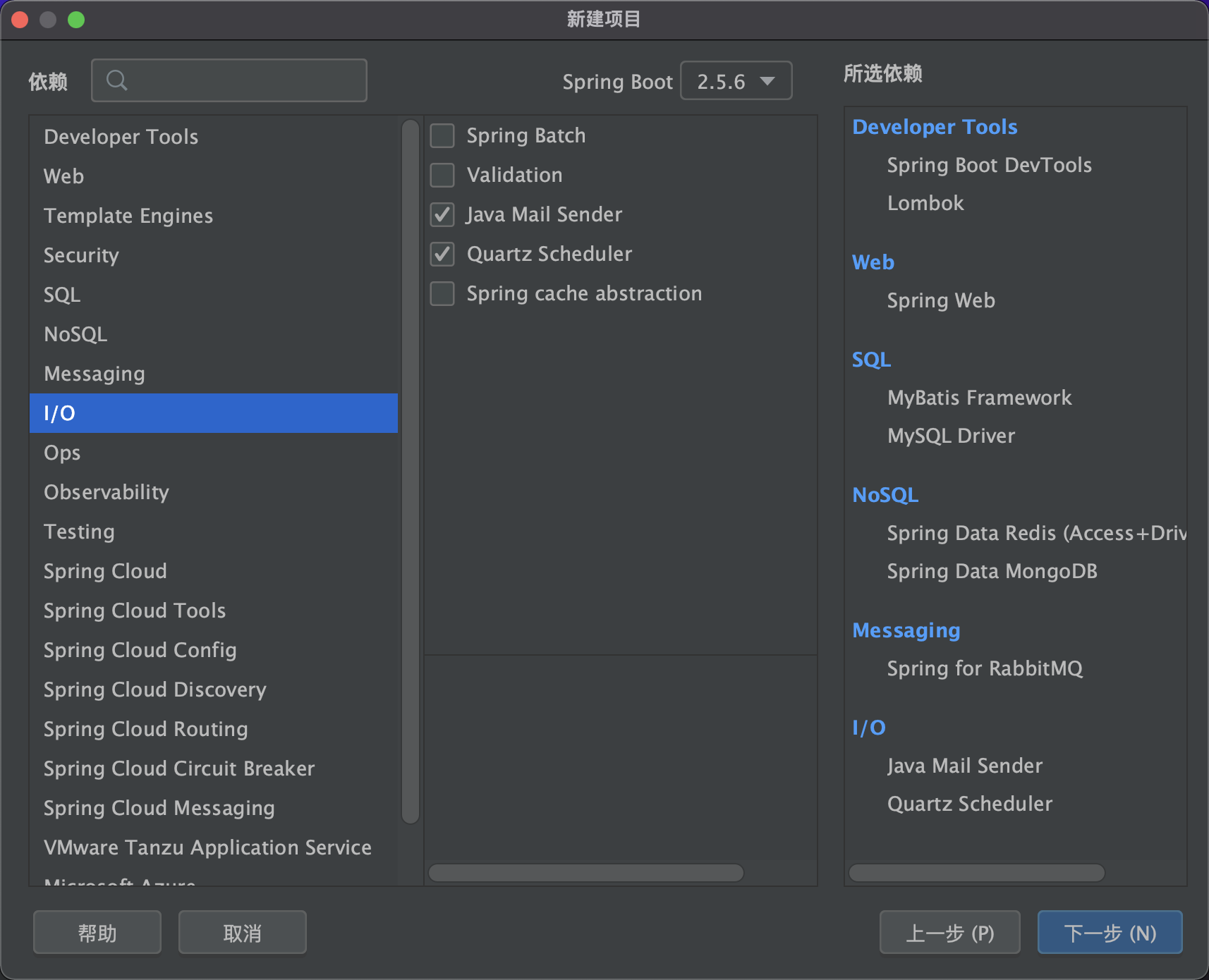
Change application.properties to application.yml
Configure tomcat
server: tomcat: uri-encoding: UTF-8 threads: max: 200 min-spare: 30 connection-timeout: 5000ms port: 8080 servlet: context-path: /emos-wx-api
Configure data connection pool
spring: datasource: type: com.alibaba.druid.pool.DruidDataSource druid: driver-class-name: com.mysql.cj.jdbc.Driver url: jdbc:mysql://localhost:3306/emos?useUnicode=true&characterEncoding=UTF-8&serverTimezone=Asia/Shanghai username: root password: 123456 initial-size: 8 max-active: 16 min-idle: 8 max-wait: 60000 test-while-idle: true test-on-borrow: false test-on-return: false
Add configuration in pom.xml
<dependency> <groupId>com.alibaba</groupId> <artifactId>druid-spring-boot-starter</artifactId> <version>1.2.8</version> </dependency>
Configure redis data source
redis: database: 0 host: localhost port: 6379 password: 123456 jedis: pool: max-active: 1000 max-wait: -1ms max-idle: 16 min-idle: 8
Configure mongo data source
data: mongodb: host: localhost port: 27017 database: emos authentication-database: admin username: admin password: 123456
3 configure MyBatis
3.1 create database connection
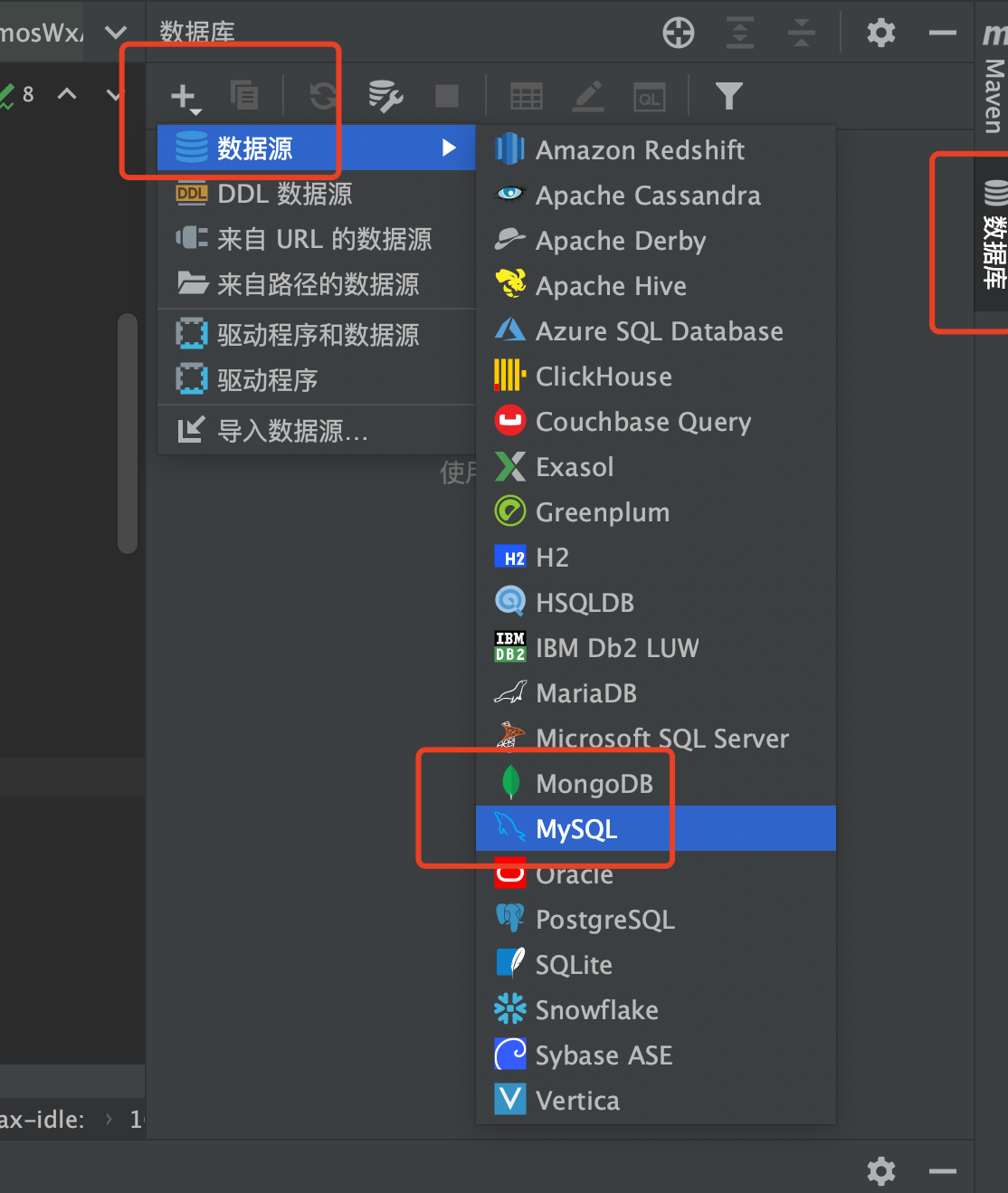
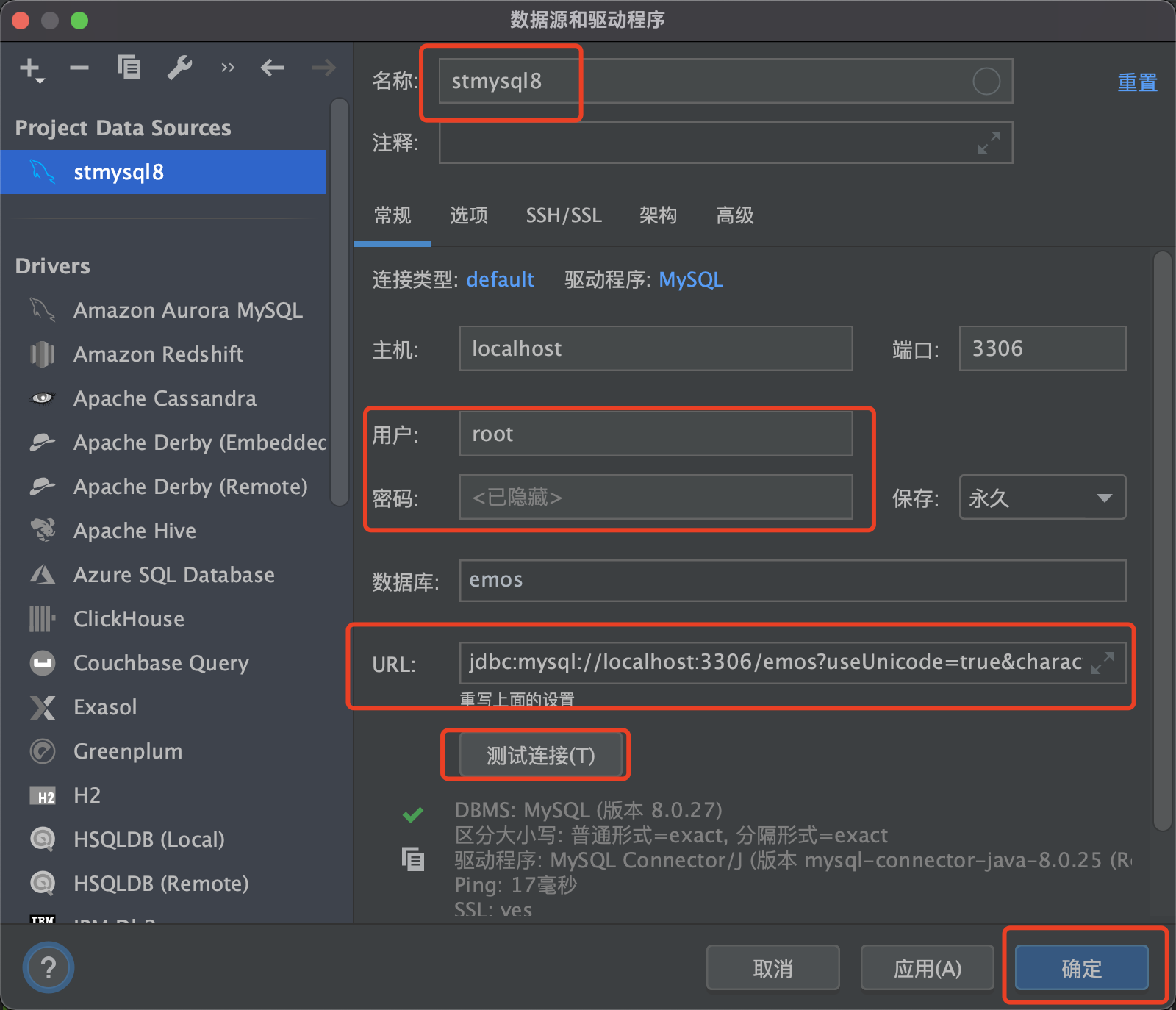
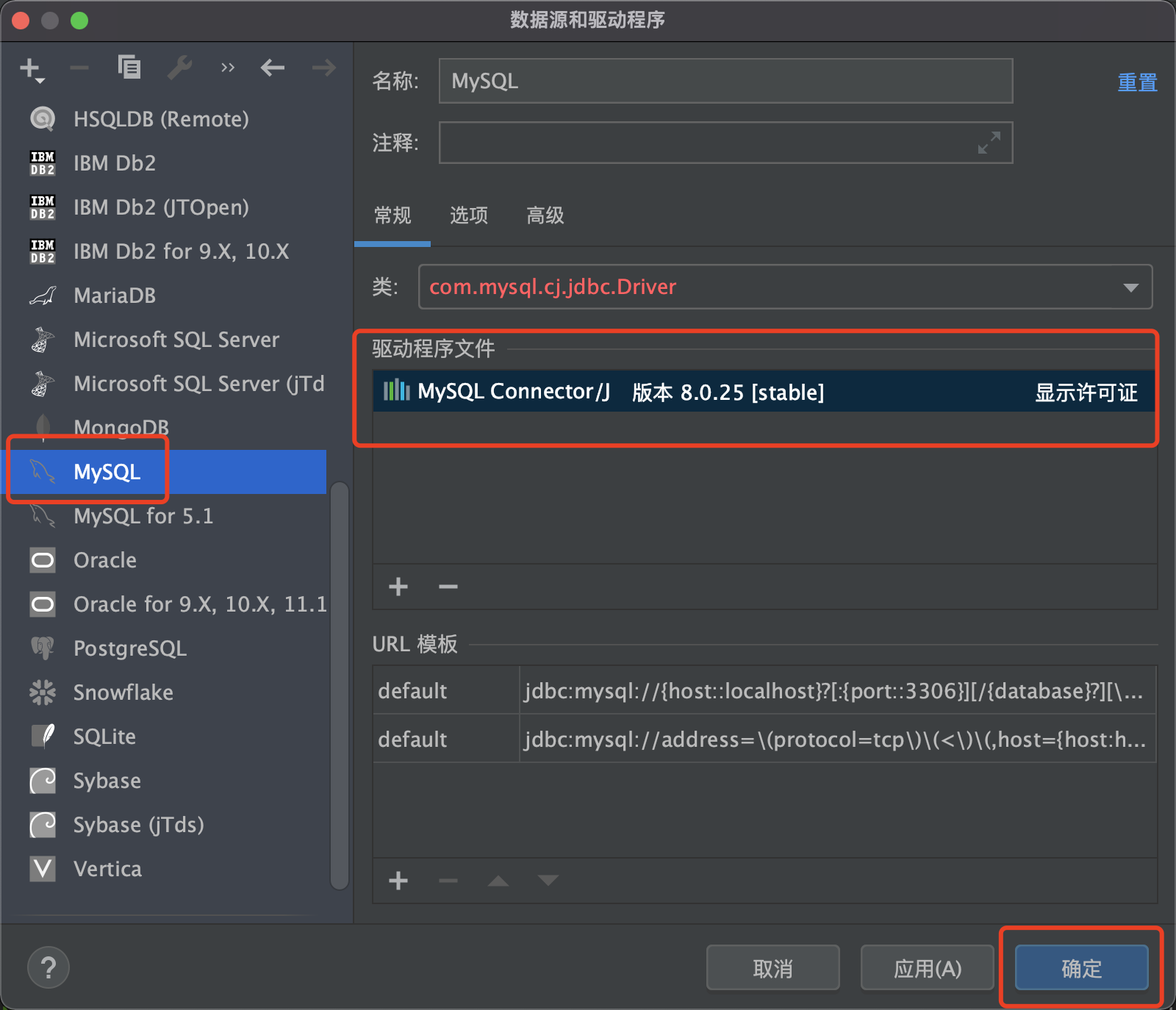
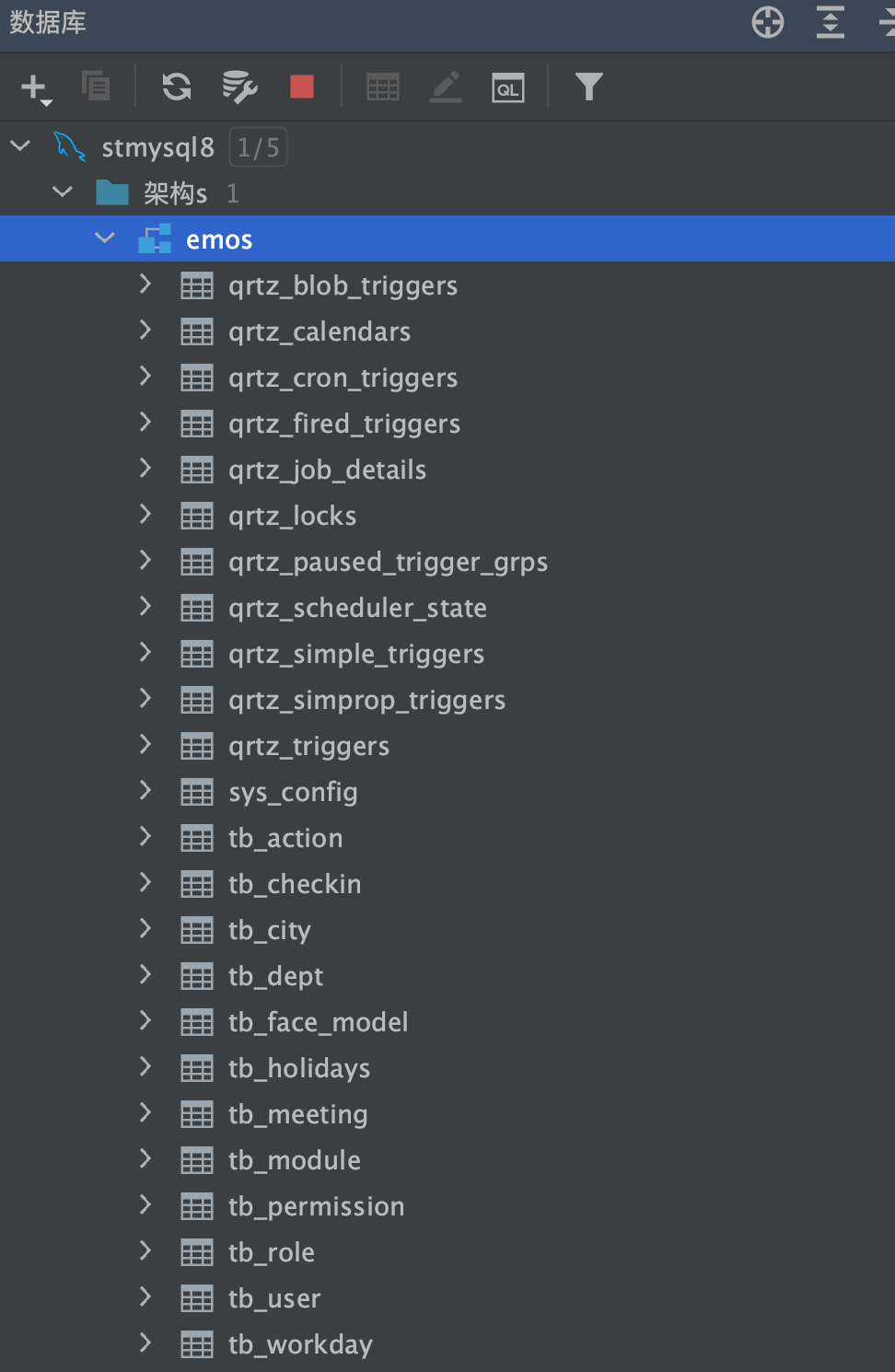
3.2 select the data table to generate MyBatis file
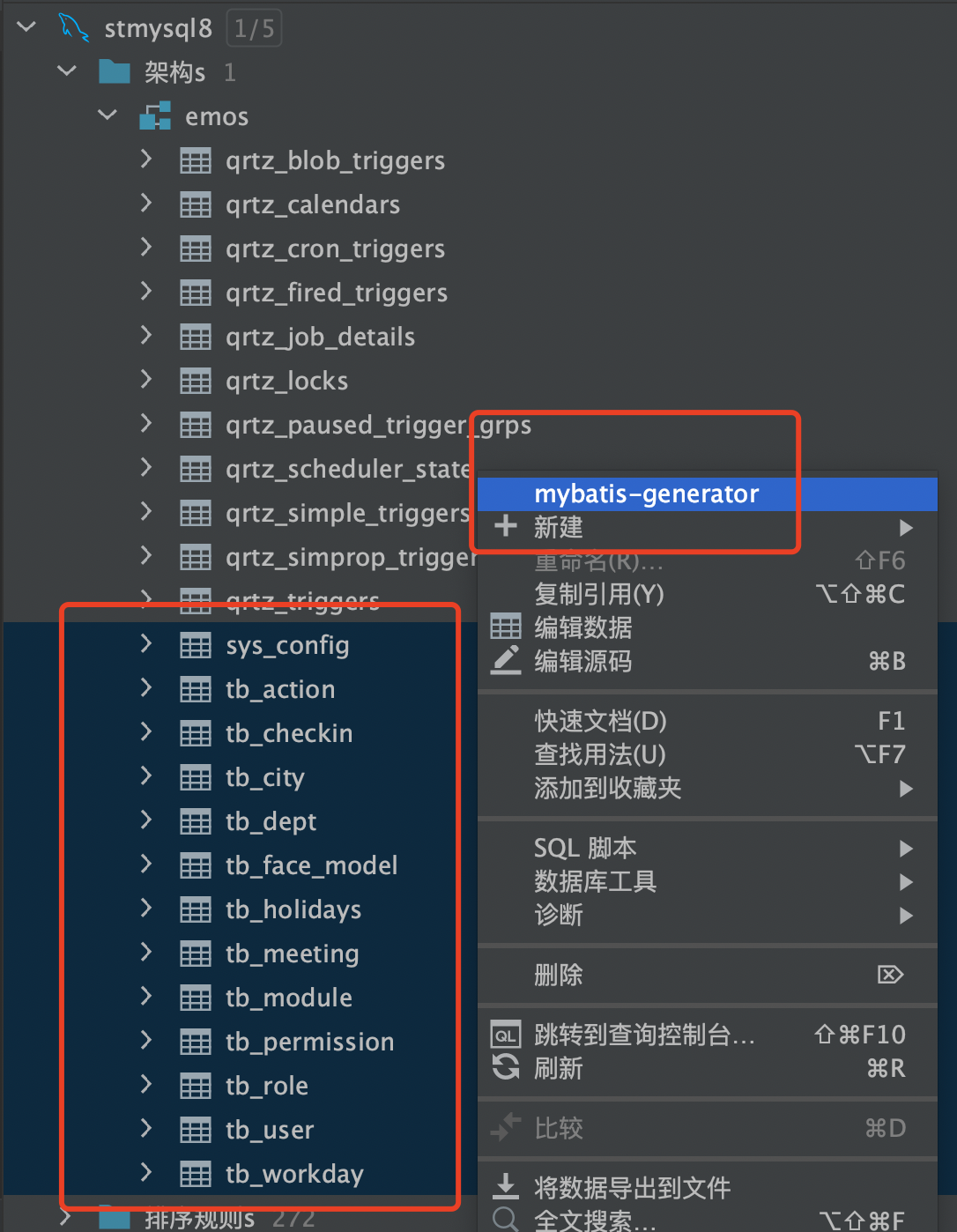
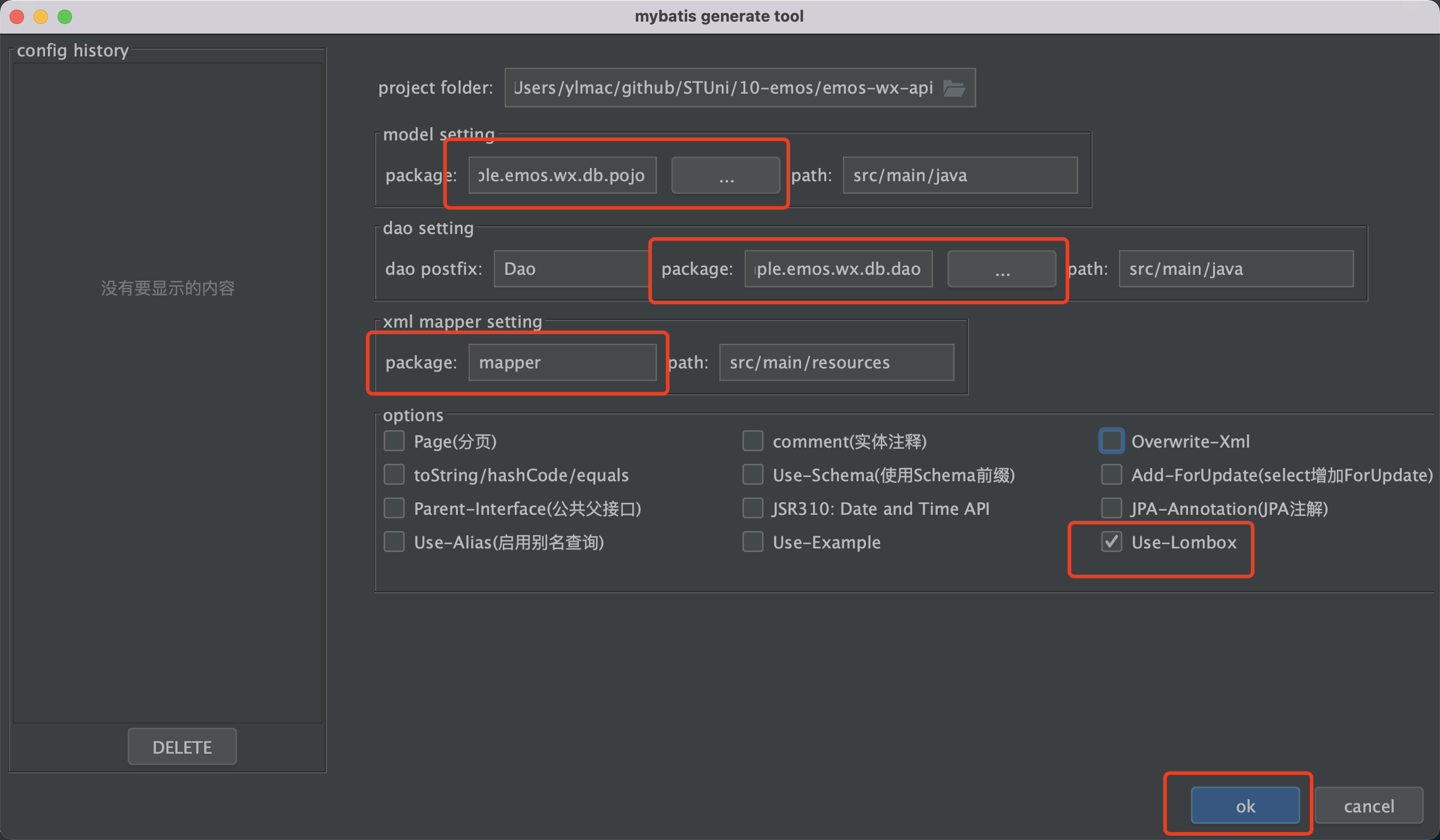
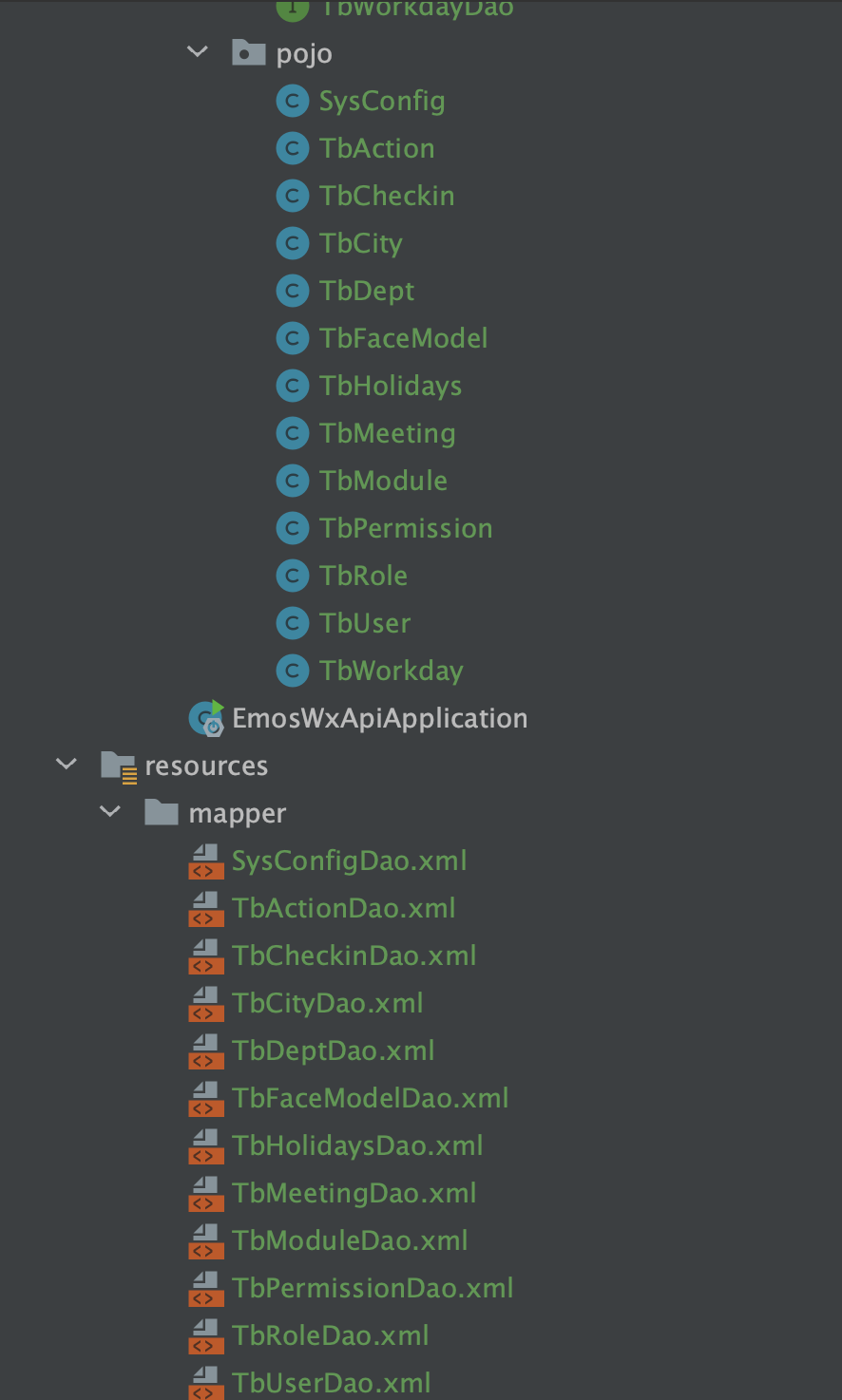
3.3 modify yml file and add MyBatis configuration information
mybatis: mapper-locations: classpath*:mapper/*.xml type-aliases-package: com.example.emos.wx.db.pojo configuration: log-impl: org.apache.ibatis.logging.stdout.StdOutImpl map-underscore-to-camel-case: true
3.4 setting log output
logging: level: root: info com.example.emos.wx.db.dao: warn pattern: console: "%d{HH:mm:ss} %-5level %msg%n"
4 create a custom exception class
- Why inherit RuntimeException?
- Exceptions of type Exception must be handled manually
- RuntimeException exceptions can be handled automatically or manually
- Included properties
- Status code
- Exception message
package com.example.emos.wx.exception; import lombok.Data; @Data public class EmosException extends RuntimeException { private String msg; private int code = 500; public EmosException(String msg) { super(msg); this.msg = msg; } public EmosException(String msg, Throwable e) { super(msg, e); this.msg = msg; } public EmosException(String msg, int code) { super(msg); this.msg = msg; this.code = code; } public EmosException(String msg, int code, Throwable e) { super(msg, e); this.msg = msg; this.code = code; } }
5 encapsulating Web return objects
- Java Web projects need a unified data return format
- Service status code
- Business message
- Business data
- Import httpcomponents Library
- Many HTTP status codes are defined
- It eliminates the need for us to customize the status code constant
- Class R inherits from HashMap
- Packaging method
- ok method
- error method
<dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpcore</artifactId> <version>4.4.14</version> </dependency>
package com.example.emos.wx.common.util; import org.apache.http.HttpStatus; import java.util.HashMap; import java.util.Map; public class R extends HashMap<String,Object> { public R(){ put("code", HttpStatus.SC_OK); put("msg","success"); } public R put(String key,Object value){ super.put(key,value); return this; } public static R ok(){ return new R(); } public static R ok(String msg){ R r=new R(); r.put("msg",msg); return r; } public static R ok(Map<String,Object> map){ R r=new R(); r.putAll(map); return r; } public static R error(int code,String msg){ R r=new R(); r.put("code",code); r.put("msg",msg); return r; } public static R error(String msg){ return error(HttpStatus.SC_INTERNAL_SERVER_ERROR,msg); } public static R error(){ return error(HttpStatus.SC_INTERNAL_SERVER_ERROR,"Unknown exception, please contact the administrator"); } }
6. Use Swagger to build REST API
6.1 Swagger2 is used in this course
6.2 adding dependent Libraries
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version>
6.3 configuring Swagger
- (ApiInfoBuilder) defines the basic information of Swagger page
- (Apis electorbuilder) which methods in classes will appear on Swagger
- Enable support for JWT
- List: what parameters do users need to enter
- AuthorizationScope []: scope of JWT authentication in Swagger
- List: scope of token
- List: token context
package com.example.emos.wx.config; public class SwaggerConfig {}
7 create Web method test Swagger
7.1 writing Web interface TestController
package com.example.emos.wx.controller; import com.example.emos.wx.common.util.R; import io.swagger.annotations.Api; import io.swagger.annotations.ApiOperation; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping("/test") @Api("test Web Interface") public class TestController { @PostMapping("/sayHello") @ApiOperation("The simplest test method") public R sayHello(){ return R.ok().put("message", "HelloWorld"); } }
7.2 class declarations should be annotated with @ API
7.3 the web method should be annotated with @ ApiOperation
Test connection:
http://127.0.0.1:8080/emos-wx-api/swagger-ui.html
8. Configure the backend authentication function
8.1 using the Validation Library
8.2 adding dependent Libraries
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-validation</artifactId> </dependency>
8.3 create Form class
- Class declares the @ ApiModel to be added
- Property declaration to add @ ApiModelProperty
- Attribute declaration to add validation annotation
- @NotNull
- @NotBlank
- @Min
- @Max
- @Range
- @Pattern
8.4 @ Valid annotation shall be used for validation data
package com.example.emos.wx.controller.form; import io.swagger.annotations.ApiModel; import io.swagger.annotations.ApiModelProperty; import lombok.Data; import javax.validation.constraints.NotBlank; import javax.validation.constraints.Pattern; @ApiModel @Data public class TestSayHelloForm { @NotBlank @Pattern(regexp = "^[\\u4e00-\\u9fa5]{2,15}$",message = "Does not conform to regular expression") @ApiModelProperty("Name: 2-15 chinese") private String name; }
9 defend against immediate cross site scripting (XSS) attacks
9.1 reasons
- XSS attack usually refers to injecting malicious instructions into web pages through clever methods by taking advantage of the vulnerability of data saved in the website system, and users will automatically execute malicious scripts when loading web pages.
- If hackers can execute javaScript on your browser, they can steal cookies or tokens
9.2 importing Hutool dependencies
<dependency> <groupId>cn.hutool</groupId> <artifactId>hutool-all</artifactId> <version>5.7.14</version> </dependency>
package com.example.emos.wx.config.xss; public class XssHttpServletRequestWrapper extends HttpServletRequestWrapper {} public class XssFilter implements Filter {}
Add @ ServletComponentScan to EmosWxApiApplication startup class
9.3 data escape in Http request
- catalog filter
- Method of overriding Http request
- HttpServletRequest is an interface that various server manufacturers will implement
- If we directly inherit the request parent class of each manufacturer, our program will be bound with the manufacturer
- HttpServletRequestWrapper class
- Decorator mode is used
- The decorator encapsulates the manufacturer's Request implementation class
- You can override the methods in the vendor request object by overriding the methods of the Wrapper class
- Create a filter and pass the Request object into the Wrapper object